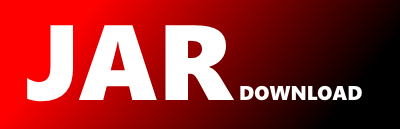
com.adobe.xfa.content.RectangleValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.content;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Element;
import com.adobe.xfa.Node;
import com.adobe.xfa.XFA;
/**
* This class implements the element in the XFA grammar.
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public final class RectangleValue extends Content {
public RectangleValue(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.RECTANGLE, XFA.RECTANGLE, null,
XFA.RECTANGLETAG, XFA.RECTANGLE);
}
public boolean equals(Object obj) {
// This implementation doesn't really support comparison of RectangleValue,
// so we force it to use reference equality semantics.
return this == obj;
}
public int hashCode() {
return System.identityHashCode(this);
}
/**
* @see Element#defaultElementImpl(int, int, boolean)
* @exclude from published api.
*/
protected Node defaultElementImpl(int eTag, int nOccurrence, boolean bAppend /* = true */) {
if (eTag == XFA.SCHEMA_DEFAULTTAG)
eTag = defaultElement();
if (eTag == XFA.SCHEMA_DEFAULTTAG)
return null;
Element e = null;
if (nOccurrence == 0) {
String className = XFA.getString(eTag);
Element parent = bAppend ? this : null;
e = getModel().createElement(parent, null, null, className,
className, null, 0, null);
}
else if (nOccurrence == 1) { // Default to 1st element
if (bAppend)
e = getElement(eTag, false, 0, false, false).clone(this, true);
else {
Element source = getElement(eTag, true, 0, false, false);
if (source != null)
e = source.clone(null, true);
else
e = getModel().createElement(eTag, null);
}
}
else if (nOccurrence == 2) { // 3rd occurrence defaults to same as 1st
// Make sure the 2nd node exists so when the 3rd node gets appended
// it's in the 3rd spot
if (bAppend) {
getElement(eTag, false, 1, false, false);
e = getElement(eTag, false, 0, false, false).clone(this, true);
}
else {
Element source = getElement(eTag, true, 0, false, false);
if (source != null)
e = source.clone(null, true);
else
e = getModel().createElement(eTag, null);
}
}
else if ((nOccurrence == 3) || getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V27_SCRIPTING)) {
// 4th property should be the same as the 2nd.
// First make sure the 3rd node exists so when the 4th gets appended
// it's in the correct position
if (bAppend) {
getElement(eTag, false, 2, false, false);
return getElement(eTag, false, 1, false, false).clone(this, true);
}
else {
// now try the first
Element source = getElement(eTag, true, 0, false, false);
if (source != null)
e = source.clone(null, true);
else
e = getModel().createElement(eTag, null);
}
}
if (e != null)
e.isTransient(true, true);
return e;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy