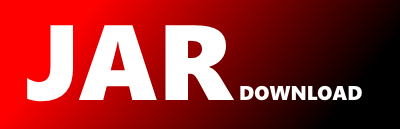
com.adobe.fontengine.math.Math2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: Math2.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2006 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.math;
/** Additional math functions.
*/
public class Math2 {
public static double roundHalfUp (double v) {
return Math.floor (v + 0.5);
}
public static double roundHalfDown (double v) {
return Math.ceil (v - 0.5);
}
public static boolean epsilonEquals(float a, float b)
{
float epsilon = (float)0.0000001;
return (Math.abs(a - b) < epsilon);
}
public static boolean epsilonEquals(double a, double b)
{
double epsilon = 0.00000001;
return (Math.abs(a - b) < epsilon);
}
/* Find the power of 2 greater than the absolute value of passed parameter */
private static final int[] bits = { 0, 1, 2, 2, 3, 3, 3, 3, 4, 4, 4, 4, 4, 4, 4, 4 };
public static int powerOf2 (int v) {
if (v < 0L) {
v = -v; }
if (v < (1 << 16)) {
if (v < (1 << 8)) {
if (v < (1 << 4)) {
return (bits [v]); }
else {
return (bits [v >> 4] + 4); }}
else {
if (v < (1 << 12)) {
return (bits [v >> 8] + 8); }
else {
return (bits [v >> 12] + 12); }}}
else {
if (v < (1 << 24)) {
if (v < (1 << 20)) {
return (bits [v >> 16] + 16); }
else {
return (bits [v >> 20] + 20); } }
else {
if (v < (1 << 28)) {
return (bits [v >> 24] + 24); }
else {
return (bits [v >> 28] + 28); }}}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy