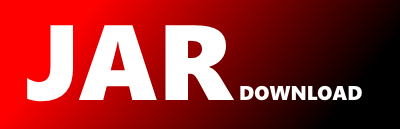
com.adobe.internal.io.stream.OutputByteStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.internal.io.stream;
import java.io.IOException;
/**
* A seekable OutputStream.
*/
public interface OutputByteStream
{
/**
* Only for internal engineering use. This api can change without notice.
*/
public static final int EOF = -1;
/**
* Only for internal engineering use. This api can change without notice.
*
* Write a byte at the current position. If the current position is at or beyond
* the end of the underlying data return a -1
. If not beyond the end
* of the underlying data the current position is incremented by 1.
*
* @throws IOException
*/
void write(int b)
throws IOException;
/**
* Only for internal engineering use. This api can change without notice.
*
* Write an array of bytes starting at the current position. The
* position is incremented by the length of the array that has been written.
* @param bytes The source array.
* @param offset The offset in the byte array to begin writing from.
* @param length The number of bytes to write.
* @throws IOException
*/
void write(byte[] bytes, int offset, int length)
throws IOException;
/**
* Only for internal engineering use. This api can change without notice.
*
* Write an array of bytes starting at the current position. The
* position is incremented by the length of the array that has been written.
* @param bytes The source array.
* @throws IOException
*/
void write(byte[] bytes)
throws IOException;
/**
* Only for internal engineering use. This api can change without notice.
*
* Set the current position in the underlying data.
* @param position Where to set the current position.
* @return This object.
* @throws IOException
*/
OutputByteStream seek(long position)
throws IOException;
/**
* Only for internal engineering use. This api can change without notice.
*
* Get the current position.
* @return The current position.
* @throws IOException
*/
long getPosition()
throws IOException;
/**
* Only for internal engineering use. This api can change without notice.
*
* The number of bytes that can exist in this OutputByteStream
.
*
* @return Total number of bytes available in this OutputByteStream
.
* @throws IOException
*/
long length()
throws IOException;
/**
* Only for internal engineering use. This api can change without notice.
*
* Is the current position of this OutputByteStream
at or beyond the
* end of the underlying data.
* @return True if the current position is beyond the end of the underlying data, false otherwise.
* @throws IOException
*/
boolean eof()
throws IOException;
/**
* Only for internal engineering use. This api can change without notice.
*/
void close()
throws IOException;
/**
* Only for internal engineering use. This api can change without notice.
*/
InputByteStream closeAndConvert()
throws IOException;
/**
* Only for internal engineering use. This api can change without notice.
*/
void flush()
throws IOException;
/**
* This will return a new OutputStream that wraps the same bytes as the
* OutputByteStream. Writing to or positioning either one will affect the other.
* This OutputStream may be safely closed without that causing the OutputByteStream
* to be closed.
*
* This is commonly used for external libraries that need an OutputStream. Do
* NOT use it to pass data around internally as this will generally be inefficient
* and cause conversion and memory usage.
* @return an OutputStream that wraps the same bytes
* @throws IOException
*/
SkippingOutputStream toOutputStream()
throws IOException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy