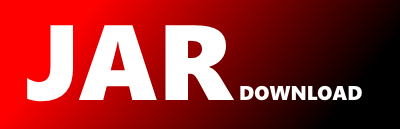
com.adobe.granite.translation.api.TranslationObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2014 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.translation.api;
import java.io.InputStream;
import java.util.List;
import java.util.Map;
import java.util.zip.ZipInputStream;
import com.adobe.granite.comments.Comment;
import com.adobe.granite.comments.CommentCollection;
import org.osgi.annotation.versioning.ProviderType;
//
/** The Interface TranslationObject. */
@ProviderType
public interface TranslationObject {
/**
* Gets the title of the TranslationObject. Title is auto-populated from TranslationObject.
* @return the title
*/
String getTitle();
/**
* Gets the path to the Translation Object being translated. The path returned is to where the target Translation
* Object is stored in AEM CRX de. The path is derived by coping that of the the source Translation Object.
* @return the content url
*/
String getTranslationObjectTargetPath();
/**
* Gets the path to the source Translation Object. The path returned is to the where the source Translation Object
* is stored in AEM CRX de.
* @return the source content url
*/
String getTranslationObjectSourcePath();
/**
* Gets the version name of the source Object. If this name is null, then latest version of source content is
* used.
* @return the version name
*/
String getSourceVersion();
/**
* Gets the mime type of TranslationObject.
* @return the mime type
*/
String getMimeType();
/**
* Gets confirmation return from the external translation service provider that they have received the
* TranslationObject for translation. Translation service provider returns a unique TranslationObjectId.
* @return Translation Object Id
*/
String getId();
/**
* Gets the comment collection. This returns all the comments added to the TranslationObject.
* @return the comment Collection
*/
CommentCollection getCommentCollection();
/**
* Gets the supporting translation objects list for each relation. Each TranslationObject can have a list of
* supporting translation objects which add reference to the TranslationObject.
* @return the Map of supporting translation objects list, key contains the relation ship name like source,
* variant, reference and value of that key is List of TranslationObject
*/
Map> getSupportingTranslationObjectsIterator() throws TranslationException;
/**
* Gets the supporting translation objects count.
* @return the supporting translation objects count
*/
@Deprecated
int getSupportingTranslationObjectsCount() throws TranslationException;
/**
* Gets the Translation Job metadata
* @return the Translation Job metadata
*/
TranslationMetadata getTranslationJobMetadata();
/**
* @deprecated Use {@link #getTranslationObjectXMLInputStream()} instead. Gets XML input stream for the
* Translation Object to be translated.
* @return the translation Object input stream
* @throws TranslationException the translation exception
*/
@Deprecated
InputStream getTranslationObjectInputStream() throws TranslationException;
/**
* Gets XML input stream for the Translation Object to be translated.
* @return The translation Object XML input stream
* @throws TranslationException the translation exception
*/
InputStream getTranslationObjectXMLInputStream() throws TranslationException;
/**
* Gets XLIFF input stream for the Translation Object to be translated.
* @param xliffVersion Requested version of XLIFF
* @return The translation Object XLIFF input stream
* @throws TranslationException the translation exception
*/
InputStream getTranslationObjectXLIFFInputStream(String xliffVersion) throws TranslationException;
/**
* Gets JSON input stream for the Translation Object to be translated.
* @return The translation Object JSON input stream
* @throws TranslationException the translation exception
*/
InputStream getTranslationObjectJSONInputStream() throws TranslationException;
/**
* Gets the translated Object input stream. Returns null in case TranslationObject was not translated.
* @return the translated Object input stream
*/
InputStream getTranslatedObjectInputStream();
/**
* Gets a zip input stream containing the preview of Translation Object. This zip contains all the files required
* for offline preview of the translation object.
* @return Zip input stream, containing the preview of Translation Object.
*/
ZipInputStream getTranslationObjectPreview();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy