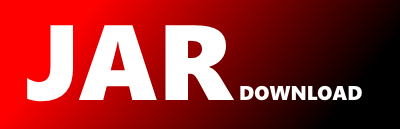
com.adobe.xfa.EnumValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa;
import com.adobe.xfa.ut.Assertions;
import com.adobe.xfa.ut.FindBugsSuppress;
import com.adobe.xfa.ut.StringUtils;
/**
* This class represents an XFA attribute whose value is one of an enumerated set.
* The contents of this class are an attribute name and enumerated value (EnumAttr) combination.
* This class ensures that there is only one canonical representation of each attribute/value combination.
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public final class EnumValue extends Attribute {
/**
* The Enumerated attribute value
*/
private final EnumAttr mValue;
/**
* The tag of the attribute holding this value.
*/
private final int meAttr;
/**
* A list of cases where we re-used the same attribute name with different
* meanings. Since the same attribute tag can have more than one enumeration,
* we look up in this secondary table to resolve the ambiguity. Duplicated
* pairs have been commented out for efficiency but retained for compleness.
*/
private static final int mAmbiguousTypes[][] = new int[][] {
{XFA.TYPETAG, EnumType.LINEAR}, // XFA.LINEARTAG
{XFA.TYPETAG, EnumType.PATTERN}, // XFA.PATTERNTAG
{XFA.TYPETAG, EnumType.RADIAL}, // XFA.RADIALTAG
{XFA.TYPETAG, EnumType.REQUIREMENT}, // XFA.HANDLERTAG
// {XFA.TYPETAG, EnumType.REQUIREMENT}, // XFA.DIGESTMETHODSTAG
// {XFA.TYPETAG, EnumType.REQUIREMENT}, // XFA.ENCODINGSTAG
// {XFA.TYPETAG, EnumType.REQUIREMENT}, // XFA.ISSUERSTAG
// {XFA.TYPETAG, EnumType.REQUIREMENT}, // XFA.KEYUSAGETAG
// {XFA.TYPETAG, EnumType.REQUIREMENT}, // XFA.OIDSTAG
// {XFA.TYPETAG, EnumType.REQUIREMENT}, // XFA.REASONSTAG
// {XFA.TYPETAG, EnumType.REQUIREMENT}, // XFA.SIGNINGTAG
// {XFA.TYPETAG, EnumType.REQUIREMENT}, // XFA.SUBJECTDNSTAG
// {XFA.TYPETAG, EnumType.REQUIREMENT}, // XFA.TIMESTAMPTAG
{XFA.TYPETAG, EnumType.SIGNATURETYPE}, // XFA.SIGNATURETAG
{XFA.OPERATIONTAG, EnumType.SIGNOPERATION}, // XFA.SIGNDATATAG
{XFA.OPERATIONTAG, EnumType.OPERATION}, // XFA.TRAVERSETAG
{XFA.OVERRIDETAG, EnumType.OVERRIDE}, // XFA.CALCULATETAG
{XFA.OVERRIDETAG, EnumType.BOOLEAN}, // XFA.VALUETAG
{XFA.TOTAG, EnumType.LOGTO}, // XFA.LOGTAG
{XFA.TOTAG, EnumType.OUTPUTTO}, // XFA.OUTPUTTAG
{XFA.FORMATTAG, EnumType.FORMAT}, // XFA.SUBMITTAG
{XFA.FORMATTAG, EnumType.BATCHOUTPUT}, // XFA.BATCHOUTPUTTAG
{XFA.RELATIONTAG, EnumType.RELATION}, // XFA.PAGESETTAG
{XFA.RELATIONTAG, EnumType.PAGESETRELATION}, // XFA.SUBFORMSETTAG
{XFA.NAMETAG, EnumType.CALENDARSYMBOLS}, // XFA.CALENDARSYMBOLSTAG
{XFA.NAMETAG, EnumType.DATETIMESYMBOLS}, // XFA.DATEPATTERNTAG
// {XFA.NAMETAG, EnumType.DATETIMESYMBOLS}, // XFA.TIMEPATTERNTAG
{XFA.NAMETAG, EnumType.NUMBERPATTERN}, // XFA.NUMBERPATTERNTAG
{XFA.NAMETAG, EnumType.NUMBERSYMBOL}, // XFA.NUMBERSYMBOLTAG
{XFA.NAMETAG, EnumType.TYPEFACE}, // XFA.TYPEFACETAG
{XFA.NAMETAG, EnumType.CURRENCYSYMBOL} // XFA.CURRENCYSYMBOLTAG
};
/**
* A list of config tags whose values are attribute values,
* i.e., config elements with a single text node child whose's
* value is an EnumType.
* We look up in this secondary table to resolve this feature.
*/
private static final int mConfigTypes[][] = new int[][] {
{XFA.ACCESSIBLECONTENTTAG, EnumType.BOOLEAN},
{XFA.ADDSILENTPRINTTAG, EnumType.BOOLEAN},
{XFA.ADDVIEWERPREFERENCESTAG, EnumType.BOOLEAN},
{XFA.ADJUSTDATATAG, EnumType.BOOLEAN},
{XFA.ATTRIBUTESTAG, EnumType.ATTRIBUTES},
{XFA.CHANGETAG, EnumType.BOOLEAN},
{XFA.COMPRESSLOGICALSTRUCTURETAG, EnumType.BOOLEAN},
{XFA.COMPRESSOBJECTSTREAMTAG, EnumType.BOOLEAN},
{XFA.CONFORMANCETAG, EnumType.PDFACONFORMANCE},
{XFA.CONTENTCOPYTAG, EnumType.BOOLEAN},
{XFA.DESTINATIONTAG, EnumType.DESTINATION},
{XFA.DOCUMENTASSEMBLYTAG, EnumType.BOOLEAN},
{XFA.DUPLEXOPTIONTAG, EnumType.DUPLEXOPTION},
{XFA.DYNAMICRENDERTAG, EnumType.DYNAMICRENDER},
{XFA.EMBEDRENDEREDOUTPUTTAG, EnumType.BOOLEAN},
{XFA.EMBEDTAG, EnumType.BOOLEAN},
{XFA.ENABLETAG, EnumType.BOOLEAN},
{XFA.ENCRYPTIONLEVELTAG, EnumType.ENCRYPTIONLEVEL},
{XFA.ENCRYPTTAG, EnumType.BOOLEAN},
{XFA.FLIPLABELTAG, EnumType.BOOLEAN},
{XFA.FORMFIELDFILLINGTAG, EnumType.BOOLEAN},
{XFA.IFEMPTYTAG, EnumType.IFEMPTY},
{XFA.INTERACTIVETAG, EnumType.BOOLEAN},
{XFA.INCREMENTALLOADTAG, EnumType.INCREMENTALLOAD},
{XFA.JOGTAG, EnumType.JOGOPTION},
{XFA.LAYOUTTAG, EnumType.XDCLAYOUT},
{XFA.LINEARIZEDTAG, EnumType.BOOLEAN},
{XFA.LOCKTAG, EnumType.BOOLEAN},
{XFA.MODETAG, EnumType.MODE},
{XFA.OVERPRINTTAG, EnumType.OVERPRINT},
{XFA.PAGINATIONTAG, EnumType.PAGINATION},
{XFA.PAGINATIONOVERRIDETAG, EnumType.PAGINATIONOVERRIDE},
{XFA.PLAINTEXTMETADATATAG, EnumType.BOOLEAN},
{XFA.PRESENCETAG, EnumType.PRESENCE},
{XFA.PRINTTAG, EnumType.BOOLEAN},
{XFA.RENDERPOLICYTAG, EnumType.RENDERPOLICY},
{XFA.RUNSCRIPTSTAG, EnumType.RUNSCRIPTS},
{XFA.SCRIPTMODELTAG, EnumType.SCRIPTMODEL},
{XFA.SEVERITYTAG, EnumType.SEVERITY},
{XFA.SUBMITFORMATTAG, EnumType.SUBMITFORMAT},
{XFA.SUPPRESSBANNERTAG, EnumType.BOOLEAN},
{XFA.TAGGEDMODETAG, EnumType.TAGGEDMODE},
{XFA.TAGGEDTAG, EnumType.BOOLEAN},
{XFA.THRESHOLDTAG, EnumType.MESSAGETYPE},
{XFA.TOTAG, EnumType.OUTPUTTO},
{XFA.TOTAG, EnumType.LOGTO},
{XFA.TYPETAG, EnumType.OUTPUTTYPE},
{XFA.VALIDATETAG, EnumType.BOOLEAN},
{XFA.WHITESPACETAG, EnumType.WHITESPACE},
{XFA.VALIDATIONMESSAGINGTAG, EnumType.VALIDATIONMESSAGING},
{XFA.PRINTSCALINGTAG, EnumType.PRINTSCALING},
{XFA.PICKTRAYBYPDFSIZETAG, EnumType.BOOLEAN}
};
/**
* Arrays of attribute values, indexed by the attribute tag -- except in cases
* where the tag is used ambiguously, in which case we switch to the mAmbiguousAttrs table
*/
static private final EnumValue mAllEnumAttrs[][] = new EnumValue[XFA.XFA_ATTR_COUNT][];
/**
* Table of Ambiguous attribute values.
*/
static private final EnumValue mAmbiguousAttrs[][] = new EnumValue[mAmbiguousTypes.length][];
/**
* Table of Config property/attribute values.
*/
static private final EnumValue mConfigAttrs[][] = new EnumValue[mConfigTypes.length][];
/**
* Constructor that supplies all possible member values.
* @param a - The EnumAttr attribute value to use.
* @param NS - namespace. This String must be interned.
* @param localName - local name. This String must be interned.
* @param qName - qualified name. This String must be interned.
* @param value - attribute value. This String must be interned.
*/
@FindBugsSuppress(code="ES")
private EnumValue(EnumType enumType, int eAttrTag, String NS, String localName, String qName, String value) {
super(NS, localName, qName, value, false);
if (Assertions.isEnabled) assert (value == value.intern());
mValue = EnumAttr.getEnum(enumType, getAttrValue());
meAttr = eAttrTag;
}
// /**
// * Create an EnumValue based on it's attribute name and value
// * @param name - The attribute name
// * @param value - the attribute value
// */
// public EnumValue(String name, EnumAttr value) {
// super(null, name, name, value.toString());
// mValue = value;
// }
/**
* Get the attribute value of the stored enum
* @return the integer enumerated value
*/
public EnumAttr getAttr() {
return mValue;
}
/**
* Get the integer value of the stored enum
* @return the integer enumerated value
*/
public int getInt() {
return mValue.getInt();
}
/**
* Get the type of the stored enum
* @return the enumerated type.
*/
public EnumType getType() {
return mValue.getType();
}
/* (non-Javadoc)
* @see Attribute#newAttribute(String)
*/
public Attribute newAttribute(String value) {
return newAttribute(null, null, null, value, true);
}
/* (non-Javadoc)
* @see Attribute#newAttribute(String, String, String, String)
*/
public Attribute newAttribute(String NS, String localName, String qName, String value) {
return newAttribute(NS, localName, qName, value, true);
}
/* (non-Javadoc)
* @see Attribute#newAttribute(String, String, String, String, boolean)
*/
public Attribute newAttribute(String NS, String localName, String qName, String value, boolean internSymbols) {
if (StringUtils.isEmpty(value))
return this;
// Normally, we only consider the NS, localName and qName parameters to be symbols,
// but for an enum, the value is a symbol. If internSymbols is false, we expect the caller
// to have interned the value parameter as well.
if (internSymbols) {
value = value.intern();
}
return getEnum(mValue.getType(), meAttr, value);
}
/**
* get the tag that corresponds to this enum value.
* @return the attribute tag.
*/
public int getAttrTag() {
return meAttr;
}
/**
* find a canonical EnumValue given the type, tag and a string value.
* @param enumType - The type of enumeration
* @param eAttrTag - The attribute tag
* @param value - a string representation of the value. This String must be interned.
* @return The canonical attribute
*/
private static EnumValue getEnum(EnumType enumType, int eAttrTag, String value) {
EnumAttr a = EnumAttr.getEnum(enumType, value);
return getEnum(eAttrTag, a);
}
/**
* get a canonical instance of an attribute, given an attribute tag and value.
* @param eAttrTag - the attribute tag
* @param value - the enumerated value
* @return the canonical attribute
*/
public static EnumValue getEnum(int eAttrTag, EnumAttr value) {
EnumType enumType = value.getType();
int eType = enumType.getInt();
int index = -1;
EnumValue[] vals = null;
// If ambiguous! e.g. multiple attributes named "type", then
if (enumType.usedAmbiguously()) {
// Find the offset into the ambiguous types table.
for (index = 0; index < mAmbiguousTypes.length; index++) {
if (mAmbiguousTypes[index][0] == eAttrTag &&
mAmbiguousTypes[index][1] == eType) {
break;
}
}
}
// Else if a conFig property then
else if (enumType.usedElementarily()) {
// Find the offset into the config types table.
for (index = 0; index < mConfigTypes.length; index++) {
if (mConfigTypes[index][0] == eAttrTag &&
mConfigTypes[index][1] == eType) {
break;
}
}
if (index == mConfigTypes.length)
index = -1;
}
// Else use attribute tag to index into the main table.
if (index == -1) {
assert (eAttrTag > XFA.XFA_ELEMENTS+2);
vals = mAllEnumAttrs[eAttrTag-(XFA.XFA_ELEMENTS+2)];
if (vals == null) {
synchronized (mAllEnumAttrs) {
vals = mAllEnumAttrs[eAttrTag-(XFA.XFA_ELEMENTS+2)];
if (vals == null) {
vals = new EnumValue[value.getValues().length];
mAllEnumAttrs[eAttrTag-(XFA.XFA_ELEMENTS+2)] = vals;
}
}
}
}
else if (enumType.usedAmbiguously()) {
// index into the alternate table.
vals = mAmbiguousAttrs[index];
if (vals == null) {
synchronized (mAmbiguousAttrs) {
vals = mAmbiguousAttrs[index];
if (vals == null) {
vals = new EnumValue[value.getValues().length];
mAmbiguousAttrs[index] = vals;
}
}
}
}
else {
// index into the alternate table.
vals = mConfigAttrs[index];
if (vals == null) {
synchronized (mConfigAttrs) {
vals = mConfigAttrs[index];
if (vals == null) {
vals = new EnumValue[value.getValues().length];
mConfigAttrs[index] = vals;
}
}
}
}
int offset = value.getInt() & EnumAttr.ENUM_VALUE_MASK;
EnumValue v = vals[offset];
if (v == null) {
synchronized (vals) {
v = vals[offset];
if (v == null) {
String name = XFA.getString(eAttrTag);
v = new EnumValue(enumType, eAttrTag, null, name, name, value.toString());
vals[offset] = v;
}
}
}
return v;
}
/**
* Get a canonical instance of an attribute, given an attribute tag and an enumerated value.
* @param eAttrTag - the attribute tag
* @param value - the enumerated value from EnumAttr
* @return the canonical attribute
*/
public static EnumValue getEnum(int eAttrTag, int value) {
return getEnum(eAttrTag, EnumAttr.getEnum(value));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy