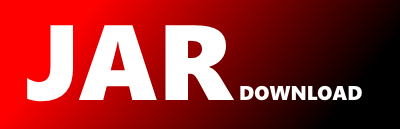
com.adobe.granite.ui.clientlibs.HtmlLibraryManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2011 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.ui.clientlibs;
import java.io.IOException;
import java.io.Writer;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
import javax.jcr.RepositoryException;
import org.apache.sling.api.SlingHttpServletRequest;
import org.osgi.annotation.versioning.ProviderType;
/**
* HtmlLibraryManager
provides access to repository defined
* html libraries.
*/
@ProviderType
public interface HtmlLibraryManager {
/**
* request parameter name for enabling debug console (firebug + cq logging)
*/
String PARAM_DEBUG_CONSOLE = "debugConsole";
/**
* request parameter name for turning on HtmlLibraryServlet debugging
*/
String PARAM_DEBUG_CLIENT_LIBS = "debugClientLibs";
/**
* request parameter name for testing a theme
*/
String PARAM_FORCE_THEME = "forceTheme";
/**
* Request attribute flag that forces the inclusion of the CQURLInfo
* @since 5.5.42
*/
String REQUEST_ATTR_FORCE_CQ_URLINFO = "com.day.cq.widget.htmllibrarymanager.forceurlinfo";
/**
* Writes the JS include snippets to the given writer. The paths to the
* JS libraries are included that match the given categories. Note that
* themed and non-themed libraries are included. If the request contains
* a {@value #PARAM_FORCE_THEME} parameter, the themed libraries are
* overlaid with their respective counterparts with that given theme.
*
* @param request request
* @param out writer
* @param categories categories
* @throws java.io.IOException if an I/O error occurs
*/
void writeJsInclude(SlingHttpServletRequest request, Writer out, String... categories)
throws IOException;
/**
* Writes the JS include snippets to the given writer. The paths to the
* JS libraries are included that match the given categories.
* If themed
is false
, only non-themed
* libraries are included.
* If themed
is true
only themed libraries are
* included and if the request contains a {@value #PARAM_FORCE_THEME}
* parameter, the themed libraries are overlaid with their respective
* counterparts with that given theme.
*
* @param request request
* @param out writer
* @param themed controls if themed or non themed libraries should be included
* @param categories categories
* @throws java.io.IOException if an I/O error occurs
*
* @since 5.4
*/
void writeJsInclude(SlingHttpServletRequest request, Writer out,
boolean themed, String... categories)
throws IOException;
/**
* Writes the CSS include snippets to the given writer. The paths to the
* CSS libraries are included that match the given categories. Note that
* themed and non-themed libraries are included. If the request contains
* a {@value #PARAM_FORCE_THEME} parameter, the themed libraries are
* overlaid with their respective counterparts with that given theme.
*
* @param request request
* @param out writer
* @param categories categories
* @throws java.io.IOException if an I/O error occurs
*/
void writeCssInclude(SlingHttpServletRequest request, Writer out, String... categories)
throws IOException;
/**
* Writes the CSS include snippets to the given writer. The paths to the
* CSS libraries are included that match the given categories.
* If themed
is false
, only non-themed
* libraries are included.
* If themed
is true
only themed libraries are
* included and if the request contains a {@value #PARAM_FORCE_THEME}
* parameter, the themed libraries are overlaid with their respective
* counterparts with that given theme.
*
* @param request request
* @param out writer
* @param themed controls if themed or non themed libraries should be included
* @param categories categories
* @throws java.io.IOException if an I/O error occurs
*
* @since 5.4
*/
void writeCssInclude(SlingHttpServletRequest request, Writer out,
boolean themed, String... categories)
throws IOException;
/**
* Writes all CSS and only themed JS include snippets to the given writer.
* The paths to the libraries are included that match the given categories.
* If the request contains a {@value #PARAM_FORCE_THEME} parameter, the
* themed libraries are overlaid with their respective counterparts with
* that given theme.
*
* Please note, that the theme include should happen after the js includes
* since the theme js might reference themes.
*
* @param request request
* @param out writer
* @param categories categories
* @throws java.io.IOException if an I/O error occurs
*/
void writeThemeInclude(SlingHttpServletRequest request, Writer out, String... categories)
throws IOException;
/**
* Writes the include snippets to the given writer. The paths to the
* libraries are included that match the given categories and the theme
* name that is extracted from the request.
*
* Same as:
*
* writeCssInclude(...);
* writeJsInclude(...);
* writeThemeInclude(...);
*
*
* If one of the libraries to be included has assigned channels, then the
* inclusion is delegated to the client side library manager.
*
* @param request request
* @param out writer
* @param categories categories
* @throws java.io.IOException if an I/O error occurs
*/
void writeIncludes(SlingHttpServletRequest request, Writer out, String... categories)
throws IOException;
/**
* Returns the html library that is configured at the given path. If no
* such library exists, null
is returned.
*
* @param type the library type
* @param path the path
* @return the library or null
*/
HtmlLibrary getLibrary(LibraryType type, String path);
/**
* Returns the html library that is address by the given request. if no
* such library exists, null
is returned.
*
* @param request the request
* @return the library or null
*/
HtmlLibrary getLibrary(SlingHttpServletRequest request);
/**
* Checks if library minification is enabled.
* @return true
if minification is enabled.
*/
boolean isMinifyEnabled();
/**
* Checks if debug support is enabled.
* @return true
if debug is enabled.
*/
boolean isDebugEnabled();
/**
* Checks if gzip compression is enabled.
* @return true
if gzip is enabled.
*/
boolean isGzipEnabled();
/**
* Returns all client libraries
* @return all client libraries
*
* @since 5.4
*/
Map getLibraries();
/**
* Returns all client libraries that match the specified filters. If a theme
* name is specified, only themed libraries are returned, otherwise only
* non-theme libraries are returned. If theme name is an empty string,
* the configured default theme is used.
*
* @param categories the categories
* @param type type or null
to match all types
* @param ignoreThemed true
to filter out themed libraries
* @param transitive true
to resolve recursively
* @return matching client libraries
*
* @since 5.4
*/
Collection getLibraries(String[] categories,
LibraryType type,
boolean ignoreThemed,
boolean transitive);
/**
* Returns all themed client libraries that match the type. If type is
* null
all libraries are returned. If theme name is an empty
* string, the configured default theme is used. If theme name is null
* all themed libraries are returned.
*
* @param categories the categories
* @param type type or null
to match all types
* @param themeName theme name or null
* @param transitive true
to resolve recursively
* @return matching client libraries
*
* @since 5.4
*/
Collection getThemeLibraries(String[] categories,
LibraryType type,
String themeName,
boolean transitive);
/**
* Returns all paths including static resources associated with the
* provided list of clientlib categories.
*
* @param categories the categories
* @since 6.6
*/
Set getLibrariesPaths(String[] categories);
/**
* Invalidates the output cache.
* @throws RepositoryException if an error occurrs
* @since 6.4
*/
void invalidateOutputCache() throws RepositoryException;
/**
* Ensures that all client libraries are compiled
*
* @throws IOException if an error occurs
* @throws RepositoryException if an error occurs
*/
void ensureCached() throws IOException, RepositoryException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy