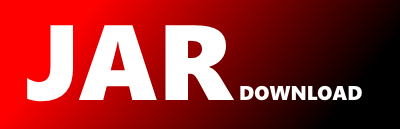
com.adobe.xfa.connectionset.proxies.WSDLConnectionSetProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Adobe Confidential
* ------------------
* Copyright 2003 Adobe Systems Incorporated
* All Rights Reserved.
*
* Notice: all information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems Inc.
* and its suppliers and may be covered by U.S. and foreign patents, patents in
* process, and are protected by trade secret or copyright law. Dissemination
* of this information or reproduction of this material is strictly forbidden
* unless prior written permission is obtained from Adobe Systems Inc.
*/
// Adobe Patent or Adobe Patent Pending Invention Included Within this File
package com.adobe.xfa.connectionset.proxies;
import java.io.BufferedInputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Element;
import com.adobe.xfa.EnumAttr;
import com.adobe.xfa.EventPseudoModel;
import com.adobe.xfa.Node;
import com.adobe.xfa.TextNode;
import com.adobe.xfa.STRS;
import com.adobe.xfa.XFA;
import com.adobe.xfa.data.DataModel;
import com.adobe.xfa.data.DataNode;
import com.adobe.xfa.form.FormModel;
import com.adobe.xfa.form.FormModel.ConnectHandler;
import com.adobe.xfa.soap.SOAP;
import com.adobe.xfa.connectionset.EffectiveInputPolicy;
import com.adobe.xfa.protocol.Protocol;
import com.adobe.xfa.protocol.AuthenticationHandler;
import com.adobe.xfa.template.TemplateModel;
import com.adobe.xfa.ut.BooleanHolder;
import com.adobe.xfa.ut.CharacterHolder;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.FindBugsSuppress;
import com.adobe.xfa.ut.MsgFormatPos;
import com.adobe.xfa.ut.ObjectHolder;
import com.adobe.xfa.ut.ResId;
import com.adobe.xfa.ut.Resolver;
import com.adobe.xfa.ut.Storage;
import com.adobe.xfa.ut.StringHolder;
import com.adobe.xfa.ut.StringUtils;
/**
* The XFAConnectionSetProxy defines a proxy for connectionSets that wish to define an execute action.
* @exclude from published api.
*/
public class WSDLConnectionSetProxy extends ConnectionSetProxy {
/**
* @exclude from published api.
* JAVAPORT TODO: In C++, the oSoapModel parameter is passed by reference
*/
public interface ConnectionSetProxyHandler {
void handleProxy(ConnectionSetProxy oProxy, Notifications eNotice, SOAP oSOAPModel, Object oHandlerData);
}
private static class FixupSOAPHandler implements ConnectHandler {
public boolean handleConnect(Node oNode, String sConnectionRootRef, String sConnectRef, String sConnectPicture, Object handlerData, ObjectHolder ioRecursingData) {
assert (handlerData instanceof StringHolder);
StringHolder sBodyFirstChildName = (StringHolder) handlerData;
String sBodyRef = "Body.";
int nBodyRefLen = sBodyRef.length();
if (sConnectRef.startsWith(sBodyRef)) {
int nFoundAt = sConnectRef.indexOf('.', nBodyRefLen);
if (nFoundAt >= 0) {
sBodyFirstChildName.value = sConnectRef.substring(nBodyRefLen, nFoundAt);
}
else {
sBodyFirstChildName.value = sConnectRef.substring(nBodyRefLen);
}
return false; // don't continue searching!
}
return true;
}
}
/**
* @exclude from published api.
*/
public enum Notifications {
PREEXECUTE /* = 0 */,
POSTEXECUTE
}
private final ConnectionSetProxyHandler moHandler;
private final Object moHandlerData;
private String msConnectionName;
private Element moWSDLConnectionNode;
private String msSOAPFile;
/**
* The default c'tor -- instantiate an ConnectionSetProxy object.
*/
public WSDLConnectionSetProxy() {
this(null, null);
}
public WSDLConnectionSetProxy(WSDLConnectionSetProxy oProxy) {
this(oProxy.moHandler, oProxy.moHandlerData);
msConnectionName = oProxy.msConnectionName;
msSOAPFile = oProxy.msSOAPFile;
//moWSDLConnectionNode = null;
}
public WSDLConnectionSetProxy(ConnectionSetProxyHandler handler, Object inHandlerData /* = null */) {
moHandler = handler;
moHandlerData = inHandlerData;
//moWSDLConnectionNode = null;
}
/**
* allow cloning
*/
public ConnectionSetProxy clone() {
return new WSDLConnectionSetProxy(this);
}
private void createBasicWSSecurityHeader(ByteArrayOutputStream oHeaderStreamFile,
String sUserId, String sPassword, boolean bDigest) {
if (oHeaderStreamFile.size() > 0) {
// we do not mess with existing soap headers
return;
}
else {
// create the SOAP header as a String, then put it into the oHeaderStreamFile
String sEscapedUserid = escapePasswordCharacters(sUserId);
String sEscapedPassword = escapePasswordCharacters(sPassword);
String sXMLHeader =
"\n" +
"" +
"" +
"" +
StringUtils.toXML(sEscapedUserid, false) +
// Watson 2371660. Add the namespace to #PasswordText
" " +
StringUtils.toXML(sEscapedPassword, false) +
" " +
" ";
// write the SOAP header into the stream file
try {
oHeaderStreamFile.write(sXMLHeader.getBytes("UTF-8"));
} catch (UnsupportedEncodingException e) {
assert false; // UTF-8 is always supported.
} catch (IOException e) {
assert false; // ByteArrayOutputStream shouldn't exception.
}
// rewind the header stream
}
}
String escapePasswordCharacters(String sText) {
// Note: not very sophisticated, but it works. It will fall down if any value you
// replace contains a key to lookup next. For example, if you replace a "<" with
// a "<" and then you search for an "&", this algorithm falls down.
StringBuilder sResult = new StringBuilder(sText);
Storage oKeys = new Storage();
oKeys.add("&");
oKeys.add("<");
oKeys.add(">");
Storage oValues = new Storage();
oValues.add("&");
oValues.add("<");
oValues.add(">");
int nKeys = oKeys.size();
for (int i = 0; i < nKeys; i++) {
int nOffset = 0; // start at the beginning
String sKey = oKeys.get(i);
int nKeyLength = sKey.length();
String sValue = oValues.get(i);
int nValueLength = sValue.length();
while (true) {
int nFoundAt = sResult.indexOf(sKey, nOffset);
if (nFoundAt > -1) {
sResult.replace(nFoundAt, nFoundAt + nKeyLength, sValue);
nOffset = nFoundAt + nValueLength;
}
else {
break;
}
}
}
return sResult.toString();
}
/**
* Adobe patent application tracking # P624,
* entitled "Form-based Data Storage And Retrieval",
* inventors: Matveief,Young,Solc
* @return true if the connection was successfully executed.
*/
@FindBugsSuppress(pattern="DLS_DEAD_LOCAL_STORE,NP_LOAD_OF_KNOWN_NULL_VALUE")
public boolean execute(Element oWSDLConnectionNode, boolean bDynamicMerge) {
//
// get the template model and event pseudo model
//
TemplateModel oTemplateModel = null;
EventPseudoModel oEventPseudoModel = null;
assert(moOwner != null && moOwner.getAppModel() != null);
if (moOwner != null && moOwner.getAppModel() != null) {
oTemplateModel = TemplateModel.getTemplateModel(moOwner.getAppModel(), false);
oEventPseudoModel = (EventPseudoModel) moOwner.getAppModel().lookupPseudoModel(STRS.DOLLAREVENT);
}
assert(oTemplateModel != null && oEventPseudoModel != null);
if (oTemplateModel == null || oEventPseudoModel == null)
return false;
moWSDLConnectionNode = oWSDLConnectionNode;
//
// get some important strings
//
msConnectionName = oWSDLConnectionNode.getName();
String sSoapAction = getElementValue(oWSDLConnectionNode, XFA.SOAPACTIONTAG);
String sSoapAddress = getElementValue(oWSDLConnectionNode, XFA.SOAPADDRESSTAG);
@SuppressWarnings("unused")
String sWSDLAddress = getElementValue(oWSDLConnectionNode, XFA.WSDLADDRESSTAG);
//
// export to data dom using the correct dataDescription.
//
FormModel formModel = FormModel.getFormModel(moOwner.getAppModel(), true);
assert(formModel != null);
formModel.exportConnectionData(msConnectionName, oWSDLConnectionNode.getAttribute(XFA.DATADESCRIPTIONTAG, true, false).toString());
SOAP oPreSOAPModel = setSOAPModelFromConnectionData(false);
if (oPreSOAPModel == null)
return false;
//
// figure out if we should invoke legacy postExecute behaviour
//
boolean bLegacyPostExecute = oTemplateModel.getLegacySetting(AppModel.XFA_LEGACY_V27_EVENTMODEL);
//
// call the event where activity == "preExecute"
//
notifyPreExecute(oPreSOAPModel);
SOAP oSOAPModel = null;
DataNode connectionDataNode = null;
DataModel dataModel = null;
//
// was execute canceled by preExecute?
//
if (oEventPseudoModel.cancelAction(STRS.EXECUTE)) {
//
// yes, if this form desires legacy postExecute behaviour, reset cancelAction manually
//
if (bLegacyPostExecute)
oEventPseudoModel.setCancelAction(false, STRS.POSTEXECUTE);
else // otherwise, dispatch postExecute
notifyPostExecute(oSOAPModel);
moWSDLConnectionNode = null;
return false;
}
SOAP oResponseModel = null;
try {
//
// the preExecute Script might have modified it the soapModel.
//
oSOAPModel = setSOAPModelFromConnectionData(true);
if (oSOAPModel == null) {
//
// if this form desires legacy postExecute behaviour, reset cancelAction manually
//
if (bLegacyPostExecute)
oEventPseudoModel.setCancelAction(false, STRS.POSTEXECUTE);
else // otherwise, dispatch postExecute
notifyPostExecute(oSOAPModel);
moWSDLConnectionNode = null;
return false;
}
//
// send the request to the soap server
//
oResponseModel = oSOAPModel.sendRequest(sSoapAddress, sSoapAction);
if (oResponseModel == null) {
//
// if this form desires legacy postExecute behaviour, reset cancelAction manually
//
if (bLegacyPostExecute)
oEventPseudoModel.setCancelAction(false, STRS.POSTEXECUTE);
else // otherwise dispatch postExecute
notifyPostExecute(oSOAPModel);
moWSDLConnectionNode = null;
return false;
}
//
// Authentication/WS-Security
//
// The oResponseModel contains the SOAP response with the fault codes, the sErrorInfo
// string is the error from the post.
//
String sScheme = "";
Protocol oProtocol = Resolver.getProtocol(sScheme);
if (oProtocol != null) {
AuthenticationHandler oAuthHandler = oProtocol.getAuthenticationHandler();
if (oAuthHandler != null) {
//
// check to see if we were authenticating the SOAP request, if we are, then we
// need to set the SOAP request status, store any fault information, and
// and call the handler checkSOAPAuthenticationStatus method
//
AuthenticationHandler.AuthenticationType eSOAPAuthType = oAuthHandler.getSOAPAuthType();
if (eSOAPAuthType != AuthenticationHandler.AuthenticationType.NONE) {
//
// tell the authentication handler what the status of the soap request is
//
if (oResponseModel.getFaultNode() == null) {
oAuthHandler.setSOAPRequestStatus(AuthenticationHandler.SOAPRequestStatus.SOAPSUCCESS);
}
else {
// This is what the response model looks like, if we got a fault due to
// and authorization failure.
//
//
//
//
//
// soapenv:Server.userException
// | [AuthenticationManagerBean] errorCode:12803 errorCodeHEX:0x3203 message:None of the Auth Provider could authenticate the user. Authentication Failed
//
//
// 12803
// None of the Auth Provider could authenticate the user.Authentication Failed
//
// xtg2-xp
//
//
//
//
oAuthHandler.setSOAPRequestStatus(AuthenticationHandler.SOAPRequestStatus.SOAPFAULT);
//
// store the and strings in the handler ...
//
String sFaultCode = oResponseModel./*getFaultNode().*/getFaultCode();
String sResponse = oResponseModel./*getFaultNode().*/getFaultString();
oAuthHandler.setSOAPFaultString(sResponse);
oAuthHandler.setSOAPFaultString(sFaultCode);
//
// "SOAP fault while connecting to '%0'. SOAP Fault code is '%1', SOAP response is '%2'."
//
MsgFormatPos oError = new MsgFormatPos(ResId.SoapFaultReported);
oError.format(sSoapAddress).format(sFaultCode).format(sResponse);
oAuthHandler.logMessage(oError);
}
//
// call the method so that the client can check the status and deal with it
//
oAuthHandler.checkSOAPAuthenticationStatus();
}//endif (eSOAPAuthType != AuthenticationHandler.AuthenticationType.NONE)
}//endif oAuthHandler
}//endif oProtocol
//
// get the body out from the response
//
ByteArrayOutputStream oResponseBodyStream = new ByteArrayOutputStream();
if (oResponseModel.getBodyNode() != null) {
if (oResponseModel.getFaultNode() == null)
fixupSOAPResponseModel(oResponseModel, oTemplateModel);
SOAP.exportContentsToXML(oResponseModel.getBodyNode(), oResponseBodyStream);
}
//
// get the header out as XML
//
ByteArrayOutputStream oResponseHeaderStream = new ByteArrayOutputStream();
if (oResponseModel.getHeaderNode() != null)
SOAP.exportContentsToXML(oResponseModel.getHeaderNode(), oResponseHeaderStream);
//
// load the XML Dom into the Data DOM under the connectionData node
//
dataModel = DataModel.getDataModel(moOwner.getAppModel(), false, false);
String sConnectionDataRoot = "!" + XFA.CONNECTIONDATA;
connectionDataNode = (DataNode) dataModel.resolveNode(sConnectionDataRoot);
if (connectionDataNode == null)
connectionDataNode = (DataNode) dataModel.createChild(false, XFA.CONNECTIONDATA);
DataNode wsdlDataNode = (DataNode) connectionDataNode.resolveNode(msConnectionName);
if (wsdlDataNode != null) {
//
// clear out the old data
//
connectionDataNode.removeChild(wsdlDataNode);
}
//
// make a new root
//
wsdlDataNode = (DataNode) connectionDataNode.createChild(false, msConnectionName);
if (oResponseHeaderStream.size() > 0) {
Element headerNode = (Element) wsdlDataNode.createChild(false, "Header");
byte[] bytes = oResponseHeaderStream.toByteArray();
((Element) headerNode).loadXML(new ByteArrayInputStream(bytes), false, false);
bytes = null;
}
if (oResponseBodyStream.size() > 0) {
Element bodyNode = (Element) wsdlDataNode.createChild(false, "Body");
byte[] bytes = oResponseBodyStream.toByteArray();
((Element) bodyNode).loadXML(new ByteArrayInputStream(bytes), false, false);
bytes = null;
}
} catch (ExFull oError) {
//
// if this form desires legacy postExecute behaviour, reset cancelAction manually
//
if (bLegacyPostExecute)
oEventPseudoModel.setCancelAction(false, STRS.POSTEXECUTE);
else // otherwise dispatch postExecute
notifyPostExecute(oSOAPModel);
moWSDLConnectionNode = null;
//Fix for watson 2525265
//in case of exception the value of connectionDataNode was not getting populated which it had created in the method call "exportConnectionData"
//it need to populate and clear that value.
///*
dataModel = DataModel.getDataModel(moOwner.getAppModel(), true, false);
String sConnectionDataRoot = "!" + XFA.CONNECTIONDATA;
connectionDataNode = (DataNode) dataModel.resolveNode(sConnectionDataRoot);
//*/
//
// clear out the connectionDataNode
//
assert(connectionDataNode != null);
dataModel.removeChild(connectionDataNode);
throw oError;
}
//
// call the event where activity == "onDataReturned"
//
notifyPostExecute(oResponseModel);
ExFull oException = new ExFull();
try {
if (oResponseModel.getFaultNode() == null) {
if (! bDynamicMerge) {
//
// import/merge the data into the XFA Form DOM
//
formModel.importConnectionData(msConnectionName);
}
else {
// we want to remerge all the data so the form will create multiple subforms if necessary
// TODO Anatole - get the value for bAdjustData from Configuration DOM
//
// first init the weighting for the connection data - data model will init the
// main data hierarchy only
connectionDataNode.setWeight(1);
//
// now merge
//
formModel.merge(false, true, msConnectionName, true, false, false);
}
}
} catch (ExFull oEx) {
oException = oEx;
}
//
// clear out the connectionDataNode
//
dataModel.removeChild(connectionDataNode);
moWSDLConnectionNode = null;
if (oException.count() > 0)
throw oException;
if (oResponseModel.getFaultNode() == null)
return true;
return false;
}
private void fixupSOAPResponseModel(SOAP oSOAPModel, Node oTemplateNode) {
// in some cases the name of the first child of the Body element can be wrong
// this is because the SOAP spec is apparently not specific about how
// SOAP responses should be named. Since we are always dealing with Doc/Literal
// we can just mask this problem by renaming that child element to what we expect
// before imorting it into the Data DOM
StringHolder sBodyFirstChildName = new StringHolder();
FormModel.recurseConnectOnNode(oTemplateNode, msConnectionName, EnumAttr.USAGE_IMPORTONLY, new FixupSOAPHandler(), sBodyFirstChildName);
if (sBodyFirstChildName.value != null && sBodyFirstChildName.value.length() > 0) {
Node oBodyNode = oSOAPModel.getBodyNode();
Node oChildNode = oBodyNode.getFirstXMLChild();
while (oChildNode != null) {
if (oChildNode instanceof Element) {
((Element) oChildNode).setName(sBodyFirstChildName.value);
break;
}
oChildNode = oChildNode.getNextXMLSibling();
}
}
}
private String getElementValue(Element oNode, int eTag) {
String sRetValue = "";
Element oChildNode = oNode.getElement(eTag, false, 0, false, false);
if (oChildNode != null) {
TextNode oText = oChildNode.getText(false, false, false);
sRetValue = oText.getValue();
}
return sRetValue;
}
/*
* Adobe patent application tracking # P624,
* entitled "Form-based Data Storage And Retrieval",
* inventors: Matveief,Young,Solc
*/
@FindBugsSuppress(pattern="DLS_DEAD_LOCAL_STORE") // dataModel
private void getEnvelopeContentsFromDataDOM(OutputStream oOutHeaderStream, OutputStream oOutBodyStream, String sConnectionName) {
@SuppressWarnings("unused")
DataModel dataModel = DataModel.getDataModel(moOwner.getAppModel(), false, false);
String sRef = '!' + XFA.CONNECTIONDATA + '.' + sConnectionName;
//
// find the "datasets.connectionData.connectionName" node and save it out as XML.
//
Node oResolvedNode = moOwner.getAppModel().resolveNode(sRef, true, false, false);
if (oResolvedNode != null) {
Node oHeader = oResolvedNode.getNodes().getNamedItem("Header");
Node oBody = oResolvedNode.getNodes().getNamedItem("Body");
if (oHeader instanceof Element)
((Element) oHeader).saveXML(oOutHeaderStream, null);
if (oBody instanceof Element)
((Element) oBody).saveXML(oOutBodyStream, null);
}
}
/**
* returns the WSDLConnectionNode that is currently executing, only valid while executing.
*/
public Node getWSDLConnectionNode() {
return moWSDLConnectionNode;
}
/**
* does this Proxy handle a particular connection type?
* @return true if this proxy handles the connecitonType.
*/
public boolean handlesConnection(int eConnectionTag) {
return (eConnectionTag == XFA.WSDLCONNECTIONTAG);
}
private void notifyPostExecute(SOAP oSOAPModel) {
if (moHandler != null) {
moHandler.handleProxy(this, Notifications.POSTEXECUTE, oSOAPModel, moHandlerData);
}
// TODO Anatole
}
private void notifyPreExecute(SOAP oSOAPModel) {
if (moHandler != null) {
moHandler.handleProxy(this, Notifications.PREEXECUTE, oSOAPModel, moHandlerData);
}
// TODO Anatole
}
/**
* temp for testing
*/
public void setSOAPFile(String inFile) {
msSOAPFile = inFile;
}
/*
* Adobe patent application tracking # P624,
* entitled "Form-based Data Storage And Retrieval",
* inventors: Matveief,Young,Solc
*/
private SOAP setSOAPModelFromConnectionData(boolean bAuthenticate /* = false */) {
SOAP oSOAPModel = null;
if (StringUtils.isEmpty(msSOAPFile)) {
//
// export from data dom to jfMemoryStreamFiles - get a Header and a Body
//
ByteArrayOutputStream oHeaderStreamFile = new ByteArrayOutputStream();
ByteArrayOutputStream oBodyStreamFile = new ByteArrayOutputStream();
getEnvelopeContentsFromDataDOM(oHeaderStreamFile, oBodyStreamFile, msConnectionName);
//
// create a soap model from the streams
//
// Javaport: no needed to flush.
// oBodyStreamFile.flush();
//
//-------------------------------------------------------------------------------------
// Authentication/WS-Security
//
if (bAuthenticate) {
//
// We need to get the child of the child
// of the package. This contains the policy for this
// wsdl connection. We then ask this input policy for information on the authentication
// requirements for the soap request.
//
// If the policy tells us that we need authentication, we will create a
// header for our SOAP request.
//
Element oWSDLConnectionNode = moWSDLConnectionNode;
Element oInputPolicyProp = oWSDLConnectionNode.peekElement(XFA.EFFECTIVEINPUTPOLICYTAG, false, 0);
if (oInputPolicyProp != null) {
EffectiveInputPolicy oInputPolicy = (EffectiveInputPolicy)oInputPolicyProp;
if (oInputPolicy != null) {
String sScheme = "";
Protocol oProtocol = Resolver.getProtocol(sScheme);
if (oProtocol != null) {
AuthenticationHandler oAuthHandler = oProtocol.getAuthenticationHandler();
if (oAuthHandler != null) {
//
// reset the handler back to a clean state, so we can update it with
// this connection's information
// the handler holds information on the transport policy and the SOAP
// policy for only the current connection
//
oAuthHandler.reset();
//
// check to see if the transport layer requires authentication
// set that information into the authentication handler. It will
// only be used on the server, not in Designer or Acrobat.
//
BooleanHolder bHTTPS = new BooleanHolder();
BooleanHolder bHTTPBasic = new BooleanHolder();
BooleanHolder bHTTPDigest = new BooleanHolder();
BooleanHolder bHTTPCert = new BooleanHolder();
oInputPolicy.getTransportPolicy(bHTTPS, bHTTPBasic, bHTTPDigest, bHTTPCert);
//
// set the type of transport authentication in the handler for this connection
// the input policy can specify that more than one type of authentication is
// supported for this connection
// in this case, we must use the most secure authentication that we
// (our protocol layer) can support. For jfProtocol, and Acrobat's EFS,
// certificate is it.
//
if (bHTTPCert.value)
oAuthHandler.setTransportAuthType(AuthenticationHandler.AuthenticationType.CERTIFICATE);
else if (bHTTPDigest.value)
oAuthHandler.setTransportAuthType(AuthenticationHandler.AuthenticationType.DIGEST);
else if (bHTTPBasic.value)
oAuthHandler.setTransportAuthType(AuthenticationHandler.AuthenticationType.BASIC);
//
// set the connection name, SOAP address and SOAP operation name into
// the handler
//
String sConnectionName = moWSDLConnectionNode.getName();
String sSOAPAddress = getElementValue(moWSDLConnectionNode, XFA.SOAPADDRESSTAG);
String sSOAPOperation = getElementValue(moWSDLConnectionNode, XFA.OPERATIONTAG);
oAuthHandler.setConnectionName(sConnectionName);
oAuthHandler.setSOAPAddress(sSOAPAddress);
oAuthHandler.setSOAPOperation(sSOAPOperation);
//
// check to see if this SOAP request requires authentication
//
BooleanHolder bUsernameClearPwd = new BooleanHolder();
BooleanHolder bUsernameHashPwd = new BooleanHolder();
BooleanHolder bSOAPCert = new BooleanHolder();
oInputPolicy.getSOAPPolicy(bUsernameClearPwd, bUsernameHashPwd, bSOAPCert);
if (bSOAPCert.value) {
//
// certificate authentication required
// November 2007. We are not going to support certificate
// authentication at this time. There do not seem to be any
// standards in place for this yet.
//
}
else if (bUsernameClearPwd.value || bUsernameHashPwd.value) {
//
// basic or digest authentication required
// get the authentication handler from the protocol handler, set the
// soap releated information, and ask for the userid/password
// set the type of SOAP authentication in the handler for this connection
// the input policy can specify that more than one type of authentication is
// supported for this connection
// in this case, we must use the most secure authentication that we
// (our SOAP layer) can support.
//
if (bUsernameHashPwd.value)
oAuthHandler.setSOAPAuthType(AuthenticationHandler.AuthenticationType.DIGEST);
else if (bUsernameClearPwd.value)
oAuthHandler.setSOAPAuthType(AuthenticationHandler.AuthenticationType.BASIC);
StringHolder sUserID = new StringHolder();
CharacterHolder sPassword = new CharacterHolder();
//
// ask the handler for the userid and password
//
if (oAuthHandler.getBasicSOAPCredentials(sSOAPAddress, sConnectionName, sSOAPOperation, sUserID, sPassword)) {
//
// set these in the handler, so that the client can access them
//
oAuthHandler.setUserID(sUserID.value);
oAuthHandler.setPassword(sPassword);
//
// this method constructs the ws-security header ( ) with
// the userid and password, and places it into the oHeaderStream
//
createBasicWSSecurityHeader(oHeaderStreamFile, sUserID.value, sPassword.value==null?null:new String(sPassword.value), bUsernameHashPwd.value);
}
else {
// could not get credentials ...
}
}
}//endif oAuthHandler
}//endif oProtocol
}//endif if (oInputPolicy != null)
}//endif if (oInputPolicyProp != null)
}//endif bAuthenticate
//-------------------------------------------------------------------------------------
byte[] headerBytes = oHeaderStreamFile.toByteArray();
oHeaderStreamFile = null;
byte[] bodyBytes = oBodyStreamFile.toByteArray();
oBodyStreamFile = null;
oSOAPModel = SOAP.createFromXMLStreams(headerBytes.length > 0 ? new ByteArrayInputStream(headerBytes) : null,
new ByteArrayInputStream(bodyBytes), "");
// JavaPort: no need to close ByteArrayInputStreams.
headerBytes = null;
bodyBytes = null;
return oSOAPModel;
}
else {
try {
BufferedInputStream oStreamFile
= new BufferedInputStream(new FileInputStream(msSOAPFile));
oSOAPModel = SOAP.loadFromStream(oStreamFile, null);
} catch (IOException e) {
return null;
}
}
return oSOAPModel;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy