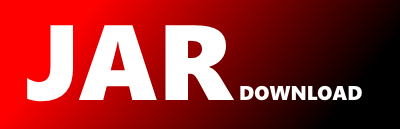
com.adobe.granite.activitystreams.ActivityManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.activitystreams;
import java.util.Collection;
import org.apache.jackrabbit.api.security.user.Authorizable;
import org.apache.jackrabbit.api.security.user.User;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import org.apache.sling.commons.json.JSONObject;
import org.osgi.annotation.versioning.ProviderType;
/**
* The activity manager is used as entry point to read, create and write activities and activity streams.
*/
@ProviderType
public interface ActivityManager {
/**
* Creates a new mutable activity for populating with the input data.
* @return a new mutable activity.
*/
MutableActivity newActivity();
/**
* Creates a new mutable activity populated with input data of a JSON object.
* @param jsonObject JSON object representing the activity.
* @return a new mutable activity.
*/
MutableActivity newActivity(JSONObject jsonObject);
/**
* Creates a new mutable activity object for populating with the input data.
* @return a new mutable activity object.
*/
MutableActivityObject newActivityObject();
/**
* Creates a new mutable media link for populating with the input data.
* @return a new mutable media link.
*/
MutableMediaLink newMediaLink();
/**
* Returns the activity collection for the given user. The collection is an aggregate of the users stream and
* all the streams the user follows.
*
* @param resolver resource resolver to resolve the path
* @param userId the userid of the user. If null
the user bound to the resource resolver is used.
* @return the ActivityCollection
* @throws ActivityException
*/
ActivityCollection getActivities(ResourceResolver resolver, String userId) throws ActivityException;
/**
* Returns the activity collection for the given user. The collection is an aggregate of the users stream and
* all the streams the user follows controlled by the aggregate options.
*
* @param resolver resource resolver
* @param userId the userid of the user. If null
the user bound to the resource resolver is used.
* @param options the aggregate options
* @return the ActivityCollection
* @throws ActivityException if an error occurs
* @since 0.0.14
*/
ActivityCollection getActivities(ResourceResolver resolver, String userId, AggregateOptions options) throws ActivityException;
/**
* Returns the activity with the given id or null
if not exists.
* @param resolver resource resolver to resolve the id
* @param id the id of the activity
* @return the activity or null
* @throws ActivityException if an error occurs.
*/
Activity getActivity(ResourceResolver resolver, String id) throws ActivityException;
/**
* Gets the distinct activity stream for the given user.
* @param resolver resource resolver to resolve the path
* @param userId the userid of the user
* @param streamName the name of the stream or null
to use the default stream
* @return the activity stream or null
if the user or his stream does not exist.
* @throws ActivityException if an error occurs.
*/
ActivityStream getUserStream(ResourceResolver resolver, String userId, String streamName, boolean create)
throws ActivityException;
/**
* Gets the distinct activity stream for the given group.
* @param resolver resource resolver to resolve the path
* @param groupName the name of the group
* @param streamName the name of the stream or null
to use the default stream
* @return the activity stream or null
if the user or his stream does not exist.
* @throws ActivityException if an error occurs.
*/
ActivityStream getGroupStream(ResourceResolver resolver, String groupName, String streamName, boolean create)
throws ActivityException;
/**
* Gets the distinct activity stream for the given authorizable.
* @param resolver resource resolver to resolve the path
* @param auth the jackrabbit authorizable
* @param streamName the name of the stream or null
to use the default stream
* @return the activity stream or null
if the user or his stream does not exist.
* @throws ActivityException if an error occurs
*/
ActivityStream getStream(ResourceResolver resolver, Authorizable auth, String streamName, boolean create) throws ActivityException;
/**
* Returns the activity stream for the given resource.
* @param streamResource the stream resource
* @return the stream
* @throws ActivityException if an error occurs
*/
ActivityStream getStream(Resource streamResource) throws ActivityException;
/**
* Returns the activity stream for the given container resource, e.g. user, group, document. If the stream does
* not exist and create
is true
a new stream is created.
* @param containerResource the container resource
* @param name the stream name
* @return the stream or null
if no such stream exists
* @throws ActivityException if an error occurs
*/
ActivityStream getStream(Resource containerResource, String name, boolean create) throws ActivityException;
/**
* Lists all streams of the given container resource (user, group, document);
* @param containerResource the resource to list the streams
* @return a collection of streams or null
if the resource is invalid.
* @throws ActivityException if an error occurs
*/
Collection listStreams(Resource containerResource) throws ActivityException;
/**
* Lists all streams for the given user that would be used to build the activity collection.
* @param resolver resource resolver
* @param userId the user id
* @param options the aggregate options
* @return a collection of streams or null
if the resource is invalid.
* @throws ActivityException if an error occurs
* @since 0.0.10
*/
Collection listStreams(ResourceResolver resolver, String userId, AggregateOptions options) throws ActivityException;
/**
* Returns an iterable over a list of users that see this activity in their default aggregate stream.
* Note that the resource resolver bound to the activity is used to resolve all paths and authorizables.
*
* @param activity the activity
* @return an iterable over all user ids.
* @throws ActivityException if an error occurs
* @throws IllegalArgumentException if the activity is not persisted in the underlying repository
* @since 0.0.10
*/
Iterable getFollowers(Activity activity) throws ActivityException, IllegalArgumentException;
/**
* Returns an iterable over a list of users that follow this stream stream.
* Note that the resource resolver bound to the activity is used to resolve all paths and authorizables.
*
* @param stream the stream
* @return an iterable over all user ids.
* @throws ActivityException if an error occurs
* @throws IllegalArgumentException if the activity is not persisted in the underlying repository
* @since 0.0.14
*/
Iterable getFollowers(ActivityStream stream) throws ActivityException, IllegalArgumentException;
/**
* Gets the distinct activity stream for the given user.
* @param resolver resource resolver to resolve the path
* @param userId the userid of the user
* @param streamName the name of the stream or null
to use the default stream
* @return the activity stream or null
if the user or his stream does not exist.
* @throws ActivityException if an error occurs.
* @deprecated since 0.0.8. use {@link #getUserStream(org.apache.sling.api.resource.ResourceResolver, String, String, boolean)} instead.
*/
@Deprecated
ActivityStream getUserStream(ResourceResolver resolver, String userId, String streamName) throws ActivityException;
/**
* Gets the distinct activity stream for the given user.
* @param resolver resource resolver to resolve the path
* @param user the jackrabbit user
* @param streamName the name of the stream or null
to use the default stream
* @return the activity stream or null
if the user or his stream does not exist.
* @throws ActivityException if an error occurs
* @deprecated since 0.0.8. use {@link #getStream(org.apache.sling.api.resource.ResourceResolver, org.apache.jackrabbit.api.security.user.Authorizable, String, boolean)}
*/
@Deprecated
ActivityStream getUserStream(ResourceResolver resolver, User user, String streamName) throws ActivityException;
/**
* Returns the activity stream for the given resource.
* @param topicResource the topic for which the stream is retrieved
* @param streamName the name of the stream or null
to use the default stream
* @return the stream
* @throws ActivityException if an error occurs
* @deprecated since 0.0.8. use {@link #getStream(org.apache.sling.api.resource.Resource, String, boolean)} instead.
*/
@Deprecated
ActivityStream getTopicStream(Resource topicResource, String streamName) throws ActivityException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy