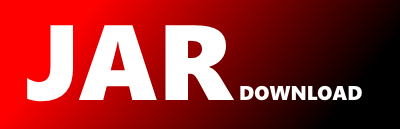
com.adobe.xfa.formcalc.DebugHost Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.formcalc;
import java.util.List;
/**
* Class DebugHost defines the interface by which the
* {@link CalcParser} (FormCalc scripting engine)
* can interact with a debugger host.
*
* In environments where this is possible and desired,
* applications will need to inform the FormCalc script engine
* that they are capable of supporting the DebugHost
* interface by invoking the
* {@link CalcParser#setDebugHost(DebugHost)}
* method.
*
*
Environments that are not able to debug
* need not define nor install a DebugHost objects.
*
* @author Darren Burns
*
* @exclude from published api.
*/
public interface DebugHost {
/**
* Enumeration of all debugging commands that can be used
* to control the FormCalc scripting engine when debugging.
*/
public static final int STEP_OVER = 0;
public static final int STEP_INTO = 1;
public static final int STEP_OUT = 2;
public static final int STOP = 3;
/**
* Abstract method that is called when the engine stops in debug mode.
*
* @param nScriptID the ID of script, which had been set via
* {@link CalcParser#setScriptID(int)}.
* @param nLine the line of script at which execution has stopped.
* @return currently not used.
*/
int stopped(int nScriptID, int nLine);
/**
* Abstract method that is called periodically during script execution.
*/
void poll();
/**
* Abstract method that sets or clears a breakpoint.
*
* @param oParser the parser to affect.
* @param nScriptID the ID of script, which had been set via
* {@link CalcParser#setScriptID(int)}.
* @param nLine the line of script.
* @param bSet true if setting breakpoint; false if clearing it.
* @return true if successful.
*/
boolean breakPoint(CalcParser oParser,
int nScriptID, int nLine, boolean bSet);
/**
* Abstract method that allows for single-stepping. Note that this
* call does not actually cause any script to be executed. Rather,
* it sets the internal state so that the next time the appropriate
* condition is met, the Stopped callback will be called.
*
* @param oParser the parser to affect.
* @param eCmd the command code (eg. step over/step into/step out).
* @return true if successful.
*/
boolean command(CalcParser oParser, int eCmd);
/**
* Abstract method that returns a new-line delimited string
* showing the current stack and parameter values.
*
* @param oParser the parser to query.
* @return a new-line delimited string showing the current
* stack and parameter values.
*/
String getStackTrace(CalcParser oParser);
/**
* Abstract method that returns parallel lists of variables/parameters
* and values (the lists are the same size). The symbols are not copied,
* so they must not be stored for later use. Note that it's only
* meaningful to call this function from within a break-point.
*
* @param oParser the parser to query.
* @param oNames returned list of variable/parameter names.
* @param oValues returned list of values.
*/
void getVariables(CalcParser oParser, List oNames, List oValues);
}