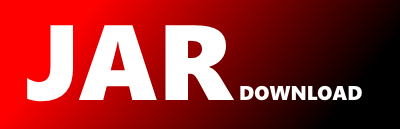
com.adobe.xfa.form.FormInstanceManagerScript Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.form;
import com.adobe.xfa.Arg;
import com.adobe.xfa.ProtoableNodeScript;
import com.adobe.xfa.Obj;
import com.adobe.xfa.Schema;
import com.adobe.xfa.ScriptFuncObj;
import com.adobe.xfa.ScriptPropObj;
import com.adobe.xfa.ScriptTable;
/**
* This class contains all the script functionality associated with the
* FormInstanceManager class. Broken out into a separate class for easier maintainability.
*
* @exclude from published api.
*/
public class FormInstanceManagerScript extends ProtoableNodeScript {
protected static final ScriptTable moScriptTable = new ScriptTable(
ProtoableNodeScript.moScriptTable,
"instanceManager",
new ScriptPropObj[] {
new ScriptPropObj(FormInstanceManagerScript.class, "min", "getMin", null, Arg.INTEGER, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_FORM_GETMININSTANCES_DESC, 0*/, 0),
new ScriptPropObj(FormInstanceManagerScript.class, "max", "getMax", null, Arg.INTEGER, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_FORM_GETMAXINSTANCES_DESC, 0*/, 0),
new ScriptPropObj(FormInstanceManagerScript.class, "count", "getCount", "setCount", Arg.INTEGER, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_FORM_COUNTINSTANCES_DESC, 0*/, 0)
},
new ScriptFuncObj[] {
new ScriptFuncObj(FormInstanceManagerScript.class, "setInstances", "setInstances", Arg.EMPTY,
new int[] { Arg.INTEGER /*,XFA_IS_FORM_SETINSTANCES_PARAM1*/}, 1, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_FORM_SETINSTANCES_DESC, 0*/, "instanceManagerPermsCheck", 0),
new ScriptFuncObj(FormInstanceManagerScript.class, "addInstance", "addInstance", Arg.OBJECT,
new int[] { Arg.BOOL /*,XFA_IS_FORM_ADDINSTANCE_PARAM1*/}, 0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_FORM_ADDINSTANCE_DESC, XFA_IS_FORM_ADDINSTANCE_RET*/, "instanceManagerPermsCheck", 0),
new ScriptFuncObj(FormInstanceManagerScript.class, "insertInstance", "insertInstance", Arg.OBJECT,
new int[] { Arg.INTEGER, Arg.BOOL /*,XFA_IS_FORM_INSERTINSTANCE_PARAM1*/ /*,XFA_IS_FORM_ADDINSTANCE_PARAM2*/}, 1, Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_FORM_INSERTINSTANCE_DESC, XFA_IS_FORM_ADDINSTANCE_RET*/, "instanceManagerPermsCheck", 0),
new ScriptFuncObj(FormInstanceManagerScript.class, "removeInstance", "removeInstance", Arg.EMPTY,
new int[] { Arg.INTEGER /*,XFA_IS_FORM_REMOVEINSTANCE_PARAM1*/}, 1, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_FORM_REMOVEINSTANCE_DESC, 0*/, "instanceManagerPermsCheck", 0),
new ScriptFuncObj(FormInstanceManagerScript.class, "moveInstance", "moveInstance", Arg.EMPTY,
new int[] { Arg.INTEGER, Arg.INTEGER /*,XFA_IS_FORM_MOVEINSTANCE_PARAM1*/ /*,XFA_IS_FORM_MOVEINSTANCE_PARAM2*/}, 2, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_FORM_MOVEINSTANCE_DESC, 0*/, "instanceManagerPermsCheck", 0)
}
);
public static ScriptTable getScriptTable() {
return moScriptTable;
}
public static void getMin(Obj obj, Arg retVal) {
retVal.setInteger(Integer.valueOf(((FormInstanceManager)obj).getMin()));
}
public static void getMax(Obj obj, Arg retVal) {
retVal.setInteger(Integer.valueOf(((FormInstanceManager)obj).getMax()));
}
public static void getCount(Obj obj, Arg retVal) {
retVal.setInteger(Integer.valueOf(((FormInstanceManager)obj).getCount()));
}
public static void setCount(Obj obj, Arg arg) {
if (arg.getArgType() != Arg.NULL)
((FormInstanceManager)obj).setInstances(arg.getInteger().intValue(), true);
}
public static void setInstances(Obj obj, Arg retVal, Arg[] args) {
((FormInstanceManager)obj).setInstances(args[0].getInteger().intValue(), true);
}
public static void addInstance(Obj obj, Arg retVal, Arg[] args) {
FormInstanceManager manager = ((FormInstanceManager)obj);
if (args.length == 0)
retVal.setObject(manager.addInstance(manager.getCount(), true));
else if (args.length == 1)
retVal.setObject(manager.addInstance(manager.getCount(), args[0].getBool().booleanValue()));
}
public static void insertInstance(Obj obj, Arg retVal, Arg[] args) {
FormInstanceManager manager = ((FormInstanceManager)obj);
if (args.length == 1)
retVal.setObject(manager.addInstance(args[0].getInteger().intValue(), true));
else if (args.length == 2)
retVal.setObject(manager.addInstance(args[0].getInteger().intValue(), args[1].getBool().booleanValue()));
}
public static void removeInstance(Obj obj, Arg retVal, Arg[] args) {
((FormInstanceManager)obj).removeInstance(args[0].getInteger().intValue(), true);
}
public static void moveInstance(Obj obj, Arg retVal, Arg[] args) {
((FormInstanceManager)obj).moveInstance(args[0].getInteger().intValue(), args[1].getInteger().intValue(), true);
}
// ************** script perms funcs ****************
public static boolean instanceManagerPermsCheck(Obj obj, Arg[] args) {
if (obj instanceof FormInstanceManager) {
if (!((FormInstanceManager)obj).instanceManagerPermsCheck())
return false;
}
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy