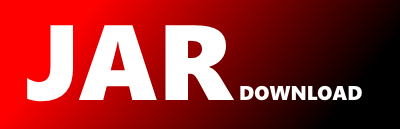
com.adobe.internal.io.ByteWriterFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: ByteWriterFactory.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.internal.io;
import java.io.IOException;
/**
* Defines a factory for the construction of {@link com.adobe.internal.io.ByteWriter ByteWriter}
* instances based on requested parameters.
*/
public interface ByteWriterFactory
{
/**
* Request a single {@link com.adobe.internal.io.ByteWriter ByteWriter} with the given
* properties. An implementation is free to ignore the properties or to use them in any
* way that it views appropriate in choosing the right kind of
* {@link com.adobe.internal.io.ByteWriter ByteWriter} to return. These should be viewed
* as "advice" to the implementation about the intended usage.
* @param longevity anticipated lifetime of the {@link com.adobe.internal.io.ByteWriter ByteWriter}
* @param encryption encryption status of the data to be placed into the {@link com.adobe.internal.io.ByteWriter ByteWriter}
* @param fixed whether the size given is the maximum data that will ever be placed into the {@link com.adobe.internal.io.ByteWriter ByteWriter}
* @param size either the maximum size of the data or an estimate of the size depending on the value of the fixed
parameter
* @param fast the requested {@link com.adobe.internal.io.ByteWriter ByteWriter} needs to be fast
* @return a {@link com.adobe.internal.io.ByteWriter ByteWriter} matching your properties to some degree of "match"
* @throws IOException
*/
ByteWriter getByteWriter(Longevity longevity, EncryptionStatus encryption,
Fixed fixed, long size, boolean fast)
throws IOException;
/**
* Request an array {@link com.adobe.internal.io.ByteWriter ByteWriter} instances with the given
* properties. An implementation is free to ignore the properties or to use them in any
* way that it views appropriate in choosing the right kind of
* {@link com.adobe.internal.io.ByteWriter ByteWriter} to return. These should be viewed
* as "advice" to the implementation about the intended usage.
* @param longevity anticipated lifetime of the {@link com.adobe.internal.io.ByteWriter ByteWriter}
* @param encryption encryption status of the data to be placed into the {@link com.adobe.internal.io.ByteWriter ByteWriter}
* @param fixed whether the size given is the maximum data that will ever be placed into the {@link com.adobe.internal.io.ByteWriter ByteWriter}
* @param size either the maximum size of the data or an estimate of the size depending on the value of the fixed
parameter
* @param fast the requested {@link com.adobe.internal.io.ByteWriter ByteWriter} needs to be fast
* @return a {@link com.adobe.internal.io.ByteWriter ByteWriter} matching your properties to some degree of "match"
* @throws IOException
*/
ByteWriter[] getByteWriter(Longevity longevity, EncryptionStatus encryption,
Fixed fixed, long[] size, boolean fast)
throws IOException;
/**
* The caller has finished with the given {@link com.adobe.internal.io.ByteWriter ByteWriter}
* and will no longer use it again. The implementation can close it.
* @param byteWriter a no longer needed {@link com.adobe.internal.io.ByteWriter ByteWriter}
* @throws IOException
*/
void closeByteWriter(ByteWriter byteWriter)
throws IOException;
/**
* The ByteWriterFactory
is no longer needed and it should close down
* and free all resources allocated. All {@link com.adobe.internal.io.ByteWriter ByteWriter}
* instances created by this factory can and may be destroyed during this process.
* @throws IOException
*/
void closeFactory()
throws IOException;
/**
* An enumeration for the anticipated longevity of a requested instance.
*/
public static class Longevity
{
private final int id;
private final String name;
private Longevity(int id1, String name)
{
// this class provides an enum
this.id = id1;
this.name = name.intern();
}
/**
* Get the name of the generic family.
* @return the name of the generic family
*/
public String name()
{
return this.name;
}
/**
* Anticipated lifetime is below that of a single method call.
*/
public static final Longevity TEMPORARY = new Longevity (0, "TEMPORARY");
/**
* Anticipated lifetime is from about a single method call up to several method calls.
*/
public static final Longevity SHORT = new Longevity (1, "SHORT");
/**
* Anticipated lifetime is approximately that of the document being processed.
*/
public static final Longevity LONG = new Longevity (2, "LONG");
/**
* Anticipated lifetime is beyond that of the document being processed.
*/
public static final Longevity PERMANENT = new Longevity (3, "PERMANENT");
private static final Longevity[] allValues
= {TEMPORARY, SHORT, LONG, PERMANENT};
public static Longevity parse (String s)
{
for (int i = 0; i < allValues.length; i++)
{
if (allValues [i].name.compareToIgnoreCase (s) == 0)
{
return allValues [i];
}
}
return null;
}
public int getId() {
return id;
}
}
/**
* An enumeration for the "fixedness" of a requested instance.
*/
public static class Fixed
{
private final int id;
private final String name;
private Fixed(int id, String name)
{
// this class provides an enum
this.id = id;
this.name = name.intern();
}
/**
* Get the name of the generic family.
* @return the name of the generic family
*/
public String name()
{
return this.name;
}
/**
* Request a FIXED size.
*/
public static final Fixed FIXED = new Fixed (0, "FIXED");
/**
* Request a GROWABLE size.
*/
public static final Fixed GROWABLE = new Fixed (1, "GROWABLE");
private static final Fixed[] allValues
= {FIXED, GROWABLE};
public static Fixed parse (String s)
{
for (int i = 0; i < allValues.length; i++)
{
if (allValues [i].name.compareToIgnoreCase (s) == 0)
{
return allValues [i];
}
}
return null;
}
public int getId() {
return id;
}
}
/**
* An enumeration for the encryptioni status of data to placed in a requested instance.
*/
public static class EncryptionStatus
{
private final int id;
private final String name;
private EncryptionStatus(int id, String name)
{
// this class provides an enum
this.id = id;
this.name = name.intern();
}
/**
* Get the name of the generic family.
* @return the name of the generic family
*/
public String name()
{
return this.name;
}
/**
* Data is not encrypted nor is it decrypted.
*/
public static final EncryptionStatus CLEAR = new EncryptionStatus (0, "CLEAR");
/**
* Data is encrypted.
*/
public static final EncryptionStatus ENCRYPTED = new EncryptionStatus (1, "ENCRYPTED");
/**
* Data is decrypted.
*/
public static final EncryptionStatus DECRYPTED = new EncryptionStatus (1, "DECRYPTED");
private static final EncryptionStatus[] allValues
= {CLEAR, ENCRYPTED, DECRYPTED};
public static EncryptionStatus parse (String s)
{
for (int i = 0; i < allValues.length; i++)
{
if (allValues [i].name.compareToIgnoreCase (s) == 0)
{
return allValues [i];
}
}
return null;
}
public int getId() {
return id;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy