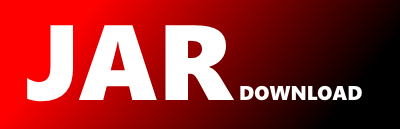
com.adobe.xfa.form.FormSubform Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.form;
import com.adobe.xfa.DependencyTracker;
import com.adobe.xfa.Element;
import com.adobe.xfa.Node;
import com.adobe.xfa.XFA;
import com.adobe.xfa.XFAList;
import com.adobe.xfa.data.DataNode;
import com.adobe.xfa.template.containers.Subform;
import com.adobe.xfa.ut.Peer;
import java.util.ArrayList;
import java.util.List;
/**
* @exclude from public api.
*/
public class FormSubform extends Subform {
private FormInstanceManager mInstanceManager;
private boolean mbIsLayoutNode; // is a leader or trailer subform;
private FormDataListener mFormDataListener;
private List mListenerTable; // list of listeners
private boolean mbValidateRegistered;
private boolean mbCalculateRegistered;
/**
* Instantiates a FormSubform container.
* @param parent the FormSubform's parent, if any.
* @param prevSibling the field's previous sibling, if any.
*/
public FormSubform(Element parent, Node prevSibling) {
super(parent, prevSibling);
}
/**
* Returns the InstanceManager for this subform
* @return an FormInstanceManager
*/
public FormInstanceManager getInstanceManager() {
return mInstanceManager;
}
/**
* Get the data node bound to this Form node
* @return The DataNode bound to this Form Node
*/
public DataNode getDataNode() {
if (null != mFormDataListener)
return (DataNode)(mFormDataListener.getDataNode());
return null;
}
/**
* @exclude from published api.
*/
public void getDeltas(Element delta, XFAList list) {
if (! isSameClass(delta))
return;
FormModel model = (FormModel)getModel();
if (model != null && model.isLoading()) {
// restore validate.disableAll if we are loading
model.restoreValidateDisableAll(this, delta);
}
super.getDeltas(delta, list);
}
/**
* @see Element#setElement(Node, int, int)
*/
public Node setElement(Node child, int eTag, int nOccurrence) {
if (eTag == XFA.OCCURTAG) {
FormInstanceManager manager = getInstanceManager();
if (manager != null)
manager.setElement(child, eTag, nOccurrence);
}
return super.setElement(child, eTag, nOccurrence);
}
void setDataNode(DataNode dataNode, boolean bPeerNode /* = true */) {
// Type and null check
if (dataNode == null || dataNode.getClassTag() != XFA.DATAGROUPTAG)
return;
if (bPeerNode) {
// remove old listener
if (mFormDataListener != null) {
mFormDataListener.dispose();
mFormDataListener = null;
}
// Establish a peer relationship with the data node
mFormDataListener = new FormDataListener(this, dataNode);
}
}
void setInstanceManager(FormInstanceManager instanceManager) {
mInstanceManager = instanceManager;
}
/**
* @exclude from public api.
*/
protected int getInstanceIndex(DependencyTracker dependencyTrack /* = null */) {
if (mInstanceManager != null) {
if (dependencyTrack != null)
dependencyTrack.addDependency(mInstanceManager);
return mInstanceManager.findIndex(this);
}
return 0;
}
/**
* @exclude from public api.
*/
public void setInstanceIndex(int nMoveTo) {
// Form method only
if (mInstanceManager != null) {
int nIndex = mInstanceManager.findIndex(this);
mInstanceManager.moveInstance(nIndex, nMoveTo, true);
}
}
List getFormListeners(boolean bCreate) {
if (bCreate && mListenerTable == null)
mListenerTable = new ArrayList();
return mListenerTable;
}
// clean up the data node
void cleanupListeners() {
if (mFormDataListener != null) {
mFormDataListener.dispose();
mFormDataListener = null;
}
if (mListenerTable != null) {
mListenerTable.clear();
mListenerTable = null;
}
}
// check if this subform is a subform generated by layout (eg a leader or trailer)
boolean isLayoutNode() {
return mbIsLayoutNode;
}
// check if this subform is a subform generated by layout (eg a leader or trailer)
void setLayoutNode() {
mbIsLayoutNode = true;
}
/**
* @exclude from public api.
*/
public void execEvent(String sActivity) {
FormModel formModel = (FormModel)getModel();
formModel.eventOccurred(sActivity, this);
}
/**
* @exclude from public api.
*/
public boolean execValidate() {
FormModel formModel = (FormModel)getModel();
FormModel.Validate validate = formModel.getDefaultValidate();
formModel.validate(validate, this, true, false);
if (validate != null && validate.getFailCount() > 0)
return false;
return true;
}
public boolean getRegistered(int eActivity) {
if (eActivity == XFA.VALIDATETAG)
return mbValidateRegistered;
else if (eActivity == XFA.CALCULATETAG)
return mbCalculateRegistered;
return false;
}
void setRegistered(int eActivity) {
if (eActivity == XFA.VALIDATETAG)
mbValidateRegistered = true;
else if (eActivity == XFA.CALCULATETAG)
mbCalculateRegistered = true;
}
/**
* @exclude from public api.
*/
public void notifyPeers(int eventType,
String arg1,
Object pArg2) {
// XFA doesn't notify of changes during loads, there is no need and it improves load performance
if (getModel() != null && getModel().isLoading())
return;
// If validate changes at run time and there are no validations are
// registered for this node, then we need to register it so that validations
// will fire.
if (!mbValidateRegistered && pArg2 instanceof FormModel.Validate) {
if (eventType == Peer.DESCENDENT_ATTR_CHANGED ||
eventType == Peer.DESCENDENT_ADDED) {
FormModel poFormModel = (FormModel)getModel();
poFormModel.registerEvents(this, FormModel.XFAEVENTTYPE_VALIDATE);
}
}
super.notifyPeers(eventType, arg1, pArg2);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy