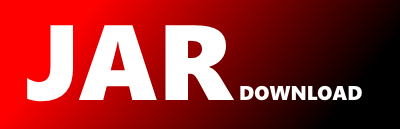
com.adobe.xfa.EnumAttr Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/* * ADOBE CONFIDENTIAL * * Copyright 2005 Adobe Systems Incorporated All Rights Reserved. * * NOTICE: All information contained herein is, and remains the property of * Adobe Systems Incorporated and its suppliers, if any. The intellectual and * technical concepts contained herein are proprietary to Adobe Systems * Incorporated and its suppliers and may be covered by U.S. and Foreign * Patents, patents in process, and are protected by trade secret or copyright * law. Dissemination of this information or reproduction of this material * is strictly forbidden unless prior written permission is obtained from * Adobe Systems Incorporated. */ package com.adobe.xfa; import com.adobe.xfa.ut.ExFull; import com.adobe.xfa.ut.FindBugsSuppress; import com.adobe.xfa.ut.ResId; /** * A class to represent the enumerated values of attributes. */ public final class EnumAttr { /* * We use a class to represent these enumerated values (rather than * simple C++/Java enums) so that these values are derived from Attribute * and can be used in the same contexts as Attribute. In practise, * there only ever one implementation/instance for each enumerated type. */ private final int mNum; private final String mName; private final int mVersionIntro; private final int mAvailability; private EnumAttr(int num, String name, int versionIntro, int availability) { mName = name; mNum = num; mVersionIntro = versionIntro; mAvailability = availability; } /** * @exclude from published api. */ public static final int ENUM_VALUE_MASK = 0x0000FFFF; /** * @exclude from published api. */ public static final int ENUM_TYPE_MASK = 0x3FFF0000; /** * @exclude from published api. */ public int getInt() { return mNum; } /** * @exclude from published api. */ public int getVersionIntro() { return mVersionIntro; } /** * @exclude from published api. */ public int getAvailability() { return mAvailability; } /** * @exclude from published api. */ static public EnumAttr getEnum(int value) { int x = (value & ENUM_TYPE_MASK) >> 16; int y = value & ENUM_VALUE_MASK; return gEnumValues[x][y]; } /** * return all the possible enumerated strings shared by this enum type. * @return an array of strings corresponding to this type. * * @exclude from published api. */ public String[] getStrings() { EnumAttr[] vals = getValues(); String[] strs = new String[vals.length]; for (int i=0; i
value. */ public static final int LOCKTYPE_UNSPECIFIED = EnumType.LOCKTYPE |0; /** * The LOCKTYPE's LOCKTYPE_READONLY value. */ public static final int LOCKTYPE_READONLY = EnumType.LOCKTYPE |1; /** * The LOCKTYPE's LOCKTYPE_PESSIMISTIC value. */ public static final int LOCKTYPE_PESSIMISTIC = EnumType.LOCKTYPE |2; /** * The LOCKTYPE's LOCKTYPE_OPTIMISTIC value. */ public static final int LOCKTYPE_OPTIMISTIC = EnumType.LOCKTYPE |3; /** * The LOCKTYPE's LOCKTYPE_BATCHOPTIMISTIC value. */ public static final int LOCKTYPE_BATCHOPTIMISTIC = EnumType.LOCKTYPE |4; /** * The incrementalload attribute's> 16]; } /** * @param t * @param v This String must be interned. * @exclude from published api. */ @FindBugsSuppress(code="ES") static public EnumAttr getEnum(EnumType t, String v) { int typePos = (t.getInt() & ~(EnumType.AMBIGUOUS_MASK | EnumType.ELEMENTAL_MASK)) >> 16; EnumAttr[] vals = gEnumValues[typePos]; for (int i = 0; i < vals.length; i++) { if (v == vals[i].toString()) return gEnumValues[typePos][i]; } throw new ExFull(ResId.InvalidEnumeratedValue, v); } /** * @exclude from published api. */ public static final int UNDEFINED = 0; /** * The access attribute's ACCESS_PROTECTED
value. */ public static final int ACCESS_PROTECTED = EnumType.ACCESS | 0; /** * The accessattribute's ACCESS_OPEN
value. */ public static final int ACCESS_OPEN = EnumType.ACCESS | 1; /** * The accessattribute's ACCESS_READONLY
value. */ public static final int ACCESS_READONLY = EnumType.ACCESS | 2; /** * The accessattribute's ACCESS_NONINTERACTIVE
value. */ public static final int ACCESS_NONINTERACTIVE = EnumType.ACCESS | 3; /** * The anchor attribute'sTOP_LEFT
value. */ public static final int TOP_LEFT = EnumType.ANCHOR | 0; /** * The anchor attribute'sTOP_CENTER
value. */ public static final int TOP_CENTER = EnumType.ANCHOR | 1; /** * The anchor attribute'sTOP_RIGHT
value. */ public static final int TOP_RIGHT = EnumType.ANCHOR | 2; /** * The anchor attribute'sMIDDLE_LEFT
value. */ public static final int MIDDLE_LEFT = EnumType.ANCHOR | 3; /** * The anchor attribute'sMIDDLE_CENTER
value. */ public static final int MIDDLE_CENTER = EnumType.ANCHOR | 4; /** * The anchor attribute'sMIDDLE_RIGHT
value. */ public static final int MIDDLE_RIGHT = EnumType.ANCHOR | 5; /** * The anchor attribute'sBOTTOM_LEFT
value. */ public static final int BOTTOM_LEFT = EnumType.ANCHOR | 6; /** * The anchor attribute'sBOTTOM_CENTER
value. */ public static final int BOTTOM_CENTER = EnumType.ANCHOR | 7; /** * The anchor attribute'sBOTTOM_RIGHT
value. */ public static final int BOTTOM_RIGHT = EnumType.ANCHOR | 8; /** * The attributesattribute's ATTRIBUTE_IGNORE
value. */ public static final int ATTRIBUTE_IGNORE = EnumType.ATTRIBUTES | 0; /** * The attributesattribute's ATTRIBUTE_DELEGATE
value. */ public static final int ATTRIBUTE_DELEGATE = EnumType.ATTRIBUTES | 1; /** * The attributesattribute's ATTRIBUTE_PRESERVE
value. */ public static final int ATTRIBUTE_PRESERVE = EnumType.ATTRIBUTES | 2; /** * The boolean attribute'sBOOL_FALSE
value. */ public static final int BOOL_FALSE = EnumType.BOOLEAN | 0; /** * The boolean attribute'sBOOL_TRUE
value. */ public static final int BOOL_TRUE = EnumType.BOOLEAN | 1; /** * The cap attribute'sBUTT
value. */ public static final int BUTT = EnumType.CAP | 0; /** * The cap attribute'sROUND
value. */ public static final int ROUND = EnumType.CAP | 1; /** * The cap attribute'sSQUARE
value. */ public static final int SQUARE = EnumType.CAP | 2; /** * The controlcode attribute'sCONTROL_ESC
value. */ public static final int CONTROL_ESC = EnumType.CONTROLCODE | 0; /** * The controlcode attribute'sCONTROL_LF
value. */ public static final int CONTROL_LF = EnumType.CONTROLCODE | 1; /** * The controlcode attribute'sCONTROL_CR
value. */ public static final int CONTROL_CR = EnumType.CONTROLCODE | 2; /** * The controlcode attribute'sCONTROL_FF
value. */ public static final int CONTROL_FF = EnumType.CONTROLCODE | 3; /** * The dest attribute'sMEMORY
value. */ public static final int MEMORY = EnumType.DEST | 0; /** * The dest attribute'sFILE
value. */ public static final int FILE = EnumType.DEST | 1; /** * The destop attribute'sAPPEND
value. */ public static final int APPEND = EnumType.DESTOP | 0; /** * The destop attribute'sSET
value. */ public static final int SET = EnumType.DESTOP | 1; /** * The encoding attribute'sISO_8859_1
value. */ public static final int ISO_8859_1 = EnumType.ENCODING | 0; /** * The encoding attribute'sISO_8859_2
value. */ public static final int ISO_8859_2 = EnumType.ENCODING | 1; /** * The encoding attribute'sISO_8859_5
value. */ public static final int ISO_8859_5 = EnumType.ENCODING | 2; /** * The encoding attribute'sISO_8859_6
value. */ public static final int ISO_8859_6 = EnumType.ENCODING | 3; /** * The encoding attribute'sISO_8859_7
value. */ public static final int ISO_8859_7 = EnumType.ENCODING | 4; /** * The encoding attribute'sISO_8859_8
value. */ public static final int ISO_8859_8 = EnumType.ENCODING | 5; /** * The encoding attribute'sISO_8859_9
value. */ public static final int ISO_8859_9 = EnumType.ENCODING | 6; /** * The encoding attribute'sSHIFT_JIS
value. */ public static final int SHIFT_JIS = EnumType.ENCODING | 7; /** * The encoding attribute'sKSC_5601
value. */ public static final int KSC_5601 = EnumType.ENCODING | 8; /** * The encoding attribute'sBIG_5
value. */ public static final int BIG_5 = EnumType.ENCODING | 9; /** * The encoding attribute'sHKSCS_BIG
value. */ public static final int HKSCS_BIG5 = EnumType.ENCODING | 10; /** * The encoding attribute'sGBK
value. */ public static final int GBK = EnumType.ENCODING | 11; /** * The encoding attribute'sUTF_8
value. */ public static final int UTF_8 = EnumType.ENCODING | 12; /** * The encoding attribute'sUTF_16
value. */ public static final int UTF_16 = EnumType.ENCODING | 13; /** * The encoding attribute'sUCS_2
value. */ public static final int UCS_2 = EnumType.ENCODING | 14; /** * The encoding attribute'sENCODING_NONE
value. */ public static final int ENCODING_NONE = EnumType.ENCODING | 15; /** * The encoding attribute'sFONT_SPECIFIC
value. */ public static final int FONT_SPECIFIC = EnumType.ENCODING | 16; /** * The encoding attribute'sIBM_PC
value. */ public static final int IBM_PC850 = EnumType.ENCODING | 17; /** * The encoding attribute'sGB
value. */ public static final int GB18030 = EnumType.ENCODING | 18; /** * The encoding attribute'sJIS
value. */ public static final int JIS2004 = EnumType.ENCODING | 19; /** * The encryptionlevel attribute'sENCRYPTLEVEL_40
value. */ public static final int ENCRYPTLEVEL_40 = EnumType.ENCRYPTIONLEVEL | 0; /** * The encryptionlevel attribute'sENCRYPTLEVEL_128
value. */ public static final int ENCRYPTLEVEL_128 = EnumType.ENCRYPTIONLEVEL | 1; /** * The exception attribute'sEXCEPTION_FATAL
value. */ public static final int EXCEPTION_FATAL = EnumType.EXCEPTION | 0; /** * The exception attribute'sEXCEPTION_IGNORE
value. */ public static final int EXCEPTION_IGNORE = EnumType.EXCEPTION | 1; /** * The exception attribute'sEXCEPTION_WARNING
value. */ public static final int EXCEPTION_WARNING = EnumType.EXCEPTION | 2; /** * The fontlinethrough attribute'sLINETHROUGH_ZERO
value. */ public static final int LINETHROUGH_ZERO = EnumType.FONTLINETHROUGH | 0; /** * The fontlinethrough attribute'sLINETHROUGH_SINGLE
value. */ public static final int LINETHROUGH_SINGLE = EnumType.FONTLINETHROUGH | 1; /** * The fontlinethrough attribute'sLINETHROUGH_DOUBLE
value. */ public static final int LINETHROUGH_DOUBLE = EnumType.FONTLINETHROUGH | 2; /** * The fontlinethroughperiod attribute'sLINETHROUGH_ALL
value. */ public static final int LINETHROUGH_ALL = EnumType.FONTLINETHROUGHPERIOD | 0; /** * The fontlinethroughperiod attribute'sLINETHROUGH_WORD
value. */ public static final int LINETHROUGH_WORD = EnumType.FONTLINETHROUGHPERIOD | 1; /** * The fontoverline attribute'sOVERLINE_ZERO
value. */ public static final int OVERLINE_ZERO = EnumType.FONTOVERLINE | 0; /** * The fontoverline attribute'sOVERLINE_SINGLE
value. */ public static final int OVERLINE_SINGLE = EnumType.FONTOVERLINE | 1; /** * The fontoverline attribute'sOVERLINE_DOUBLE
value. */ public static final int OVERLINE_DOUBLE = EnumType.FONTOVERLINE | 2; /** * The fontoverlineperiod attribute'sOVER_ALL
value. */ public static final int OVER_ALL = EnumType.FONTOVERLINEPERIOD | 0; /** * The fontoverlineperiod attribute'sOVER_WORD
value. */ public static final int OVER_WORD = EnumType.FONTOVERLINEPERIOD | 1; /** * The fontpitch attribute'sFIXED
value. */ public static final int FIXED = EnumType.FONTPITCH | 0; /** * The fontpitch attribute'sVARIABLE
value. */ public static final int VARIABLE = EnumType.FONTPITCH | 1; /** * The fontposture attribute'sPOSTURE_ITALIC
value. */ public static final int POSTURE_ITALIC = EnumType.FONTPOSTURE | 0; /** * The fontposture attribute'sPOSTURE_NORMAL
value. */ public static final int POSTURE_NORMAL = EnumType.FONTPOSTURE | 1; /** * The fontunderline attribute'sUNDER_ZERO
value. */ public static final int UNDER_ZERO = EnumType.FONTUNDERLINE | 0; /** * The fontunderline attribute'sUNDER_SINGLE
value. */ public static final int UNDER_SINGLE = EnumType.FONTUNDERLINE | 1; /** * The fontunderline attribute'sUNDER_DOUBLE
value. */ public static final int UNDER_DOUBLE = EnumType.FONTUNDERLINE | 2; /** * The fontunderlineperiod attribute'sUNDER_ALL
value. */ public static final int UNDER_ALL = EnumType.FONTUNDERLINEPERIOD | 0; /** * The fontunderlineperiod attribute'sUNDER_WORD
value. */ public static final int UNDER_WORD = EnumType.FONTUNDERLINEPERIOD | 1; /** * The fontweight attribute'sWEIGHT_NORMAL
value. */ public static final int WEIGHT_NORMAL = EnumType.FONTWEIGHT | 0; /** * The fontweight attribute'sWEIGHT_BOLD
value. */ public static final int WEIGHT_BOLD = EnumType.FONTWEIGHT | 1; /** * The fraction attribute'sFRACTION_ROUND
value. */ public static final int FRACTION_ROUND = EnumType.FRACTION | 0; /** * The fraction attribute'sFRACTION_TRUNC
value. */ public static final int FRACTION_TRUNC = EnumType.FRACTION | 1; /** * The halign attribute'sHALIGN_LEFT
value. */ public static final int HALIGN_LEFT = EnumType.HALIGN | 0; /** * The halign attribute'sHALIGN_CENTER
value. */ public static final int HALIGN_CENTER = EnumType.HALIGN | 1; /** * The halign attribute'sHALIGN_RIGHT
value. */ public static final int HALIGN_RIGHT = EnumType.HALIGN | 2; /** * The halign attribute'sHALIGN_JUSTIFY
value. */ public static final int HALIGN_JUSTIFY = EnumType.HALIGN | 3; /** * The halign attribute'sHALIGN_JUSTIFY_ALL
value. */ public static final int HALIGN_JUSTIFY_ALL = EnumType.HALIGN | 4; /** * The halign attribute'sHALIGN_RADIX
value. */ public static final int HALIGN_RADIX = EnumType.HALIGN | 5; /** * The hand attribute'sHAND_LEFT
value. */ public static final int HAND_LEFT = EnumType.HAND | 0; /** * The hand attribute'sHAND_RIGHT
value. */ public static final int HAND_RIGHT = EnumType.HAND | 1; /** * The hand attribute'sHAND_EVEN
value. */ public static final int HAND_EVEN = EnumType.HAND | 2; /** * The ifempty attribute'sIFEMPTY_IGNORE
value. */ public static final int IFEMPTY_IGNORE = EnumType.IFEMPTY | 0; /** * The ifempty attribute'sIFEMPTY_REMOVE
value. */ public static final int IFEMPTY_REMOVE = EnumType.IFEMPTY | 1; /** * The ifempty attribute'sIFEMPTY_DATAGROUP
value. */ public static final int IFEMPTY_DATAGROUP = EnumType.IFEMPTY | 2; /** * The ifempty attribute'sIFEMPTY_DATAVALUE
value. */ public static final int IFEMPTY_DATAVALUE = EnumType.IFEMPTY | 3; /** * The imagingbbox attribute'sIMAGINGBBOX_NONE
value. */ public static final int IMAGINGBBOX_NONE = EnumType.IMAGINGBBOX | 0; /** * The join attribute'sJOIN_ROUND
value. */ public static final int JOIN_ROUND = EnumType.JOIN | 0; /** * The join attribute'sJOIN_SQUARE
value. */ public static final int JOIN_SQUARE = EnumType.JOIN | 1; /** * The layout attribute'sLEFT_RIGHT_TOP_BOTTOM
value. */ public static final int LEFT_RIGHT_TOP_BOTTOM = EnumType.LAYOUT | 0; /** * The layout attribute'sRIGHT_LEFT_TOP_BOTTOM
value. */ public static final int RIGHT_LEFT_TOP_BOTTOM = EnumType.LAYOUT | 1; /** * The layout attribute'sTOP_BOTTOM
value. */ public static final int TOP_BOTTOM = EnumType.LAYOUT | 2; /** * The layout attribute'sPOSITION
value. */ public static final int POSITION = EnumType.LAYOUT | 3; /** * The layout attribute'sTABLE
value. */ public static final int TABLE = EnumType.LAYOUT | 4; /** * The layout attribute'sROW
value. */ public static final int ROW = EnumType.LAYOUT | 5; /** * The layout attribute'sRIGHT_LEFT_ROW
value. */ public static final int RIGHT_LEFT_ROW = EnumType.LAYOUT | 6; /** * The linear attribute'sTO_RIGHT
value. */ public static final int TO_RIGHT = EnumType.LINEAR | 0; /** * The linear attribute'sTO_BOTTOM
value. */ public static final int TO_BOTTOM = EnumType.LINEAR | 1; /** * The linear attribute'sTO_LEFT
value. */ public static final int TO_LEFT = EnumType.LINEAR | 2; /** * The linear attribute'sTO_TOP
value. */ public static final int TO_TOP = EnumType.LINEAR | 3; /** * The lineend attribute'sLINEEND_ITC
value. */ public static final int LINEEND_ITC = EnumType.LINEEND | 0; /** * The lineend attribute'sLINEEND_ITCCR
value. */ public static final int LINEEND_ITCCR = EnumType.LINEEND | 1; /** * The lineend attribute'sLINEEND_CRCR
value. */ public static final int LINEEND_CRCR = EnumType.LINEEND | 2; /** * The lineend attribute'sLINEEND_CR
value. */ public static final int LINEEND_CR = EnumType.LINEEND | 3; /** * The logto attribute'sLOG_URI
value. */ public static final int LOG_URI = EnumType.LOGTO | 0; /** * The logto attribute'sLOG_MEMORY
value. */ public static final int LOG_MEMORY = EnumType.LOGTO | 1; /** * The logto attribute'sLOG_STDOUT
value. */ public static final int LOG_STDOUT = EnumType.LOGTO | 2; /** * The logto attribute'sLOG_NULL
value. */ public static final int LOG_NULL = EnumType.LOGTO | 3; /** * The logto attribute'sLOG_STDERROR
value. */ public static final int LOG_STDERROR = EnumType.LOGTO | 4; /** * The logto attribute'sLOG_SYSTEM
value. */ public static final int LOG_SYSTEM = EnumType.LOGTO | 5; /** * The match attribute'sMATCH_NONE
value. */ public static final int MATCH_NONE = EnumType.MATCH | 0; /** * The match attribute'sMATCH_ONCE
value. */ public static final int MATCH_ONCE = EnumType.MATCH | 1; /** * The match attribute'sMATCH_GLOBAL
value. */ public static final int MATCH_GLOBAL = EnumType.MATCH | 2; /** * The match attribute'sMATCH_DATAREF
value. */ public static final int MATCH_DATAREF = EnumType.MATCH | 3; /** * The match attribute'sMATCH_DESCENDANT
value. */ public static final int MATCH_DESCENDANT = EnumType.MATCH | 4; // match once but must be a descendant; only used during runtime // /** // * The match attribute'sMATCH_MANY
value. // */ // public static final int MATCH_MANY = EnumType.MATCH | 5; // scoped but match many. /** * The mergeMode attribute'sMERGEMODE_CONSUMEDATA
value. */ public static final int MERGEMODE_CONSUMEDATA = EnumType.MERGEMODE | 0; /** * The mergeMode attribute'sMERGEMODE_MATCHTEMPLATE
value. */ public static final int MERGEMODE_MATCHTEMPLATE = EnumType.MERGEMODE | 1; /** * The messagetype attribute'sMESSAGE_TRACE
value. */ public static final int MESSAGE_TRACE = EnumType.MESSAGETYPE | 0; /** * The messagetype attribute'sMESSAGE_INFORMATION
value. */ public static final int MESSAGE_INFORMATION = EnumType.MESSAGETYPE | 1; /** * The messagetype attribute'sMESSAGE_WARNING
value. */ public static final int MESSAGE_WARNING = EnumType.MESSAGETYPE | 2; /** * The messagetype attribute'sMESSAGE_ERROR
value. */ public static final int MESSAGE_ERROR = EnumType.MESSAGETYPE | 3; /** * The mode attribute'sMODE_APPEND
value. */ public static final int MODE_APPEND = EnumType.MODE | 0; /** * The mode attribute'sMODE_OVERWRITE
value. */ public static final int MODE_OVERWRITE = EnumType.MODE | 1; /** * The nodepresence attribute'sNODEPRESENCE_IGNORE
value. */ public static final int NODEPRESENCE_IGNORE = EnumType.NODEPRESENCE | 0; /** * The nodepresence attribute'sNODEPRESENCE_DISSOLVE
value. */ public static final int NODEPRESENCE_DISSOLVE = EnumType.NODEPRESENCE | 1; /** * The nodepresence attribute'sNODEPRESENCE_PRESERVE
value. */ public static final int NODEPRESENCE_PRESERVE = EnumType.NODEPRESENCE | 2; /** * The nodepresence attribute'sNODEPRESENCE_DISSOLVESTRUCTURE
value. */ public static final int NODEPRESENCE_DISSOLVESTRUCTURE = EnumType.NODEPRESENCE | 3; /** * The open attribute'sUSER_CONTROL
value. */ public static final int USER_CONTROL = EnumType.OPEN | 0; /** * The open attribute'sON_ENTRY
value. */ public static final int ON_ENTRY = EnumType.OPEN | 1; /** * The open attribute'sALWAYS
value. */ public static final int ALWAYS = EnumType.OPEN | 2; /** * The open attribute'sMULTISELECT
value. */ public static final int MULTISELECT = EnumType.OPEN | 3; /** * The operation attribute'sOPERATION_UP
value. */ public static final int OPERATION_UP = EnumType.OPERATION | 0; /** * The operation attribute'sOPERATION_DOWN
value. */ public static final int OPERATION_DOWN = EnumType.OPERATION | 1; /** * The operation attribute'sOPERATION_LEFT
value. */ public static final int OPERATION_LEFT = EnumType.OPERATION | 2; /** * The operation attribute'sOPERATION_RIGHT
value. */ public static final int OPERATION_RIGHT = EnumType.OPERATION | 3; /** * The operation attribute'sOPERATION_BACK
value. */ public static final int OPERATION_BACK = EnumType.OPERATION | 4; /** * The operation attribute'sOPERATION_FIRST
value. */ public static final int OPERATION_FIRST = EnumType.OPERATION | 5; /** * The operation attribute'sOPERATION_NEXT
value. */ public static final int OPERATION_NEXT = EnumType.OPERATION | 6; /** * The order attribute'sORDER_DOCUMENT
value. */ public static final int ORDER_DOCUMENT = EnumType.ORDER | 0; /** * The order attribute'sORDER_DATAVALUESFIRST
value. */ public static final int ORDER_DATAVALUESFIRST = EnumType.ORDER | 1; /** * The orientation attribute'sPORTRAIT
value. */ public static final int PORTRAIT = EnumType.ORIENTATION | 0; /** * The orientation attribute'sLANDSCAPE
value. */ public static final int LANDSCAPE = EnumType.ORIENTATION | 1; /** * The outputto attribute'sOUTPUT_URI
value. */ public static final int OUTPUT_URI = EnumType.OUTPUTTO | 0; /** * The outputto attribute'sOUTPUT_MEMORY
value. */ public static final int OUTPUT_MEMORY = EnumType.OUTPUTTO | 1; /** * The outputto attribute'sOUTPUT_STDOUT
value. */ public static final int OUTPUT_STDOUT = EnumType.OUTPUTTO | 2; /** * The outputto attribute'sOUTPUT_NULL
value. */ public static final int OUTPUT_NULL = EnumType.OUTPUTTO | 3; /** * The outputtype attribute'sOUTPUT_NATIVE
value. */ public static final int OUTPUT_NATIVE = EnumType.OUTPUTTYPE | 0; /** * The outputtype attribute'sOUTPUT_XDP
value. */ public static final int OUTPUT_XDP = EnumType.OUTPUTTYPE | 1; /** * The outputtype attribute'sOUTPUT_MERGEDXDP
value. */ public static final int OUTPUT_MERGEDXDP = EnumType.OUTPUTTYPE | 2; /** * The override attribute'sOVERRIDE_DISABLED
value. */ public static final int OVERRIDE_DISABLED = EnumType.OVERRIDE | 0; /** * The override attribute'sOVERRIDE_WARNING
value. */ public static final int OVERRIDE_WARNING = EnumType.OVERRIDE | 1; /** * The override attribute'sOVERRIDE_ERROR
value. */ public static final int OVERRIDE_ERROR = EnumType.OVERRIDE | 2; /** * The override attribute'sOVERRIDE_IGNORE
value. */ public static final int OVERRIDE_IGNORE = EnumType.OVERRIDE | 3; /** * The pattern attribute'sHORIZONTAL_HATCHING
value. */ public static final int HORIZONTAL_HATCHING = EnumType.PATTERN | 0; /** * The pattern attribute'sVERTICAL_HATCHING
value. */ public static final int VERTICAL_HATCHING = EnumType.PATTERN | 1; /** * The pattern attribute'sCROSS_HATCHING
value. */ public static final int CROSS_HATCHING = EnumType.PATTERN | 2; /** * The pattern attribute'sDIAGONAL_LEFT_HATCHING
value. */ public static final int DIAGONAL_LEFT_HATCHING = EnumType.PATTERN | 3; /** * The pattern attribute'sDIAGONAL_RIGHT_HATCHING
value. */ public static final int DIAGONAL_RIGHT_HATCHING = EnumType.PATTERN | 4; /** * The pattern attribute'sCROSS_DIAGONAL_HATCHING
value. */ public static final int CROSS_DIAGONAL_HATCHING = EnumType.PATTERN | 5; /** * The presence attribute'sPRESENCE_VISIBLE
value. */ public static final int PRESENCE_VISIBLE = EnumType.PRESENCE | 0; /** * The presence attribute'sPRESENCE_INVISIBLE
value. */ public static final int PRESENCE_INVISIBLE = EnumType.PRESENCE | 1; /** * The presence attribute'sPRESENCE_HIDDEN
value. */ public static final int PRESENCE_HIDDEN = EnumType.PRESENCE | 2; /** * The presence attribute'sPRESENCE_INACTIVE
value. */ public static final int PRESENCE_INACTIVE = EnumType.PRESENCE | 3; /** * The radial attribute'sTO_CENTER
value. */ public static final int TO_CENTER = EnumType.RADIAL | 0; /** * The radial attribute'sTO_EDGE
value. */ public static final int TO_EDGE = EnumType.RADIAL | 1; /** * The relation attribute'sRELATION_ORDERED
value. */ public static final int RELATION_ORDERED = EnumType.RELATION | 0; /** * The relation attribute'sRELATION_UNORDERED
value. */ public static final int RELATION_UNORDERED = EnumType.RELATION | 1; /** * The relation attribute'sRELATION_CHOICE
value. */ public static final int RELATION_CHOICE = EnumType.RELATION | 2; /** * The scriptmodel attribute'sSCRIPTMODEL_XFA
value. */ public static final int SCRIPTMODEL_XFA = EnumType.SCRIPTMODEL | 0; /** * The scriptmodel attribute'sSCRIPTMODEL_NONE
value. */ public static final int SCRIPTMODEL_NONE = EnumType.SCRIPTMODEL | 1; /** * The select attribute'sIMPLICITLINK
value. */ public static final int IMPLICITLINK = EnumType.SELECT | 0; /** * The select attribute'sEMBED
value. */ public static final int EMBED = EnumType.SELECT | 1; /** * The select attribute'sEMBEDSUBSET
value. */ public static final int EMBEDSUBSET = EnumType.SELECT | 2; /** * The severity attribute'sSEV_IGNORE
value. */ public static final int SEV_IGNORE = EnumType.SEVERITY | 0; /** * The severity attribute'sSEV_TRACE
value. */ public static final int SEV_TRACE = EnumType.SEVERITY | 1; /** * The severity attribute'sSEV_INFORMATION
value. */ public static final int SEV_INFORMATION = EnumType.SEVERITY | 2; /** * The severity attribute'sSEV_WARNING
value. */ public static final int SEV_WARNING = EnumType.SEVERITY | 3; /** * The severity attribute'sSEV_ERROR
value. */ public static final int SEV_ERROR = EnumType.SEVERITY | 4; /** * The shape attribute'sSHAPE_SQUARE
value. */ public static final int SHAPE_SQUARE = EnumType.SHAPE | 0; /** * The shape attribute'sSHAPE_ROUND
value. */ public static final int SHAPE_ROUND = EnumType.SHAPE | 1; /** * The slope attribute'sSLOPE_POSITIVE
value. */ public static final int SLOPE_POSITIVE = EnumType.SLOPE | 0; /** * The slope attribute'sSLOPE_NEGATIVE
value. */ public static final int SLOPE_NEGATIVE = EnumType.SLOPE | 1; /** * The splitbiasattribute's SPLITBIAS_HORIZONTAL
value. */ public static final int SPLITBIAS_HORIZONTAL = EnumType.SPLITBIAS | 0; /** * The splitbiasattribute's SPLITBIAS_VERTICAL
value. */ public static final int SPLITBIAS_VERTICAL = EnumType.SPLITBIAS | 1; /** * The splitbiasattribute's SPLITBIAS_NONE
value. */ public static final int SPLITBIAS_NONE = EnumType.SPLITBIAS | 2; /** * The stroke attribute'sSTROKE_SOLID
value. */ public static final int STROKE_SOLID = EnumType.STROKE | 0; /** * The stroke attribute'sSTROKE_DASHED
value. */ public static final int STROKE_DASHED = EnumType.STROKE | 1; /** * The stroke attribute'sSTROKE_DOTTED
value. */ public static final int STROKE_DOTTED = EnumType.STROKE | 2; /** * The stroke attribute'sSTROKE_LOWERED
value. */ public static final int STROKE_LOWERED = EnumType.STROKE | 3; /** * The stroke attribute'sSTROKE_RAISED
value. */ public static final int STROKE_RAISED = EnumType.STROKE | 4; /** * The stroke attribute'sSTROKE_ETCHED
value. */ public static final int STROKE_ETCHED = EnumType.STROKE | 5; /** * The stroke attribute'sSTROKE_EMBOSSED
value. */ public static final int STROKE_EMBOSSED = EnumType.STROKE | 6; /** * The stroke attribute'sSTROKE_DASHDOT
value. */ public static final int STROKE_DASHDOT = EnumType.STROKE | 7; /** * The stroke attribute'sSTROKE_DASHDOTDOT
value. */ public static final int STROKE_DASHDOTDOT = EnumType.STROKE | 8; /** * The styletype attribute'sBITPATTERN
value. */ public static final int BITPATTERN = EnumType.STYLETYPE | 0; /** * The subformbreak attribute'sBREAK_AUTO
value. */ public static final int BREAK_AUTO = EnumType.SUBFORMBREAK | 0; /** * The subformbreak attribute'sBREAK_CONTENTAREA
value. */ public static final int BREAK_CONTENTAREA = EnumType.SUBFORMBREAK | 1; /** * The subformbreak attribute'sBREAK_PAGE
value. */ public static final int BREAK_PAGE = EnumType.SUBFORMBREAK | 2; /** * The subformbreak attribute'sBREAK_PAGEEVEN
value. */ public static final int BREAK_PAGEEVEN = EnumType.SUBFORMBREAK | 3; /** * The subformbreak attribute'sBREAK_PAGEODD
value. */ public static final int BREAK_PAGEODD = EnumType.SUBFORMBREAK | 4; /** * The subformkeep attribute'sKEEP_NONE
value. */ public static final int KEEP_NONE = EnumType.SUBFORMKEEP | 0; /** * The subformkeep attribute'sKEEP_CONTENTAREA
value. */ public static final int KEEP_CONTENTAREA = EnumType.SUBFORMKEEP | 1; /** * The subformkeep attribute'sKEEP_PAGE
value. */ public static final int KEEP_PAGE = EnumType.SUBFORMKEEP | 2; /** * The submitformat attribute'sSUBMITFORMAT_HTML
value. */ public static final int SUBMITFORMAT_HTML = EnumType.SUBMITFORMAT | 0; /** * The submitformat attribute'sSUBMITFORMAT_FDF
value. */ public static final int SUBMITFORMAT_FDF = EnumType.SUBMITFORMAT | 1; /** * The submitformat attribute'sSUBMITFORMAT_XML
value. */ public static final int SUBMITFORMAT_XML = EnumType.SUBMITFORMAT | 2; /** * The submitformat attribute'sSUBMITFORMAT_PDF
value. */ public static final int SUBMITFORMAT_PDF = EnumType.SUBMITFORMAT | 3; /** * The test attribute'sTEST_DISABLED
value. */ public static final int TEST_DISABLED = EnumType.TEST | 0; /** * The test attribute'sTEST_WARNING
value. */ public static final int TEST_WARNING = EnumType.TEST | 1; /** * The test attribute'sTEST_ERROR
value. */ public static final int TEST_ERROR = EnumType.TEST | 2; /** * The transferencoding attribute'sTRANSFER_NONE
value. */ public static final int TRANSFER_NONE = EnumType.TRANSFERENCODING | 0; /** * The transferencoding attribute'sTRANSFER_BASE
value. */ public static final int TRANSFER_BASE64 = EnumType.TRANSFERENCODING | 1; /** * The transferencoding attribute'sTRANSFER_PACKAGE
value. */ public static final int TRANSFER_PACKAGE = EnumType.TRANSFERENCODING | 2; /** * The trayin attribute'sTRAYIN_AUTO
value. */ public static final int TRAYIN_AUTO = EnumType.TRAYIN | 0; /** * The trayin attribute'sTRAYIN_MANUAL
value. */ public static final int TRAYIN_MANUAL = EnumType.TRAYIN | 1; /** * The trayin attribute'sTRAYIN_DELEGATE
value. */ public static final int TRAYIN_DELEGATE = EnumType.TRAYIN | 2; /** * The trayout attribute'sTRAYOUT_AUTO
value. */ public static final int TRAYOUT_AUTO = EnumType.TRAYOUT | 0; /** * The trayout attribute'sTRAYOUT_DELEGATE
value. */ public static final int TRAYOUT_DELEGATE = EnumType.TRAYOUT | 1; /** * The unit attribute'sPOINT
value. */ public static final int POINT = EnumType.UNIT | 0; /** * The unit attribute'sINCH
value. */ public static final int INCH = EnumType.UNIT | 1; /** * The unit attribute'sCENTIMETER
value. */ public static final int CENTIMETER = EnumType.UNIT | 2; /** * The unit attribute'sMILLIMETER
value. */ public static final int MILLIMETER = EnumType.UNIT | 3; /** * The unit attribute'sMILLIPOINT
value. */ public static final int MILLIPOINT = EnumType.UNIT | 4; /** * The unit attribute'sPICA
value. */ public static final int PICA = EnumType.UNIT | 5; /** * The unsign attribute'sANY
value. */ public static final int ANY = EnumType.UNSIGN | 0; /** * The unsign attribute'sSIGNER
value. */ public static final int SIGNER = EnumType.UNSIGN | 1; /** * The valign attribute'sVALIGN_TOP
value. */ public static final int VALIGN_TOP = EnumType.VALIGN | 0; /** * The valign attribute'sVALIGN_MIDDLE
value. */ public static final int VALIGN_MIDDLE = EnumType.VALIGN | 1; /** * The valign attribute'sVALIGN_BOTTOM
value. */ public static final int VALIGN_BOTTOM = EnumType.VALIGN | 2; /** * The whitespace attribute'sWHITESPACE_TRIM
value. */ public static final int WHITESPACE_TRIM = EnumType.WHITESPACE | 0; /** * The whitespace attribute'sWHITESPACE_RTRIM
value. */ public static final int WHITESPACE_RTRIM = EnumType.WHITESPACE | 1; /** * The whitespace attribute'sWHITESPACE_LTRIM
value. */ public static final int WHITESPACE_LTRIM = EnumType.WHITESPACE | 2; /** * The whitespace attribute'sWHITESPACE_NORMALIZE
value. */ public static final int WHITESPACE_NORMALIZE = EnumType.WHITESPACE | 3; /** * The whitespace attribute'sWHITESPACE_PRESERVE
value. */ public static final int WHITESPACE_PRESERVE = EnumType.WHITESPACE | 4; /** * The placement attribute'sPLACEMENT_TOP
value. */ public static final int PLACEMENT_TOP = EnumType.PLACEMENT | 0; /** * The placement attribute'sPLACEMENT_RIGHT
value. */ public static final int PLACEMENT_RIGHT = EnumType.PLACEMENT | 1; /** * The placement attribute'sPLACEMENT_BOTTOM
value. */ public static final int PLACEMENT_BOTTOM = EnumType.PLACEMENT | 2; /** * The placement attribute'sPLACEMENT_LEFT
value. */ public static final int PLACEMENT_LEFT = EnumType.PLACEMENT | 3; /** * The placement attribute'sPLACEMENT_INLINE
value. */ public static final int PLACEMENT_INLINE = EnumType.PLACEMENT | 4; /** * The destination attribute'sDESTINATION_NONE
value. */ public static final int DESTINATION_NONE = EnumType.DESTINATION | 0; /** * The destination attribute'sDESTINATION_PAGEFIT
value. */ public static final int DESTINATION_PAGEFIT = EnumType.DESTINATION | 1; /** * The db attribute'sDB_ADO
value. */ public static final int DB_ADO = EnumType.DB | 0; /** * The db attribute'sDB_HTTP
value. */ public static final int DB_HTTP = EnumType.DB | 1; /** * The cmdtype attribute'sCMDTYPE_UNKNOWN
value. */ public static final int CMDTYPE_UNKNOWN = EnumType.CMDTYPE | 0; /** * The cmdtype attribute'sCMDTYPE_TEXT
value. */ public static final int CMDTYPE_TEXT = EnumType.CMDTYPE | 1; /** * The cmdtype attribute'sCMDTYPE_TABLE
value. */ public static final int CMDTYPE_TABLE = EnumType.CMDTYPE | 2; /** * The cmdtype attribute'sCMDTYPE_STOREDPROC
value. */ public static final int CMDTYPE_STOREDPROC = EnumType.CMDTYPE | 3; /** * The cursortype attribute'sCURSORTYPE_UNSPECIFIED
value. */ public static final int CURSORTYPE_UNSPECIFIED = EnumType.CURSORTYPE | 0; /** * The cursortype attribute'sCURSORTYPE_FORWARDONLY
value. */ public static final int CURSORTYPE_FORWARDONLY = EnumType.CURSORTYPE | 1; /** * The cursortype attribute'sCURSORTYPE_KEYSET
value. */ public static final int CURSORTYPE_KEYSET = EnumType.CURSORTYPE | 2; /** * The cursortype attribute'sCURSORTYPE_DYNAMIC
value. */ public static final int CURSORTYPE_DYNAMIC = EnumType.CURSORTYPE | 3; /** * The cursortype attribute'sCURSORTYPE_STATIC
value. */ public static final int CURSORTYPE_STATIC = EnumType.CURSORTYPE | 4; /** * The cursorlocation attribute'sCURSORLOCATION_CLIENT
value. */ public static final int CURSORLOCATION_CLIENT = EnumType.CURSORLOCATION | 0; /** * The cursorlocation attribute'sCURSORLOCATION_SERVER
value. */ public static final int CURSORLOCATION_SERVER = EnumType.CURSORLOCATION | 1; /** * The LOCKTYPE's LOCKTYPE_UNSPECIFIEDINCREMENTALLOAD_NONE
value. */ public static final int INCREMENTALLOAD_NONE = EnumType.INCREMENTALLOAD | 0; /** * The incrementalload attribute'sINCREMENTALLOAD_FORWARDONLY
value. */ public static final int INCREMENTALLOAD_FORWARDONLY = EnumType.INCREMENTALLOAD | 1; /** * The bofaction attribute'sBOFACTION_MOVEFIRST
value. */ public static final int BOFACTION_MOVEFIRST = EnumType.BOFACTION | 0; /** * The bofaction attribute'sBOFACTION_STAYBOF
value. */ public static final int BOFACTION_STAYBOF = EnumType.BOFACTION | 1; /** * The eofaction attribute'sEOFACTION_MOVELAST
value. */ public static final int EOFACTION_MOVELAST = EnumType.EOFACTION | 0; /** * The eofaction attribute'sEOFACTION_STAYEOF
value. */ public static final int EOFACTION_STAYEOF = EnumType.EOFACTION | 1; /** * The eofaction attribute'sEOFACTION_ADDNEW
value. */ public static final int EOFACTION_ADDNEW = EnumType.EOFACTION | 2; /** * The nodetype attribute'sNODETYPE_ATTRIBUTE
value. */ public static final int NODETYPE_ATTRIBUTE = EnumType.NODETYPE | 0; /** * The nodetype attribute'sNODETYPE_ELEMENT
value. */ public static final int NODETYPE_ELEMENT = EnumType.NODETYPE | 1; /** * The activity attribute'sACTIVITY_INITIALIZE
value. */ public static final int ACTIVITY_INITIALIZE = EnumType.ACTIVITY | 0; /** * The activity attribute'sACTIVITY_ENTER
value. */ public static final int ACTIVITY_ENTER = EnumType.ACTIVITY | 1; /** * The activity attribute'sACTIVITY_EXIT
value. */ public static final int ACTIVITY_EXIT = EnumType.ACTIVITY | 2; /** * The activity attribute'sACTIVITY_MOUSEENTER
value. */ public static final int ACTIVITY_MOUSEENTER = EnumType.ACTIVITY | 3; /** * The activity attribute'sACTIVITY_MOUSEEXIT
value. */ public static final int ACTIVITY_MOUSEEXIT = EnumType.ACTIVITY | 4; /** * The activity attribute'sACTIVITY_CHANGE
value. */ public static final int ACTIVITY_CHANGE = EnumType.ACTIVITY | 5; /** * The activity attribute'sACTIVITY_CLICK
value. */ public static final int ACTIVITY_CLICK = EnumType.ACTIVITY | 6; /** * The activity attribute'sACTIVITY_PRESAVE
value. */ public static final int ACTIVITY_PRESAVE = EnumType.ACTIVITY | 7; /** * The activity attribute'sACTIVITY_POSTSAVE
value. */ public static final int ACTIVITY_POSTSAVE = EnumType.ACTIVITY | 8; /** * The activity attribute'sACTIVITY_PREPRINT
value. */ public static final int ACTIVITY_PREPRINT = EnumType.ACTIVITY | 9; /** * The activity attribute'sACTIVITY_POSTPRINT
value. */ public static final int ACTIVITY_POSTPRINT = EnumType.ACTIVITY | 10; /** * The activity attribute'sACTIVITY_READY
value. */ public static final int ACTIVITY_READY = EnumType.ACTIVITY | 11; /** * The activity attribute'sACTIVITY_DOCREADY
value. */ public static final int ACTIVITY_DOCREADY = EnumType.ACTIVITY | 12; /** * The activity attribute'sACTIVITY_DOCCLOSE
value. */ public static final int ACTIVITY_DOCCLOSE = EnumType.ACTIVITY | 13; /** * The activity attribute'sACTIVITY_MOUSEUP
value. */ public static final int ACTIVITY_MOUSEUP = EnumType.ACTIVITY | 14; /** * The activity attribute'sACTIVITY_MOUSEDOWN
value. */ public static final int ACTIVITY_MOUSEDOWN = EnumType.ACTIVITY | 15; /** * The activity attribute'sACTIVITY_FULL
value. */ public static final int ACTIVITY_FULL = EnumType.ACTIVITY | 16; /** * The activity attribute'sACTIVITY_PRESUBMIT
value. */ public static final int ACTIVITY_PRESUBMIT = EnumType.ACTIVITY | 17; /** * The activity attribute'sACTIVITY_PREEXECUTE
value. */ public static final int ACTIVITY_PREEXECUTE = EnumType.ACTIVITY | 18; /** * The activity attribute'sACTIVITY_POSTEXECUTE
value. */ public static final int ACTIVITY_POSTEXECUTE = EnumType.ACTIVITY | 19; /** * The activity attribute'sACTIVITY_PREOPEN
value. */ public static final int ACTIVITY_PREOPEN = EnumType.ACTIVITY | 20; /** * The activity attribute'sACTIVITY_INDEXCHANGE
value. */ public static final int ACTIVITY_INDEXCHANGE = EnumType.ACTIVITY | 21; /** * The activity attribute'sACTIVITY_PRESIGN
value. */ public static final int ACTIVITY_PRESIGN = EnumType.ACTIVITY | 22; /** * The activity attribute'sACTIVITY_POSTSIGN
value. */ public static final int ACTIVITY_POSTSIGN = EnumType.ACTIVITY | 23; /** * The activity attribute'sACTIVITY_POSTSUBMIT
value. */ public static final int ACTIVITY_POSTSUBMIT = EnumType.ACTIVITY | 24; /** * The activity attribute'sACTIVITY_POSTOPEN
value. */ public static final int ACTIVITY_POSTOPEN = EnumType.ACTIVITY | 25; /** * The activity attribute'sACTIVITY_VALIDATIONSTATE
value. */ public static final int ACTIVITY_VALIDATIONSTATE = EnumType.ACTIVITY | 26; /** * The format attribute'sFORMAT_XFD
value. */ public static final int FORMAT_XFD = EnumType.FORMAT | 0; /** * The format attribute'sFORMAT_XDP
value. */ public static final int FORMAT_XDP = EnumType.FORMAT | 1; /** * The format attribute'sFORMAT_FORMDATA
value. */ public static final int FORMAT_FORMDATA = EnumType.FORMAT | 2; /** * The format attribute'sFORMAT_XML
value. */ public static final int FORMAT_XML = EnumType.FORMAT | 3; /** * The format attribute'sFORMAT_PDF
value. */ public static final int FORMAT_PDF = EnumType.FORMAT | 4; /** * The format attribute'sFORMAT_URL
value. */ public static final int FORMAT_URL = EnumType.FORMAT | 5; /** * The nulltype attribute'sNULLTYPE_XSI
value. */ public static final int NULLTYPE_XSI = EnumType.NULLTYPE | 0; /** * The nulltype attribute'sNULLTYPE_EXCLUDE
value. */ public static final int NULLTYPE_EXCLUDE = EnumType.NULLTYPE | 1; /** * The nulltype attribute'sNULLTYPE_EMPTY
value. */ public static final int NULLTYPE_EMPTY = EnumType.NULLTYPE | 2; /** * The aspect attribute'sASPECT_NONE
value. */ public static final int ASPECT_NONE = EnumType.ASPECT | 0; /** * The aspect attribute'sASPECT_FIT
value. */ public static final int ASPECT_FIT = EnumType.ASPECT | 1; /** * The aspect attribute'sASPECT_ACTUAL
value. */ public static final int ASPECT_ACTUAL = EnumType.ASPECT | 2; /** * The aspect attribute'sASPECT_WIDTH
value. */ public static final int ASPECT_WIDTH = EnumType.ASPECT | 3; /** * The aspect attribute'sASPECT_HEIGHT
value. */ public static final int ASPECT_HEIGHT = EnumType.ASPECT | 4; /** * The calendarsymbolsattribute's CALENDAR
value. */ public static final int CALENDAR = EnumType.CALENDARSYMBOLS | 0; /** * The datetimesymbolsattribute's PATTERN_FULL
value. */ public static final int PATTERN_FULL = EnumType.DATETIMESYMBOLS | 0; /** * The datetimesymbolsattribute's PATTERN_LONG
value. */ public static final int PATTERN_LONG = EnumType.DATETIMESYMBOLS | 1; /** * The datetimesymbolsattribute's PATTERN_MED
value. */ public static final int PATTERN_MED = EnumType.DATETIMESYMBOLS | 2; /** * The datetimesymbolsattribute's PATTERN_SHORT
value. */ public static final int PATTERN_SHORT = EnumType.DATETIMESYMBOLS | 3; /** * The numberpattern attribute'sPATTERN_NUMERIC
value. */ public static final int PATTERN_NUMERIC = EnumType.NUMBERPATTERN | 0; /** * The numberpattern attribute'sPATTERN_CURRENCY
value. */ public static final int PATTERN_CURRENCY = EnumType.NUMBERPATTERN | 1; /** * The numberpattern attribute'sPATTERN_PERCENT
value. */ public static final int PATTERN_PERCENT = EnumType.NUMBERPATTERN | 2; /** * The numbersymbol attribute'sNUMERIC_DECIMAL
value. */ public static final int NUMERIC_DECIMAL = EnumType.NUMBERSYMBOL | 0; /** * The numbersymbol attribute'sNUMERIC_GROUPING
value. */ public static final int NUMERIC_GROUPING = EnumType.NUMBERSYMBOL | 1; /** * The numbersymbol attribute'sNUMERIC_PERCENT
value. */ public static final int NUMERIC_PERCENT = EnumType.NUMBERSYMBOL | 2; /** * The numbersymbol attribute'sNUMERIC_MINUS
value. */ public static final int NUMERIC_MINUS = EnumType.NUMBERSYMBOL | 3; /** * The numbersymbol attribute'sNUMERIC_ZERO
value. */ public static final int NUMERIC_ZERO = EnumType.NUMBERSYMBOL | 4; /** * The currencysymbol attribute'sCURRENCY_SYMBOL
value. */ public static final int CURRENCY_SYMBOL = EnumType.CURRENCYSYMBOL | 0; /** * The currencysymbol attribute'sCURRENCY_ISONAME
value. */ public static final int CURRENCY_ISONAME = EnumType.CURRENCYSYMBOL | 1; /** * The currencysymbol attribute'sCURRENCY_DECIMAL
value. */ public static final int CURRENCY_DECIMAL = EnumType.CURRENCYSYMBOL | 2; /** * The parity attribute'sBARCODE_PARITY_NONE
value. */ public static final int BARCODE_PARITY_NONE = EnumType.PARITY | 0; /** * The parity attribute'sBARCODE_PARITY_ODD
value. */ public static final int BARCODE_PARITY_ODD = EnumType.PARITY | 1; /** * The parity attribute'sBARCODE_PARITY_EVEN
value. */ public static final int BARCODE_PARITY_EVEN = EnumType.PARITY | 2; /** * The textlocation attribute'sTEXTLOCATION_NONE
value. */ public static final int TEXTLOCATION_NONE = EnumType.TEXTLOCATION | 0; /** * The textlocation attribute'sTEXTLOCATION_ABOVE
value. */ public static final int TEXTLOCATION_ABOVE = EnumType.TEXTLOCATION | 1; /** * The textlocation attribute'sTEXTLOCATION_BELOW
value. */ public static final int TEXTLOCATION_BELOW = EnumType.TEXTLOCATION | 2; /** * The textlocation attribute'sTEXTLOCATION_ABOVEEMBEDDED
value. */ public static final int TEXTLOCATION_ABOVEEMBEDDED = EnumType.TEXTLOCATION | 3; /** * The textlocation attribute'sTEXTLOCATION_BELOWEMBEDDED
value. */ public static final int TEXTLOCATION_BELOWEMBEDDED = EnumType.TEXTLOCATION | 4; /** * The checksum attribute'sCHECKSUM_AUTO
value. */ public static final int CHECKSUM_AUTO = EnumType.CHECKSUM | 0; /** * The checksum attribute'sCHECKSUM_NONE
value. */ public static final int CHECKSUM_NONE = EnumType.CHECKSUM | 1; /** * The checksum attribute'sCHECKSUM_1MOD10
value. */ public static final int CHECKSUM_1MOD10 = EnumType.CHECKSUM | 2; /** * The checksum attribute'sCHECKSUM_2MOD10
value. */ public static final int CHECKSUM_2MOD10 = EnumType.CHECKSUM | 3; /** * The checksum attribute'sCHECKSUM_1MOD10_1MOD11
value. */ public static final int CHECKSUM_1MOD10_1MOD11 = EnumType.CHECKSUM | 4; /** * The signaturetype attribute'sPDF_13
value. */ public static final int PDF_13 = EnumType.SIGNATURETYPE | 0; /** * The signaturetype attribute'sPDF_16
value. */ public static final int PDF_16 = EnumType.SIGNATURETYPE | 1; /** * The borderbreak attribute'sBORDER_OPEN
value. */ public static final int BORDER_OPEN = EnumType.BORDERBREAK | 0; /** * The borderbreak attribute'sBORDER_CLOSED
value. */ public static final int BORDER_CLOSED = EnumType.BORDERBREAK | 1; /** * The support attribute'sSUPPORT_NONE
value. */ public static final int SUPPORT_NONE = EnumType.SUPPORT | 0; /** * The support attribute'sSUPPORT_HARDWARE
value. */ public static final int SUPPORT_HARDWARE = EnumType.SUPPORT | 1; /** * The support attribute'sSUPPORT_SOFTWARE
value. */ public static final int SUPPORT_SOFTWARE = EnumType.SUPPORT | 2; /** * The scope attribute'sSCOPE_NAME
value. */ public static final int SCOPE_NAME = EnumType.SCOPE | 0; /** * The scope attribute'sSCOPE_NONE
value. */ public static final int SCOPE_NONE = EnumType.SCOPE | 1; /** * The xdclayout attribute'sXDCLAYOUT_PAGINATE
value. */ public static final int XDCLAYOUT_PAGINATE = EnumType.XDCLAYOUT | 0; /** * The xdclayout attribute'sXDCLAYOUT_PANEL
value. */ public static final int XDCLAYOUT_PANEL = EnumType.XDCLAYOUT | 1; /** * The runat attribute'sRUNAT_CLIENT
value. */ public static final int RUNAT_CLIENT = EnumType.RUNAT | 0; /** * The runat attribute'sRUNAT_SERVER
value. */ public static final int RUNAT_SERVER = EnumType.RUNAT | 1; /** * The runat attribute'sRUNAT_BOTH
value. */ public static final int RUNAT_BOTH = EnumType.RUNAT | 2; /** * The priority attribute'sPRIORITY_CUSTOM
value. */ public static final int PRIORITY_CUSTOM = EnumType.PRIORITY | 0; /** * The priority attribute'sPRIORITY_TOOLTIP
value. */ public static final int PRIORITY_TOOLTIP = EnumType.PRIORITY | 1; /** * The priority attribute'sPRIORITY_CAPTION
value. */ public static final int PRIORITY_CAPTION = EnumType.PRIORITY | 2; /** * The priority attribute'sPRIORITY_NAME
value. */ public static final int PRIORITY_NAME = EnumType.PRIORITY | 3; /** * The usage attribute'sUSAGE_EXPORTONLY
value. */ public static final int USAGE_EXPORTONLY = EnumType.USAGE | 0; /** * The usage attribute'sUSAGE_IMPORTONLY
value. */ public static final int USAGE_IMPORTONLY = EnumType.USAGE | 1; /** * The usage attribute'sUSAGE_EXPORTANDIMPORT
value. */ public static final int USAGE_EXPORTANDIMPORT = EnumType.USAGE | 2; /** * The executetype attribute'sEXECUTETYPE_IMPORT
value. */ public static final int EXECUTETYPE_IMPORT = EnumType.EXECUTETYPE | 0; /** * The executetype attribute'sEXECUTETYPE_REMERGE
value. */ public static final int EXECUTETYPE_REMERGE = EnumType.EXECUTETYPE | 1; /** * The data attribute'sDATA_LINK
value. */ public static final int DATA_LINK = EnumType.DATA | 0; /** * The data attribute'sDATA_EMBED
value. */ public static final int DATA_EMBED = EnumType.DATA | 1; /** * The DATAPREP attribute's DATAPREP_NONE value. */ public static final int DATAPREP_NONE = EnumType.DATAPREP | 0; /** * The dataprep attribute'sDATAPREP_FLATECOMPRESS
value. */ public static final int DATAPREP_FLATECOMPRESS = EnumType.DATAPREP | 1; /** * The signoperation attribute'sSIGNOPERATION_SIGN
value. */ public static final int SIGNOPERATION_SIGN = EnumType.SIGNOPERATION | 0; /** * The signoperation attribute'sSIGNOPERATION_VERIFY
value. */ public static final int SIGNOPERATION_VERIFY = EnumType.SIGNOPERATION | 1; /** * The signoperation attribute'sSIGNOPERATION_CLEAR
value. */ public static final int SIGNOPERATION_CLEAR = EnumType.SIGNOPERATION | 2; /** * The requirement attribute'sREQUIREMENT_REQUIRED
value. */ public static final int REQUIREMENT_REQUIRED = EnumType.REQUIREMENT | 0; /** * The requirement attribute'sREQUIREMENT_OPTIONAL
value. */ public static final int REQUIREMENT_OPTIONAL = EnumType.REQUIREMENT | 1; /** * The commiton attribute'sCOMMITON_EXIT
value. */ public static final int COMMITON_EXIT = EnumType.COMMITON | 0; /** * The commiton attribute'sCOMMITON_SELECT
value. */ public static final int COMMITON_SELECT = EnumType.COMMITON | 1; /** * The dynamicrender attribute'sDYNAMICRENDER_FORBIDDEN
value. */ public static final int DYNAMICRENDER_FORBIDDEN = EnumType.DYNAMICRENDER | 0; /** * The dynamicrender attribute'sDYNAMICRENDER_REQUIRED
value. */ public static final int DYNAMICRENDER_REQUIRED = EnumType.DYNAMICRENDER | 1; /** * The batchformat attribute'sBATCHFORMAT_NONE
value. */ public static final int BATCHFORMAT_NONE = EnumType.BATCHFORMAT | 0; /** * The batchformat attribute'sBATCHFORMAT_ZIP
value. */ public static final int BATCHFORMAT_ZIP = EnumType.BATCHFORMAT | 1; /** * The batchformat attribute'sBATCHFORMAT_ZIPCOMPRESS
value. */ public static final int BATCHFORMAT_ZIPCOMPRESS = EnumType.BATCHFORMAT | 2; /** * The batchformat attribute'sBATCHFORMAT_CONCAT
value. */ public static final int BATCHFORMAT_CONCAT = EnumType.BATCHFORMAT | 3; /** * The renderpolicy attribute'sRENDERPOLICY_SERVER
value. */ public static final int RENDERPOLICY_SERVER = EnumType.RENDERPOLICY | 0; /** * The renderpolicy attribute'sRENDERPOLICY_CLIENT
value. */ public static final int RENDERPOLICY_CLIENT = EnumType.RENDERPOLICY | 1; /** * The writingscript attribute'sWRITINGSCRIPT_STAR
value. */ public static final int WRITINGSCRIPT_STAR = EnumType.WRITINGSCRIPT | 0; /** * The writingscript attribute'sWRITINGSCRIPT_ROMAN
value. */ public static final int WRITINGSCRIPT_ROMAN = EnumType.WRITINGSCRIPT | 1; /** * The writingscript attribute'sWRITINGSCRIPT_EASTEUROPEANROMAN
value. */ public static final int WRITINGSCRIPT_EASTEUROPEANROMAN = EnumType.WRITINGSCRIPT | 2; /** * The writingscript attribute'sWRITINGSCRIPT_GREEK
value. */ public static final int WRITINGSCRIPT_GREEK = EnumType.WRITINGSCRIPT | 3; /** * The writingscript attribute'sWRITINGSCRIPT_CYRILLIC
value. */ public static final int WRITINGSCRIPT_CYRILLIC = EnumType.WRITINGSCRIPT | 4; /** * The writingscript attribute'sWRITINGSCRIPT_JAPANESE
value. */ public static final int WRITINGSCRIPT_JAPANESE = EnumType.WRITINGSCRIPT | 5; /** * The writingscript attribute'sWRITINGSCRIPT_TRADITIONALCHINESE
value. */ public static final int WRITINGSCRIPT_TRADITIONALCHINESE = EnumType.WRITINGSCRIPT | 6; /** * The writingscript attribute'sWRITINGSCRIPT_SIMPLIFIEDCHINESE
value. */ public static final int WRITINGSCRIPT_SIMPLIFIEDCHINESE = EnumType.WRITINGSCRIPT | 7; /** * The writingscript attribute'sWRITINGSCRIPT_KOREAN
value. */ public static final int WRITINGSCRIPT_KOREAN = EnumType.WRITINGSCRIPT | 8; /** * The writingscript attribute'sWRITINGSCRIPT_ARABIC
value. */ public static final int WRITINGSCRIPT_ARABIC = EnumType.WRITINGSCRIPT | 9; /** * The writingscript attribute'sWRITINGSCRIPT_HEBREW
value. */ public static final int WRITINGSCRIPT_HEBREW = EnumType.WRITINGSCRIPT | 10; /** * The writingscript attribute'sWRITINGSCRIPT_THAI
value. */ public static final int WRITINGSCRIPT_THAI = EnumType.WRITINGSCRIPT | 11; /** * The writingscript attribute'sWRITINGSCRIPT_VIETNAMESE
value. */ public static final int WRITINGSCRIPT_VIETNAMESE = EnumType.WRITINGSCRIPT | 12; /** * The taggedmode attribute'sTAGGEDMODE_NORMAL
value. */ public static final int TAGGEDMODE_NORMAL = EnumType.TAGGEDMODE | 0; /** * The taggedmode attribute'sTAGGEDMODE_CUSTOM
value. */ public static final int TAGGEDMODE_CUSTOM = EnumType.TAGGEDMODE | 1; /** * The runscriptsattribute's RUNSCRIPTS_CLIENT
value. */ public static final int RUNSCRIPTS_CLIENT = EnumType.RUNSCRIPTS | 0; /** * The runscriptsattribute's RUNSCRIPTS_SERVER
value. */ public static final int RUNSCRIPTS_SERVER = EnumType.RUNSCRIPTS | 1; /** * The runscriptsattribute's RUNSCRIPTS_BOTH
value. */ public static final int RUNSCRIPTS_BOTH = EnumType.RUNSCRIPTS | 2; /** * The runscriptsattribute's RUNSCRIPTS_NONE
value. */ public static final int RUNSCRIPTS_NONE = EnumType.RUNSCRIPTS | 3; /** * The manifestaction attribute'sMANIFESTACTION_INCLUDE
value. */ public static final int MANIFESTACTION_INCLUDE = EnumType.MANIFESTACTION | 0; /** * The manifestaction attribute'sMANIFESTACTION_EXCLUDE
value. */ public static final int MANIFESTACTION_EXCLUDE = EnumType.MANIFESTACTION | 1; /** * The manifestaction attribute'sMANIFESTACTION_ALL
value. */ public static final int MANIFESTACTION_ALL = EnumType.MANIFESTACTION | 2; /** * The mdpsignaturetype attribute'sMDPSIGNATURETYPE_FILLER
value. */ public static final int MDPSIGNATURETYPE_FILLER = EnumType.MDPSIGNATURETYPE | 0; /** * The mdpsignaturetype attribute'sMDPSIGNATURETYPE_AUTHOR
value. */ public static final int MDPSIGNATURETYPE_AUTHOR = EnumType.MDPSIGNATURETYPE | 1; /** * The mdppermissionsattribute's MDPPERMISSIONS_ONE
value. */ public static final int MDPPERMISSIONS_ONE = EnumType.MDPPERMISSIONS | 0; /** * The mdppermissionsattribute's MDPPERMISSIONS_TWO
value. */ public static final int MDPPERMISSIONS_TWO = EnumType.MDPPERMISSIONS | 1; /** * The mdppermissionsattribute's MDPPERMISSIONS_THREE
value. */ public static final int MDPPERMISSIONS_THREE = EnumType.MDPPERMISSIONS | 2; /** * The pagesetrelation attribute'sPAGESETRELATION_ORDERED_OCCURRENCE
value. */ public static final int PAGESETRELATION_ORDERED_OCCURRENCE = EnumType.PAGESETRELATION | 0; /** * The pagesetrelation attribute'sPAGESETRELATION_SIMPLEX_PAGINATED
value. */ public static final int PAGESETRELATION_SIMPLEX_PAGINATED = EnumType.PAGESETRELATION | 1; /** * The pagesetrelation attribute'sPAGESETRELATION_DUPLEX_PAGINATED
value. */ public static final int PAGESETRELATION_DUPLEX_PAGINATED = EnumType.PAGESETRELATION | 2; /** * The pageposition attribute'sPAGEPOSITION_FIRST
value. */ public static final int PAGEPOSITION_FIRST = EnumType.PAGEPOSITION | 0; /** * The pageposition attribute'sPAGEPOSITION_LAST
value. */ public static final int PAGEPOSITION_LAST = EnumType.PAGEPOSITION | 1; /** * The pageposition attribute'sPAGEPOSITION_REST
value. */ public static final int PAGEPOSITION_REST = EnumType.PAGEPOSITION | 2; /** * The pageposition attribute'sPAGEPOSITION_ONLY
value. */ public static final int PAGEPOSITION_ONLY = EnumType.PAGEPOSITION | 3; /** * The pageposition attribute'sPAGEPOSITION_ANY
value. */ public static final int PAGEPOSITION_ANY = EnumType.PAGEPOSITION | 4; /** * The oddoreven attribute'sODDOREVEN_ODD
value. */ public static final int ODDOREVEN_ODD = EnumType.ODDOREVEN | 0; /** * The oddoreven attribute'sODDOREVEN_EVEN
value. */ public static final int ODDOREVEN_EVEN = EnumType.ODDOREVEN | 1; /** * The oddoreven attribute'sODDOREVEN_ANY
value. */ public static final int ODDOREVEN_ANY = EnumType.ODDOREVEN | 2; /** * The blankornotblank attribute'sBLANKORNOTBLANK_BLANK
value. */ public static final int BLANKORNOTBLANK_BLANK = EnumType.BLANKORNOTBLANK | 0; /** * The blankornotblank attribute'sBLANKORNOTBLANK_NOTBLANK
value. */ public static final int BLANKORNOTBLANK_NOTBLANK = EnumType.BLANKORNOTBLANK | 1; /** * The blankornotblank attribute'sBLANKORNOTBLANK_ANY
value. */ public static final int BLANKORNOTBLANK_ANY = EnumType.BLANKORNOTBLANK | 2; /** * The scrollpolicy attribute'sSCROLLPOLICY_AUTO
value. */ public static final int SCROLLPOLICY_AUTO = EnumType.SCROLLPOLICY | 0; /** * The scrollpolicy attribute'sSCROLLPOLICY_ON
value. */ public static final int SCROLLPOLICY_ON = EnumType.SCROLLPOLICY | 1; /** * The scrollpolicy attribute'sSCROLLPOLICY_OFF
value. */ public static final int SCROLLPOLICY_OFF = EnumType.SCROLLPOLICY | 2; /** * The baseprofile attribute'sBASEPROFILE_FULL
value. */ public static final int BASEPROFILE_FULL = EnumType.BASEPROFILE | 0; /** * The baseprofile attribute'sBASEPROFILE_INTERACTIVEFORMS
value. */ public static final int BASEPROFILE_INTERACTIVEFORMS = EnumType.BASEPROFILE | 1; /** * The duplexoption attribute'sDUPLEXOPTION_SIMPLEX
value. */ public static final int DUPLEXOPTION_SIMPLEX = EnumType.DUPLEXOPTION | 0; /** * The duplexoption attribute'sDUPLEXOPTION_DUPLEXFLIPSHORTEDGE
value. */ public static final int DUPLEXOPTION_DUPLEXFLIPSHORTEDGE = EnumType.DUPLEXOPTION | 1; /** * The duplexoption attribute'sDUPLEXOPTION_DUPLEXFLIPLONGEDGE
value. */ public static final int DUPLEXOPTION_DUPLEXFLIPLONGEDGE = EnumType.DUPLEXOPTION | 2; /** * The mark attribute'sMARK_CHECK
value. */ public static final int MARK_CHECK = EnumType.MARK | 0; /** * The mark attribute'sMARK_CIRCLE
value. */ public static final int MARK_CIRCLE = EnumType.MARK | 1; /** * The mark attribute'sMARK_CROSS
value. */ public static final int MARK_CROSS = EnumType.MARK | 2; /** * The mark attribute'sMARK_DIAMOND
value. */ public static final int MARK_DIAMOND = EnumType.MARK | 3; /** * The mark attribute'sMARK_SQUARE
value. */ public static final int MARK_SQUARE = EnumType.MARK | 4; /** * The mark attribute'sMARK_STAR
value. */ public static final int MARK_STAR = EnumType.MARK | 5; /** * The mark attribute'sMARK_DEFAULT
value. */ public static final int MARK_DEFAULT = EnumType.MARK | 6; /** * The highlight attribute'sHIGHLIGHT_NONE
value. */ public static final int HIGHLIGHT_NONE = EnumType.HIGHLIGHT | 0; /** * The highlight attribute'sHIGHLIGHT_INVERTED
value. */ public static final int HIGHLIGHT_INVERTED = EnumType.HIGHLIGHT | 1; /** * The highlight attribute'sHIGHLIGHT_PUSH
value. */ public static final int HIGHLIGHT_PUSH = EnumType.HIGHLIGHT | 2; /** * The highlight attribute'sHIGHLIGHT_OUTLINE
value. */ public static final int HIGHLIGHT_OUTLINE = EnumType.HIGHLIGHT | 3; /** * The restorestate attribute'sRESTORESTATE_MANUAL
value. */ public static final int RESTORESTATE_MANUAL = EnumType.RESTORESTATE | 0; /** * The restorestate attribute'sRESTORESTATE_AUTO
value. */ public static final int RESTORESTATE_AUTO = EnumType.RESTORESTATE | 1; /** * The pagination attribute'sPAGINATION_SIMPLEX
value. */ public static final int PAGINATION_SIMPLEX = EnumType.PAGINATION | 0; /** * The pagination attribute'sPAGINATION_DUPLEX_LONG_EDGE
value. */ public static final int PAGINATION_DUPLEX_LONG_EDGE = EnumType.PAGINATION | 1; /** * The pagination attribute'sPAGINATION_DUPLEX_SHORT_EDGE
value. */ public static final int PAGINATION_DUPLEX_SHORT_EDGE = EnumType.PAGINATION | 2; /** * The jogoption attribute'sJOG_USEPRINTERSETTING
value. */ public static final int JOG_USEPRINTERSETTING = EnumType.JOGOPTION | 0; /** * The jogoption attribute'sJOG_NONE
value. */ public static final int JOG_NONE = EnumType.JOGOPTION | 1; /** * The jogoption attribute'sJOG_PAGESET
value. */ public static final int JOG_PAGESET = EnumType.JOGOPTION | 2; /** * The addrevocationinfo attribute'sADDREVOCATIONINFO_REQUIRED
value. */ public static final int ADDREVOCATIONINFO_REQUIRED = EnumType.ADDREVOCATIONINFO | 0; /** * The addrevocationinfo attribute'sADDREVOCATIONINFO_OPTIONAL
value. */ public static final int ADDREVOCATIONINFO_OPTIONAL = EnumType.ADDREVOCATIONINFO | 1; /** * The addrevocationinfo attribute'sADDREVOCATIONINFO_NONE
value. */ public static final int ADDREVOCATIONINFO_NONE = EnumType.ADDREVOCATIONINFO | 2; /** * The upsmode attribute'sUPSMODE_USCARRIER
value. */ public static final int UPSMODE_USCARRIER = EnumType.UPSMODE | 0; /** * The upsmode attribute'sUPSMODE_INTERNATIONALCARRIER
value. */ public static final int UPSMODE_INTERNATIONALCARRIER = EnumType.UPSMODE | 1; /** * The upsmode attribute'sUPSMODE_STANDARDSYMBOL
value. */ public static final int UPSMODE_STANDARDSYMBOL = EnumType.UPSMODE | 2; /** * The upsmode attribute'sUPSMODE_SECURESYMBOL
value. */ public static final int UPSMODE_SECURESYMBOL = EnumType.UPSMODE | 3; /** * The sourcebelow attribute'sSOURCEBELOW_UPDATE
value. */ public static final int SOURCEBELOW_UPDATE = EnumType.SOURCEBELOW | 0; /** * The sourcebelow attribute'sSOURCEBELOW_MAINTAIN
value. */ public static final int SOURCEBELOW_MAINTAIN = EnumType.SOURCEBELOW | 1; /** * The sourceabove attribute'sSOURCEABOVE_WARN
value. */ public static final int SOURCEABOVE_WARN = EnumType.SOURCEABOVE | 0; /** * The sourceabove attribute'sSOURCEABOVE_ERROR
value. */ public static final int SOURCEABOVE_ERROR = EnumType.SOURCEABOVE | 1; /** * The outputbelow attribute'sOUTPUTBELOW_UPDATE
value. */ public static final int OUTPUTBELOW_UPDATE = EnumType.OUTPUTBELOW | 0; /** * The outputbelow attribute'sOUTPUTBELOW_WARN
value. */ public static final int OUTPUTBELOW_WARN = EnumType.OUTPUTBELOW | 1; /** * The outputbelow attribute'sOUTPUTBELOW_ERROR
value. */ public static final int OUTPUTBELOW_ERROR = EnumType.OUTPUTBELOW | 2; /** * The compressscope attribute'sCOMPRESSSCOPE_IMAGEONLY
value. */ public static final int COMPRESSSCOPE_IMAGEONLY = EnumType.COMPRESSSCOPE | 0; /** * The compressscope attribute'sCOMPRESSSCOPE_DOCUMENT
value. */ public static final int COMPRESSSCOPE_DOCUMENT = EnumType.COMPRESSSCOPE | 1; /** * The staplemode attribute'sSTAPLEMODE_ON
value. */ public static final int STAPLEMODE_ON = EnumType.STAPLEMODE | 0; /** * The staplemode attribute'sSTAPLEMODE_OFF
value. */ public static final int STAPLEMODE_OFF = EnumType.STAPLEMODE | 1; /** * The staplemode attribute'sSTAPLEMODE_USEPRINTERSETTING
value. */ public static final int STAPLEMODE_USEPRINTERSETTING = EnumType.STAPLEMODE | 2; /** * The pdfaconformance attribute'sPDFACONFORMANCE_A
value. */ public static final int PDFACONFORMANCE_A = EnumType.PDFACONFORMANCE | 0; /** * The pdfaconformance attribute'sPDFACONFORMANCE_B
value. */ public static final int PDFACONFORMANCE_B = EnumType.PDFACONFORMANCE | 1; /** * The genericfamily attribute'sGENERICFAMILY_SANSSERIF
value. */ public static final int GENERICFAMILY_SANSSERIF = EnumType.GENERICFAMILY | 0; /** * The genericfamily attribute'sGENERICFAMILY_SERIF
value. */ public static final int GENERICFAMILY_SERIF = EnumType.GENERICFAMILY | 1; /** * The genericfamily attribute'sGENERICFAMILY_CURSIVE
value. */ public static final int GENERICFAMILY_CURSIVE = EnumType.GENERICFAMILY | 2; /** * The genericfamily attribute'sGENERICFAMILY_FANTASY
value. */ public static final int GENERICFAMILY_FANTASY = EnumType.GENERICFAMILY | 3; /** * The genericfamily attribute'sGENERICFAMILY_MONOSPACE
value. */ public static final int GENERICFAMILY_MONOSPACE = EnumType.GENERICFAMILY | 4; /** * The genericfamily attribute'sGENERICFAMILY_UNKNOWN
value. */ public static final int GENERICFAMILY_UNKNOWN = EnumType.GENERICFAMILY | 5; /** * The overprint attribute'sOVERPRINT_NONE
value. */ public static final int OVERPRINT_NONE = EnumType.OVERPRINT | 0; /** * The overprint attribute'sOVERPRINT_DRAWS
value. */ public static final int OVERPRINT_DRAWS = EnumType.OVERPRINT | 1; /** * The overprint attribute'sOVERPRINT_FIELDS
value. */ public static final int OVERPRINT_FIELDS = EnumType.OVERPRINT | 2; /** * The overprint attribute'sOVERPRINT_DRAWSANDFIELDS
value. */ public static final int OVERPRINT_DRAWSANDFIELDS = EnumType.OVERPRINT | 3; /** * The kerningmode attribute'sKERNINGMODE_NONE
value. */ public static final int KERNINGMODE_NONE = EnumType.KERNINGMODE | 0; /** * The kerningmode attribute'sKERNINGMODE_PAIR
value. */ public static final int KERNINGMODE_PAIR = EnumType.KERNINGMODE | 1; /** * The picker attribute'sPICKER_NONE
value. */ public static final int PICKER_NONE = EnumType.PICKER | 0; /** * The picker attribute'sPICKER_HOST
value. */ public static final int PICKER_HOST = EnumType.PICKER | 1; /** * The paginationoverride attribute'sPAGINATIONOVERRIDE_NONE
value. */ public static final int PAGINATIONOVERRIDE_NONE = EnumType.PAGINATIONOVERRIDE | 0; /** * The paginationoverride attribute'sPAGINATIONOVERRIDE_FORCE_SIMPLEX
value. */ public static final int PAGINATIONOVERRIDE_FORCE_SIMPLEX = EnumType.PAGINATIONOVERRIDE | 1; /** * The paginationoverride attribute'sPAGINATIONOVERRIDE_FORCE_DUPLEX
value. */ public static final int PAGINATIONOVERRIDE_FORCE_DUPLEX = EnumType.PAGINATIONOVERRIDE | 2; /** * The paginationoverride attribute'sPAGINATIONOVERRIDE_FORCE_DUPLEX_LONG_EDGE
value. */ public static final int PAGINATIONOVERRIDE_FORCE_DUPLEX_LONG_EDGE = EnumType.PAGINATIONOVERRIDE | 3; /** * The paginationoverride attribute'sPAGINATIONOVERRIDE_FORCE_DUPLEX_SHORT_EDGE
value. */ public static final int PAGINATIONOVERRIDE_FORCE_DUPLEX_SHORT_EDGE = EnumType.PAGINATIONOVERRIDE | 4; /** * The validationmessaging attribute'sVALIDATIONMESSAGING_NOMESSAGES
value. */ public static final int VALIDATIONMESSAGING_NOMESSAGES = EnumType.VALIDATIONMESSAGING | 0; /** * The validationmessaging attribute'sVALIDATIONMESSAGING_FIRSTMESSAGEONLY
value. */ public static final int VALIDATIONMESSAGING_FIRSTMESSAGEONLY = EnumType.VALIDATIONMESSAGING | 1; /** * The validationmessaging attribute'sVALIDATIONMESSAGING_ALLMESSAGESINDIVIDUALLY
value. */ public static final int VALIDATIONMESSAGING_ALLMESSAGESINDIVIDUALLY = EnumType.VALIDATIONMESSAGING | 2; /** * The validationmessaging attribute'sVALIDATIONMESSAGING_ALLMESSAGESTOGETHER
value. */ public static final int VALIDATIONMESSAGING_ALLMESSAGESTOGETHER = EnumType.VALIDATIONMESSAGING | 3; /** * The eventlisten attribute'sEVENTLISTEN_REFONLY
value. */ public static final int EVENTLISTEN_REFONLY = EnumType.EVENTLISTEN | 0; /** * The eventlisten attribute'sEVENTLISTEN_REFANDDESCENDENTS
value. */ public static final int EVENTLISTEN_REFANDDESCENDENTS = EnumType.EVENTLISTEN | 1; /** * The printscaling attribute'sPRINTSCALING_NOSCALING
value. */ public static final int PRINTSCALING_NOSCALING = EnumType.PRINTSCALING | 0; /** * The printscaling attribute'sPRINTSCALING_APPDEFAULT
value. */ public static final int PRINTSCALING_APPDEFAULT = EnumType.PRINTSCALING | 1; /** * The dupleximposition attribute'sDUPLEXIMPOSITION_LONGEDGE
value. */ public static final int DUPLEXIMPOSITION_LONGEDGE = EnumType.DUPLEXIMPOSITION | 0; /** * The dupleximposition attribute'sDUPLEXIMPOSITION_SHORTEDGE
value. */ public static final int DUPLEXIMPOSITION_SHORTEDGE = EnumType.DUPLEXIMPOSITION | 1; /** * The credentialpolicy attribute'sCREDENTIAL_REQUIRED
value. */ public static final int CREDENTIAL_REQUIRED = EnumType.CREDENTIALPOLICY | 0; /** * The credentialpolicy attribute'sCREDENTIAL_OPTIONAL
value. */ public static final int CREDENTIAL_OPTIONAL = EnumType.CREDENTIALPOLICY | 1; static final EnumAttr[][] gEnumValues = new EnumAttr[][] { // JavaPort { new EnumAttr(EnumAttr.UNDEFINED, "", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // access { new EnumAttr(EnumAttr.ACCESS_PROTECTED, "protected", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFASUBSET | Schema.XFAAVAILABILITY_XFAF), // ACCESS_* added with CL 30903 new EnumAttr(EnumAttr.ACCESS_OPEN, "open", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFASUBSET | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACCESS_READONLY, "readOnly", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFASUBSET | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACCESS_NONINTERACTIVE, "nonInteractive", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFASUBSET | Schema.XFAAVAILABILITY_XFAF) }, // ACCESS_NONINTERACTIVE with CL 68172 // anchor { new EnumAttr(EnumAttr.TOP_LEFT, "topLeft", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // TOP_* added with CL 30903 new EnumAttr(EnumAttr.TOP_CENTER, "topCenter", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TOP_RIGHT, "topRight", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MIDDLE_LEFT, "middleLeft", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MIDDLE_CENTER, "middleCenter", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MIDDLE_RIGHT, "middleRight", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.BOTTOM_LEFT, "bottomLeft", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.BOTTOM_CENTER, "bottomCenter", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.BOTTOM_RIGHT, "bottomRight", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // attributes { new EnumAttr(EnumAttr.ATTRIBUTE_IGNORE, "ignore", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.ATTRIBUTE_DELEGATE, "delegate", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.ATTRIBUTE_PRESERVE, "preserve", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // ATTRIBUTE_* added with CL 34081 // boolean { new EnumAttr(EnumAttr.BOOL_FALSE, "0", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.BOOL_TRUE, "1", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // BOOL_* added with CL 30903 // cap { new EnumAttr(EnumAttr.BUTT, "butt", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ROUND, "round", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.SQUARE, "square", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // BUTT ROUND SQUARE added with CL 30903 // controlCode { new EnumAttr(EnumAttr.CONTROL_ESC, "ESC", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.CONTROL_LF, "LF", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.CONTROL_CR, "CR", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.CONTROL_FF, "FF", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // CONTROL_* added with CL 30903 // dest { new EnumAttr(EnumAttr.MEMORY, "memory", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.FILE, "file", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // MEMORY FILE added with CL 30903 // destOp { new EnumAttr(EnumAttr.APPEND, "append", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.SET, "set", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // APPEND SET added with CL 30903 // encoding { new EnumAttr(EnumAttr.ISO_8859_1, "ISO-8859-1", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), // ISO_* added with CL 30903 new EnumAttr(EnumAttr.ISO_8859_2, "ISO-8859-2", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.ISO_8859_5, "ISO-8859-5", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), // ISO_8859_5 with CL 108585 new EnumAttr(EnumAttr.ISO_8859_6, "ISO-8859-6", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.ISO_8859_7, "ISO-8859-7", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), // ISO_8859_7 with CL 35338 new EnumAttr(EnumAttr.ISO_8859_8, "ISO-8859-8", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), // ISO_8859_8 with CL 108585 new EnumAttr(EnumAttr.ISO_8859_9, "ISO-8859-9", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.SHIFT_JIS, "Shift-JIS", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), // SHIFT_JIS with CL 30903 new EnumAttr(EnumAttr.KSC_5601, "KSC-5601", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.BIG_5, "BIG-5", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), // BIG_5 with CL 65764 new EnumAttr(EnumAttr.HKSCS_BIG5, "HKSCS-BIG5", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.GBK, "GBK", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.UTF_8, "UTF-8", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), // UTF_8 with CL 30903 new EnumAttr(EnumAttr.UTF_16, "UTF-16", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.UCS_2, "UCS-2", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.ENCODING_NONE, "none", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.FONT_SPECIFIC, "fontSpecific", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.IBM_PC850, "IBM850", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_ALL), // IBM_PC850 with CL 241286 new EnumAttr(EnumAttr.GB18030, "GB18030", Schema.XFAVERSION_28, Schema.XFAAVAILABILITY_ALL), // GB18030 with CL 416817 new EnumAttr(EnumAttr.JIS2004, "JIS2004", Schema.XFAVERSION_28, Schema.XFAAVAILABILITY_ALL) }, // JIS2004 with CL 433356 // encryptionLevel { new EnumAttr(EnumAttr.ENCRYPTLEVEL_40, "40bit", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.ENCRYPTLEVEL_128, "128bit", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // ENCRYPTLEVEL_* added with CL 38097 // exception { new EnumAttr(EnumAttr.EXCEPTION_FATAL, "fatal", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.EXCEPTION_IGNORE, "ignore", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.EXCEPTION_WARNING, "warning", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // EXCEPTION_* added with CL 30903 // fontLineThrough { new EnumAttr(EnumAttr.LINETHROUGH_ZERO, "0", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.LINETHROUGH_SINGLE, "1", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.LINETHROUGH_DOUBLE, "2", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // LINETHROUGH_* added with CL 30903 // fontLineThroughPeriod { new EnumAttr(EnumAttr.LINETHROUGH_ALL, "all", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.LINETHROUGH_WORD, "word", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // LINETHROUGH_* added with CL 30903 // fontOverline { new EnumAttr(EnumAttr.OVERLINE_ZERO, "0", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.OVERLINE_SINGLE, "1", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.OVERLINE_DOUBLE, "2", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // OVERLINE_* added with CL 30903 // fontOverlinePeriod { new EnumAttr(EnumAttr.OVER_ALL, "all", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.OVER_WORD, "word", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // OVER_* added with CL 30903 // fontPitch { new EnumAttr(EnumAttr.FIXED, "fixed", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.VARIABLE, "variable", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // FIXED VARIABLE added with CL 30903 // fontPosture { new EnumAttr(EnumAttr.POSTURE_ITALIC, "italic", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.POSTURE_NORMAL, "normal", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // POSTURE_* added with CL 30903 // fontUnderline { new EnumAttr(EnumAttr.UNDER_ZERO, "0", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.UNDER_SINGLE, "1", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.UNDER_DOUBLE, "2", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // UNDER_* added with CL 30903 // fontUnderlinePeriod { new EnumAttr(EnumAttr.UNDER_ALL, "all", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.UNDER_WORD, "word", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // UNDER_* added with CL 30903 // fontWeight { new EnumAttr(EnumAttr.WEIGHT_NORMAL, "normal", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.WEIGHT_BOLD, "bold", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // WEIGHT_* added with CL 30903 // fraction { new EnumAttr(EnumAttr.FRACTION_ROUND, "round", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.FRACTION_TRUNC, "trunc", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // FRACTION_* added with CL 30903 // hAlign { new EnumAttr(EnumAttr.HALIGN_LEFT, "left", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // HALIGN_* added with CL 30903 new EnumAttr(EnumAttr.HALIGN_CENTER, "center", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.HALIGN_RIGHT, "right", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.HALIGN_JUSTIFY, "justify", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.HALIGN_JUSTIFY_ALL, "justifyAll", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.HALIGN_RADIX, "radix", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // HALIGN_RADIX with CL 66265 // hand { new EnumAttr(EnumAttr.HAND_LEFT, "left", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.HAND_RIGHT, "right", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.HAND_EVEN, "even", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // HAND_* added with CL 30903 // ifEmpty { new EnumAttr(EnumAttr.IFEMPTY_IGNORE, "ignore", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.IFEMPTY_REMOVE, "remove", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.IFEMPTY_DATAGROUP, "dataGroup", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.IFEMPTY_DATAVALUE, "dataValue", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // IFEMPTY_* added with CL 34691 // imagingBBox { new EnumAttr(EnumAttr.IMAGINGBBOX_NONE, "none", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // IMAGINGBBOX_* added with CL 30903 // join { new EnumAttr(EnumAttr.JOIN_ROUND, "round", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.JOIN_SQUARE, "square", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // JOIN_* added with CL 30903 // layout { new EnumAttr(EnumAttr.LEFT_RIGHT_TOP_BOTTOM, "lr-tb", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // LEFT_* added with CL 30903 new EnumAttr(EnumAttr.RIGHT_LEFT_TOP_BOTTOM, "rl-tb", Schema.XFAVERSION_33, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TOP_BOTTOM, "tb", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.POSITION, "position", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // POSITION with CL 33120 new EnumAttr(EnumAttr.TABLE, "table", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // TABLE with CL 39739 new EnumAttr(EnumAttr.ROW, "row", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ROW with CL 59420 new EnumAttr(EnumAttr.RIGHT_LEFT_ROW, "rl-row", Schema.XFAVERSION_33, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, //RIGHT_LEFT_ROW with CL 621982 // linear { new EnumAttr(EnumAttr.TO_RIGHT, "toRight", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TO_BOTTOM, "toBottom", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TO_LEFT, "toLeft", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TO_TOP, "toTop", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // TO_* added with CL 30903 // lineend { new EnumAttr(EnumAttr.LINEEND_ITC, "itc", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.LINEEND_ITCCR, "itccr", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.LINEEND_CRCR, "crcr", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.LINEEND_CR, "cr", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // LINEEND_* added with CL 34092 // logto { new EnumAttr(EnumAttr.LOG_URI, "uri", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.LOG_MEMORY, "memory", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.LOG_STDOUT, "stdout", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.LOG_NULL, "null", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.LOG_STDERROR, "stderror", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.LOG_SYSTEM, "system", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // LOG_* added with CL 34081 // match { new EnumAttr(EnumAttr.MATCH_NONE, "none", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // MATCH_* added with CL 34691 new EnumAttr(EnumAttr.MATCH_ONCE, "once", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MATCH_GLOBAL, "global", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MATCH_DATAREF, "dataRef", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // MATCH_DATAREF with CL 37708 // messageType { new EnumAttr(EnumAttr.MESSAGE_TRACE, "trace", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), // MESSAGE_* added with CL 34081 new EnumAttr(EnumAttr.MESSAGE_INFORMATION, "information", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.MESSAGE_WARNING, "warning", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.MESSAGE_ERROR, "error", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // MESSAGE_WARNING with CL 30903 // mode { new EnumAttr(EnumAttr.MODE_APPEND, "append", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.MODE_OVERWRITE, "overwrite", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // MODE_* added with CL 34081 // nodePresence { new EnumAttr(EnumAttr.NODEPRESENCE_IGNORE, "ignore", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), // NODEPRESENCE_* added with CL 34691 new EnumAttr(EnumAttr.NODEPRESENCE_DISSOLVE, "dissolve", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.NODEPRESENCE_PRESERVE, "preserve", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.NODEPRESENCE_DISSOLVESTRUCTURE, "dissolveStructure", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) },// NODEPRESENCE_DISSOLVESTRUCTURE with CL 40028 // open { new EnumAttr(EnumAttr.USER_CONTROL, "userControl", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF),// USER_* added with CL 30903 new EnumAttr(EnumAttr.ON_ENTRY, "onEntry", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ALWAYS, "always", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MULTISELECT, "multiSelect", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) },// MULTISELECT with CL 70412 // operation { new EnumAttr(EnumAttr.OPERATION_UP, "up", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.OPERATION_DOWN, "down", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.OPERATION_LEFT, "left", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.OPERATION_RIGHT, "right", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.OPERATION_BACK, "back", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.OPERATION_FIRST, "first", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.OPERATION_NEXT, "next", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // OPERATION_* added with CL 30903 // order { new EnumAttr(EnumAttr.ORDER_DOCUMENT, "document", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.ORDER_DATAVALUESFIRST, "dataValuesFirst", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // ORDER_* added with CL 34092 // orientation { new EnumAttr(EnumAttr.PORTRAIT, "portrait", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.LANDSCAPE, "landscape", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // PORTRAIT LANDSCAPE_* added with CL 30903 // outputto { new EnumAttr(EnumAttr.OUTPUT_URI, "uri", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.OUTPUT_MEMORY, "memory", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.OUTPUT_STDOUT, "stdout", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.OUTPUT_NULL, "null", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // OUTPUT_* added with CL 34081 // override { new EnumAttr(EnumAttr.OVERRIDE_DISABLED, "disabled", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.OVERRIDE_WARNING, "warning", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.OVERRIDE_ERROR, "error", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.OVERRIDE_IGNORE, "ignore", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // OVERRIDE_* added with CL 34081 // pattern { new EnumAttr(EnumAttr.HORIZONTAL_HATCHING, "horizontal", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.VERTICAL_HATCHING, "vertical", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.CROSS_HATCHING, "crossHatch", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.DIAGONAL_LEFT_HATCHING, "diagonalLeft", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.DIAGONAL_RIGHT_HATCHING, "diagonalRight", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.CROSS_DIAGONAL_HATCHING, "crossDiagonal", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) },// HORIZONTAL_* added with CL 30903 // presense { new EnumAttr(EnumAttr.PRESENCE_VISIBLE, "visible", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFASUBSET | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.PRESENCE_INVISIBLE, "invisible", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFASUBSET | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.PRESENCE_HIDDEN, "hidden", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFASUBSET | Schema.XFAAVAILABILITY_XFAF), // PRESENCE_* added with CL 30903 new EnumAttr(EnumAttr.PRESENCE_INACTIVE, "inactive", Schema.XFAVERSION_30, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFASUBSET | Schema.XFAAVAILABILITY_XFAF) }, // radial { new EnumAttr(EnumAttr.TO_CENTER, "toCenter", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TO_EDGE, "toEdge", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // TO_* added with CL 30903 // relation for subform and subformSet { new EnumAttr(EnumAttr.RELATION_ORDERED, "ordered", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.RELATION_UNORDERED, "unordered", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.RELATION_CHOICE, "choice", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE) }, // RELATION_* added with CL 30903 // scriptModel { new EnumAttr(EnumAttr.SCRIPTMODEL_XFA, "XFA", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), // SCRIPTMODEL_* added with CL 38097 new EnumAttr(EnumAttr.SCRIPTMODEL_NONE, "none", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // SCRIPTMODEL_NONE with CL 68567 // select { new EnumAttr(EnumAttr.IMPLICITLINK, "implicitLink", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), // IMPLICITLINK, EMBED added with CL 30903 new EnumAttr(EnumAttr.EMBED, "embed", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.EMBEDSUBSET, "embedSubset", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // EMBEDSUBSET with CL 34986 // severity { new EnumAttr(EnumAttr.SEV_IGNORE, "ignore", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.SEV_TRACE, "trace", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.SEV_INFORMATION, "information", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.SEV_WARNING, "warning", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.SEV_ERROR, "error", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // SEV_* added with CL 34081 // shape { new EnumAttr(EnumAttr.SHAPE_SQUARE, "square", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.SHAPE_ROUND, "round", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // SHAPE_* added with CL 30903 // slope { new EnumAttr(EnumAttr.SLOPE_POSITIVE, "/", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.SLOPE_NEGATIVE, "\\", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // SLOPE_* added with CL 30903 // splitBias { new EnumAttr(EnumAttr.SPLITBIAS_HORIZONTAL, "horizontal", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.SPLITBIAS_VERTICAL, "vertical", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.SPLITBIAS_NONE, "none", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // SPLITBIAS_* added with CL 30903 // stroke { new EnumAttr(EnumAttr.STROKE_SOLID, "solid", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.STROKE_DASHED, "dashed", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.STROKE_DOTTED, "dotted", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.STROKE_LOWERED, "lowered", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.STROKE_RAISED, "raised", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.STROKE_ETCHED, "etched", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.STROKE_EMBOSSED, "embossed", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.STROKE_DASHDOT, "dashDot", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.STROKE_DASHDOTDOT, "dashDotDot", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) },// STROKE_* added with CL 30903 // styleType { new EnumAttr(EnumAttr.BITPATTERN, "bitPattern", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // BITPATTERN_* added with CL 30903 // subformBreak { new EnumAttr(EnumAttr.BREAK_AUTO, "auto", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.BREAK_CONTENTAREA, "contentArea", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.BREAK_PAGE, "pageArea", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.BREAK_PAGEEVEN, "pageEven", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.BREAK_PAGEODD, "pageOdd", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // BREAK_* added with CL 30903 // subformKeep { new EnumAttr(EnumAttr.KEEP_NONE, "none", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // KEEP_* added with CL 34939 new EnumAttr(EnumAttr.KEEP_CONTENTAREA, "contentArea", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.KEEP_PAGE, "pageArea", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // KEEP_CONTENTAREA with CL 30903 // submitFormat { new EnumAttr(EnumAttr.SUBMITFORMAT_HTML, "html", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.SUBMITFORMAT_FDF, "fdf", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.SUBMITFORMAT_XML, "xml", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.SUBMITFORMAT_PDF, "pdf", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // SUBMITFORMAT_* added with CL 38097 // test { new EnumAttr(EnumAttr.TEST_DISABLED, "disabled", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TEST_WARNING, "warning", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TEST_ERROR, "error", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // TEST_* added with CL 30903 // transferEncoding { new EnumAttr(EnumAttr.TRANSFER_NONE, "none", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), // TRANSFER_* added with CL 30903 new EnumAttr(EnumAttr.TRANSFER_BASE64, "base64", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.TRANSFER_PACKAGE, "package", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_ALL) }, // TRANSFER_PACKAGE with CL 349142 // trayIn { new EnumAttr(EnumAttr.TRAYIN_AUTO, "auto", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.TRAYIN_MANUAL, "delegate", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.TRAYIN_DELEGATE, "pageFront", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // TRAYIN_* added with CL 30903 // trayOut { new EnumAttr(EnumAttr.TRAYOUT_AUTO, "auto", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.TRAYOUT_DELEGATE, "delegate", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // TRAYOUT_* added with CL 30903 // unit { new EnumAttr(EnumAttr.POINT, "pt", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.INCH, "in", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.CENTIMETER, "cm", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.MILLIMETER, "mm", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.MILLIPOINT, "mp", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), // POINT INCH CENTIMETER MILLIMETER MILLIPOINT added with CL 30903 new EnumAttr(EnumAttr.PICA, "pc", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // unsign { new EnumAttr(EnumAttr.ANY, "any", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.SIGNER, "signer", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // ANY SIGNER added with CL 30903 // vAlign { new EnumAttr(EnumAttr.VALIGN_TOP, "top", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.VALIGN_MIDDLE, "middle", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.VALIGN_BOTTOM, "bottom", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // VALIGN_* added with CL 30903 // whitespace { new EnumAttr(EnumAttr.WHITESPACE_TRIM, "trim", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), // WHITESPACE_* added with CL 34691 new EnumAttr(EnumAttr.WHITESPACE_RTRIM, "rtrim", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WHITESPACE_LTRIM, "ltrim", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WHITESPACE_NORMALIZE, "normalize", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), // WHITESPACE_NORMALIZE with CL 34891 new EnumAttr(EnumAttr.WHITESPACE_PRESERVE, "preserve", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // WHITESPACE_PRESERVE with CL 34691 // placement { new EnumAttr(EnumAttr.PLACEMENT_TOP, "top", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.PLACEMENT_RIGHT, "right", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.PLACEMENT_BOTTOM, "bottom", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.PLACEMENT_LEFT, "left", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.PLACEMENT_INLINE, "inline", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) },// PLACEMENT_* added with CL 36733 // destination { new EnumAttr(EnumAttr.DESTINATION_NONE, "none", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.DESTINATION_PAGEFIT, "pageFit", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // DESTINATION_* added with CL 38714 // db { new EnumAttr(EnumAttr.DB_ADO, "ado", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.DB_HTTP, "http", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN) }, // DB_* added with CL 39739 // cmdType { new EnumAttr(EnumAttr.CMDTYPE_UNKNOWN, "unknown", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.CMDTYPE_TEXT, "text", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.CMDTYPE_TABLE, "table", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.CMDTYPE_STOREDPROC, "storedProc", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN) },// CMDTYPE_* added with CL 39739 // cursorType { new EnumAttr(EnumAttr.CURSORTYPE_UNSPECIFIED, "unspecified", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.CURSORTYPE_FORWARDONLY, "forwardOnly", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.CURSORTYPE_KEYSET, "keyset", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.CURSORTYPE_DYNAMIC, "dynamic", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.CURSORTYPE_STATIC, "static", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN) }, // CURSORTYPE_* added with CL 39739 // cursorLocation { new EnumAttr(EnumAttr.CURSORLOCATION_CLIENT, "client", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.CURSORLOCATION_SERVER, "server", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN) }, // CURSORLOCATION_* added with CL 39739 // lockType { new EnumAttr(EnumAttr.LOCKTYPE_UNSPECIFIED, "unspecified", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.LOCKTYPE_READONLY, "readOnly", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.LOCKTYPE_PESSIMISTIC, "pessimistic", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.LOCKTYPE_OPTIMISTIC, "optimistic", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.LOCKTYPE_BATCHOPTIMISTIC, "batchOptimistic", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN) }, // LOCKTYPE_* added with CL 39739 // incrementalLoad { new EnumAttr(EnumAttr.INCREMENTALLOAD_NONE, "none", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.INCREMENTALLOAD_FORWARDONLY, "forwardOnly", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // INCREMENTALLOAD_* added with CL 40149 // bofAction { new EnumAttr(EnumAttr.BOFACTION_MOVEFIRST, "moveFirst", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.BOFACTION_STAYBOF, "stayBOF", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN) }, // BOFACTION_* added with CL 40215 // eofAction { new EnumAttr(EnumAttr.EOFACTION_MOVELAST, "moveLast", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.EOFACTION_STAYEOF, "stayEOF", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.EOFACTION_ADDNEW, "addNew", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN) }, // EOFACTION_* added with CL 40215 // nodetype { new EnumAttr(EnumAttr.NODETYPE_ATTRIBUTE, "attribute", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.NODETYPE_ELEMENT, "element", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // NODETYPE_* added with CL 40262 // activity { new EnumAttr(EnumAttr.ACTIVITY_INITIALIZE, "initialize", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ACTIVITY_* added with CL 40275 new EnumAttr(EnumAttr.ACTIVITY_ENTER, "enter", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_EXIT, "exit", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_MOUSEENTER, "mouseEnter", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_MOUSEEXIT, "mouseExit", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_CHANGE, "change", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_CLICK, "click", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_PRESAVE, "preSave", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_POSTSAVE, "postSave", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_PREPRINT, "prePrint", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_POSTPRINT, "postPrint", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_READY, "ready", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ACTIVITY_READY with CL 53884 new EnumAttr(EnumAttr.ACTIVITY_DOCREADY, "docReady", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ACTIVITY_DOCREADY with CL 70769 new EnumAttr(EnumAttr.ACTIVITY_DOCCLOSE, "docClose", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_MOUSEUP, "mouseUp", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_MOUSEDOWN, "mouseDown", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_FULL, "full", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_PRESUBMIT, "preSubmit", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ACTIVITY_PRESUBMIT with CL 78826 new EnumAttr(EnumAttr.ACTIVITY_PREEXECUTE, "preExecute", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_POSTEXECUTE, "postExecute", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ACTIVITY_PREOPEN, "preOpen", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ACTIVITY_PREOPEN with CL 101960 new EnumAttr(EnumAttr.ACTIVITY_INDEXCHANGE, "indexChange", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ACTIVITY_INDEXCHANGE with CL 270697 new EnumAttr(EnumAttr.ACTIVITY_PRESIGN, "preSign", Schema.XFAVERSION_28, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ACTIVITY_PRESIGN with CL 411431 new EnumAttr(EnumAttr.ACTIVITY_POSTSIGN, "postSign", Schema.XFAVERSION_28, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ACTIVITY_POSTSIGN with CL 411431 new EnumAttr(EnumAttr.ACTIVITY_POSTSUBMIT, "postSubmit", Schema.XFAVERSION_28, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ACTIVITY_POSTSUBMIT with CL 413768 new EnumAttr(EnumAttr.ACTIVITY_POSTOPEN, "postOpen", Schema.XFAVERSION_28, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // ACTIVITY_POSTOPEN with CL 420442 new EnumAttr(EnumAttr.ACTIVITY_VALIDATIONSTATE, "validationState", Schema.XFAVERSION_30, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // format { new EnumAttr(EnumAttr.FORMAT_XFD, "xfd", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // FORMAT_* added with CL 40683 new EnumAttr(EnumAttr.FORMAT_XDP, "xdp", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.FORMAT_FORMDATA, "formdata", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // FORMAT_FORMDATA with CL 50044 new EnumAttr(EnumAttr.FORMAT_XML, "xml", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // FORMAT_XML with CL 40735 new EnumAttr(EnumAttr.FORMAT_PDF, "pdf", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // FORMAT_PDF with CL 50044 new EnumAttr(EnumAttr.FORMAT_URL, "urlencoded", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // FORMAT_URL with CL 196665 // nullType { new EnumAttr(EnumAttr.NULLTYPE_XSI, "xsi", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.NULLTYPE_EXCLUDE, "exclude", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.NULLTYPE_EMPTY, "empty", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // NULLTYPE_* added with CL 41540 // aspect { new EnumAttr(EnumAttr.ASPECT_NONE, "none", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ASPECT_FIT, "fit", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ASPECT_ACTUAL, "actual", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ASPECT_WIDTH, "width", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.ASPECT_HEIGHT, "height", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // ASPECT_* added with CL 51925 // outputtype { new EnumAttr(EnumAttr.OUTPUT_NATIVE, "native", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), // OUTPUT_* added with CL 54668 new EnumAttr(EnumAttr.OUTPUT_XDP, "xdp", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.OUTPUT_MERGEDXDP, "mergedXDP", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // OUTPUT_MERGEDXDP with CL 66189 // calendarSymbols { new EnumAttr(EnumAttr.CALENDAR, "gregorian", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL) }, // CALENDAR_* added with CL 57104 // datePattern & timePattern { new EnumAttr(EnumAttr.PATTERN_FULL, "full", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.PATTERN_LONG, "long", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.PATTERN_MED, "med", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.PATTERN_SHORT, "short", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL) }, // PATTERN_* added with CL 57104 // numberPattern { new EnumAttr(EnumAttr.PATTERN_NUMERIC, "numeric", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.PATTERN_CURRENCY, "currency", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.PATTERN_PERCENT, "percent", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL) }, // PATTERN_* added with CL 57104 // numericSymbol { new EnumAttr(EnumAttr.NUMERIC_DECIMAL, "decimal", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), // NUMERIC_* added with CL 57104 new EnumAttr(EnumAttr.NUMERIC_GROUPING, "grouping", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.NUMERIC_PERCENT, "percent", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.NUMERIC_MINUS, "minus", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.NUMERIC_ZERO, "zero", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL) }, // NUMERIC_ZERO with CL 133789 // currencySymbol { new EnumAttr(EnumAttr.CURRENCY_SYMBOL, "symbol", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.CURRENCY_ISONAME, "isoname", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.CURRENCY_DECIMAL, "decimal", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL) }, // CURRENCY_* added with CL 57104 // parity { new EnumAttr(EnumAttr.BARCODE_PARITY_NONE, "none", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.BARCODE_PARITY_ODD, "odd", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.BARCODE_PARITY_EVEN, "even", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // BARCODE_* added with CL 59830 // textLocation { new EnumAttr(EnumAttr.TEXTLOCATION_NONE, "none", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TEXTLOCATION_ABOVE, "above", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TEXTLOCATION_BELOW, "below", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TEXTLOCATION_ABOVEEMBEDDED, "aboveEmbedded", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.TEXTLOCATION_BELOWEMBEDDED, "belowEmbedded", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // TEXTLOCATION_* added with CL 59830 // checksum { new EnumAttr(EnumAttr.CHECKSUM_AUTO, "auto", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // CHECKSUM_* added with CL 67755 new EnumAttr(EnumAttr.CHECKSUM_NONE, "none", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.CHECKSUM_1MOD10, "1mod10", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // CHECKSUM_1MOD10 with CL 59830 new EnumAttr(EnumAttr.CHECKSUM_2MOD10, "2mod10", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // CHECKSUM_2MOD10 with CL 61716 new EnumAttr(EnumAttr.CHECKSUM_1MOD10_1MOD11, "1mod10_1mod11", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // CHECKSUM_1MOD10_1MOD11 with CL 59830 // signatureType { new EnumAttr(EnumAttr.PDF_13, "PDF1.3", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_PLUGIN), // PDF_* added with CL 62912 new EnumAttr(EnumAttr.PDF_16, "PDF1.6", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_PLUGIN) }, // PDF_16 with CL 262678 // border break { new EnumAttr(EnumAttr.BORDER_OPEN, "open", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.BORDER_CLOSED, "close", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // BORDER_* added with CL 63173 // support { new EnumAttr(EnumAttr.SUPPORT_NONE, "none", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.SUPPORT_HARDWARE, "hardware", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.SUPPORT_SOFTWARE, "software", Schema.XFAVERSION_OBS, Schema.XFAAVAILABILITY_ALL) }, // SUPPORT_* added with CL 63831 // scope { new EnumAttr(EnumAttr.SCOPE_NAME, "name", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.SCOPE_NONE, "none", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // SCOPE_* added with CL 65242 // xdclayout { new EnumAttr(EnumAttr.XDCLAYOUT_PAGINATE, "paginate", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.XDCLAYOUT_PANEL, "panel", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // XDCLAYOUT_* added with CL 66189 // runAt { new EnumAttr(EnumAttr.RUNAT_CLIENT, "client", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.RUNAT_SERVER, "server", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.RUNAT_BOTH, "both", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // RUNAT_* added with CL 66570 // priority { new EnumAttr(EnumAttr.PRIORITY_CUSTOM, "custom", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.PRIORITY_TOOLTIP, "toolTip", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.PRIORITY_CAPTION, "caption", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.PRIORITY_NAME, "name", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // PRIORITY_* added with CL 70412 // usage { new EnumAttr(EnumAttr.USAGE_EXPORTONLY, "exportOnly", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.USAGE_IMPORTONLY, "importOnly", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.USAGE_EXPORTANDIMPORT, "exportAndImport", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // USAGE_* added with CL 74309 // executeType { new EnumAttr(EnumAttr.EXECUTETYPE_IMPORT, "import", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.EXECUTETYPE_REMERGE, "remerge", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // EXECUTETYPE_* added with CL 78835 // data { new EnumAttr(EnumAttr.DATA_LINK, "link", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.DATA_EMBED, "embed", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // DATA_* added with CL 100875 // dataPrep { new EnumAttr(EnumAttr.DATAPREP_NONE, "none", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.DATAPREP_FLATECOMPRESS, "flateCompress", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // DATAPREP_* added with CL 125529 // signOperation { new EnumAttr(EnumAttr.SIGNOPERATION_SIGN, "sign", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.SIGNOPERATION_VERIFY, "verify", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.SIGNOPERATION_CLEAR, "clear", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_PLUGIN) }, // SIGNOPERATION_* added with CL 130260 // requirement { new EnumAttr(EnumAttr.REQUIREMENT_REQUIRED, "required", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.REQUIREMENT_OPTIONAL, "optional", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_PLUGIN) }, // REQUIREMENT_* added with CL 130260 // commitOn { new EnumAttr(EnumAttr.COMMITON_EXIT, "exit", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.COMMITON_SELECT, "select", Schema.XFAVERSION_22, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // COMMITON_* added with CL 129344 // dynamicRender { new EnumAttr(EnumAttr.DYNAMICRENDER_FORBIDDEN, "forbidden", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.DYNAMICRENDER_REQUIRED, "required", Schema.XFAVERSION_22, Schema.XFAAVAILABILITY_CORE) }, // DYNAMICRENDER_* added with CL 140213 // batchFormat { new EnumAttr(EnumAttr.BATCHFORMAT_NONE, "none", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.BATCHFORMAT_ZIP, "zip", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.BATCHFORMAT_ZIPCOMPRESS, "zipCompress", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.BATCHFORMAT_CONCAT, "concat", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE) }, // BATCHFORMAT_* added with CL 192754 // renderPolicy { new EnumAttr(EnumAttr.RENDERPOLICY_SERVER, "server", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.RENDERPOLICY_CLIENT, "client", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE) }, // RENDERPOLICY_* added with CL 193630 // writingScript { new EnumAttr(EnumAttr.WRITINGSCRIPT_STAR, "*", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_ROMAN, "Roman", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_EASTEUROPEANROMAN, "EastEuropeanRoman", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_GREEK, "Greek", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_CYRILLIC, "Cyrillic", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_JAPANESE, "Japanese", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_TRADITIONALCHINESE, "TraditionalChinese", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_SIMPLIFIEDCHINESE, "SimplifiedChinese", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_KOREAN, "Korean", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_ARABIC, "Arabic", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_HEBREW, "Hebrew", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_THAI, "Thai", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.WRITINGSCRIPT_VIETNAMESE, "Vietnamese", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE) }, // WRITINGSCRIPT_* added with CL 204496 // taggedMode { new EnumAttr(EnumAttr.TAGGEDMODE_NORMAL, "normal", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.TAGGEDMODE_CUSTOM, "custom", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE) }, // TAGGEDMODE_* added with CL 212605 // runScripts { new EnumAttr(EnumAttr.RUNSCRIPTS_CLIENT, "client", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.RUNSCRIPTS_SERVER, "server", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.RUNSCRIPTS_BOTH, "both", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.RUNSCRIPTS_NONE, "none", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE) }, // RUNSCRIPTS_* added with CL 66189 // manifestAction { new EnumAttr(EnumAttr.MANIFESTACTION_INCLUDE, "include", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), // MANIFESTACTION_* added with CL 262678 new EnumAttr(EnumAttr.MANIFESTACTION_EXCLUDE, "exclude", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.MANIFESTACTION_ALL, "all", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF | Schema.XFAAVAILABILITY_PLUGIN) }, // MANIFESTACTION_ALL with CL 284307 // mdpSignatureType { new EnumAttr(EnumAttr.MDPSIGNATURETYPE_FILLER, "filler", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.MDPSIGNATURETYPE_AUTHOR, "author", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_PLUGIN) }, // MDPSIGNATURETYPE_* added with CL 262678 // mdpPermissions { new EnumAttr(EnumAttr.MDPPERMISSIONS_ONE, "1", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.MDPPERMISSIONS_TWO, "2", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.MDPPERMISSIONS_THREE, "3", Schema.XFAVERSION_24, Schema.XFAAVAILABILITY_PLUGIN) }, // MDPPERMISSIONS_* added with CL 262678 // pageSetRelation { new EnumAttr(EnumAttr.PAGESETRELATION_ORDERED_OCCURRENCE, "orderedOccurrence", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGESETRELATION_SIMPLEX_PAGINATED, "simplexPaginated", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGESETRELATION_DUPLEX_PAGINATED, "duplexPaginated", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE) },// PAGESET_* added with CL 271273 // pagePosition { new EnumAttr(EnumAttr.PAGEPOSITION_FIRST, "first", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGEPOSITION_LAST, "last", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGEPOSITION_REST, "rest", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGEPOSITION_ONLY, "only", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGEPOSITION_ANY, "any", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE) }, // PAGEPOSITION_* added with CL 271273 // oddOrEven { new EnumAttr(EnumAttr.ODDOREVEN_ODD, "odd", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.ODDOREVEN_EVEN, "even", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.ODDOREVEN_ANY, "any", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE) }, // ODDOREVEN_* added with CL 271273 // blankOrNotBlank { new EnumAttr(EnumAttr.BLANKORNOTBLANK_BLANK, "blank", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.BLANKORNOTBLANK_NOTBLANK, "notBlank", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.BLANKORNOTBLANK_ANY, "any", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE) }, // BLANKORNOTBLANK_* added with CL 271273 // vScrollPolicy & hScrollPolicy { new EnumAttr(EnumAttr.SCROLLPOLICY_AUTO, "auto", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_PLUGIN), // SCROLLPOLICY_* added with CL 272860 new EnumAttr(EnumAttr.SCROLLPOLICY_ON, "on", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.SCROLLPOLICY_OFF, "off", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_PLUGIN) }, // SCROLLPOLICY_OFF with CL 275268 // baseProfile { new EnumAttr(EnumAttr.BASEPROFILE_FULL, "full", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.BASEPROFILE_INTERACTIVEFORMS, "interactiveForms", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // BASEPROFILE_* added with CL 289148 // duplexOption { new EnumAttr(EnumAttr.DUPLEXOPTION_SIMPLEX, "simplex", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.DUPLEXOPTION_DUPLEXFLIPSHORTEDGE, "duplexFlipShortEdge", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.DUPLEXOPTION_DUPLEXFLIPLONGEDGE, "duplexFlipLongEdge", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE) },// DUPLEXOPTION_* added with CL 289690 // checkbutton.mark { new EnumAttr(EnumAttr.MARK_CHECK, "check", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), // MARK_* added with CL 290364 new EnumAttr(EnumAttr.MARK_CIRCLE, "circle", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MARK_CROSS, "cross", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MARK_DIAMOND, "diamond", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MARK_SQUARE, "square", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MARK_STAR, "star", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.MARK_DEFAULT, "default", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // MARK_DEFAULT with CL 298010 // button.highlight { new EnumAttr(EnumAttr.HIGHLIGHT_NONE, "none", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.HIGHLIGHT_INVERTED, "inverted", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.HIGHLIGHT_PUSH, "push", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.HIGHLIGHT_OUTLINE, "outline", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_PLUGIN) }, // HIGHLIGHT_* added with CL 290364 // restoreState { new EnumAttr(EnumAttr.RESTORESTATE_MANUAL, "manual", Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.RESTORESTATE_AUTO, "auto", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // RESTORESTATE_* added with CL 291994 // pagination { new EnumAttr(EnumAttr.PAGINATION_SIMPLEX, "simplex", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGINATION_DUPLEX_LONG_EDGE, "duplexLongEdge", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGINATION_DUPLEX_SHORT_EDGE, "duplexShortEdge", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE) }, // PAGINATION_* added with CL 296209 // jog { new EnumAttr(EnumAttr.JOG_USEPRINTERSETTING, STRS.USEPRINTERSETTING, Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.JOG_NONE, "none", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.JOG_PAGESET, "pageSet", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE) }, // JOG_* added with CL 343014 // addRevocationInfo { new EnumAttr(EnumAttr.ADDREVOCATIONINFO_REQUIRED, "required", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.ADDREVOCATIONINFO_OPTIONAL, "optional", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_PLUGIN), new EnumAttr(EnumAttr.ADDREVOCATIONINFO_NONE, "none", Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_PLUGIN) }, // ADDREVOCATIONINFO_* added with CL 315612 // upsMode { new EnumAttr(EnumAttr.UPSMODE_USCARRIER, "usCarrier", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.UPSMODE_INTERNATIONALCARRIER, "internationalCarrier", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.UPSMODE_STANDARDSYMBOL, "standardSymbol", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF), new EnumAttr(EnumAttr.UPSMODE_SECURESYMBOL, "secureSymbol", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE | Schema.XFAAVAILABILITY_XFAF) }, // UPSMODE_* added with CL 334068 // sourceBelow { new EnumAttr(EnumAttr.SOURCEBELOW_UPDATE, "update", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.SOURCEBELOW_MAINTAIN, "maintain", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // SOURCEBELOW_* added with CL 335230 // sourceAbove { new EnumAttr(EnumAttr.SOURCEABOVE_WARN, "warn", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.SOURCEABOVE_ERROR, "error", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // SOURCEABOVE_* added with CL 335230 // outputBelow { new EnumAttr(EnumAttr.OUTPUTBELOW_UPDATE, "update", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.OUTPUTBELOW_WARN, "warn", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.OUTPUTBELOW_ERROR, "error", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_CORE) }, // OUTPUTBELOW_* added with CL 335230 // compressScope { new EnumAttr(EnumAttr.COMPRESSSCOPE_IMAGEONLY, "imageOnly", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.COMPRESSSCOPE_DOCUMENT, "document", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE) }, // COMPRESSSCOPE_* added with CL 340535 // stapleMode { new EnumAttr(EnumAttr.STAPLEMODE_ON, "on", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.STAPLEMODE_OFF, "off", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.STAPLEMODE_USEPRINTERSETTING, STRS.USEPRINTERSETTING, Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE) },// STAPLEMODE_* added with CL 343014 // pfdaConformance { new EnumAttr(EnumAttr.PDFACONFORMANCE_A, "A", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PDFACONFORMANCE_B, "B", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE) }, // PDFACONFORMANCE_* added with CL 343791 // overprint { new EnumAttr(EnumAttr.OVERPRINT_NONE, "none", Schema.XFAVERSION_10 /* default behavior of 1.0 docs */, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.OVERPRINT_DRAWS, "draws", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.OVERPRINT_FIELDS, "fields", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.OVERPRINT_DRAWSANDFIELDS, "drawsAndFields", Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_CORE) }, // OVERPRINT_* added with CL 356195 // genericFamily { new EnumAttr(EnumAttr.GENERICFAMILY_SANSSERIF, "sansSerif", Schema.XFAVERSION_27, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.GENERICFAMILY_SERIF, "serif", Schema.XFAVERSION_27, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.GENERICFAMILY_CURSIVE, "cursive", Schema.XFAVERSION_27, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.GENERICFAMILY_FANTASY, "fantasy", Schema.XFAVERSION_27, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.GENERICFAMILY_MONOSPACE, "monospace", Schema.XFAVERSION_27, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.GENERICFAMILY_UNKNOWN, "unknown", Schema.XFAVERSION_10 /* default behavior of 1.0 docs*/, Schema.XFAAVAILABILITY_CORE) }, // GENERICFAMILY_* added with CL 349042 // kerningMode { new EnumAttr(EnumAttr.KERNINGMODE_NONE, "none", Schema.XFAVERSION_21 /* default behavior of 2.1 templates */, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.KERNINGMODE_PAIR, "pair", Schema.XFAVERSION_28, Schema.XFAAVAILABILITY_CORE) }, // picker { new EnumAttr(EnumAttr.PICKER_NONE, "none", Schema.XFAVERSION_21 /* default behavior of 2.1 templates */, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PICKER_HOST, "host", Schema.XFAVERSION_28, Schema.XFAAVAILABILITY_CORE) }, // paginationOverride { new EnumAttr(EnumAttr.PAGINATIONOVERRIDE_NONE, "none", Schema.XFAVERSION_10 /* legacy behavior */, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGINATIONOVERRIDE_FORCE_SIMPLEX, "forceSimplex", Schema.XFAVERSION_27, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGINATIONOVERRIDE_FORCE_DUPLEX, "forceDuplex", Schema.XFAVERSION_27, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGINATIONOVERRIDE_FORCE_DUPLEX_LONG_EDGE, "forceDuplexLongEdge", Schema.XFAVERSION_27, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PAGINATIONOVERRIDE_FORCE_DUPLEX_SHORT_EDGE, "forceDuplexShortEdge", Schema.XFAVERSION_27, Schema.XFAAVAILABILITY_CORE) }, // validationMessaging { new EnumAttr(EnumAttr.VALIDATIONMESSAGING_NOMESSAGES, "noMessages", Schema.XFAVERSION_30, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.VALIDATIONMESSAGING_FIRSTMESSAGEONLY, "firstMessageOnly", Schema.XFAVERSION_30, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.VALIDATIONMESSAGING_ALLMESSAGESINDIVIDUALLY, "allMessagesIndividually", Schema.XFAVERSION_21 /* default behavior of 2.1 templates */, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.VALIDATIONMESSAGING_ALLMESSAGESTOGETHER, "allMessagesTogether", Schema.XFAVERSION_30, Schema.XFAAVAILABILITY_CORE) }, // listen { new EnumAttr(EnumAttr.EVENTLISTEN_REFONLY, "refOnly", Schema.XFAVERSION_21 /* default behavior of 2.1 templates */, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.EVENTLISTEN_REFANDDESCENDENTS, "refAndDescendents", Schema.XFAVERSION_30, Schema.XFAAVAILABILITY_CORE) }, // printScaling { new EnumAttr(EnumAttr.PRINTSCALING_NOSCALING, "noScaling", Schema.XFAVERSION_30, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.PRINTSCALING_APPDEFAULT, "appDefault", Schema.XFAVERSION_10 /* legacy behavior */, Schema.XFAAVAILABILITY_CORE) }, // duplexImposition { new EnumAttr(EnumAttr.DUPLEXIMPOSITION_LONGEDGE, "longEdge", Schema.XFAVERSION_21 /* legacy behavior */, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.DUPLEXIMPOSITION_SHORTEDGE, "shortEdge", Schema.XFAVERSION_31, Schema.XFAAVAILABILITY_CORE) }, // DUPLEXIMPOSITION_* added with CL 547714 // mergeMode { new EnumAttr(EnumAttr.MERGEMODE_CONSUMEDATA, "consumeData", Schema.XFAVERSION_21 /*legacy behaviour*/, Schema.XFAAVAILABILITY_CORE), new EnumAttr(EnumAttr.MERGEMODE_MATCHTEMPLATE, "matchTemplate", Schema.XFAVERSION_31, Schema.XFAAVAILABILITY_ALL) }, // credentialServerPolicy { new EnumAttr(EnumAttr.CREDENTIAL_REQUIRED, "required", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL), new EnumAttr(EnumAttr.CREDENTIAL_OPTIONAL, "optional", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // CREDENTIAL_* added for JAVAPORT // submit { new EnumAttr(EnumAttr.UNDEFINED, "", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // UNDEFINED sentinel added for JAVAPORT // batchoutput { new EnumAttr(EnumAttr.UNDEFINED, "", Schema.XFAVERSION_10, Schema.XFAAVAILABILITY_ALL) }, // UNDEFINED sentinel added for JAVAPORT // typeface { new EnumAttr(EnumAttr.UNDEFINED, "", Schema.XFAVERSION_27, Schema.XFAAVAILABILITY_ALL) } // UNDEFINED sentinel added for JAVAPORT }; /** * @exclude from published api. */ public static final int ENUM_TYPE_MAX = EnumType.TYPE_MAX; /** * @exclude from published api. */ public static final int ENUM_WIDEST = 21; // The most values you'll find // in the largest group + 1 /** * return the enumerated type for this enum. * @return the EnumAttr::TYPE value for this class. * * @exclude from published api. */ public EnumType getType() { return EnumType.getEnum(mNum); } /** * Determines if two EnumAttr instances are equal. * Since we should only ever create a single instance of each EnumAttr value, * it should be possible to use reference comparisons. This implementation * relies on each EnumAttr having a unique integer value. * @returntrue
if equal. * * @exclude from published api. */ public boolean equals(Object object) { if (this == object) return true; // This overrides Object.equals(boolean) directly, so... if (object == null) return false; if (object.getClass() != getClass()) return false; EnumAttr compare = (EnumAttr) object; return compare.getInt() == getInt(); } /** * Returns a hash code value for the object. * @exclude from published api. */ public int hashCode() { return getInt(); } /** * Get the string that corresponds to this enumerated value. * @param eVal * the EnumAttr::VALUE to find a string for * * @exclude from published api. */ public static String getString(int eVal) { return getEnum(eVal).toString(); } /** * * {@link Attribute#newAttribute(String)} * * @exclude from published api. */ public EnumAttr newEnum(String value) { if (value.length() == 0) { EnumAttr oRet = getEnum(EnumType.getEnum(EnumAttr.UNDEFINED), ""); return oRet; } return getEnum(getType(), value); } /** * @exclude from published api. */ public String toString() { return mName; } }
© 2015 - 2024 Weber Informatics LLC | Privacy Policy