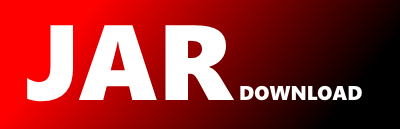
com.adobe.xfa.dom.AttrImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2009 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.dom;
import org.w3c.dom.Attr;
import org.w3c.dom.DOMException;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.TypeInfo;
import com.adobe.xfa.XFA;
import com.adobe.xfa.ut.FindBugsSuppress;
import com.adobe.xfa.ut.StringUtils;
/**
* Implements the W3C DOM Attr interface, wrapping an XFA DOM attribute.
* @exclude from published api.
*/
public class AttrImpl extends NodeImpl implements Attr {
static final int CHECK_DEFAULT = 0x1;
static final int CHECK_PREFIX = 0x2;
private final ElementImpl mOwner;
private final com.adobe.xfa.Attribute mXFAAttr;
private QNameImpl mQName;
private final String mValue;
private static final QNameImpl gTemporaryQName = new QNameImpl ("");
public com.adobe.xfa.Attribute getmXFAAttr() {
return mXFAAttr;
}
AttrImpl (ElementImpl owner, com.adobe.xfa.Attribute xfaAttr) {
super (owner);
mOwner = owner;
mXFAAttr = xfaAttr;
mValue = mXFAAttr.getAttrValue();
// debugInit ("Attribute", xfaAttr.getQName());
// debugNewChild (owner);
}
public String getLocalName() {
populate();
// debugReturn ("getLocalName", mQName.getLocalName());
return mQName.getLocalName();
}
public String getName() {
populate();
// debugReturn ("getName", mQName.getQName());
return mQName.getQName();
}
public String getNamespaceURI() {
populate();
// debugReturn ("getNamespaceURI", mQName.getNamespace());
return mQName.getNamespace();
}
public String getPrefix() {
populate();
// debugReturn ("getPrefix", mQName.getPrefix());
return mQName.getPrefix();
}
public Element getOwnerElement() {
return mOwner;
}
public TypeInfo getSchemaTypeInfo() {
return null;
}
public boolean getSpecified() {
return true;
}
public String getValue() {
// debugReturn ("getValue", mValue);
return mValue;
}
@FindBugsSuppress(code="ES")
public boolean isEqualNode(Node other) {
if (this == other) {
return true;
}
if (! (other instanceof AttrImpl)) {
return false;
}
AttrImpl otherAttr = (AttrImpl) other;
if ((mQName.getLocalName() != otherAttr.mQName.getLocalName())
|| (mQName.getNamespace() != otherAttr.mQName.getNamespace())) {
return false;
}
return StringUtils.equalsWithNull (mValue, otherAttr.mValue);
}
public boolean isId() {
return mOwner.getXFAElement().getModel().getDocument().isId(
mOwner.getNamespaceURI(),
mOwner.getLocalName(),
mQName.getNamespace() == null ? "" : mQName.getNamespace(),
mQName.getLocalName());
}
public boolean isSameNode(Node other) {
if (! (other instanceof AttrImpl)) {
return false;
}
AttrImpl otherAttr = (AttrImpl) other;
return mXFAAttr == otherAttr.mXFAAttr;
}
public void setValue(String value) throws DOMException {
throw new DOMException (DOMException.NO_MODIFICATION_ALLOWED_ERR, "");
}
public String getNodeName () {
return getName();
}
public short getNodeType() {
return ATTRIBUTE_NODE;
}
public String getNodeValue() throws DOMException {
// debugReturn ("getNodeValue", mValue);
return mValue;
}
final boolean isNamespaceAttr (int checkType) {
String prefix = mXFAAttr.getPrefix();
if ((checkType & CHECK_PREFIX) != 0) {
if (prefix == XFA.XMLNS) {
return true;
}
}
if ((checkType & CHECK_DEFAULT) != 0) {
if (StringUtils.isEmpty (prefix) && (mXFAAttr.getName() == XFA.XMLNS)) {
return true;
}
}
return false;
}
final boolean isPopulated () {
return mQName != null;
}
final void populate () {
if (mQName == null) {
doPopulate();
}
}
/*
* Populates the attribute object's fields.
*
* An attribute object is populated on first access to its contents.
* This is not for performance or resource usage reasons. Because the
* XFA DOM does not store namespace URIs for its attribute objects (the
* appropriate method always returns null), the act of population may
* need to look the URI. Looking up the URI is a parent element
* operation that requires all namespace-related attributes (xmlns)
* already be populated (an operation that doesn't require namespace
* lookup). So, the element populates its attributes in two passes.
* Namespace-related attributes are populated as the attribute objects
* are created. Non-namespace attributes are populated (subsequently)
* on demand.
*/
private void doPopulate () {
assert (mQName == null);
mQName = gTemporaryQName;
try {
String localName = mXFAAttr.getLocalName();
String prefix = mXFAAttr.getPrefix();
String namespaceURI = "";
if ((prefix != QNameImpl.XMLNS) && (localName != QNameImpl.XMLNS)) {
namespaceURI = mOwner.lookupNamespaceURI (StringUtils.isEmpty (prefix) ? null : prefix);
}
mQName = new QNameImpl (namespaceURI, prefix, localName);
} catch (Exception e) {
mQName = null;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy