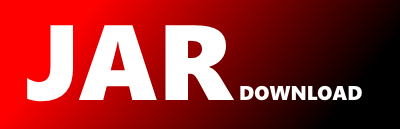
com.adobe.xfa.content.FloatValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.content;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Element;
import com.adobe.xfa.LogMessage;
import com.adobe.xfa.Node;
import com.adobe.xfa.TextNode;
import com.adobe.xfa.ScriptTable;
import com.adobe.xfa.XFA;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.MsgFormatPos;
import com.adobe.xfa.ut.Numeric;
import com.adobe.xfa.ut.ResId;
import com.adobe.xfa.ut.StringUtils;
/**
* An element that creates a unit of data content representing an floating point value.
*
* float-data is PCDATA that obeys the following rules:
* 1. no limit on the number of digits
* 2. optional leading sign
* 3. fractional digits
* 4. optional exponent
*
* @exclude from published api.
*/
public final class FloatValue extends Content {
public FloatValue(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.FLOAT, XFA.FLOAT, null,
XFA.FLOATTAG, XFA.FLOAT);
}
public boolean equals(Object object) {
if (this == object)
return true;
return super.equals(object) && getValue() == ((FloatValue) object).getValue();
}
public int hashCode() {
long bits = Double.doubleToLongBits(getValue());
return super.hashCode() ^ (int)(bits ^ (bits >>> 32));
}
public ScriptTable getScriptTable() {
return FloatScript.getScriptTable();
}
/**
* Return the value as double. Note that you should call checkValidState()
* to determine if the value was parsed properly.
*
* @return the value as double.
*/
public double getValue() {
double dVal = 0.0;
String sVal = getStrValue();
if (!StringUtils.isEmpty(sVal))
dVal = Numeric.stringToDouble(sVal, false);
return dVal;
}
/**
* Update the double value
*
* @param newValue
* new value as double
*/
public void setValue(double newValue,
boolean bNotify /* = true*/, boolean bDefault /* = false*/ /*CL#710206*/) {
String sNum = Numeric.doubleToString(newValue, 8, false);
super.setStrValue(sNum, true, false);
}
/**
* Update the double value
*
* @param sValue
* new value as string. Use this method to set the value to null.
*/
public void setValue(String sValue, boolean bFromData /* = false */
, boolean bNotify /* = true */ , boolean bDefault /* = false */ /*CL#710206*/) {
if (StringUtils.isEmpty(sValue)) {
setStrValue("", true, false);
}
else {
double dVal = Numeric.stringToDouble(sValue, true);
boolean bTypeMismatch = Double.isNaN(dVal);
if (bTypeMismatch) {
if (!getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V30_SCRIPTING)) {
MsgFormatPos oMessage = new MsgFormatPos(ResId.TypeMismatch);
oMessage.format(getSOMExpression());
oMessage.format(sValue);
getModel().addErrorList(new ExFull(oMessage), LogMessage.MSG_WARNING, this);
}
// SAP requires error in case of any mismatch and it is protected using patch-W-2447677 flag and hence
// not setting to zero in case the patch flag is set.
if (getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V29_SCRIPTING) &&
!getAppModel().getLegacySetting(AppModel.XFA_PATCH_W_2447677)) {
setValue(0.0, true, false); // legacy behavior is to set to zero
}
else {
if (bFromData) // data is king, even if it's a type mismatch
setStrValue(sValue, true, false);
// else
// ; // otherwise leave existing value unchanged
}
return;
}
setValue(dVal, true, false);
}
}
public String toString() {
return Numeric.doubleToString(getValue(), 8, false);
}
public void appendChild(Node child, boolean bValidate) {
if (child instanceof TextNode) {
TextNode textChild = (TextNode)child;
textChild.setValue(normalizeValue(textChild.getValue()), false, textChild.isDefault(true));
}
super.appendChild(child, bValidate);
}
public void insertChild(Node child, Node poRefNode, boolean bValidate) {
if (child instanceof TextNode) {
TextNode textChild = (TextNode)child;
textChild.setValue(normalizeValue(textChild.getValue()), false, textChild.isDefault(true));
}
super.insertChild(child, poRefNode, bValidate);
}
private static String formatValue(double dValue) {
return Numeric.doubleToString(dValue, 8, false);
}
private static String normalizeValue(String sValue) {
if (StringUtils.isEmpty(sValue))
return "";
else
return formatValue(Numeric.stringToDouble(sValue, false));
}
/**
* Returns true
if the current value does not legally parse
* into a float.
* @exclude from published api.
*/
public boolean valueHasTypeMismatch() {
String sVal = getStrValue();
if (StringUtils.isEmpty(sVal))
return false;
double dVal = Numeric.stringToDouble(sVal, true);
return Double.isNaN(dVal);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy