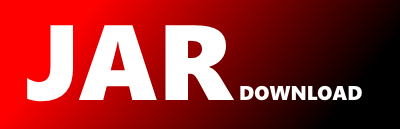
com.adobe.xfa.content.BooleanValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.content;
import com.adobe.xfa.Bool;
import com.adobe.xfa.Element;
import com.adobe.xfa.LogMessage;
import com.adobe.xfa.Node;
import com.adobe.xfa.ScriptTable;
import com.adobe.xfa.XFA;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.MsgFormatPos;
import com.adobe.xfa.ut.ResId;
/**
* An element that creates a unit of data content representing a
* boolean value.
*
* boolean-data is PCDATA that obeys the following rules: the content is '1'
* (one), representing the value true. the content is '0' (zero), representing
* the value false.
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public final class BooleanValue extends Content {
public BooleanValue(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.BOOLEAN, XFA.BOOLEAN, null,
XFA.BOOLEANTAG, XFA.BOOLEAN);
}
public boolean equals(Object object) {
if (this == object)
return true;
return super.equals(object) &&
getValue() == ((BooleanValue) object).getValue();
}
public int hashCode() {
return super.hashCode() ^ Boolean.valueOf(getValue()).hashCode();
}
/**
* @exclude from published api.
*/
public ScriptTable getScriptTable() {
return BooleanScript.getScriptTable();
}
/**
* return the Boolean value corresponding to the content of this element
*
* @return true/false
*/
public boolean getValue() {
// need to use an intermediate String otherwise
// the incorrect return value will get constructed
// on Tru64 and HP-UX (64 bit)
// this must have something to do with int to long
// conversion somewhere
String sValue = getStrValue();
try {
return Bool.getBool(sValue) == Bool.trueValue();
}
catch (ExFull ex) {
// Watson bug 1704232 since we can't update the value when ::setStrValue is called because that
// could accidentally change the value stored in the XFA Data Dom, we have to ensure we don't throw
// when the value of this object is queried.
// log a warning
MsgFormatPos message = new MsgFormatPos(ResId.FoundBadElementValueException);
message.format(sValue);
message.format(getSOMExpression());
message.format("0");
getModel().addErrorList(new ExFull(message), LogMessage.MSG_WARNING, this);
// like XFADoubleImpl and XFAFloatImpl return the default value of 0/FALSE.
return false; // default
}
}
/**
* Set the content of this element from a boolean value
*
* @param bValue
* the boolean value.
*/
public void setValue(boolean bValue) {
setStrValue(Bool.toString(bValue), true, false);
}
public String toString() {
return Bool.toString(getValue());
}
/**
* Returns true
if the current value does not legally parse
* into a boolean.
*
* @exclude from published api.
*/
public boolean valueHasTypeMismatch() {
String sValue = getStrValue();
try {
Bool.getBool(sValue);
return false;
}
catch (ExFull ex) {
return true;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy