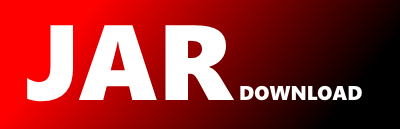
com.adobe.fontengine.inlineformatting.infontformatting.KannadaFormatter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: TeluguFormatter.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.inlineformatting.infontformatting;
import com.adobe.fontengine.inlineformatting.AttributedRun;
public class KannadaFormatter extends IndicFormatter {
protected int splitVowelsAndNormalize (AttributedRun run, int start, int limit) {
while (start < limit) {
int usv = run.elementAt (start);
switch (usv) {
case 0x0CC0: {
run.replace (start, new int[] {0x0CBF, 0x0CD5});
limit++;
start += 2;
break; }
case 0x0CC7: {
run.replace (start, new int[] {0x0CC6, 0x0CD5});
limit++;
start += 2;
break; }
case 0x0CC8: {
run.replace (start, new int[] {0x0CC6, 0x0CD6});
limit++;
start += 2;
break; }
case 0x0CCA: {
run.replace (start, new int[] {0x0CC6, 0x0CC2});
limit++;
start += 2;
break; }
case 0x0CCB: {
run.replace (start, new int[] {0x0CC6, 0xCC2, 0x0CD5});
limit += 2;
start += 3;
break; }
default: {
start++;
break; }}}
return limit;
}
protected int nukta () {
return 0xCBC;
}
protected int virama () {
return 0xCCD;
}
protected boolean isConsonant (int usv) {
return ( 0xC95 <= usv && usv <= 0xCB9
|| 0x25cc == usv);
}
protected boolean hasNukta (int usv) {
return false;
}
protected int removeNukta (int usv) {
return usv;
}
protected boolean isMark (int usv) {
return ( 0x0C01 <= usv && usv <= 0x0C03
|| 0x0CBC == usv
|| 0x0CBE <= usv && usv <= 0xcD6);
}
protected boolean isIndependentVowel (int usv) {
return ( 0x0C85 <= usv && usv <= 0x0C94
|| 0x25cc == usv);
}
protected Position getPosition (int usv) {
switch (usv) {
case 0xCBE:
case 0xCBF:
case 0xCC6:
case 0xCCC: return Position.topMatra;
case 0xCC1:
case 0xCC2:
case 0xCC3:
case 0xCC4:
case 0xCD5:
case 0xCD6: return Position.rightMatra;
case 0xC01:
case 0xC02:
case 0xC03: return Position.rightOther;
default: return Position.any; }
}
protected Shape rephLike (int usv) {
if (usv == 0xCB0) {
return Shape.rephVowel; }
else {
return Shape.any; }
}
protected boolean subjoins (int usv) {
return isConsonant (usv);
}
protected boolean postjoins (int usv) {
return false;
}
protected boolean postjoinsIndependentVowels (int usv) {
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy