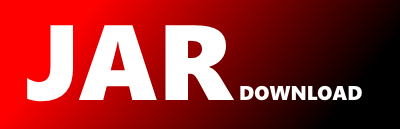
com.adobe.internal.util.WeakHashSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: WeakHashSet.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.internal.util;
import java.util.AbstractSet;
import java.util.Collection;
import java.util.Iterator;
import java.util.Set;
import java.util.WeakHashMap;
/**
* The WeakHashSet
is an implementation of the Set
* interface that uses a WeakHashMap
.
*
*/
public class WeakHashSet extends AbstractSet implements Set
{
private transient WeakHashMap map;
private static final Object dummy = new Object();
/**
*
*/
public WeakHashSet()
{
this.map = new WeakHashMap();
}
public WeakHashSet(Collection c)
{
this.map = new WeakHashMap(Math.max((int) (c.size() / 0.75f) + 1, 16));
addAll(c);
}
public WeakHashSet(int initialCapacity, float loadFactor)
{
this.map = new WeakHashMap(initialCapacity, loadFactor);
}
public WeakHashSet(int initialCapacity)
{
this.map = new WeakHashMap(initialCapacity);
}
/* (non-Javadoc)
* @see java.util.AbstractCollection#iterator()
*/
public Iterator iterator()
{
return this.map.keySet().iterator();
}
/* (non-Javadoc)
* @see java.util.AbstractCollection#size()
*/
public int size()
{
return this.map.size();
}
public boolean isEmpty()
{
return map.isEmpty();
}
public boolean contains(Object obj)
{
return map.containsKey(obj);
}
/* (non-Javadoc)
* @see java.util.AbstractCollection#add(java.lang.Object)
*/
public boolean add(Object obj)
{
return this.map.put(obj, dummy) == null;
}
public boolean remove(Object obj)
{
return this.map.remove(obj) == dummy;
}
public void clear()
{
this.map.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy