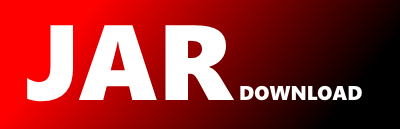
com.adobe.xfa.ScriptDynamicPropObj Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa;
/**
* This class stores the definition of the methods used to set/get dynamic
* properties for the XFA scripting interface.
*
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public abstract class ScriptDynamicPropObj {
// Fields used in the C++ implementation that are only of interest to Designer,
// and aren't used in XFA4J. References to these properties have been removed
// elsewhere.
//private final boolean mbRead;
//private final boolean mbWrite;
//private final int meParamType; // return type
//private final int mnVersionDep; // deprecated version
private final int mnXFAVersion; // intro version
private final int mnAvailability; // 0 means deprecated
public ScriptDynamicPropObj(int nXFAVersion, int nAvailability) {
mnXFAVersion = nXFAVersion;
mnAvailability = nAvailability;
}
public abstract boolean invokeGetProp(Obj scriptThis, Arg retValue, String sPropertyName);
public boolean invokeSetProp(Obj scriptThis, Arg propertyValue, String sPropertyName) {
// by default, assume that there is no setter
assert false;
return false;
}
public final boolean invokePermsFunc(Obj scriptThis) {
// For (dynamic) properties, the permission function is always ObjScript#setPropPermsCheck(Obj),
// so we call it directly rather than invoking through Reflection.
return ObjScript.setPropPermsCheck(scriptThis);
}
/**
* Determines whether getting this property is supported.
*
* Since all dynamic properties currently support getting, this returns true.
* If the unusual case where a write-only property is implemented, this would
* have to be overridden to return false.
* @return true
if getting this property is supported.
*/
public boolean hasGetter() {
return true;
}
/**
* Determines whether setting this property is supported.
*
* Since most dynamic properties don't support set operations,
* this returns false
by default. If a derived class
* implements a settable property, it must override this method
* to return true
.
* @return true
if setting this property is supported.
*/
public boolean hasSetter() {
return false;
}
public final int getXFAVersion() {
return mnXFAVersion;
}
public final int getAvailability() {
return mnAvailability;
}
}