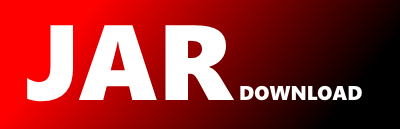
com.adobe.granite.workflow.event.WorkflowEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.workflow.event;
import java.util.Date;
import java.util.Dictionary;
import org.osgi.service.event.Event;
import com.adobe.granite.workflow.exec.WorkItem;
import com.adobe.granite.workflow.exec.Workflow;
import com.adobe.granite.workflow.exec.WorkflowData;
import com.adobe.granite.workflow.model.WorkflowModel;
import com.adobe.granite.workflow.model.WorkflowNode;
/**
* WorkflowEvent
provides an extension of {@link Event} that is
* used for propagating workflow related information as OSGI events.
*/
public class WorkflowEvent extends Event {
/**
* The job topic for adding an entry to the audit log.
*/
public static final String EVENT_TOPIC = "com/adobe/granite/workflow/event";
/**
* Time stamp of the time the event was created.
*/
public static final String TIME_STAMP = "TimeStamp";
/**
* The user whose that has triggered the event.
*/
public static final String USER = "User";
/**
* The name of the {@link WorkflowModel} the event relates to.
*/
public static final String WORKFLOW_NAME = "WorkflowName";
/**
* The version of the {@link WorkflowModel} the event relates to.
*/
public static final String WORKFLOW_VERSION = "WorkflowVersion";
/**
* The node of the {@link WorkflowModel} the event relates to.
*/
public static final String WORKFLOW_NODE = "WorkflowNode";
/**
* The ID of the {@link Workflow} instance the event relates to.
*/
public static final String WORKFLOW_INSTANCE_ID = "WorkflowInstanceId";
/**
* ID of the parent {@link Workflow} of the {@link Workflow} instance the
* event relates to.
*/
public static final String PARENT_WORKFLOW_ID = "ParentWorkflowId";
/**
* The {@link WorkflowData} that relates to the event.
*/
public static final String WORK_DATA = "Workdata";
/**
* The {@link WorkItem} that relates to the event.
*/
public static final String WORK_ITEM = "Workitem";
/**
* The {@link WorkflowNode} that is the source of a
* {@link #NODE_TRANSITION_EVENT}.
*/
public static final String FROM_NODE_NAME = "fromNodeName";
/**
* The {@link WorkflowNode} that is the target of a
* {@link #NODE_TRANSITION_EVENT}.
*/
public static final String TO_NODE_NAME = "toNodeName";
/**
* The name of the variable that has changed when a
* {@link #VARIABLE_UPDATE_EVENT} is triggered.
*/
public static final String VARIABLE_NAME = "VariableName";
/**
* The new value of the variable that has changed when a
* {@link #VARIABLE_UPDATE_EVENT} is triggered.
*/
public static final String VARIABLE_VALUE = "VariableValue";
/**
* Delegate name
*/
public static final String DELEGATEE = "Delagatee";
/**
* The name of the user to whom the workItem is returned
*/
public static final String RETURNED_TO = "ReturnedTo";
// ---- Event types --------------------------------------------------------
/**
* The type of the event. Can be one of
*
* - {@link #MODEL_DEPLOYED_EVENT}
* - {@link #WORKFLOW_STARTED_EVENT}
* - {@link #WORKFLOW_ABORTED_EVENT}
* - {@link #WORKFLOW_SUSPENDED_EVENT}
* - {@link #WORKFLOW_RESUMED_EVENT}
* - {@link #WORKFLOW_COMPLETED_EVENT}
* - {@link #NODE_TRANSITION_EVENT}
* - {@link #VARIABLE_UPDATE_EVENT}
* - {@link #PROCESS_TIMEOUT_EVENT}
* - {@link #WORKITEM_DELEGATION_EVENT}
*
*/
public static final String EVENT_TYPE = "EventType";
public static final String MODEL_DEPLOYED_EVENT = "ModelDeployed";
public static final String MODEL_DELETED_EVENT = "ModelDeleted";
public static final String WORKFLOW_STARTED_EVENT = "WorkflowStarted";
public static final String WORKFLOW_ABORTED_EVENT = "WorkflowAborted";
public static final String WORKFLOW_SUSPENDED_EVENT = "WorkflowSuspended";
public static final String WORKFLOW_RESUMED_EVENT = "WorkflowResumed";
public static final String WORKFLOW_COMPLETED_EVENT = "WorkflowCompleted";
public static final String NODE_TRANSITION_EVENT = "NodeTransition";
public static final String VARIABLE_UPDATE_EVENT = "VariableUpdate";
public static final String PROCESS_TIMEOUT_EVENT = "ProcessTimeout";
public static final String WORKITEM_DELEGATION_EVENT = "WorkItemDelegated";
public static final String WORKITEM_UNCLAIM_EVENT = "WorkItemUnclaimed";
public static final String WORKITEM_CLAIM_EVENT = "WorkItemClaimed";
public static final String JOB_FAILED_EVENT = "JobFailed";
public static final String RESOURCE_COLLECTION_MODIFIED = "ResourceCollectionModified";
/**
* Default constructor used for creating WorkflowEvent
* instances.
*
* @param props The properties assigned to the event.
*/
public WorkflowEvent(Dictionary, ?> props) {
super(EVENT_TOPIC, getUntypedDictionary(props));
}
private static final Dictionary getUntypedDictionary(Dictionary p) {
return p;
}
/**
* Time stamp of the time the event was created.
* @return the time stamp of the time the event was created
*/
public Date getTimeStamp() {
return (Date)getProperty(TIME_STAMP);
}
/**
* The user who triggered the event.
* @return the user who triggered the event
*/
public String getUser() {
return (String)getProperty(USER);
}
/**
* The name of the {@link WorkflowModel} the event relates to.
* @return the name of the {@link WorkflowModel} the event relates to
*/
public String getWorkflowName() {
return (String) getProperty(WORKFLOW_NAME);
}
/**
* The version of the {@link WorkflowModel} the event relates to.
* @return the version of the {@link WorkflowModel} the event relates to
*/
public String getWorkflowVersion() {
return (String) getProperty(WORKFLOW_VERSION);
}
/**
* The node of the {@link WorkflowModel} the event relates to.
* @return the node of the {@link WorkflowModel} the event relates to
*/
public String getWorkflowNode() {
return (String) getProperty(WORKFLOW_NODE);
}
/**
* The ID of the {@link Workflow} instance the event relates to.
* @return the ID of the {@link Workflow} instance the event relates to
*/
public String getWorkflowInstanceId() {
return (String) getProperty(WORKFLOW_INSTANCE_ID);
}
/**
* ID of the parent {@link Workflow} of the {@link Workflow} instance the
* event relates to.
* @return the ID of the parent {@link Workflow} of the {@link Workflow} instance the
* event relates to
*/
public String getParentWorkflowId() {
return (String) getProperty(PARENT_WORKFLOW_ID);
}
/**
* The {@link WorkflowData} that relates to the event.
* @return the {@link WorkflowData} that relates to the event
*/
public WorkflowData getWorkflowData() {
return (WorkflowData) getProperty(WORK_DATA);
}
/**
* The {@link WorkItem} that relates to the event.
* @return the {@link WorkItem} that relates to the event
*/
public WorkItem getWorkItem() {
return (WorkItem) getProperty(WORK_ITEM);
}
/**
* The {@link WorkflowNode} that is the source of a
* {@link #NODE_TRANSITION_EVENT}.
* @return the {@link WorkflowNode} that is the source of a
* {@link #NODE_TRANSITION_EVENT}
*/
public String getFromNodeName() {
return (String) getProperty(FROM_NODE_NAME);
}
/**
* The {@link WorkflowNode} that is the target of a
* {@link #NODE_TRANSITION_EVENT}.
* @return the {@link WorkflowNode} that is the target of a
* {@link #NODE_TRANSITION_EVENT}
*/
public String getToNodeName() {
return (String) getProperty(TO_NODE_NAME);
}
/**
* The name of the variable that has changed when a
* {@link #VARIABLE_UPDATE_EVENT} is triggered.
* @return the name of the variable that has changed when a
* {@link #VARIABLE_UPDATE_EVENT} is triggered
*/
public String getVariableName() {
return (String) getProperty(VARIABLE_NAME);
}
/**
* The new value of the variable that has changed when a
* {@link #VARIABLE_UPDATE_EVENT} is triggered.
* @return the new value of the variable that has changed when a
* {@link #VARIABLE_UPDATE_EVENT} is triggered
*/
public Object getVariableValue() {
return getProperty(VARIABLE_VALUE);
}
/**
* Delegate name
* @return the delegate name
*/
public String getDelegateName() {
return (String) getProperty(DELEGATEE);
}
/**
* Returns the name of the user to whom the workItem is returned
* @return user name of the user to whom the workitem is returned.
*/
public String getReturnedToName() {
return (String) getProperty(RETURNED_TO);
}
/**
* The type of the event. Can be one of
*
* - {@link #MODEL_DEPLOYED_EVENT}
* - {@link #WORKFLOW_STARTED_EVENT}
* - {@link #WORKFLOW_ABORTED_EVENT}
* - {@link #WORKFLOW_SUSPENDED_EVENT}
* - {@link #WORKFLOW_RESUMED_EVENT}
* - {@link #WORKFLOW_COMPLETED_EVENT}
* - {@link #NODE_TRANSITION_EVENT}
* - {@link #VARIABLE_UPDATE_EVENT}
* - {@link #PROCESS_TIMEOUT_EVENT}
* - {@link #WORKITEM_DELEGATION_EVENT}
*
* @return the event type
*/
public String getEventType() {
return (String) getProperty(EVENT_TYPE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy