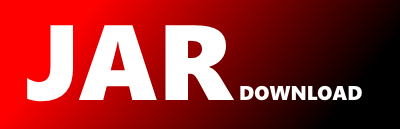
com.adobe.granite.translation.api.TranslationConstants Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2014 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.translation.api;
//
/** Class TranslationConstants. */
public final class TranslationConstants {
/**
* Types of relationships (source, derived, reference ect...) supported by the Translation Framework
*/
public class TranslationRelationShips {
/**
* Source relation from a {@link TranslationObject}, where current {@link TranslationObject} is generated
* using source {@link TranslationObject}
*/
public static final String SOURCE = "translation_object_relation_source";
/**
* Reference relations from a {@link TranslationObject}, where current {@link TranslationObject} is referenced
* by related {@link TranslationObject}
*/
public static final String REFERENCE = "translation_object_relation_reference";
/**
* Related relation from a {@link TranslationObject}, where related {@link TranslationObject} is referenced
* by current {@link TranslationObject}
*/
public static final String RELATED = "translation_object_relation_related";
/**
* Derived relation from a {@link TranslationObject}, where derived {@link TranslationObject} is generated
* using current {@link TranslationObject}
*/
public static final String DERIVED = "translation_object_relation_derived";
};
/** Instantiates a new instance of TranslationConstants. */
private TranslationConstants() {
/** This prevents native classes from calling this constructor. */
throw new AssertionError();
}
/** Enum TranslationMethod. */
public static enum TranslationMethod {
/** machine translation */
MACHINE_TRANSLATION,
/** human translation */
HUMAN_TRANSLATION
}
/**
* Enum TranslationStatus. This is the complete list of statuses used with Translation Jobs and Translation
* Objects. Status is the principal means by Objects and Jobs are moved through the translation process. All
* STATUS values are set by the Translation Framework but for each status it has been noted (Set by the Vendor or
* Set by the Translation Framework) to indicated who will be invoking the call
*/
public static enum TranslationStatus {
/**
* DRAFT. (Set by the Translation Framework) The DRAFT status is the very first status in the Translation
* process. All objects that need to be translated or Translation Jobs begin in a DRAFT state.
*/
DRAFT, // CQ-37143, i18n.get("DRAFT"), workaround to get this string extracted for translation without touching code
/**
* SUBMITTED. (Set by the Translation Framework) The SUBMITTED state is returned when the content upload to
* the Translation vendor is complete.
*/
SUBMITTED, // CQ-37143, i18n.get("SUBMITTED"), workaround to get this string extracted for translation without touching code
/**
* SCOPE_REQUESTED (Set by the Translation Framework) Scoping is the process in which text submitted for
* translation is tokenized and compared against existing Translation Memory (The existing pool of stored and
* subject relevant translations.) Scoping is generally provided by a translation service as a pre-translation
* courtesy to help estimate the total cost of the translation work to be done. If a match exists in
* translation memory then that match is returned. The scope returned by SCOPE_REQUESTED is generally speaking
* the difference between the total number of words submitted for translation and the number for words for
* which translations already exist in Translation Memory. This difference is also known as the number of
* words leveraged. SCOPE_REQUESTED state is returned when the content uploaded to the Translation vendor
* requires that scope be calculated by the translation vendor.
*/
SCOPE_REQUESTED, // CQ-37143, i18n.get("SCOPE_REQUESTED"), workaround to get this string extracted for translation without touching code
/**
* SCOPE_COMPLETED. (Set by the Vendor) Scoping is the process in which the submitted text is tokenized and
* compared against a Translation memory. Scoping is generally Free for the customer. The Translation memory
* is the store/pool of existing translation. If a match exists in the translation memory, that match is
* returned. Scoping returns what is the scope for translation. Total number of words - Words already
* available in translation memory (leverage words). SCOPE_COMPLETED status is returned when a job is scoped
* to figure out how much translation is necessary. Scoping is an Optional Step.
*/
SCOPE_COMPLETED, // CQ-37143, i18n.get("SCOPE_COMPLETED"), workaround to get this string extracted for translation without touching code
/**
* COMMITED_FOR_TRANSLATION. (Set by the Vendor) Finalizes the agreement for Translation. This is done after
* Scoping, and is a sign-off to the vendor to go and translated.
*/
COMMITTED_FOR_TRANSLATION, // CQ-37143, i18n.get("COMMITTED_FOR_TRANSLATION"), workaround to get this string extracted for translation without touching code
/**
* TRANSLATION_IN_PROGRESS. (Set by the Vendor) Status returned by the Vendor when translation has started for
* an object. Once the TRANSLATION_IN_PROGRESS status is returned, the translation can't be canceled.
*/
TRANSLATION_IN_PROGRESS, // CQ-37143, i18n.get("TRANSLATION_IN_PROGRESS"), workaround to get this string extracted for translation without touching code
/**
* TRANSLATED. (Set by the Vendor) Vendor communicates they have completed Translation of the Object or Job.
* This status indicates that the translation is complete, and ready for import.
*/
TRANSLATED, // CQ-37143, i18n.get("TRANSLATED"), workaround to get this string extracted for translation without touching code
/**
* Ready for review. (Set by the Translation Framework) Once the status is returned as TRANSLATED, the Object
* is imported back and the status changes to READY_FOR_REVIEW
*/
READY_FOR_REVIEW, // CQ-37143, i18n.get("READY_FOR_REVIEW"), workaround to get this string extracted for translation without touching code
/**
* REJECTED. (Set by the Translation Framework, based on Customer action) If the translation needs rework, the
* customer can REJECT it for further cleanup.
*/
REJECTED, // CQ-37143, i18n.get("REJECTED"), workaround to get this string extracted for translation without touching code
/**
* APPROVED. (Set by the Translation Framework, based on Customer action) If the translation is satisfactory,
* the customer can APPROVE it.
*/
APPROVED, // CQ-37143, i18n.get("APPROVED"), workaround to get this string extracted for translation without touching code
/**
* COMPLETE. (Set by the Translation Framework, based on Customer action) The translation, payment and
* everything about the asset in question is COMPLETE. At this point, vendors should go ahead and update the
* translation memory.
*/
COMPLETE, // CQ-37143, i18n.get("COMPLETE"), workaround to get this string extracted for translation without touching code
/**
* CANCEL. (Set by the Translation Framework, based on Customer action) This is an intimation to the
* Translation provider to CANCEL the translation process. This can occur after Scoping or even when
* translation is in progress for a large job.
*/
CANCEL, // CQ-37143, i18n.get("CANCEL"), workaround to get this string extracted for translation without touching code
/**
* ARCHIVE. (Set by the Translation Framework, based on Customer action) If a translation job is COMPLETE, or
* CANCELED and doesn't belong in the UI anymore, the customer can choose to archive it.
*/
ARCHIVE, // CQ-37143, i18n.get("ARCHIVE"), workaround to get this string extracted for translation without touching code
/**
* ERROR_UPDATE. (Set by the Translation Framework/Vendor) Indicates an ERROR in Synchronization. This could
* occur when uploading an asset to the vendor goes wrong or importing a translation fails.
*/
ERROR_UPDATE, // CQ-37143, i18n.get("ERROR_UPDATE"), workaround to get this string extracted for translation without touching code
/**
* UNKNOWN_STATE. (Set by the Translation Framework) Generic Try/Catch loop to cover any unforeseen ERRORS.
*/
UNKNOWN_STATE; // CQ-37143, i18n.get("UNKNOWN_STATE"), workaround to get this string extracted for translation without touching code
public static TranslationStatus fromString(String text) {
if (text != null) {
for (TranslationStatus b : TranslationStatus.values()) {
if (text.equalsIgnoreCase(b.toString())) {
return b;
}
}
}
return UNKNOWN_STATE;
}
}
/** Type of content data (plain text, html, etc...) */
public static enum ContentType {
/** plain. */
PLAIN("text/plain"),
/** html. */
HTML("text/html");
/** type. */
private String type;
/**
* Instantiates a new content type.
* @param type type
*/
private ContentType(final String type) {
this.type = type;
}
/**
* Gets type.
* @return type
*/
public String getType() {
return this.type;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy