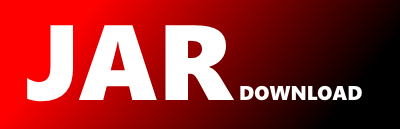
com.adobe.versioncue.nativecomm.IRequest Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/***************************************************************************/
/* */
/* ADOBE CONFIDENTIAL */
/* _ _ _ _ _ _ _ _ _ _ */
/* */
/* Copyright 2001-2002, Adobe Systems Incorporated */
/* All Rights Reserved. */
/* */
/* NOTICE: All information contained herein is, and remains the property */
/* of Adobe Systems Incorporated and its suppliers, if any. The */
/* intellectual and technical concepts contained herein are proprietary */
/* to Adobe Systems Incorporated and its suppliers and may be covered by */
/* U.S. and Foreign Patents, patents in process, and are protected by */
/* trade secret or copyright law. Dissemination of this information or */
/* reproduction of this material is strictly forbidden unless prior */
/* written permission is obtained from Adobe Systems Incorporated. */
/* */
/***************************************************************************/
package com.adobe.versioncue.nativecomm;
import java.nio.ByteBuffer;
import com.adobe.versioncue.nativecomm.msg.INCExternalizable;
import com.adobe.versioncue.nativecomm.msg.NCMap;
import com.adobe.versioncue.nativecomm.msg.NCType;
/**
* @author Timo Naroska
* @version $Revision: #1 $
*/
public interface IRequest
{
// ------------------------------------------------------------------------------------- Methods
/** @return INativeService to execute on
* @deprecated use {@link #service()} instead
*/
@Deprecated INativeService processPool();
/** @return INativeService to execute on */
INativeService service();
/** @return NativeService call name */
String call();
/** @return NativeService parameters dictionary */
NCMap params();
/**
* @param params NativeService parameters dictionary
* @return the IRequest
*/
IRequest params(NCMap params);
/** @return timeout of this request in milliseconds; 0 == no timeout */
int timeout();
/** Sets the timeout of this request in milliseconds
* @param timeout timeout of this request in milliseconds; 0 == no timeout
* @return the IRequest
*/
IRequest timeout(int timeout);
/** @return maximum number of retries for this request; 0 == do not retry */
int retries();
/** Sets the maximum number of retries for this request.
* @param retries maximum number of retries for this request; 0 == do not retry
* @return the IRequest
*/
IRequest retries(int retries);
/** @return the progress listener of this request */
IListener listener();
/** Sets the progress listener of this request
* @param listener progress listener of this request
* @return the IRequest
*/
IRequest listener(IListener listener);
/**
* Performs a synchronous message transaction with the underlying native process.
* The request is send to the next idle native process.
* The method then blocks until a response is received or the request times out.
* The response is returned to the caller.
*
* @return native process repsonse
* @exception NativeCommException on failure
*/
IResult execute() throws NativeCommException;
/** Add a request parameter
* @param name parameter name
* @param value parameter value
* @return the IRequest
*/
IRequest put(String name, NCType value);
/** Add a request parameter
* @param name parameter name
* @param value parameter value
* @return the IRequest
*/
IRequest put(String name, INCExternalizable value);
/** Add a request parameter
* @param name parameter name
* @param value parameter value
* @return the IRequest
*/
IRequest put(String name, String value);
/** Add a request parameter
* @param name parameter name
* @param value parameter value
* @return the IRequest
*/
IRequest put(String name, boolean value);
/** Add a request parameter
* @param name parameter name
* @param value parameter value
* @return the IRequest
*/
IRequest put(String name, int value);
/** Add a request parameter
* @param name parameter name
* @param value parameter value
* @return the IRequest
*/
IRequest put(String name, long value);
/** Add a request parameter
* @param name parameter name
* @param value parameter value
* @return the IRequest
*/
IRequest put(String name, double value);
/** Add a request parameter
* @param name parameter name
* @param value parameter value
* @return the IRequest
*/
IRequest put(String name, ByteBuffer value);
// --------------------------------------------------------------------------------------- Types
/** Listener interface to get progress information during request execution */
static interface IListener
{
/** Called to notify the listener about updated progress information for a request.
*
* @param request IRequest object being executed
* @param taskname current taskname
* @param progress the new progress value [0.0 ... 1.0]
* @throws ServiceAbortException if the request was aborted
*/
void progressChanged(IRequest request, String taskname, double progress)
throws ServiceAbortException;
}
}