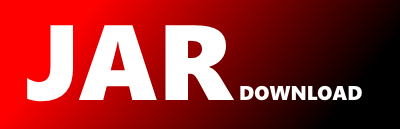
com.adobe.xfa.connectionset.EffectivePolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/**
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2007 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and may be covered by U.S. and Foreign Patents,
* patents in process, and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*/
package com.adobe.xfa.connectionset;
import com.adobe.xfa.Document;
import com.adobe.xfa.Element;
import com.adobe.xfa.Model;
import com.adobe.xfa.Node;
import com.adobe.xfa.ProtoableNode;
import com.adobe.xfa.ut.BooleanHolder;
import com.adobe.xfa.ut.ObjectHolder;
import com.adobe.xfa.wspolicy.Policy;
import com.adobe.xfa.wspolicy.PrimitiveAssertion;
import com.adobe.xfa.wspolicy.CompoundAssertion;
/**
* @exclude from published api.
*/
public class EffectivePolicy extends ProtoableNode {
public EffectivePolicy(Element oParent, Node oNode, Model oModel) {
super(oParent, oNode/*, oModel*/);
}
protected EffectivePolicy (Element parent, Node prevSibling, int classTag, String className) {
super (parent, prevSibling, null, className, className, null, classTag, className);
}
public Element clone(Element oParent, boolean bDeep) {
EffectivePolicy oClone = (EffectivePolicy) super.clone(oParent, bDeep);
return oClone;
}
/*
* Note: in the event that these return multiple supported authentication
* methods, we are required to execute the strongest method we support
*
* NB: While LC 8.2 & Designer 9 may only support username/pwd authentication,
* this library will also return certificate policies.
*/
public void getTransportPolicy(BooleanHolder obHTTPS, BooleanHolder obBasic, BooleanHolder obDigest, BooleanHolder obHTTPCert) {
// Set defaults (no policies)
obHTTPS.value = false;
obBasic.value = false;
obDigest.value = false;
obHTTPCert.value = false;
if (moPolicy == null)
moPolicy = Policy.resolvePolicy(this);
if (moPolicy == null)
return;
/*
*/
PrimitiveAssertion oTransBinding = moPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_TRANSPORTBINDING, 0);
if (oTransBinding != null && oTransBinding.hasNestedPolicy()) {
CompoundAssertion oTransBindingPolicy = oTransBinding.getNestedPolicy();
PrimitiveAssertion oTransToken = oTransBindingPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_TRANSPORTTOKEN, 0);
if (oTransToken != null && oTransToken.hasNestedPolicy()) {
CompoundAssertion oTransTokenPolicy = oTransToken.getNestedPolicy();
PrimitiveAssertion oHTTPSToken = oTransTokenPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_HTTPSTOKEN, 0);
if (oHTTPSToken != null) {
obHTTPS.value = true;
CompoundAssertion oHTTPSTokenPolicy = oHTTPSToken.getNestedPolicy();
if (oHTTPSTokenPolicy != null) {
//
// We expect a single wsp:ExactlyOne child or a single primitive child. More
// complex structures are not supported.
//
Policy oPolicy = oHTTPSTokenPolicy.getFirstPolicyChild();
if (oPolicy != null && oPolicy.getAssertionType() == Policy.WSP_EXACTLY_ONE_ASSERTION)
oHTTPSTokenPolicy = (CompoundAssertion) oPolicy;
//
// Note: the HTTPSTokenPolicy could list several authentication methods. We must
// support the strongest one that we know how to.
//
PrimitiveAssertion oClientCertToken = oHTTPSTokenPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_REQUIRECLIENTCERTIFICATE, 0);
if (oClientCertToken != null) {
obHTTPCert.value = true;
}
PrimitiveAssertion oDigestToken = oHTTPSTokenPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_HTTPDIGESTAUTHENTICATION, 0);
if (oDigestToken != null) {
obDigest.value = true;
}
PrimitiveAssertion oBasicToken = oHTTPSTokenPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_HTTPBASICAUTHENTICATION, 0);
if (oBasicToken != null) {
obBasic.value = true;
}
}
}
}
}
}
public void getSOAPPolicy(BooleanHolder oUsernameClearPwd, BooleanHolder oUsernameHashPwd, BooleanHolder oSOAPCert) {
// Set defaults (no policies)
oUsernameClearPwd.value = false;
oUsernameHashPwd.value = false;
oSOAPCert.value = false;
if (moPolicy == null)
moPolicy = Policy.resolvePolicy(this.getXMLParent());
if (moPolicy == null)
return;
/*
*/
PrimitiveAssertion oSupTokens = moPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_SUPPORTINGTOKENS, 0);
if (oSupTokens != null && oSupTokens.hasNestedPolicy()) {
CompoundAssertion oSupTokensPolicy = oSupTokens.getNestedPolicy();
if (oSupTokensPolicy != null) {
//
// We expect a single wsp:ExactlyOne child or a single primitive child. More
// complex structures are not supported.
//
Policy oPolicy = oSupTokensPolicy.getFirstPolicyChild();
if (oPolicy != null && oPolicy.getAssertionType() == Policy.WSP_EXACTLY_ONE_ASSERTION)
oSupTokensPolicy = (CompoundAssertion) oPolicy;
PrimitiveAssertion oUsernameToken = oSupTokensPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_USERNAMETOKEN, 0);
if (oUsernameToken != null) {
CompoundAssertion oUsernameTokenPolicy = oUsernameToken.getNestedPolicy();
PrimitiveAssertion oHashPwdToken = null;
if (oUsernameTokenPolicy != null)
oHashPwdToken = oUsernameTokenPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_HASHPASSWORD, 0);
if (oHashPwdToken == null)
oUsernameClearPwd.value = true;
else
oUsernameHashPwd.value = true;
}
//
// Check for a second username token (which might have hashPassword set differently)
//
oUsernameToken = oSupTokensPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_USERNAMETOKEN, 1);
if (oUsernameToken != null) {
CompoundAssertion oUsernameTokenPolicy = oUsernameToken.getNestedPolicy();
PrimitiveAssertion oHashPwdToken = null;
if (oUsernameTokenPolicy != null)
oHashPwdToken = oUsernameTokenPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_HASHPASSWORD, 0);
if (oHashPwdToken == null)
oUsernameClearPwd.value = true;
else
oUsernameHashPwd.value = true;
}
PrimitiveAssertion oX509Token = oSupTokensPolicy.getPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_X509TOKEN, 0);
if (oX509Token != null) {
oSOAPCert.value = true;
}
}
}
}
/*
* Note that because the implementation is required to pick the strongest
* assertion they support, it is quite legal to write several assertions
* into a single policy (ie: HTTPBasic + HTTPDigest + HTTPCert).
*/
public void setPolicy(boolean bHTTPS, boolean bBasic, boolean bDigest, boolean bHTTPCert,
boolean bUsernameClearPwd, boolean bUsernameHashPwd, boolean bSOAPCert) {
//
// Rationalizing markup would be much better, but also much more work. We're not going
// to do anything with hand-edited values anyway, so what's the point in keeping them?
//
Policy.deletePolicyInfo(this);
moPolicy = null;
makeDefault();
if (bHTTPS || bBasic || bDigest || bHTTPCert || bUsernameClearPwd || bUsernameHashPwd || bSOAPCert) {
Document oDoc = this.getOwnerDocument();
ObjectHolder oPolicyNode = new ObjectHolder();
moPolicy = Policy.newPolicy(oDoc, oPolicyNode);
appendChild(oPolicyNode.value);
makeNonDefault(false);
}
/*
*/
if (bHTTPS || bBasic || bDigest || bHTTPCert) {
PrimitiveAssertion oTransBinding = moPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_TRANSPORTBINDING);
CompoundAssertion oTransBindingPolicy = oTransBinding.addNestedPolicy();
PrimitiveAssertion oTransToken = oTransBindingPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_TRANSPORTTOKEN);
CompoundAssertion oTransTokenPolicy = oTransToken.addNestedPolicy();
PrimitiveAssertion oHTTPSToken = oTransTokenPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_HTTPSTOKEN);
int count = (bBasic ? 1 : 0) + (bDigest ? 1 : 0) + (bHTTPCert ? 1 : 0);
if (count > 0) {
CompoundAssertion oHTTPSTokenPolicy = oHTTPSToken.addNestedPolicy();
if (count > 1)
oHTTPSTokenPolicy = oHTTPSTokenPolicy.addChoicePolicy();
if (bBasic)
oHTTPSTokenPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_HTTPBASICAUTHENTICATION);
if (bDigest)
oHTTPSTokenPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_HTTPDIGESTAUTHENTICATION);
if (bHTTPCert)
oHTTPSTokenPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_REQUIRECLIENTCERTIFICATE);
}
}
/*
*/
int count = (bUsernameClearPwd ? 1 : 0) + (bUsernameHashPwd ? 1 : 0) + (bSOAPCert ? 1 : 0);
if (count > 0) {
PrimitiveAssertion oSupTokens = moPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_SUPPORTINGTOKENS);
CompoundAssertion oSupTokensPolicy = oSupTokens.addNestedPolicy();
if (count > 1)
oSupTokensPolicy = oSupTokensPolicy.addChoicePolicy();
if (bUsernameClearPwd)
oSupTokensPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_USERNAMETOKEN);
if (bUsernameHashPwd) {
PrimitiveAssertion oUsernameToken = oSupTokensPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_USERNAMETOKEN);
CompoundAssertion oUsernameTokenPolicy = oUsernameToken.addNestedPolicy();
oUsernameTokenPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_HASHPASSWORD);
}
if (bSOAPCert)
oSupTokensPolicy.addPrimitiveAssertion(Policy.aSP_NAMESPACE_URI, Policy.aSP_X509TOKEN);
}
}
private CompoundAssertion moPolicy;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy