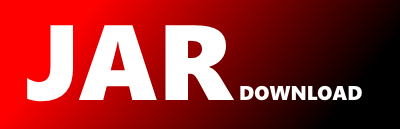
com.adobe.internal.io.InputStreamByteWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: InputStreamByteWriter.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.internal.io;
import java.io.IOException;
import java.io.InputStream;
import java.io.RandomAccessFile;
/**
* This class provides convenience functionality to take an InputStream
* and buffer the bytes from it and create an appropriate ByteReader
* implementation to wrap those bytes.
*/
public final class InputStreamByteWriter implements ByteWriter
{
private ByteWriter byteWriter;
/**
* Creates a new InputStreamByteWriter from the given InputStream.
* This reads all of the bytes from the InputStream into memory and then
* wraps those bytes with a ByteArrayByteReader. Once the constructor returns
* the stream will have been fully read and closed.
* @param inputStream the source of the bytes.
* @throws IOException
*/
public InputStreamByteWriter(InputStream inputStream) throws IOException
{
ByteArrayByteWriter writer = new ByteArrayByteWriter();
byte[] buffer = new byte[4096];
int bytesRead;
long position = 0;
while ((bytesRead = inputStream.read(buffer)) != -1)
{
writer.write(position, buffer, 0, bytesRead);
position += bytesRead;
}
this.byteWriter = writer;
inputStream.close();
}
/**
* Creates a new InputStreamByteWriter from the given InputStream.
* This reads all of the bytes from the InputStream into the
* RandomAccessFile provided and then wraps that file with a
* RandomAccessFileByteReader. Once the constructor returnss the stream
* will have been fully read and closed. The provided RandomAccessFile
* must have read and write access.
* @param inputStream the source of the bytes.
* @param raf the location to buffer those bytes to.
* @throws IOException
*/
public InputStreamByteWriter(InputStream inputStream, RandomAccessFile raf) throws IOException
{
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1)
{
raf.write(buffer, 0, bytesRead);
}
this.byteWriter = new RandomAccessFileByteWriter(raf);
inputStream.close();
}
/**
* @see com.adobe.internal.io.ByteReader#read(long)
*/
public int read(long position) throws IOException
{
return this.byteWriter.read(position);
}
/**
* @see com.adobe.internal.io.ByteReader#read(long, byte[], int, int)
*/
public int read(long position, byte[] b, int offset, int length) throws IOException
{
return this.byteWriter.read(position, b, offset, length);
}
/**
* @see com.adobe.internal.io.ByteReader#length()
*/
public long length() throws IOException
{
return this.byteWriter.length();
}
/**
* @see com.adobe.internal.io.ByteReader#close()
*/
public void close() throws IOException
{
this.byteWriter.close();
}
/**
* @see java.lang.Object#toString()
*/
public String toString()
{
return this.byteWriter.toString();
}
/**
* Write the byte given at the position given.
* @param position the zero-based offset within the byte array.
* @param b the byte to write.
* @throws IOException
*/
public void write(long position, int b) throws IOException
{
this.byteWriter.write(position,b);
}
/**
* Write an array of bytes at the position given.
* @param position the zero-based offset within the byte array.
* @param b the array of bytes to write from.
* @param offset the offset within the byte array to start writing from.
* @param length the number of bytes to write from the byte array.
* @throws IOException
*/
public void write(long position, byte[] b, int offset, int length) throws IOException
{
this.byteWriter.write(position,b,offset,length);
}
/**
* Flushes this ByteWriter
and forces any buffered output bytes to be written out.
* @throws IOException
*/
public void flush() throws IOException
{
this.byteWriter.flush();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy