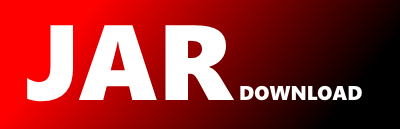
com.adobe.xfa.formcalc.Stack Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.formcalc;
/**
* Class Stack defines the runtime stack that's used by
* the FormCalc scripting engine.
* Specifically, the FormCalc interpreter is a stack machine;
* it doesn't have general purpose registers. Rather all
* intermediate values are preserved on a stack.
*
* For example, when the FormCalc interpreter performs an add
* instruction, it will pop two operands off the stack, add them
* together, and push the result onto the stack.
*
* @author Paul Imerson, Mike P. Tardif
*
* @exclude from published api.
*/
public final class Stack {
static final int SIZE = 64; // initial size of stack
private int mnStackSize; // size of stack.
private CalcSymbol[] moStackBase; // stack of CalcSymbols.
private int moStackPtr; // stack pointer.
/**
* Instantiate a Stack object.
*/
Stack() {
// empty
}
/**
* Create storage for this Stack object.
* @param nStackSize an initial size for the stack.
* @return integer 1 upon success, and 0 otherwise.
*/
int create(int nStackSize /* = SIZE */) {
mnStackSize = nStackSize;
moStackBase = new CalcSymbol[mnStackSize];
moStackPtr = 0;
return 1;
}
/**
* Reinitialize this Stack object.
*/
void init() {
for (int i = 0; i < mnStackSize; i++)
moStackBase[i] = null;
moStackPtr = 0;
}
/**
* Pops the symbol on top of this Stack object.
* @return the symbol on top of stack. The symbol is pop off the stack.
*/
CalcSymbol pop() {
assert(moStackPtr > 0);
return moStackBase[--moStackPtr];
}
/**
* Pushes the given symbol onto this Stack object.
* @param sym the symbol to push onto the stack.
*/
void push(CalcSymbol sym) {
//
// grow Stack as needed.
//
if (moStackPtr >= mnStackSize) {
mnStackSize <<= 1;
CalcSymbol[] oNewBase = new CalcSymbol[mnStackSize];
System.arraycopy(moStackBase, 0, oNewBase, 0, mnStackSize >> 1);
moStackBase = oNewBase;
moStackPtr = mnStackSize >> 1;
}
moStackBase[moStackPtr++] = sym;
}
/**
* Gets the relative offset of this object's stack pointer from its base.
* Use this method instead of the stack pointer because the stack may
* get relocated at runtime, whereas the offset in invariant.
* @return the stack pointer's relative offset.
*/
int getOffset() {
return moStackPtr;
}
/**
* Peeks at a symbol within this Stack object.
* @param nOffset an offset within the stack.
* @return the symbol within the stack.
*/
public CalcSymbol peek(int nOffset) {
assert (0 <= nOffset && nOffset <= moStackPtr);
return moStackBase[nOffset];
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy