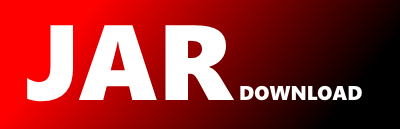
com.adobe.granite.rest.ResourceManager Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2014 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.rest;
import java.util.List;
import org.apache.sling.api.resource.Resource;
import org.osgi.annotation.versioning.ConsumerType;
/**
* The {@code ResourceManager} defines the API which might be asked to find,
* copy, move and delete specific {@link org.apache.sling.api.resource.Resource resources}.
*
* Implementors should ensure that a ResourceManager can be retrieved adapting a
* {@link org.apache.sling.api.SlingHttpServletRequest} by implementing an
* {@link org.apache.sling.api.adapter.AdapterFactory}.
*/
@ConsumerType
public interface ResourceManager {
/**
* Copies all properties and resources of the implementor to the
* {@code destUri}. The method expects the {@code destUri} to be a
* non-remote URI, absolute, normalized and within the same name space as
* the {@code resource}.
*
* The method should throw a {@link RequestException} with a HTTP status 403
* if the destination is not part of the resources ResourceProvider name
* space. If the parent resource of the destination does not exist the
* method should throw a {@link RequestException} with the HTTP status code
* 412. In case an error occurs while copying one of the resources child
* elements a {@link RequestException} with HTTP status code 207 pointing to
* the failing resource should be thrown.
*
* @param resource Resource to copy
* @param destUri Destination URI to copy resource to
* @param depth Depth value indicating the depth of the copy. A value of -1
* is equal to infinite, a value of 0 indicates that only the
* resource itself and its properties should be copied but no
* children.
* @return {@code true} if destination has been created, {@code false} if it
* has been overwritten.
* @throws UnsupportedOperationException If the operation is not supported
* for the {@code resource}
* @throws RequestException if a precondition for the operation is not met
* @throws RestException if an error occurs during the operation
*/
boolean copy(Resource resource, String destUri, int depth) throws UnsupportedOperationException, RequestException,
RestException;
/**
* Moves all properties and resources of the implementor to the
* {@code destUri}. The method expects the {@code destUri} to be a
* non-remote URI, absolute, normalized and within the same name space as
* the {@code resource}. The method should throw a {@link RequestException}
* with a HTTP status 403 if the destination is not part of the resources
* ResourceProvider name space. If the parent resource of the destination
* does not exist the method should throw a {@link RequestException} with
* the HTTP status code 412. In case an error occurs while copying one of
* the resources child elements a {@link RequestException} with HTTP status
* code 207 pointing to the failing resource should be thrown.
*
* @param resource Resource to move
* @param destUri Destination URI to move resource to
* @return {@code true} if destination has been created, {@code false} if it
* has been overwritten.
* @throws UnsupportedOperationException If the operation is not supported
* for the {@code resource}
* @throws RequestException if a precondition for the operation is not met
* @throws RestException if an error occurs during the operation
*/
boolean move(Resource resource, String destUri) throws UnsupportedOperationException, RequestException,
RestException;
/**
* Returns the query result from the provided {@code query} statement as a
* {@link java.util.List}.
*
* @param resource Base resource. Is used as a starting point in the tree to
* search from.
* @param query Query statement
* @return A {@link List} of {@link org.apache.sling.api.resource.Resource resources} found matching the
* query
* @throws UnsupportedOperationException If the operation is not supported
* for the {@code resource}
* @throws RequestException if a precondition for the operation is not met
* @throws RestException if an error occurs during the search
*/
public List find(Resource resource, String query) throws UnsupportedOperationException, RequestException,
RestException;
}