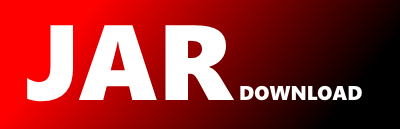
com.adobe.granite.asset.api.AssetMetadata Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2013 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.asset.api;
import java.util.List;
import org.osgi.annotation.versioning.ProviderType;
import com.adobe.xmp.core.XMPMetadata;
import com.adobe.xmp.path.XMPPath;
import com.adobe.xmp.schema.service.SchemaService;
/**
* AssetMetadata
defines the {@link Asset} metadata.
*
* To get AssetMetadata, use {@link com.adobe.granite.asset.api.Asset#getAssetMetadata()}
*
*
*
* Example:
*
* Asset asset = assetManager.getAsset("/path/to/asset/document.pdf");
*
* // get metadata
* AssetMetadata assetMetadata = asset.getAssetMetadata();
*
*
*
*/
@ProviderType
public interface AssetMetadata {
/**
*
* Get XMP. An empty XMPMetadata object is returned if there is no existing metadata
*
* @return XMP metadata object
*
* @throws AssetException If an error occurs trying to load the XMPMetadata object
*
* */
XMPMetadata getXMP();
/**
* Set XMP. This method will replace any existing XMP previously stored.
*
* To update existing XMP data, either use #setProperty or #getXMP- update the resulting object and #setXMP
*
* @param meta, XMP metadata object to be set
* @param schemaService, optional schema service to be used to persist property types
*
* @throws AssetException if the given XMP cannot be stored in Asset
* @throws NullPointerException if the given XMPMetadata is null
*
* */
void setXMP(XMPMetadata meta, SchemaService schemaService);
/**
* Set XMP. This method will replace any existing XMP previously stored.
*
* To update existing XMP data, either use #setProperty or #getXMP- update the resulting object and #setXMP.
*
* ignoreHierarchy parameter defines a list of fully qualified xmp node names for which qualifiers and
* language alternatives needs to be ignored.
*
* @param meta, XMP metadata object to be set
* @param schemaService, optional schema service to be used to persist property types
* @param ignoreHierarchy, list of nodes for which hierarchy needs to be ignored
*
* @throws AssetException if the given XMP cannot be stored in Asset
* @throws NullPointerException if the given XMPMetadata is null
*
* */
void setXMP(XMPMetadata meta, SchemaService schemaService, List ignoreHierarchy);
/**
* Set a simple XMP property. If there is no property found at the given path, a new property is created,
* otherwise value is updated.
*
* This method supports String, Calendar, Long, BigDecimal & Boolean
*
* @param the type of the value
* @param path, XMP path including the property name which needs to be updated
* @param value, to be set
*
* @throws AssetException if property cannot be set
* @throws NullPointerException if the given XMPPath is null
*
* */
void setProperty(XMPPath path, T value);
/**
* Get a simple xmp property value.
*
* This method supports String, Calendar, Long, BigDecimal & Boolean
*
* @param the type
* @param path, XMP path
* @param type, type of the value expected
*
* @return property value or null if the property does not exist
*
* @throws AssetException if property cannot be read or typed to a requested type
* @throws NullPointerException if the given XMPPath is null
*
* */
T getProperty(XMPPath path, Class type);
}