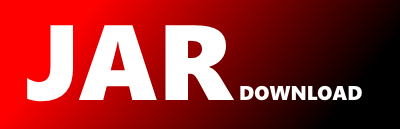
com.adobe.granite.references.Reference Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2013 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.references;
import org.apache.sling.api.resource.Resource;
import org.osgi.annotation.versioning.ConsumerType;
import java.util.Objects;
/**
* A Reference
represents a reference to the {@link Resource} backing the {@link ReferenceList}.
* References can only be retrieved via a {@link ReferenceList}.
* Each reference has a reference type.
* The types available are defined by the {@link ReferenceProvider}s registered in the system and may vary.
*
* @since 1.0
*/
@ConsumerType
public class Reference {
private final Resource source;
private final Resource target;
private final String type;
public Reference(final Resource source, final Resource target, final String type) {
this.source = source;
this.target = target;
this.type = type;
}
/**
* Returns the source resource for which the references have been retrieved through the {@link ReferenceProvider}.
*
* @return The source resource.
*/
public Resource getSource() {
return source;
}
/**
* Returns the target resource of the reference, i.e. one of the results retrieved by the {@link ReferenceProvider}.
*
* @return The target resource.
*/
public Resource getTarget() {
return target;
}
/**
* Returns the reference type of this reference.
*
* @return The reference type.
*/
public String getType() {
return type;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Reference reference = (Reference) o;
// Check that both source and target resources are equals and that the type is equals as well
return equalsResource(source, reference.source) &&
equalsResource(target, reference.target) &&
Objects.equals(type, reference.type);
}
private boolean equalsResource(Resource resource, Resource otherResource) {
if (resource == null) {
// If source is null then the object is equals when target is null too
return otherResource == null;
} else {
// If source is not null then target must not be null and paths must be equals
return otherResource != null && resource.getPath().equals(otherResource.getPath());
}
}
@Override
public int hashCode() {
return Objects.hash(source != null ? source.getPath() : null,
target != null ? target.getPath() : null,
type);
}
/**
* {@inheritDoc}
*/
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("Reference => {\n");
sb.append("\tsource: ").append(getSource() != null ? getSource().getPath() : null).append("\n");
sb.append("\ttarget: ").append(getTarget() != null ? getTarget().getPath() : null).append("\n");
sb.append("\ttype: ").append(getType()).append("\n");
sb.append("\tclass: ").append(getClass().getCanonicalName()).append("\n");
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy