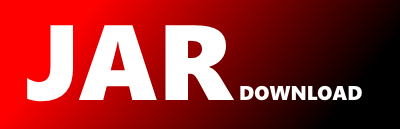
com.adobe.granite.security.user.UserManagementService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.security.user;
import org.apache.jackrabbit.api.security.user.UserManager;
import org.osgi.annotation.versioning.ProviderType;
import javax.annotation.Nonnull;
import javax.jcr.RepositoryException;
import javax.jcr.Session;
/**
* Service interface for common user management related tasks and utility methods.
*/
@ProviderType
public interface UserManagementService {
/**
* Retrieve the {@code UserManager} associated with the given session. This
* method is a shortcut for calling {@link org.apache.jackrabbit.api.JackrabbitSession#getUserManager()}.
*
* @param session The editing session.
* @return The {@code UserManager} associated with the given session.
* @throws java.lang.UnsupportedOperationException If the specified session is not
* an {@link org.apache.jackrabbit.api.JackrabbitSession}.
* @throws javax.jcr.RepositoryException If an error occurs or if the JCR
* repository doesn't support user managent.
*/
@Nonnull
UserManager getUserManager(@Nonnull Session session) throws UnsupportedOperationException, RepositoryException;
/**
* @return the path of the common ancestor node for all users and groups.
*/
@Nonnull
String getAuthorizableRootPath();
/**
* @return the configured path of the user root node.
*/
@Nonnull
String getUserRootPath();
/**
* @return the configured path of the group root node.
*/
@Nonnull
String getGroupRootPath();
/**
* @return the configured path of the system users root node.
*/
@Nonnull
String getSystemUserRootPath();
/**
* @return The user ID of the anonymous (guest) user.
*/
@Nonnull
String getAnonymousId();
/**
* Retrieve the user identifier of currently logged in user
* for eg:- jcr:uuid of logged in user
*
* @param session The current user session
* @return The user id(uuid) associated with user
* @throws java.lang.UnsupportedOperationException If the specified session is not
* an {@link org.apache.jackrabbit.api.JackrabbitSession} or if Authorizable is not of User type.
* @throws javax.jcr.RepositoryException If an error occurs or user id doesnt exist or if the JCR
* repository doesn't support user management.
*/
@Nonnull
String getUserInternalId(@Nonnull Session session) throws UnsupportedOperationException, RepositoryException;
/**
* @return The user ID of the administrative user.
*/
@Nonnull
String getAdminId();
/**
* @return The name of the built-in everyone principal.
*/
@Nonnull
String getEveryoneName();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy