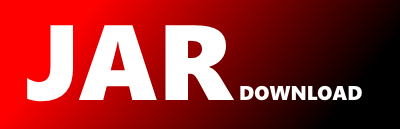
com.adobe.granite.oauth.jwt.JwsBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2013 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.oauth.jwt;
import aQute.bnd.annotation.ProviderType;
import com.adobe.granite.crypto.CryptoException;
/**
* The JwsBuilder
provides a simple API to issue JWS formatted token
* as defined in http://tools.ietf.org/html/draft-ietf-oauth-json-web-token-16
* and http://tools.ietf.org/html/draft-ietf-jose-json-web-signature-21
*/
@ProviderType
public interface JwsBuilder {
/**
* @return a signed JWS as defined in http://tools.ietf.org/html/draft-ietf-jose-json-web-signature-21
* @throws CryptoException if any problem occurs signing the token
*/
public String build() throws CryptoException;
/**
* Set the (Issuer) Claim as for http://tools.ietf.org/html/draft-ietf-oauth-json-web-token-16#section-4.1.1
*
* @param iss The (Issuer) Claim
* @return JwsBuilder
*/
public JwsBuilder setIssuer(String iss);
/**
* Set the (Subject) Claim as for http://tools.ietf.org/html/draft-ietf-oauth-json-web-token-16#section-4.1.2
*
* @param sub The (Subject) Claim as
* @return JwsBuilder
*/
public JwsBuilder setSubject(String sub);
/**
* Set the (Audience) Claim as for http://tools.ietf.org/html/draft-ietf-oauth-json-web-token-16#section-4.1.3
*
* @param aud The (Audience) Claim
* @return JwsBuilder
*/
public JwsBuilder setAudience(String aud);
/**
* Set the expiration time for the token expressed in seconds
*
* @param expiresIn The expiration time for the token expressed in seconds
* @return JwsBuilder
*/
public JwsBuilder setExpiresIn(long expiresIn);
/**
* Set the scope associate with the token
*
* @param scope The scope associate with the token
* @return JwsBuilder
*/
public JwsBuilder setScope(String scope);
/**
* Set a custom claim field
*
* @param key The claim field custom name
* @param value The claim field value
* @return JwsBuilder
*/
public JwsBuilder setCustomClaimsSetField(String key, Object value);
/**
* Set the (Issued At) Claim as for https://tools.ietf.org/html/draft-ietf-oauth-json-web-token-25#section-4.1.6
* If the set Issued At is too far away in the future (20 seconds or more than current time) the set Issued At
* will be ignored and the current time is used instead to build the JWT
*
* @param iat The (Issued At) Claim expressed in seconds
* @return JwsBuilder
* @since 1.1
*/
public JwsBuilder setIssuedAt(long iat);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy