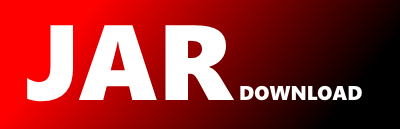
com.adobe.fontengine.inlineformatting.infontformatting.ZapfDingbatsEncoding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: AdobePiRemapping.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.inlineformatting.infontformatting;
import com.adobe.fontengine.inlineformatting.AttributedRun;
import com.adobe.fontengine.inlineformatting.ElementAttribute;
/** Mapping between ZapfDingbats encoding and Unicode.
*
* The ZapfDingbats encoding is defined by the PDF specification,
* p935 in the 1.6 version.*/
public class ZapfDingbatsEncoding {
public static void remap (AttributedRun run, int start, int limit) {
for (int i = start; i < limit; i++) {
if (run.getElementStyle(i, ElementAttribute.isGlyph) == Boolean.FALSE) {
run.replace (i, ZapfDingbatsEncoding.toUnicode [run.elementAt (i)]); }}
}
private static char[] toUnicode = {
0x20, // 0 - .notdef
0x20, // 1 - .notdef
0x20, // 2 - .notdef
0x20, // 3 - .notdef
0x20, // 4 - .notdef
0x20, // 5 - .notdef
0x20, // 6 - .notdef
0x20, // 7 - .notdef
0x20, // 8 - .notdef
0x20, // 9 - .notdef
0x20, // 10 - .notdef
0x20, // 11 - .notdef
0x20, // 12 - .notdef
0x20, // 13 - .notdef
0x20, // 14 - .notdef
0x20, // 15 - .notdef
0x20, // 16 - .notdef
0x20, // 17 - .notdef
0x20, // 18 - .notdef
0x20, // 19 - .notdef
0x20, // 20 - .notdef
0x20, // 21 - .notdef
0x20, // 22 - .notdef
0x20, // 23 - .notdef
0x20, // 24 - .notdef
0x20, // 25 - .notdef
0x20, // 26 - .notdef
0x20, // 27 - .notdef
0x20, // 28 - .notdef
0x20, // 29 - .notdef
0x20, // 30 - .notdef
0x20, // 31 - .notdef
0x20, // 32 - space
0x2701, // 33 - scissors.upblade
0x2702, // 34 - scissors.blk
0x2703, // 35 - scissors.lowblade
0x2704, // 36 - scissors.white
0x260E, // 37 - telephone.blk
0x2706, // 38 - telephone.location
0x2707, // 39 - tapedrive
0x2708, // 40 - airplane
0x2709, // 41 - envelope
0x261B, // 42 - pointingindex.rtblk
0x261E, // 43 - pointingindex.rtwhite
0x270C, // 44 - victoryhand
0x270D, // 45 - writinghand
0x270E, // 46 - pencil.lowrt
0x270F, // 47 - pencil
0x2710, // 48 - pencil.uprt
0x2711, // 49 - pen.whitenib
0x2712, // 50 - pen.blknib
0x2713, // 51 - checkmark
0x2714, // 52 - checkmark.hvy
0x2715, // 53 - multiply.x
0x2716, // 54 - multiply.xhvy
0x2717, // 55 - ballot.x
0x2718, // 56 - ballot.xhvy
0x2719, // 57 - cross.grkout
0x271A, // 58 - cross.grkhvy
0x271B, // 59 - cross.openctr
0x271C, // 60 - cross.openctrhvy
0x271D, // 61 - cross.latin
0x271E, // 62 - cross.latinshadwhite
0x271F, // 63 - cross.latinout
0x2720, // 64 - cross.maltese
0x2721, // 65 - star.david
0x2722, // 66 - asterisk.fourtearspoke
0x2723, // 67 - asterisk.fourballspoke
0x2724, // 68 - asterisk.fourballspokehvy
0x2725, // 69 - asterisk.fourclubspoke
0x2726, // 70 - star.fourptblk
0x2727, // 71 - star.fourptwhite
0x2605, // 72 - star.blk
0x2729, // 73 - star.stressoutwhite
0x272A, // 74 - star.circlewhite
0x272B, // 75 - star.openctrblk
0x272C, // 76 - star.blkctrwhite
0x272D, // 77 - star.outblk
0x272E, // 78 - star.outhvyblk
0x272F, // 79 - star.pinwhl
0x2730, // 80 - star.shadwhite
0x2731, // 81 - asterisk.hvy
0x2732, // 82 - asterisk.openctr
0x2733, // 83 - asterisk.eightspoke
0x2734, // 84 - star.eightptblk
0x2735, // 85 - star.eightptpinwhl
0x2736, // 86 - star.sixptblk
0x2737, // 87 - star.eightptrectilinblk
0x2738, // 88 - star.eightptrectilinhvyblk
0x2739, // 89 - star.twelveptblk
0x273A, // 90 - asterisk.sixteenpt
0x273B, // 91 - asterisk.tearspoke
0x273C, // 92 - asterisk.tearspokeopenctr
0x273D, // 93 - asterisk.tearspokedhvy
0x273E, // 94 - florette.sixpetalblkwhite
0x273F, // 95 - florette.blk
0x2740, // 96 - florette.white
0x2741, // 97 - florette.eightpetaloutblk
0x2742, // 98 - star.eightptopenctr
0x2743, // 99 - asterisk.tearspokepinwhlhvy
0x2744, // 100 - snowflake
0x2745, // 101 - snowflake.tighttrifoliate
0x2746, // 102 - snowflake.chevronhvy
0x2747, // 103 - sparkle
0x2748, // 104 - sparkle.hvy
0x2749, // 105 - asterisk.ballspoke
0x274A, // 106 - asterisk.eighttearspokeprop
0x274B, // 107 - asterisk.eighttearspokeprophvy
0x25CF, // 108 - circle.blk
0x274D, // 109 - circle.shadwhite
0x25A0, // 110 - square.blk
0x274F, // 111 - square.lowrtdropshadwhite
0x2750, // 112 - square.uprtdropshadwhite
0x2751, // 113 - square.lowrtshadwhite
0x2752, // 114 - square.uprtshadwhite
0x25B2, // 115 - triangle.upptblk
0x25BC, // 116 - triangle.downptblk
0x25C6, // 117 - diamond.blk
0x2756, // 118 - diamond.blkminuswhitex
0x25D7, // 119 - circle.rthalfblk
0x2758, // 120 - bar.lt
0x2759, // 121 - bar.med
0x275A, // 122 - bar.hvy
0x275B, // 123 - quoteleft.turncommahvy
0x275C, // 124 - quoteright.commahvy
0x275D, // 125 - quotedblleft.turncommahvy
0x275E, // 126 - quotedblright.commahvy
0x20, // 127 - .notdef
0x2768, // 128 - parenleft.med
0x2769, // 129 - parenright.med
0x276A, // 130 - parenleft.medflat
0x276B, // 131 - parenright.medflat
0x276C, // 132 - anglebracketleftpt.med
0x276D, // 133 - anglebracketrightpt.med
0x276E, // 134 - anglequoteleftpt.hvy
0x276F, // 135 - anglequoterightpt.hvy
0x2770, // 136 - anglebracketleftpt.hvy
0x2771, // 137 - anglebracketrightpt.hvy
0x2772, // 138 - tortshellbracketleft.lt
0x2773, // 139 - tortshellbracketright.lt
0x2774, // 140 - braceleft.med
0x2775, // 141 - braceright.med
0x20, // 142 - .notdef
0x20, // 143 - .notdef
0x20, // 144 - .notdef
0x20, // 145 - .notdef
0x20, // 146 - .notdef
0x20, // 147 - .notdef
0x20, // 148 - .notdef
0x20, // 149 - .notdef
0x20, // 150 - .notdef
0x20, // 151 - .notdef
0x20, // 152 - .notdef
0x20, // 153 - .notdef
0x20, // 154 - .notdef
0x20, // 155 - .notdef
0x20, // 156 - .notdef
0x20, // 157 - .notdef
0x20, // 158 - .notdef
0x20, // 159 - .notdef
0x20, // 160 - .notdef
0x2761, // 161 - pagraph.curvedstem
0x2762, // 162 - exclam.hvy
0x2763, // 163 - exclam.hvyheart
0x2764, // 164 - heart.hvyblk
0x2765, // 165 - heart.rotatedhvyblk
0x2766, // 166 - heart.floral
0x2767, // 167 - heart.rotatedfloral
0x2663, // 168 - suit.clubblk
0x2666, // 169 - suit.diamondblk
0x2665, // 170 - suit.heartblk
0x2660, // 171 - suit.spadeblk
0x2460, // 172 - one.circleserif
0x2461, // 173 - two.circleserif
0x2462, // 174 - three.circleserif
0x2463, // 175 - four.circleserif
0x2464, // 176 - five.circleserif
0x2465, // 177 - six.circleserif
0x2466, // 178 - seven.circleserif
0x2467, // 179 - eight.circleserif
0x2468, // 180 - nine.circleserif
0x2469, // 181 - ten.circleserif
0x2776, // 182 - one.circleserifneg
0x2777, // 183 - two.circleserifneg
0x2778, // 184 - three.circleserifneg
0x2779, // 185 - four.circleserifneg
0x277A, // 186 - five.circleserifneg
0x277B, // 187 - six.circleserifneg
0x277C, // 188 - seven.circleserifneg
0x277D, // 189 - eight.circleserifneg
0x277E, // 190 - nine.circleserifneg
0x277F, // 191 - ten.circleserifneg
0x2780, // 192 - one.circlesans
0x2781, // 193 - two.circlesans
0x2782, // 194 - three.circlesans
0x2783, // 195 - four.circlesans
0x2784, // 196 - five.circlesans
0x2785, // 197 - six.circlesans
0x2786, // 198 - seven.circlesans
0x2787, // 199 - eight.circlesans
0x2788, // 200 - nine.circlesans
0x2789, // 201 - ten.circlesans
0x278A, // 202 - one.circlesansneg
0x278B, // 203 - two.circlesansneg
0x278C, // 204 - three.circlesansneg
0x278D, // 205 - four.circlesansneg
0x278E, // 206 - five.circlesansneg
0x278F, // 207 - six.circlesansneg
0x2790, // 208 - seven.circlesansneg
0x2791, // 209 - eight.circlesansneg
0x2792, // 210 - nine.circlesansneg
0x2793, // 211 - ten.circlesansneg
0x2794, // 212 - arrowright.hvywide
0x2192, // 213 - arrowright
0x2194, // 214 - arrowleftright
0x2195, // 215 - arrowupdown
0x2798, // 216 - arrowsoutheast.hvy
0x2799, // 217 - arrowright.hvy
0x279A, // 218 - arrownortheast.hvy
0x279B, // 219 - arrowright.draftingpt
0x279C, // 220 - arrowright.rndtiphvy
0x279D, // 221 - arrowright.trianglehead
0x279E, // 222 - arrowright.triangleheadhvy
0x279F, // 223 - arrowright.triangleheaddash
0x27A0, // 224 - arrowright.triangleheaddashhvy
0x27A1, // 225 - arrowright.blk
0x27A2, // 226 - arrowheadright.thredtoplight
0x27A3, // 227 - arrowheadright.thredbotlight
0x27A4, // 228 - arrowheadright.blk
0x27A5, // 229 - arrowright.curvdownhvyblk
0x27A6, // 230 - arrowright.curvuphvyblk
0x27A7, // 231 - arrowright.squatblk
0x27A8, // 232 - arrowright.concavehvyblk
0x27A9, // 233 - arrowright.rtshadwhite
0x27AA, // 234 - arrowright.leftshadwhite
0x27AB, // 235 - arrowright.backtiltshadwhite
0x27AC, // 236 - arrowright.fronttiltshadwhite
0x27AD, // 237 - arrowright.lowrtshadhvywhite
0x27AE, // 238 - arrowright.uprtshadhvywhite
0x27AF, // 239 - arrowright.notchlowrtshadwhite
0x20, // 240 - .notdef
0x27B1, // 241 - arrowright.notchuprtshadwhite
0x27B2, // 242 - arrowright.circlehvywhite
0x27B3, // 243 - arrowright.whitefeather
0x27B4, // 244 - arrowsoutheast.blkfeather
0x27B5, // 245 - arrowright.blkfeather
0x27B6, // 246 - arrownortheast.blkfeather
0x27B7, // 247 - arrowsoutheast.blkfeatherhvy
0x27B8, // 248 - arrowright.blkfeatherhvy
0x27B9, // 249 - arrownortheast.blkfeatherhvy
0x27BA, // 250 - arrowright.tearbarbed
0x27BB, // 251 - arrowright.tearbarbed
0x27BC, // 252 - arrowright.wedgetail
0x27BD, // 253 - arrowright.wedgetailhvy
0x27BE, // 254 - arrowright.openout
0x20 // 255 - .notdef
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy