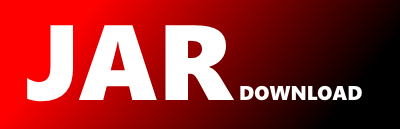
com.adobe.xfa.ScriptDebugger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa;
import java.util.ArrayList;
import java.util.List;
/**
* An ScriptDebugger provides script debugging access to an ScriptHandler.
*
* @author Darren Burns
*
* @exclude from published api for now.
*/
public class ScriptDebugger {
private final List moScriptHandlers = new ArrayList();
/**
* Provide a unique script ID for the specified script, given its
* context information. It's up the the derived class to look up
* and possibly create an identifier. The value has no particular
* meaning to the debugging framework. Typically the derived class
* will maintain a table, and the ID is the index into that table.
*
* @param oScriptHandler the script handler of the script.
* @param sScriptContents the executable code of the script.
* @param oContextNode the Node that is the current node when the script
* runs.
* @param eReason the reason for the execution of the script.
*
* @return the ID for the script.
*/
public int getScriptID(ScriptHandler oScriptHandler,
String sScriptContents,
Node oContextNode,
int eReason) {
return -1;
}
/**
* For remote debugging. To support remote debugging (i.e. debugging
* an application in another executable) the derived class must override
* this method. It's identical to the other version of getScriptID
* except that the context node is provided as a SOM expression instead
* of as an Node.
*/
public int getScriptID(ScriptHandler oScriptHandler,
String sScriptContents,
String sContextNodeSOM,
int eReason) {
return -1;
}
/**
* Signals that the specified script is about to execute. At this point
* the derived class can optionally display the script. If breakpoints
* have been set for this script ID, then the derived class must
* re-establish them by calling ScriptHandler::debugBreakPoint. The
* reason for this is that the script handler doesn't have the big
* picture -- it simply executes a script when told to do so.
*
* @param nScriptID the unique identifier of the script.
*/
public void willExecuteScript(int nScriptID) {
}
/**
* Signals that the specified script has finished executing. The return
* value is provided. The derived class may choose to ignore this, or
* display the result to the user.
*
* @param nScriptID the unique identifier of the script.
* @param oReturnValue the return value of the script.
*/
public void didExecuteScript(int nScriptID, Arg oReturnValue) {
}
/**
* Signals that the specified script has stopped momentarily. Either
* a breakpoint or single-stepping through code can cause this. The
* derived class should display the script at the specified line.
*
* @param nScriptID the unique identifier of the script.
* @param nLine the current execution line of the script.
*/
public void stopped(int nScriptID, int nLine) {
}
/**
* Called to indicate that a script handler resolved a value internally.
* The debugger can regard these as a form of trace message.
*
* @param sValueName the name of the value that was resolved.
* @param oRetrievedValue the resultant value.
*/
public void resolvedValue(String sValueName, Arg oRetrievedValue) {
}
/**
* The poll() method is periodically called during the execution
* of a script.
*
* @param oScriptHandler the script handler that is active.
*/
public void poll(ScriptHandler oScriptHandler) {
}
/**
* Determine if this debugger is currently active (in use). If the
* debugger is not displayed or in use it can return false here.
* This may make certain operations more efficient.
*
* @return true if active, false otherwise.
*/
boolean isActive() {
return true;
}
/**
* Signals that the specified script handler is no longer associated
* with this debugger.
*
* Warning: in detach(), the ScriptHandler may be in its destructor,
* so calling any virtual functions on oScriptHandler can result in
* a pure virtual function call error. The only valid use of this
* pointer is to compare to a stored ScriptHandler pointer.
*
* @param oScriptHandler the script handler that is detaching from
* the debugger.
*/
void detach(ScriptHandler oScriptHandler) {
}
protected void private_attach(ScriptHandler oScriptHandler) {
assert (oScriptHandler != null);
moScriptHandlers.add(oScriptHandler);
}
protected void private_detach(ScriptHandler oScriptHandler) {
assert (oScriptHandler != null);
detach(oScriptHandler); // call derived function
//
// Clear out any reference to the specified script handler,
// and null out its pointer to us.
//
for (int i = 0; i < moScriptHandlers.size(); i++) {
assert (moScriptHandlers.get(i) != null);
ScriptHandler oHandler = moScriptHandlers.get(i);
if (oHandler == oScriptHandler) {
assert (oHandler.moDebugger == this);
oHandler.moDebugger = null;
moScriptHandlers.remove(i);
return;
}
}
assert (false); // should be found!
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy