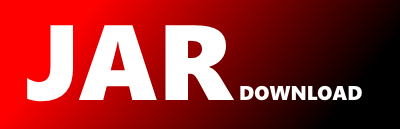
com.adobe.xfa.gfx.GFXColour Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
//----------------------------------------------------------------------
//
// ADOBE CONFIDENTIAL
// __________________
//
// Copyright 1995 - 2004 Adobe Systems Incorporated. All
// Rights Reserved.
//
// NOTICE: All information contained herein is, and remains
// the property of Adobe Systems Incorporated and its
// suppliers, if any. The intellectual and technical
// concepts contained herein are proprietary to Adobe Systems
// Incorporated and its suppliers and may be covered by U.S.
// and Foreign Patents, patents in process, and are protected
// by trade secret or copyright law. Dissemination of this
// information or reproduction of this material is strictly
// forbidden unless prior written permission is obtained from
// Adobe Systems Incorporated.
//
//----------------------------------------------------------------------
package com.adobe.xfa.gfx;
/**
* The graphic colour object is a convinience class for basic colour handling.
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public class GFXColour {
public final static int JF_GFXCOLOUR_DEF_SCALE = 255;
/**
* enumeration StandardColour: supported standard colours.
*/
public final static int BLACK_INDEX = 0;
public final static int WHITE_INDEX = 1;
public final static int LIGHTGRAY_INDEX = 2;
public final static int GRAY_INDEX = 3;
public final static int DARKGRAY_INDEX = 4;
public final static int OTHER_INDEX = 5;
public final static GFXColour BLACK = new GFXColour (0, 0, 0);
public final static GFXColour WHITE = new GFXColour (255, 255, 255);
public final static GFXColour LIGHTGRAY = new GFXColour (192, 192, 192);
public final static GFXColour GRAY = new GFXColour (128, 128, 128);
public final static GFXColour DARKGRAY = new GFXColour (64, 64, 64);
private final int mnRed; // current red value associated with the colour.
private final int mnGreen; // current green value associated with the colour.
private final int mnBlue; // current blue value associated with the colour.
private final int mnScale; // current scale to apply to RGB triple.
/**
* Default constructor.
*
* Assigns the colour object with the following values:
*
* Red = 0
* Green = 0
* Blue = 0
* Scale = 255
*
*/
public GFXColour () {
mnRed = 0;
mnGreen = 0;
mnBlue = 0;
mnScale = JF_GFXCOLOUR_DEF_SCALE;
}
/**
* Copy consturctor.
* @param oSource - Source attribute object to copy
*/
public GFXColour (GFXColour oSource) {
mnRed = oSource.mnRed;
mnGreen = oSource.mnGreen;
mnBlue = oSource.mnBlue;
mnScale = oSource.mnScale;
}
/**
* Constructor.
*
* Creates a Colour object with the values as specified
* by the input values.
* @param lNewR - Colour Red value
* @param lNewG - Colour Green value
* @param lNewB - Colour Blue value
* @param lNewScale - Scale setting
*/
public GFXColour (int lNewR, int lNewG, int lNewB, int lNewScale) {
if (lNewScale <= 0) {
mnScale = JF_GFXCOLOUR_DEF_SCALE;
}
else {
mnScale = lNewScale;
}
mnRed = range (lNewR, mnScale);
mnGreen = range (lNewG, mnScale);
mnBlue = range (lNewB, mnScale);
}
public GFXColour (int lNewR, int lNewG, int lNewB) {
this (lNewR, lNewG, lNewB, 255);
}
/**
* Constructor.
*
* Set the all the colour values based on a text string.
* @param sColour - A string containing three integer values representing
* red, green and blue.
*/
public GFXColour (String sColour) {
int nStart = 0, nEnd;
String sRGB;
int lRValue, lGValue, lBValue;
nEnd = sColour.indexOf (' ');
sRGB = sColour.substring (nStart, nEnd);
lRValue = Integer.parseInt (sRGB);
nStart = nEnd + 1;
nEnd = sColour.indexOf (' ', nStart);
sRGB = sColour.substring (nStart, nEnd);
lGValue = Integer.parseInt (sRGB);
nStart += nEnd + 1;
nEnd = sColour.length();
sRGB = sColour.substring (nStart, nEnd);
lBValue = Integer.parseInt (sRGB);
mnScale = 255;
mnRed = lRValue;
mnGreen = lGValue;
mnBlue = lBValue;
}
public static GFXColour create (int lNewR, int lNewG, int lNewB, int lNewScale) {
if ((lNewR == BLACK.r())
&& (lNewG == BLACK.g())
&& (lNewB == BLACK.b())
&& (lNewScale == BLACK.scale())) {
return BLACK;
}
if ((lNewR == WHITE.r())
&& (lNewG == WHITE.g())
&& (lNewB == WHITE.b())
&& (lNewScale == WHITE.scale())) {
return WHITE;
}
if ((lNewR == LIGHTGRAY.r())
&& (lNewG == LIGHTGRAY.g())
&& (lNewB == LIGHTGRAY.b())
&& (lNewScale == LIGHTGRAY.scale())) {
return LIGHTGRAY;
}
if ((lNewR == GRAY.r())
&& (lNewG == GRAY.g())
&& (lNewB == GRAY.b())
&& (lNewScale == GRAY.scale())) {
return GRAY;
}
if ((lNewR == DARKGRAY.r())
&& (lNewG == DARKGRAY.g())
&& (lNewB == DARKGRAY.b())
&& (lNewScale == DARKGRAY.scale())) {
return DARKGRAY;
}
return new GFXColour (lNewR, lNewG, lNewB, lNewScale);
}
public static GFXColour create (String sColour) {
return new GFXColour (sColour);
}
// Some Standard Colour Definitions
/**
* Get a Black colour object.
*
* This will return a Colour object with the following settings:
*
* Red = 0
* Green = 0
* Blue = 0
* Scale = 255
*
* @return A Colour object with black settings
*/
public static GFXColour black () {
return BLACK;
}
/**
* Get a White colour object.
*
* This will return a Colour object with the following settings:
*
* Red = 255
* Green = 255
* Blue = 255
* Scale = 255
*
* @return A Colour object with white settings
*/
public static GFXColour white () {
return WHITE;
}
/**
* Get a Light gray colour object.
*
* This will return a Colour object with the following settings:
*
* Red = 192
* Green = 192
* Blue = 192
* Scale = 255
*
* @return A Colour object with light gray settings
*/
public static GFXColour lightGray () {
return LIGHTGRAY;
}
/**
* Get a Gray colour object.
*
* This will return a Colour object with the following settings:
*
* Red = 128
* Green = 128
* Blue = 128
* Scale = 255
*
* @return A Colour object with gray settings
*/
public static GFXColour gray () {
return GRAY;
}
/**
* Get a Dark gray colour object.
*
* This will return a Colour object with the following settings:
*
* Red = 64
* Green = 64
* Blue = 64
* Scale = 255
*
* @return A Colour object with dark gray settings
*/
public static GFXColour darkGray () {
return DARKGRAY;
}
/**
* Get one of the predefined standard colour objects.
* @param colourIndex - the requested colour as a StandardColour enumeration.
* @return The requested standard colour object.
*/
public static GFXColour getStandardColour (int colourIndex) {
switch (colourIndex) {
case BLACK_INDEX:
return BLACK;
case WHITE_INDEX:
return WHITE;
case LIGHTGRAY_INDEX:
return LIGHTGRAY;
case GRAY_INDEX:
return GRAY;
case DARKGRAY_INDEX:
return DARKGRAY;
}
return null;
}
/**
* Get the defined standard colour as a StandardColour enumeration.
* @param oColour - the colour object
* @return The standard colour enumeration value
*/
public static int getStandardColourIndex (GFXColour oColour) {
if (oColour.equivalent (BLACK)) {
return BLACK_INDEX;
} else if (oColour.equivalent (WHITE)) {
return WHITE_INDEX;
} else if (oColour.equivalent (DARKGRAY)) {
return DARKGRAY_INDEX;
} else if (oColour.equivalent (LIGHTGRAY)) {
return LIGHTGRAY_INDEX;
} else if (oColour.equivalent (GRAY)) {
return GRAY_INDEX;
} else {
return OTHER_INDEX;
}
}
/**
* Check if a Colour object is set to black.
* @param oColour - the colour object
* @return TRUE if colour object is set to black; otherwise FALSE
*/
public static boolean isBlack (GFXColour oColour) {
return (oColour.r() == 0 && oColour.g() == 0 && oColour.b() == 0);
}
/**
* Check if a Colour object is set to white.
* @param oColour - the colour object
* @return TRUE if colour object is set to white; otherwise FALSE
*/
public static boolean isWhite (GFXColour oColour) {
int nScale = oColour.scale();
return (oColour.r() == nScale && oColour.g() == nScale && oColour.b() == nScale);
}
/**
* Get the current setting for Red.
* @return The Red value
*/
public int r () {
return mnRed;
}
/**
* Get the current setting for Green.
* @return The Green value
*/
public int g () {
return mnGreen;
}
/**
* Get the current setting for Blue.
* @return The Blue value
*/
public int b () {
return mnBlue;
}
/**
* Checks if this colour maps to white in a black and white scheme.
* @return TRUE if this colour maps to white in a black and white scheme,
* FALSE if it maps to black.
*/
public boolean whiteMono () {
return (normalR() + normalG() + normalB()) > 1.5;
}
/**
* Get the normalized value for Red.
* @return The normalized value for Red
*/
public double normalR () {
double dRed = mnRed;
double dScale = mnScale;
return dRed / dScale;
}
/**
* Get the normalized value for Green.
* @return The normalized value for Green
*/
public double normalG () {
double dGreen = mnGreen;
double dScale = mnScale;
return dGreen / dScale;
}
/**
* Get the normalized value for Blue.
* @return The normalized value for Blue
*/
public double normalB () {
double dBlue = mnBlue;
double dScale = mnScale;
return dBlue / dScale;
}
/**
* Get the scale setting for this colour object.
* @return The scale setting
*/
public int scale () {
return mnScale;
}
public GFXColour scale (int newScale) {
if (newScale == mnScale) {
return this;
}
double scaleScale = ((double) newScale) / ((double) mnScale);
return new GFXColour ((int) Math.round (scaleScale * mnRed),
(int) Math.round (scaleScale * mnGreen),
(int) Math.round (scaleScale * mnBlue),
newScale);
}
/**
* Equality comparison.
*
* Two colour objects are considered equal if all their
* values compare for equality.
* @param oCompare - Colour object to compare against.
* @return TRUE if all members are equal, FALSE otherwise.
*/
public boolean equivalent (GFXColour oCompare) {
if ((mnScale == oCompare.mnScale)
&& (mnRed == oCompare.mnRed)
&& (mnBlue == oCompare.mnBlue)
&& (mnGreen == oCompare.mnGreen)) {
return true;
}
double lhs;
double rhs;
lhs = normalR();
rhs = oCompare.normalR();
if (lhs != rhs) {
return false;
}
lhs = normalG();
rhs = oCompare.normalG();
if (lhs != rhs) {
return false;
}
lhs = normalB();
rhs = oCompare.normalB();
if (lhs != rhs) {
return false;
}
return true;
}
/**
* Equality comparison operator.
*
* Two colour objects are considered equal if all their
* values compare for equality.
* @param object the object to compare against.
* @return TRUE if all members are equal, FALSE otherwise.
*/
public boolean equals (Object object) {
if (this == object)
return true;
// This overrides Object.equals(boolean) directly, so...
if (object == null)
return false;
if (object.getClass() != getClass())
return false;
GFXColour colour = (GFXColour) object;
return equivalent(colour);
}
public int hashCode () {
int hash = 19;
long color = Double.doubleToLongBits(normalR());
hash = (hash * 31) ^ (int) (color ^ (color >>> 32));
color = Double.doubleToLongBits(normalR());
hash = (hash * 31) ^ (int) (color ^ (color >>> 32));
color = Double.doubleToLongBits(normalR());
hash = (hash * 31) ^ (int) (color ^ (color >>> 32));
return hash;
}
/**
* Non-equality comparison operator.
*
* Two colour objects are considered equal if all their
* values compare for equality.
* @param oCompare - Colour object to compare against.
* @return TRUE if any members are different, FALSE otherwise.
*/
public boolean notEqual (GFXColour oCompare) {
return ! equals (oCompare);
}
/**
* Get the converted grayscale value of the RGB triplet
* @return converted grayscale value of the RGB triplet
*/
public int getGrayScale () {
return (((mnRed * 30) + (mnGreen * 59) + (mnBlue * 11)) / 100);
}
/**
* Get the converted grayscale value of the RGB triplet as a percentage
* @return converted grayscale value of the RGB triplet as a percentage
*/
public double getGrayRate () {
return ((mnScale - getGrayScale()) / 2.55); // TODO: works only for mlScale == 255
}
public static GFXColour weightedAverage (GFXColour base, GFXColour weighted, double weight) {
return new GFXColour (weighComponent (base.normalR(), weighted.normalR(), weight, weighted.scale()),
weighComponent (base.normalG(), weighted.normalG(), weight, weighted.scale()),
weighComponent (base.normalB(), weighted.normalB(), weight, weighted.scale()),
weighted.scale());
}
private static int weighComponent (double base, double weighted, double weight, int scale) {
return (int) Math.round ((base + (weight * (weighted - base))) * scale);
}
// ---------------------------------------------------------------------------
// Range
//
// Returns an in-range (i.e. between 0 and lScale) version of the colour
// component passed in.
// ---------------------------------------------------------------------------
private int range (int lColour, int lScale) {
if (lColour < 0) {
return 0;
}
if (lColour > lScale) {
return lScale;
}
return lColour;
}
}