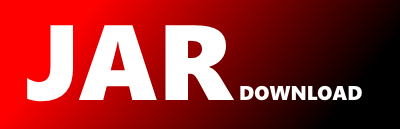
com.adobe.xfa.dom.XFANodeHolder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2009 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.dom;
import org.w3c.dom.Node;
import com.adobe.xfa.Element.DualDomNode;
/**
* Base class for all classes that wrap XFA nodes (e.g., element,
* processing instruction). Indeed, the only notable exception to using
* this as a base is the AttrImpl class, since the XFA class attribute
* is not derived from the XFA node class.
* @exclude from published api.
*/
public abstract class XFANodeHolder extends NodeImpl {
private final com.adobe.xfa.Node mXFANode;
public com.adobe.xfa.Node getmXFANode() {
return mXFANode;
}
XFANodeHolder (ParentNode parent, com.adobe.xfa.Node newNode) {
super (parent);
// Ensure that we are on the XML side
if (newNode instanceof com.adobe.xfa.Element.DualDomNode)
newNode = ((com.adobe.xfa.Element.DualDomNode)newNode).getXmlPeer();
mXFANode = newNode;
}
public Node getNextSibling() {
NodeImpl result = forceNext();
// debugReturn ("getNextSibling", result);
return result;
}
public Node getPreviousSibling() {
NodeImpl result = forcePrev();
// debugReturn ("getPreviousSibling", result);
return result;
}
public boolean isSameNode(Node other) {
if (! (other instanceof XFANodeHolder)) {
return false;
}
XFANodeHolder otherXFANodeHolder = (XFANodeHolder) other;
return mXFANode == otherXFANodeHolder.mXFANode;
}
XFANodeHolder forceNext () {
NodeImpl nextNode = getNext();
if (nextNode != null) {
assert (nextNode instanceof XFANodeHolder);
return (XFANodeHolder) nextNode;
}
ParentNode parent = getParent();
return (parent != null) ? parent.forceNext (this) : null;
}
XFANodeHolder forcePrev () {
NodeImpl prevNode = getPrev();
if (prevNode != null) {
assert (prevNode instanceof XFANodeHolder);
return (XFANodeHolder) prevNode;
}
ParentNode parent = getParent();
return (parent != null) ? parent.forcePrev (this) : null;
}
final com.adobe.xfa.Node getXFANode () {
return mXFANode;
}
static final com.adobe.xfa.Element getXFAParent (com.adobe.xfa.Node node) {
com.adobe.xfa.Element parent = node.getXMLParent();
// Jump to the XML DOM, if necessary
if (parent instanceof DualDomNode)
parent = (com.adobe.xfa.Element)((DualDomNode)parent).getXmlPeer();
return parent;
}
static XFANodeHolder createNode (ParentNode parent, com.adobe.xfa.Node source) {
// Ensure that we are on the XML side
if (source instanceof com.adobe.xfa.Element.DualDomNode)
source = ((com.adobe.xfa.Element.DualDomNode)source).getXmlPeer();
if (source instanceof com.adobe.xfa.Chars) {
return new TextImpl (parent, (com.adobe.xfa.Chars) source);
} else if (source instanceof com.adobe.xfa.Comment) {
return new CommentImpl (parent, (com.adobe.xfa.Comment) source);
} else if (source instanceof com.adobe.xfa.Document) {
return new DocumentImpl ((com.adobe.xfa.Document) source);
} else if (source instanceof com.adobe.xfa.Element) {
return new ElementImpl (parent, (com.adobe.xfa.Element) source);
} else if (source instanceof com.adobe.xfa.ProcessingInstruction) {
return new ProcessingInstructionImpl (parent, (com.adobe.xfa.ProcessingInstruction) source);
}
assert (false);
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy