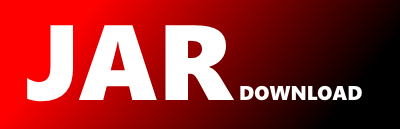
com.adobe.granite.contexthub.commons.Utils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2016 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
*/
package com.adobe.granite.contexthub.commons;
import org.apache.commons.lang3.StringUtils;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceUtil;
import org.apache.sling.api.resource.ValueMap;
import static org.apache.jackrabbit.JcrConstants.JCR_CONTENT;
public class Utils {
private static final String PN_PREFIX = "./" + JCR_CONTENT;
/**
* Returns the content property of the given page. If the resource does not
* contain this property, the parent resource is checked against given property
* or null
if resource has no parent.
*
* @param resource - resource
* @param property - property name
* @return the property value or null
*/
public static String getInheritedProperty(Resource resource, String property) {
String previousPath = null;
String value = null;
String localProperty = property;
//If the property has the form "./jcr:content/propertyName". It removes the "jcr:content" prefix
//leaving the property name as ./propertyName. This is needed because the algorithm below
//always checks for the property on the jcr:content node.
if (StringUtils.startsWith(localProperty, PN_PREFIX)) {
localProperty = "." + localProperty.substring(PN_PREFIX.length());
}
while ((value == null) && (resource != null)) {
Resource here = resource;
/* get "jcr:content" child if needed */
if (!JCR_CONTENT.equals(here.getName())) {
here = here.getChild(JCR_CONTENT);
}
/* is resource found? */
if (here != null) {
String currentPath = here.getPath();
if (!currentPath.equals(previousPath)) {
ValueMap properties = ResourceUtil.getValueMap(here);
previousPath = currentPath;
value = properties.get(localProperty, String.class);
/* check parent if value is empty */
if ((value != null) && (value.length() == 0)) {
value = null;
}
}
}
/* get parent */
resource = resource.getParent();
}
return value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy