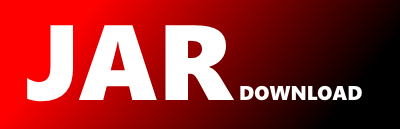
com.adobe.xfa.connectionset.ConnectionSetModel Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2006 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.connectionset;
import java.io.File;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.Element;
import com.adobe.xfa.Generator;
import com.adobe.xfa.Model;
import com.adobe.xfa.ModelFactory;
import com.adobe.xfa.Node;
import com.adobe.xfa.STRS;
import com.adobe.xfa.Schema;
import com.adobe.xfa.TextNode;
import com.adobe.xfa.XFA;
import com.adobe.xfa.connectionset.proxies.ConnectionSetProxy;
import com.adobe.xfa.template.TemplateModel;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.FindBugsSuppress;
import com.adobe.xfa.ut.Numeric;
import com.adobe.xfa.ut.Peer;
import com.adobe.xfa.ut.ResId;
import com.adobe.xfa.ut.Storage;
import com.adobe.xfa.ut.StringUtils;
import com.adobe.xfa.wspolicy.Policy;
/**
* A class to model the collection of all XFA nodes that make
* up form connectionset.
* @exclude from published api.
*/
public final class ConnectionSetModel extends Model {
private static final ConnectionSetSchema gConnectionSetSchema
= new ConnectionSetSchema();
private static final String gsConnectionSetUri = STRS.XFACONNECTIONSETNS_CURRENT;
Storage mProxyArray = new Storage();
/**
* @exclude from published api.
*/
public ConnectionSetModel(Element parent, Node prevSibling) {
super(parent, prevSibling, gsConnectionSetUri, XFA.CONNECTIONSET,
XFA.CONNECTIONSET, STRS.DOLLARCONNECTIONSET,
XFA.CONNECTIONSETTAG, XFA.CONNECTIONSET,
getModelSchema());
setModel(this);
}
/**
* Namespace
* @return The connectionset namespace URI string
*
* @exclude from published api.
*/
public static String connectionSetNS() {
return gsConnectionSetUri;
}
/**
* Gets the connectionset model held within an XFA DOM hierarchy.
*
* @param appModel the application model.
*
* @param bCreateIfNotFound when set, create a model if necessary.
*
* @return the connectionset model or null if none found.
*/
public static ConnectionSetModel getConnectionSetModel(AppModel appModel,
boolean bCreateIfNotFound /* = false */) {
ConnectionSetModel model = (ConnectionSetModel) Model.getNamedModel(appModel, XFA.CONNECTIONSET);
if (bCreateIfNotFound && model == null) {
ConnectionSetModelFactory factory = new ConnectionSetModelFactory();
model = (ConnectionSetModel) factory.createDOM((Element)appModel.getXmlPeer());
model.setDocument(appModel.getDocument());
model.loadChildren(model, new Generator("", ""));
appModel.notifyPeers(Peer.CHILD_ADDED, XFA.CONNECTIONSET, model);
}
return model;
}
/**
* @exclude from published api.
*/
protected static Schema getModelSchema() {
return gConnectionSetSchema;
}
/**
* @see Model#createNode(int, Element, String, String, boolean)
*
* @exclude from published api.
*/
@FindBugsSuppress(code="ES")
public Node createNode(int eTag, Element parent, String aName, String aNS, boolean bDoVersionCheck) {
assert (aName != null);
assert (aNS != null);
Element retVal = getSchema().getInstance(eTag, this, parent, null, bDoVersionCheck);
if (aName != "") {
retVal.setName(aName);
}
return retVal;
}
/**
* @exclude from published api.
*/
public Node doLoadNode(Node parent, Node node, Generator genTag) {
// WS-Policy grammar under elements is opaque to XFA
if (node instanceof Element && ((Element) node).getNS() == Policy.aWSP_NAMESPACE_URI)
return null;
return doLoadNode(parent, node, genTag);
}
/**
* Executes the given connection.
*
* Adobe patent application tracking # P624, entitled
* "Form-based Data Storage And Retrieval", inventors: Matveief,Young,Solc
* @exclude from published api.
*/
public boolean execute(Element oConnectionNode, boolean bDynamicMerge /* = false */) {
// for now we only handle wsdlconnections
for (int i = 0; i < mProxyArray.size(); i++) {
if (mProxyArray.get(i).handlesConnection(XFA.WSDLCONNECTIONTAG)) {
return mProxyArray.get(i).execute(oConnectionNode, bDynamicMerge);
}
}
return false;
}
/**
* Executes the given connection.
*
* Adobe patent application tracking # P624, entitled
* "Form-based Data Storage And Retrieval", inventors: Matveief,Young,Solc
* @param sConnectionName the name of the connection to execute.
* @param bDynamicMerge do a full remerge, killing all that is
* in the data DOM.
* @return true if connection was executed, false otherwise.
* @exclude from published api.
*/
public boolean execute(String sConnectionName, boolean bDynamicMerge /* = false */) {
// for now we only handle wsdlconnections
Node oExecuteNode = getNamedConnection(sConnectionName, XFA.WSDLCONNECTIONTAG);
if (! (oExecuteNode instanceof Element))
return false;
return execute((Element) oExecuteNode, bDynamicMerge);
}
/**
* @see Model#getBaseNS()
*
* @exclude from published api.
*/
public String getBaseNS() {
return STRS.XFACONNECTIONSETNS;
}
/**
* Gets the name of the dataDescription property for the given connection.
*
* @param sConnectionName the name of the connection.
*
* @return name of the dataDescription.
*
* @exclude from published api.
*/
public String getDataDescriptionName(String sConnectionName) {
String sDDName = null;
Node oConnection = getNamedConnection(sConnectionName);
if (oConnection instanceof Element) {
Attribute oDDAttr
= ((Element) oConnection).getAttribute(XFA.DATADESCRIPTIONTAG, true, false);
if (oDDAttr != null)
sDDName = oDDAttr.toString();
}
return sDDName;
}
/**
* Gets the default data connection, if any.
* @return The default data connection or null if not found.
*
* @exclude from published api.
*/
public Node getDefaultDataConnection() {
//
// default data connection is the first XML Schema
// or Sample XML connection
//
Node child = getFirstXFAChild();
while (child != null) {
if (child.isSameClass(XFA.XSDCONNECTIONTAG) || child.isSameClass(XFA.XMLCONNECTIONTAG))
return child;
child = child.getNextXFASibling();
}
return null;
}
/**
* Gets whether the events should be propagated or not.
* @return true or false.
*/
boolean getEventDispatch() {
throw new ExFull(ResId.UNSUPPORTED_OPERATION, "ConnectionSetModel#getEventDispatch");
}
/**
* @exclude from published api.
*/
public String getHeadNS() {
return ConnectionSetModel.connectionSetNS();
}
/**
* @exclude from published api.
*/
public int getHeadVersion() {
return Schema.XFAVERSION_CONNECTIONSETHEAD;
}
/**
* @exclude from published api.
*/
protected Node getNamedConnection(String sConnectionName) {
if (sConnectionName == null)
return null;
String aConnectionName = sConnectionName.intern();
Node child = getFirstXFAChild();
while (child != null) {
if (child.getName() == aConnectionName)
return child;
child = child.getNextXFASibling();
}
return null;
}
/**
* @exclude from published api.
*/
public Node getNamedConnection(String sConnectionName, int eClass) {
if (sConnectionName == null)
return null;
String aConnectionName = sConnectionName.intern();
Node child = getFirstXFAChild();
while (child != null) {
if (child.isSameClass(eClass)) {
if (child.getName() == aConnectionName)
return child;
}
child = child.getNextXFASibling();
}
return null;
}
/**
* @exclude from published api.
*/
public String getNS() {
int nVersion = getCurrentVersion();
// Check if there is a template in the AppModel. If so
// then we want to set the version of the ConnectionSet
// based on the template.
AppModel appModel = getAppModel();
if (appModel != null) {
for (Node child = appModel.getFirstXFAChild(); child != null; child = child.getNextXFASibling()) {
if (child instanceof TemplateModel) {
nVersion = ((TemplateModel)child).getCurrentVersion();
break;
}
}
}
// if we have a version set use it.
if (nVersion > 0) {
// The ConnectionSet was bumped for Acrobat 9.0
if (nVersion >= Schema.XFAVERSION_28)
nVersion = Schema.XFAVERSION_28;
// The connectionSet was bumped for P2B/P3A
// to 2.4
else if ((nVersion > Schema.XFAVERSION_25) && (nVersion < Schema.XFAVERSION_28))
nVersion = Schema.XFAVERSION_24;
// the ns stayed the same between 1.0 and 2.5
else if (nVersion <= Schema.XFAVERSION_25)
nVersion = Schema.XFAVERSION_21;
double dVersion = ((double) nVersion) / 10.0;
String sNS = getBaseNS() + Numeric.doubleToString(dVersion, 1, false) + '/'; // add trailing '/'
// generate an atom with the version.
return sNS.intern();
}
return super.getNS();
}
/**
* @exclude from published api.
*/
public void loadChildren(Node startNode, Generator genTag) {
isLoading(true);
// Document doc = getOwnerDocument();
// Javaport: TODO addId()? -- use model NS
// doc.addId("", XFA.ID, startNode);
// loadChildren(startNode, genTag);
// Resolve nodes that refer elsewhere
resolveProtos(false);
isLoading(false);
}
/**
* @exclude from published api.
*/
public void loadNode(Node parent, Node node, Generator genTag) {
doLoadNode(parent, node, genTag);
}
/**
* @see Model#postLoad()
*
* @exclude from published api.
*/
protected void postLoad() {
AppModel oApp = getAppModel();
List factories = oApp.factories();
for (int i = 0; i < factories.size(); i++) {
ModelFactory oFactory = factories.get(i);
//
// root of an XFA Model,
// must call isRootName becasue isRootNode for XFA-Data
// will always return true
//
if (oFactory.isRootName(getLocalName())) {
assert(oFactory instanceof ConnectionSetModelFactory);
ConnectionSetModelFactory factory = (ConnectionSetModelFactory) oFactory;
for (int j = 0; j < factory.mProxyArray.size(); j++) {
registerProxy(factory.mProxyArray.get(j));
}
}
}
}
/**
* @exclude from published api.
*/
public boolean publish(Model.Publisher publisher) {
Map fileRefCache = new HashMap();
publishNode(publisher, this, fileRefCache);
return super.publish(publisher);
}
/*
* recursive version of publish()
*/
private void publishNode(Model.Publisher publisher, Node node, Map fileRefCache) {
if (node.isSameClass(XFA.URITAG)) {
Node child = node.getFirstXFAChild();
if (child instanceof TextNode) {
TextNode textNode = (TextNode)node.getFirstXFAChild();
String sFileRef = textNode.getValue();
String sNewFileRef = fileRefCache.get(sFileRef);
if (StringUtils.isEmpty(sNewFileRef)) {
// call publisher's virtual method updateExternalRef() to get a new value
sNewFileRef = publisher.updateExternalRef(node, XFA.TEXTNODETAG, sFileRef);
fileRefCache.put(sFileRef, sNewFileRef);
}
if (!sFileRef.equals(sNewFileRef))
textNode.setValue(sNewFileRef, true, false);
}
}
else if (node.isSameClass(XFA.WSDLADDRESSTAG)) {
Node child = node.getFirstXFAChild();
if (child instanceof TextNode) {
TextNode textNode = (TextNode)child;
String sFileRef = textNode.getValue();
// Do we have an actual file ref or a http:// URL?
if (new File(sFileRef).isFile()) {
String sNewFileRef = fileRefCache.get(sFileRef);
if (StringUtils.isEmpty(sNewFileRef)) {
// call publisher's virtual method updateExternalRef() to get a new value
sNewFileRef = publisher.updateExternalRef(node, XFA.TEXTNODETAG, sFileRef);
fileRefCache.put(sFileRef, sNewFileRef);
}
if (!sFileRef.equals(sNewFileRef))
textNode.setValue(sNewFileRef, true, false);
}
}
}
for (Node child = node.getFirstXFAChild(); child != null; child = child.getNextXFASibling()) {
publishNode(publisher, child, fileRefCache);
}
}
/**
* @exclude from published api.
*/
public void registerProxy(ConnectionSetProxy oProxy) {
ConnectionSetProxy oClone = oProxy.clone();
oClone.moOwner = this;
mProxyArray.add(oClone);
}
/**
* Sets whether the events should be propagated or not.
* @param state true or false.
*/
void setEventDispatch(boolean state) {
throw new ExFull(ResId.UNSUPPORTED_OPERATION, "ConnectionSetModel#setEventDispatch");
}
/**
* Special check. The template, configuration xdc and sourceSet models
* support this operation to reduce the resolution time. These models
* will collect the protos as they are loading the model. This flag will
* be used to make sure we don't filter all the nodes again, if we've
* already collected them.
* @exclude from published api.
*/
protected boolean supportsSaveProtoInformation() {
return true;
}
}