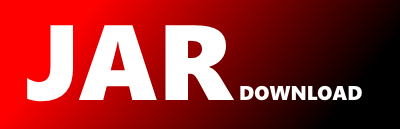
com.adobe.internal.io.NonCachedRandomAccessFileByteWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: RandomAccessFileByteWriter.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.internal.io;
import java.io.IOException;
import java.io.RandomAccessFile;
/**
* This class implements a ByteWriter in which the bytes are written to
* a {@link java.io.RandomAccessFile RandomAccessFile}.
*/
public class NonCachedRandomAccessFileByteWriter implements ByteWriter
{
private RandomAccessFile file;
// private long length;
/**
* Create a new RandomAccessFileByteWriter using the provided
* {@link java.io.RandomAccessFile RandomAccessFile}.
* This {@link java.io.RandomAccessFile RandomAccessFile} should not be written to
* while it is being used by this RandomAccessFileByteWriter.
* @param file the {@link java.io.RandomAccessFile RandomAccessFile} to use.
* @throws IOException
*/
public NonCachedRandomAccessFileByteWriter(RandomAccessFile file) throws IOException
{
this.file = file;
//this.length = this.file.length();
}
/**
* @see com.adobe.internal.io.ByteWriter#write(long, byte[], int, int)
*/
public void write(long position, byte[] b, int offset, int length) throws IOException
{
// XXX synchronization
//synchronized(this.buffer)
{
this.file.seek(position);
this.file.write(b, offset, length);
}
}
/**
* @see com.adobe.internal.io.ByteWriter#write(long, int)
*/
public void write(long position, int b) throws IOException
{
// XXX synchronization
//synchronized(this.buffer)
{
this.file.seek(position);
this.file.write(b);
}
}
/**
* @see com.adobe.internal.io.ByteWriter#length()
*/
public long length() throws IOException
{
return this.file.length();
}
/**
* @see com.adobe.internal.io.ByteWriter#flush()
*/
public void flush() throws IOException
{
// RandomAccessFile's don't implement flush()
}
/**
* @see com.adobe.internal.io.ByteWriter#close()
*/
public void close() throws IOException
{
this.file.close();
}
/**
* @see java.lang.Object#toString()
*/
public String toString()
{
// TODO Auto-generated method stub
return this.file.toString();
}
/************************ Reader methods ************************/
/**
* @see com.adobe.internal.io.ByteReader#read(long)
*/
public int read(long position) throws IOException
{
if ((position < 0) || (position >= this.length()))
{
return ByteReader.EOF;
}
// XXX synchronization
//synchronized(this.file)
{
this.file.seek(position);
return this.file.read();
}
}
/**
* @see com.adobe.internal.io.ByteReader#read(long, byte[], int, int)
*/
public int read(long position, byte[] b, int offset, int length) throws IOException
{
if ((position < 0) || (position >= this.file.length()))
{
return ByteReader.EOF;
}
// XXX synchronization
//synchronized(this.file)
{
this.file.seek(position);
return this.file.read(b, offset, (int) Math.min(length, this.file.length() - position));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy