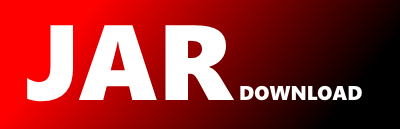
com.adobe.granite.confmgr.Conf Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2014 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.confmgr;
import java.util.List;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ValueMap;
import org.osgi.annotation.versioning.ProviderType;
/**
* Provides access to configuration.
*
* @deprecated Use of the open source
*
* Apache Sling Context-Aware Configuration API is recommended for new code.
* The ConfMgr API is backed by the Sling CA implementation and will continue
* to be supported for existing code.
*/
@Deprecated
@ProviderType
public interface Conf extends ConfConstants {
// -------------------------------------------< single configs >-----------------------------------
/**
* Returns configuration values for the given config item which can be a relative path.
* See also {@link #getItemResource(String)} if you need to the resource behind the value map.
* If the config item has a "jcr:content" child, this will be returned as the source
* of the value map.
*
*
* The itemName can be a relative path and is looked up beneath the resolved configuration tree.
* For example, if the original resource from which this Conf object was created,
* points to a configuration at "/conf/adobe/ch", and an item path like "dam/presets/pdf"
* is requested, this will return a value map for the resource at "/conf/adobe/ch/dam/presets/pdf".
* Note that itemName should be a fixed name specific to the application config.
*
*
* If no configuration resource can be found, an empty map will be returned.
*
* @param itemName a relative path to an item within a specific configuration tree
* @return a value map for a configuration resource; an empty map if nothing is found
*/
ValueMap getItem(String itemName);
/**
* Returns a configuration resource for the given item which can be a relative path.
* Use this instead of {@link #getItem(String)} if you need to iterate over the child structure,
* for example a list of resources.
*
*
* The itemName can be a relative path and is looked up beneath the resolved configuration tree.
* For example, if the original resource from which this Conf object was created,
* points to a configuration at "/conf/adobe/ch", and an item path like "dam/presets/pdf"
* is requested, this will return the resource at "/conf/adobe/ch/dam/presets/pdf".
* Note that itemName should be a fixed name specific to the application config.
*
*
* If no configuration resource can be found, {@code null} will be returned.
*
* @param itemName a relative path to an item within a specific configuration tree
* @return a configuration resource or {@code null} if not found
*/
Resource getItemResource(String itemName);
// -------------------------------------------< list config access >-------------------------------
/**
* Returns ValueMaps for a list of items beneath the given configuration item. Depending
* on the configuration item, this list could be automatically merged between the different
* applicable configurations.
*
*
* See {@link #getListResources(String)} for the merge logic.
*
* @param parentItemName a relative path to an item within a specific configuration tree
* @return a possibly merged list of items as ValueMaps; an empty list if nothing is found
*/
List getList(String parentItemName);
/**
* Returns a list of items beneath the given configuration item. This allows for cases where
* there is a choice of different configuration entries. Note that folders and nested structures
* are not supported as applications should map them to entirely separate sub-project configurations.
*
*
* If the most specific configuration parent item has {@link #PN_MERGE_LIST} set to true,
* the list will be merged with the lists for all applicable configurations.
* Starting with the lowest level configuration up to the most specific one, for each
* configuration, if the parent item is present, these rules will be applied:
*
* - a new item will be added to the list
* - same name item with a jcr:content child will overwrite
* - same name item without a jcr:content child will remove it from the list
*
*
*
* Note that for merged lists, no order is guaranteed. For fixed lists, the order of the
* child resources will be kept.
*
* @param parentItemName a relative path to an item within a specific configuration tree
* @return a possibly merged list of items as Resources; an empty list if nothing is found
*/
List getListResources(String parentItemName);
}