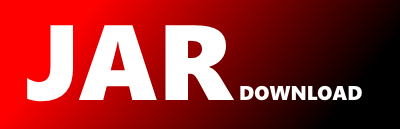
com.adobe.internal.io.stream.ByteReaderInputByteStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: ByteReaderInputByteStream.java
*
* ****************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2003-2006 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
* ***************************************************************************/
package com.adobe.internal.io.stream;
import java.io.IOException;
import com.adobe.internal.io.ByteReader;
/**
* Only for internal engineering use. This api can change without notice.
*
* This class provides access to a seekable input stream with two sets of methods. The
* first set are for internal use and will wrap all exceptions as PDFIOException
.
* The second set of methods implement the InputStream
interface and are for use
* when passing instances of this class to outside methods (i.e. in the JDK). These methods are
* marked as deprecated as a reminder to not use them internally.
*
* The actual underlying seekable source is provided by the outside client as an object that
* implements the ByteReader
interface. There can and will be many different implementations
* of the ByteReader
interface with different operating characteristics. So, make no
* assumptions about the speed of reading and seeking - do so judiciously.
*/
/*package protected*/ final class ByteReaderInputByteStream extends InputByteStreamImpl
{
private StreamManager streamManager;
private boolean isSlice = false;
public ByteReader byteReader = null;
private long position; // absolute offset in the 0-based ByteReader
private long start; // absolute offset in the 0-based ByteReader
private long length;
public boolean lengthInitialized;
private boolean registered = false;
/**
* Construct an InputByteStream from a ByteReader.
* @param byteReader
*/
/*package protected*/ ByteReaderInputByteStream(StreamManager streamManager, ByteReader byteReader, boolean register)
throws IOException
{
this(streamManager, byteReader, 0, -1, register);
}
/**
* Construct an InputByteStream from a ByteReader.
* @param byteReader
*/
/*package protected*/ ByteReaderInputByteStream(StreamManager streamManager, ByteReader byteReader,
long startOffset, long length, boolean register)
throws IOException
{
if (byteReader == null)
{
throw new IOException("Null ByteReader parameter.");
}
this.streamManager = streamManager;
this.byteReader = byteReader;
this.start = startOffset;
this.length = length;
this.lengthInitialized = false;
this.registered = register;
if (this.registered)
{
this.streamManager.registerInputByteStream(this, this.byteReader, this.isSlice);
}
}
/*package protected*/ ByteReaderInputByteStream(ByteReaderInputByteStream original, long startOffset, long length)
throws IOException
{
if ((startOffset < 0) || (length < 0) || ((startOffset + length) > original.length()))
{
throw new IOException("Invalid slice of Bytestream");
}
this.streamManager = original.streamManager;
this.byteReader = original.byteReader;
this.start = original.start + startOffset;
this.length = length;
this.position = this.start;
this.lengthInitialized = original.lengthInitialized;
this.isSlice = true;
this.registered = original.registered;
if (this.registered)
{
this.streamManager.registerInputByteStream(this, this.byteReader, this.isSlice);
}
}
/**
* Only for internal engineering use. This api can change without notice.
*/
/* (non-Javadoc)
* @see com.adobe.internal.pdftoolkit.core.cos.util.InputByteStream#slice(long, long)
*/
public InputByteStream slice(long begin, long length)
throws IOException
{
return new ByteReaderInputByteStream(this, begin, length);
}
/**
* Only for internal engineering use. This api can change without notice.
*/
/* (non-Javadoc)
* @see com.adobe.internal.pdftoolkit.core.cos.io.InputByteStream#release()
*/
public void close()
throws IOException
{
if (this.registered)
{
this.streamManager.deregisterInputByteStream(this, this.byteReader, this.isSlice);
} else {
if (!this.isSlice)
{
this.byteReader.close();
}
}
this.byteReader = null;
}
/**
* Only for internal engineering use. This api can change without notice.
*/
/* (non-Javadoc)
* @see com.adobe.internal.pdftoolkit.core.cos.util.InputByteStream#setPosition(long)
*/
public InputByteStream seek(long position)
throws IOException
{
if (position < 0)
{
position = 0;
}
if (position > this.length())
{
position = length();
}
this.position = position + this.start;
return this;
}
/**
* Only for internal engineering use. This api can change without notice.
*/
/* (non-Javadoc)
* @see com.adobe.internal.pdftoolkit.core.cos.util.InputByteStream#getPosition()
*/
public long getPosition()
throws IOException
{
return this.position - this.start;
}
/**
* Only for internal engineering use. This api can change without notice.
*/
/* (non-Javadoc)
* @see com.adobe.internal.pdftoolkit.core.cos.util.InputByteStream#limit()
*/
public long length()
throws IOException
{
if (!this.lengthInitialized)
{
if (this.length == -1)
{
this.length = this.byteReader.length();
} else {
this.length = Math.min(this.byteReader.length() - this.start, this.length);
}
this.lengthInitialized = true;
}
return this.length;
}
/**
* Only for internal engineering use. This api can change without notice.
* @throws IOException
*/
public int read()
throws IOException
{
int b = ByteReader.EOF;
if (((this.position - this.start) < this.length) || (!lengthInitialized && (this.getPosition() < this.length())))
{
b = this.byteReader.read(this.position);
this.position++;
}
return b;
}
/**
* Only for internal engineering use. This api can change without notice.
*/
public int read(byte[] b, int offset, int length)
throws IOException
{
int bytesRead;
length = (int) Math.min(length, this.length() - this.getPosition());
if (length == 0)
{
return InputByteStream.EOF;
}
bytesRead = this.byteReader.read(this.position, b, offset, length);
this.seek(this.getPosition() + bytesRead);
return bytesRead;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy