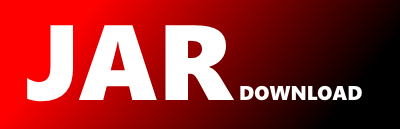
com.adobe.granite.security.user.UserPropertiesQueryParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2017 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.security.user;
import org.osgi.annotation.versioning.ProviderType;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
/**
* Groups together all the criteria and controls that can be used to configure the search for authorizables.
*/
@ProviderType
public interface UserPropertiesQueryParams {
/**
* Returns the offset of the query.
*
* @return The index of the first resulted item to be returned by the query.
*/
long getOffset();
/**
* Sets the offset of the query.
*
* @param offset
* The index of the first resulted item to be returned by the query.
* @return {@code this}
*/
@Nonnull
UserPropertiesQueryParams withOffset(long offset);
/**
* Returns the limit of the query.
*
* @return The maximum count of items to be returned by the query.
*/
long getLimit();
/**
* Sets the limit of the query.
*
* @param limit
* The maximum count of items to be returned by the query.
* @return {@code this}
*/
@Nonnull
UserPropertiesQueryParams withLimit(long limit);
/**
* Returns the string used during fulltext search.
*
* @return The fulltext query string.
*/
@Nullable
String getFulltextQuery();
/**
* Sets the string used during fulltext search.
*
* @param fulltextQuery
* The fulltext query string.
* @return {@code this}
*/
@Nonnull
UserPropertiesQueryParams withFulltextQuery(@Nullable String fulltextQuery);
/**
* Returns the {@link AuthorizableTypes} of the query.
*
* @return The {@link AuthorizableTypes}.
*/
@Nullable
AuthorizableTypes getAuthorizableTypes();
/**
* Sets the {@link AuthorizableTypes} of the query.
*
* @param authorizableTypes
* The {@link AuthorizableTypes}.
* @return {@code this}
*/
@Nonnull
UserPropertiesQueryParams withAuthorizableTypes(@Nonnull AuthorizableTypes authorizableTypes);
/**
* Returns whether to filter for the users that the provided user can impersonate:
*
*
* Boolean.TRUE
* - Include only the users that the provided user can impersonate.
*
* Boolean.FALSE
* - Currently not supported
*
* null
* - No filtering is performed with regards to impersonation.
*
*
* When this methods returns a non null value, it is guaranteed that a non null impersonator principal name has been set.
*
* Using this feature requires that the user making the call has {@code jcr:read} permissions for the
* authorizable node.
*
* @return The Boolean whether to filter the impersonatees.
*/
@Nullable
Boolean getImpersonableUserFilter();
/**
* Gets the name of the user that can or cannot impersonate the users from the query.
* @return the name of the user or {@code null} if no filtering is performed with regards to impersonation.
*/
@Nullable
String getImpersonatorPrincipalName();
/**
* Sets whether to filter for the users that the provided user can
*
* @param impersonatorPrincipalName the name of the user that can impersonate the users from the query result.
* If set to {@code null}, no filter will be performed with regards to impersonation
*
* @return {@code this}
*/
@Nonnull
UserPropertiesQueryParams withOnlyUsersThatCanBeImpersonatedBy(@Nullable String impersonatorPrincipalName);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy