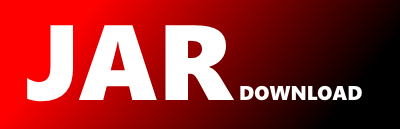
com.adobe.fontengine.inlineformatting.css20.CSS20FontSet Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
*
* File: CSSPDF16FontSet.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2006 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.inlineformatting.css20;
import java.io.Serializable;
import com.adobe.agl.util.ULocale;
import com.adobe.fontengine.font.Font;
import com.adobe.fontengine.font.FontException;
import com.adobe.fontengine.font.FontLoadingException;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.UnsupportedFontException;
import com.adobe.fontengine.fontmanagement.FontResolutionPriority;
import com.adobe.fontengine.inlineformatting.FallbackFontSet;
/**
* This interface provides access to the methods that a client can use to build a font set
* that is used by a {@link com.adobe.fontengine.inlineformatting.PDF16RichTextFormatter PDF16RichTextFormatter} object
* to resolve CSS font specifications into actual {@link com.adobe.fontengine.font.FontData} objects.
*
* CSS20FontSet objects implement the Serializable interface. The intent is that CSS20FontSets would generally
* not be entirely regenerated from scratch. Instead, they would be deserialized.
*
* Concurrency
*
* All classes that implement this interface are in general not guaranteed to be threadsafe. Specific
* implementations may offer tighter guarantees.
*/
public interface CSS20FontSet extends Serializable
{
/**
* Set the resolution mechanism that the
* {@link com.adobe.fontengine.inlineformatting.css20.CSS20FontSet CSS20FontSet} should use to decide which
* font should be chosen when two fonts appear identical under the CSS font selection
* algorithm. The default resolution priority if none is specified is
* {@link com.adobe.fontengine.fontmanagement.FontResolutionPriority#FIRST
* FontResolutionPriority#FIRST}
* {@link com.adobe.fontengine.fontmanagement.FontResolutionPriority#INTELLIGENT
* FontResolutionPriority#INTELLIGENT} specifies
* that an "intelligent" determination is made about the fonts and the one that "best"
* represents the CSS attributes where they collide is chosen.
*
{@link com.adobe.fontengine.fontmanagement.FontResolutionPriority#FIRST
* FontResolutionPriority#FIRST} specifies
* the first font added to the font set that matches the CSS attributes is chosen.
*
{@link com.adobe.fontengine.fontmanagement.FontResolutionPriority#LAST
* FontResolutionPriority#LAST} specifies
* the last font added to the font set that matches the CSS attributes is chosen.
* @param priority The resolution mechanism to use
* @return The old resolution priority setting.
*/
public FontResolutionPriority setResolutionPriority(FontResolutionPriority priority);
/**
* Adds a font to the set of fonts that are examined for resolving a set of
* CSS attributes into an actual font during the formatting process in
* {@link com.adobe.fontengine.inlineformatting.PDF16RichTextFormatter#format(com.adobe.fontengine.inlineformatting.AttributedRun, int, int)}
* or {@link com.adobe.fontengine.inlineformatting.PDF16RichTextFormatter#format(com.adobe.fontengine.inlineformatting.AttributedRun, int, int, boolean)}. If this
* font is indistinguisable from another font already in the font set then which
* of the two that is preferred is determined by the settings of
* {@link com.adobe.fontengine.inlineformatting.css20.CSS20FontSet#setResolutionPriority}.
* @param font the font to add to the fonts used for CSS resolution
* @throws UnsupportedFontException
* @throws InvalidFontException
*/
public void addFont(Font font) throws UnsupportedFontException, InvalidFontException, FontLoadingException;
/**
* Adds a font to the set of fonts that are examined for resolving a set of
* CSS attributes into an actual font during the formatting process in
* {@link com.adobe.fontengine.inlineformatting.PDF16RichTextFormatter#format(com.adobe.fontengine.inlineformatting.AttributedRun, int, int)}
* and {@link com.adobe.fontengine.inlineformatting.PDF16RichTextFormatter#format(com.adobe.fontengine.inlineformatting.AttributedRun, int, int, boolean)}. If this
* font is indistinguisable from another font already in the font set then which
* of the two that is preferred is determined by the settings of
* {@link com.adobe.fontengine.inlineformatting.css20.CSS20FontSet#setResolutionPriority}. The
* CSS attributes of the font are ignored and those provided are used instead.
* @param fontDesc the CSS properties to use for this font
* @param font the font to add to the fonts used for CSS resolution
* @throws UnsupportedFontException
* @throws InvalidFontException
*/
public void addFont(CSS20FontDescription fontDesc, Font font) throws UnsupportedFontException, InvalidFontException, FontLoadingException;
/**
* Tests if the CSS20FontSet has had no fonts added. It does not check the fallback or generic fonts.
* @return True if the
* {@link com.adobe.fontengine.inlineformatting.css20.CSS20FontSet CSS20FontSet} is empty (has no fonts).
*/
public boolean isEmpty();
/**
* Set the list of replacement font family names for the specified CSS generic font family.
* @param genericFamily the CSS generic font family to assign the replacement fonts to.
* @param replacementFontNames a List of {@link java.lang.String} objects with the
* replacement font family names.
* @see com.adobe.fontengine.inlineformatting.css20.CSS20GenericFontFamily
*/
public void setGenericFont(CSS20GenericFontFamily genericFamily, String[] replacementFontNames);
/**
* Set the fallback fonts.
*
* @param ffs search the fonts in this set when none of the fonts added
* by the addFont
methods are suitable.
*/
public void setFallbackFonts (FallbackFontSet ffs);
/**
* Search for a font using the same search as would used by a layout engine using
* this fontset. Only the first font that would be found using this search is returned.
* If the CSS 2.0 attributes used for the search don't lead to a font in the fontset then
* the locale is used to search the fallback fonts for a match.
*
* @param attributes the CSS 2.0 attributes to search for
* @param locale the locale for the font search
* @return the font found using the CSS 2.0 search algorithm
* @throws FontException
*/
public Font findFont(CSS20Attribute attributes, ULocale locale)
throws FontException;
/**
* Push the current state of the fontset so that fonts added after this can be removed
* by popping the fontset to original state. It is possible to push the state of the
* fontset multiple times and subsequently pop it to return it to the original state.
*
* The push and pop operations only affect the basic fonts added and it does not
* change any locale fallback fonts, generic fonts, or resolution priority.
*/
public void pushFontSet();
/**
* Pop the state of the fontset so that fonts that were added after the last push operation
* are removed and no longer available for use.
*
* The push and pop operations only affect the basic fonts added and it does not
* change any locale fallback fonts, generic fonts, or resolution priority.
*/
public void popFontSet();
/**
* This is equivalent to calling {@link #popFontSet()} as many times as {@link #pushFontSet()}
* has already been called. It will restore the fontset to the state it was in before the
* first push operation.
*
* The push and pop operations only affect the basic fonts added and it does not
* change any locale fallback fonts, generic fonts, or resolution priority.
*/
public void restoreOriginalFontSet();
/**
* Flatten the fontset so that any state information is removed and any fonts that
* have been added to the fontset are "permanent". After this method returns it
* will not be possible to pop the state of the fontset and return to any previous
* state.
*
* The push and pop operations only affect the basic fonts added and it does not
* change any locale fallback fonts, generic fonts, or resolution priority.
*/
public void flattenFontSet();
}