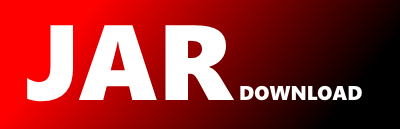
com.adobe.xfa.font.FontInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.xfa.font;
import com.adobe.fontengine.inlineformatting.css20.CSS20Attribute;
import com.adobe.fontengine.inlineformatting.css20.CSS20FontDescription;
/**
* @exclude from published api.
*/
public class FontInfo implements Comparable {
public final static int WEIGHT_UNKNOWN = 0;
public final static int WEIGHT_NORMAL = 400;
public final static int WEIGHT_BOLD = 700;
public final static int STYLE_UNKNOWN = -1;
public final static int STYLE_UPRIGHT = 0;
public final static int STYLE_ITALIC = 1;
private final static double LEGACY_SAMPLE_FONT_SIZE = 10.0; // TODO: need to understand how AFE and CoolType handle sizes/metrics
private final String mTypeface;
private final int mWeight;
private final boolean mItalic;
private final double mLegacySizeDiff;
private final int mStretchDiff;
public FontInfo () {
this("", WEIGHT_NORMAL, false, 0, 0);
}
public FontInfo (FontInfo source) {
this(source.mTypeface, source.mWeight, source.mItalic, source.mLegacySizeDiff, source.mStretchDiff);
}
public FontInfo (String typeface, int weight, boolean italic) {
this(typeface, weight, italic, 0, 0);
}
public FontInfo (String typeface, int weight, boolean italic, double legacySizeDiff, int stretchDiff) {
mTypeface = typeface;
mWeight = weight;
mItalic = italic;
mLegacySizeDiff = legacySizeDiff;
mStretchDiff = stretchDiff;
}
public String getTypeface () {
return mTypeface;
}
public int getWeight () {
return mWeight;
}
public boolean getItalic () {
return mItalic;
}
public double getLegacySizeDiff () {
return mLegacySizeDiff;
}
public int getStretchDiff () {
return mStretchDiff;
}
public boolean equals (Object object) {
if (this == object)
return true;
// This overrides Object.equals(boolean) directly, so...
if (object == null)
return false;
if (object.getClass() != getClass())
return false;
FontInfo other = (FontInfo)object;
if ((mWeight != other.mWeight) || (mItalic != other.mItalic)) {
return false;
}
return mTypeface.equals(other.mTypeface);
}
public static FontInfo createFromAFEDescription (CSS20FontDescription desc) {
boolean italic = desc.getStyle() != CSS20Attribute.CSSStyleValue.NORMAL;
return new FontInfo (desc.getFamilyName(), desc.getWeight(), italic, getLegacySizeDiff (desc), getStretchDiff (desc));
}
public static boolean match (FontInfo o1, FontInfo o2) {
if (o1 == o2) {
return true;
}
if ((o1 == null) || (o2 == null)) {
return false;
}
return o1.equals (o2);
}
public int hashCode () {
int result = mWeight;
result = result * 31 ^ Boolean.valueOf(mItalic).hashCode();
return result * 31 ^ mTypeface.hashCode();
}
public String toString () {
String result = "Typeface: " + mTypeface;
result = result + ", Weight: " + ((mWeight >= WEIGHT_BOLD) ? "bold" : "normal");
result = result + ", Italic: " + (mItalic ? "on" : "off");
return result;
}
public int compareTo (FontInfo other) {
if (other == null)
throw new NullPointerException();
int result = mTypeface.compareTo (other.mTypeface);
if (result != 0) {
return result;
}
if (mItalic != other.mItalic) {
return mItalic ? 1 : -1;
}
if (mWeight < other.mWeight) {
return -1;
} else if (mWeight > other.mWeight) {
return 1;
}
return 0;
}
static double getLegacySizeDiff (CSS20FontDescription desc) {
double minSize = desc.getLowPointSize();
double maxSize = desc.getHighPointSize();
if (LEGACY_SAMPLE_FONT_SIZE < minSize) {
return minSize - LEGACY_SAMPLE_FONT_SIZE;
} else if (LEGACY_SAMPLE_FONT_SIZE > maxSize) {
return LEGACY_SAMPLE_FONT_SIZE - maxSize;
}
return 0.0;
}
static int getStretchDiff (CSS20FontDescription desc) {
CSS20Attribute.CSSStretchValue stretch = desc.getStretch();
if ((stretch == CSS20Attribute.CSSStretchValue.SEMICONDENSED) || (stretch == CSS20Attribute.CSSStretchValue.SEMIEXPANDED)) {
return 1;
} else if ((stretch == CSS20Attribute.CSSStretchValue.CONDENSED) || (stretch == CSS20Attribute.CSSStretchValue.EXPANDED)) {
return 2;
} else if ((stretch == CSS20Attribute.CSSStretchValue.EXTRACONDENSED) || (stretch == CSS20Attribute.CSSStretchValue.EXTRAEXPANDED)) {
return 3;
} else if ((stretch == CSS20Attribute.CSSStretchValue.ULTRACONDENSED) || (stretch == CSS20Attribute.CSSStretchValue.ULTRAEXPANDED)) {
return 4;
}
return 0;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy