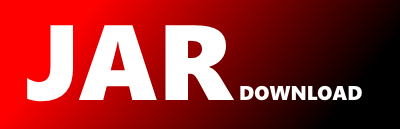
com.adobe.xfa.gfx.GFXDriver Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
package com.adobe.xfa.gfx;
import com.adobe.xfa.font.FontInstance;
import com.adobe.xfa.font.FontService;
import com.adobe.xfa.ut.Angle;
import com.adobe.xfa.ut.CoordPair;
import com.adobe.xfa.ut.Rect;
import com.adobe.xfa.ut.UnitSpan;
import java.util.ArrayList;
import java.util.List;
/**
* A base class used by the
* text and gfx packages to render/position objects
* within their respective environment.
*
* @exclude from published api.
*/
public abstract class GFXDriver {
private static class AnglePair {
public AnglePair(Angle angle, CoordPair point) {
this.angle = angle;
this.point = point;
}
final Angle angle;
final CoordPair point;
}
/*
* enumeration int: Specifies the current mode.
*
* MODE_OPAQUE - Opaque mode
* MODE_TRANSPARENT - Transparent mode
*/
/**
* @exclude from published api.
*/
public static final int MODE_OPAQUE = 0;
/**
* @exclude from published api.
*/
public static final int MODE_TRANSPARENT = 1;
/*
* enumeration CapCode: Specifies the current device capabilities.
*
* TYPE_PRINTER - Printer device
* CAP_TYPE - ?? as integer
* CAP_PIX_WIDTH - Device width in pixels as int
* CAP_PIX_HEIGHT - Device height in pixels as int
* CAP_REAL_WIDTH - Device width as a UnitSpan
* CAP_REAL_HEIGHT - Device heigth as a UnitSpan
*
*/
/**
* @exclude from published api.
*/
public static final int TYPE_PRINTER = 0;
/**
* @exclude from published api.
*/
public static final int TYPE_SCREEN = 1;
/**
* @exclude from published api.
*/
public static final int CAP_TYPE = 2;
/**
* @exclude from published api.
*/
public static final int CAP_PIX_WIDTH = 3;
/**
* @exclude from published api.
*/
public static final int CAP_PIX_HEIGHT = 4;
/**
* @exclude from published api.
*/
public static final int CAP_REAL_WIDTH = 5;
/**
* @exclude from published api.
*/
public static final int CAP_REAL_HEIGHT = 6;
/**
* @exclude from published api.
*/
public static final int DRAW_2D = 0;
/**
* @exclude from published api.
*/
public static final int DRAW_3D = 1;
/**
* @exclude from published api.
*/
public static final int DRAW_WELL = 2;
/**
* @exclude from published api.
*/
public static final int ACTIVE = 0;
/**
* @exclude from published api.
*/
public static final int INACTIVE = 1;
/**
* @exclude from published api.
*/
public static final int PUSHED = 2;
/**
* Character to glyph mapping level required by the driver.
*
* Some driver implementations do more than rendering; they may need to
* reconstiture the original text and understand how it relates to the
* rendered glyphs. This enumeration allows a driver to indicate what
* sort of character/glyph mapping it requires. If the caller is using
* the driver to render text, it must provide mapping information in
* accordance with what the driver needs.
*
* -
* MAPPING_SUPPRESS: No character/glyph mapping is required.
*
* -
* MAPPING_FULL: Full mapping information must be provided by the
* caller. Note that there may be a performance hit if the caller must
* generate full mapping information when it is not required by the
* driver.
*
* -
* MAPPING_LEGACY: The caller must simpler, legacy mapping information.
*
*
*
* @exclude from published api.
*/
public static final int MAPPING_SUPPRESS = 0;
/**
* @exclude from published api.
*/
public static final int MAPPING_FULL = 1;
/**
* @exclude from published api.
*/
public static final int MAPPING_LEGACY = 2;
private static int FU_PER_INCH = UnitSpan.unitsPerInch (UnitSpan.INCHES_72K);
private GFXEnv mpoEnv;
// Member Variables for Coordinate Translation
private int mlDUInchX; // Device Units Per Inch - X Axis
private int mlDUInchY; // Device Units Per Inch - Y Axis
private int mlDUOffsetX; // Non-Printable X Offset
private int mlDUOffsetY; // Non-Printable Y Offset
private int mlDUOriginX; // Device Origin X Coordinate
private int mlDUOriginY; // Device Origin Y Coordinate
private double mdXScale;
private double mdYScale;
private double mdScale; // Legacy weird scale
// private int mlScale; // Attempt to store scale as a int
// // integer so we can do integer
// // arithmetic where possible.
private CoordPair moOrigin; // Form Unit Origin
private CoordPair moAbsPosition; // Current absolute position
private boolean mb3dEffects;
private int meMode;
private GFXLineAttr mpoLineAttr;
private GFXFillAttr mpoFillAttr;
private GFXTextAttr mpoTextAttr;
private FontInstance moFontInstance;
// Member Variables for Relative Placement Stacks and Cacheing
/**
* @exclude from published api.
*/
public List moOffsetStack;
/**
* @exclude from published api.
*/
public List moAngleStack;
/**
* @exclude from published api.
*/
public List moClipStack;
// public Storage moCache;
// private int mnCacheSize;
// Have graphic variables around so we can get default values.
private static final GFXLineAttr moDefaultLineAttr = GFXLineAttr.BLACK_LINE;
private static final GFXFillAttr moDefaultFillAttr = GFXFillAttr.WHITE_FILL;
private static final GFXTextAttr moDefaultTextAttr = GFXTextAttr.defaultTextAttr;
// Member variable for Glyph Orientation
private int meGlyphOrientation;
/**
* @exclude from published api.
*/
public GFXDriver (GFXEnv env) {
mpoEnv = env;
mdXScale = 1.0;
mdYScale = 1.0;
mdScale = 1.0;
// mlScale = 1;
moOrigin = CoordPair.zeroZero();
meMode = MODE_TRANSPARENT;
// mnCacheSize = DEF_CACHE_SIZE;
meGlyphOrientation = GFXGlyphOrientation.HORIZONTAL;
}
/**
* @exclude from published api.
*/
public GFXEnv env () {
return mpoEnv;
}
/**
* @exclude from published api.
*/
public int devW (UnitSpan oWidth) {
checkInitialized();
int oWMI = UnitSpan.convertUnit (UnitSpan.INCHES_72K, oWidth.units(), oWidth.value());
// if (mlScale == -1) {
return devW (oWMI);
// } else {
// return JfApplyFactor (oWMI.value(), mlDUInchX * mlScale, FU_PER_INCH);
// }
}
/**
* @exclude from published api.
*/
public int devH (UnitSpan oHeight) {
checkInitialized();
int oHMI = UnitSpan.convertUnit (UnitSpan.INCHES_72K, oHeight.units(), oHeight.value());
// if (mlScale == -1) {
return devH (oHMI);
// } else {
// return JfApplyFactor (oHMI.value(), mlDUInchY * mlScale, FU_PER_INCH);
// }
}
/**
* @exclude from published api.
*/
public GFXDevPoint devPoint (CoordPair oPoint) {
return new GFXDevPoint (devX (oPoint.x()), devY (oPoint.y()));
}
/**
* @exclude from published api.
*/
public GFXDevRect devRect (Rect oRect) {
return new GFXDevRect (devX (oRect.left()),
devY (oRect.top()),
devX (oRect.right()),
devY (oRect.bottom()));
}
/**
* @exclude from published api.
*/
public int devX (UnitSpan oX) {
checkInitialized();
int oXMI = UnitSpan.convertUnit (UnitSpan.INCHES_72K, oX.units(), oX.value());
// if (mlScale == -1) {
return devW (oXMI - moOrigin.x().value()) + mlDUOriginX;
// } else {
// return JfApplyFactor (oXMI.value() - moOrigin.X().value(), mlDUInchX * mlScale, FU_PER_INCH) + mlDUOriginX;
// }
}
/**
* @exclude from published api.
*/
public int devY (UnitSpan oY) {
checkInitialized();
int oYMI = UnitSpan.convertUnit (UnitSpan.INCHES_72K, oY.units(), oY.value());
// if (mlScale == -1) {
return devH (oYMI - moOrigin.y().value()) + mlDUOriginY;
// } else {
// return JfApplyFactor (oYMI.value() - moOrigin.Y().value(), mlDUInchY * mlScale, FU_PER_INCH) + mlDUOriginY;
// }
}
/**
* @exclude from published api.
*/
public UnitSpan devUnitX () {
return new UnitSpan (UnitSpan.INCHES_72K, FU_PER_INCH / mlDUInchX);
}
/**
* @exclude from published api.
*/
public UnitSpan devUnitY () {
return new UnitSpan (UnitSpan.INCHES_72K, FU_PER_INCH / mlDUInchY);
}
/**
* @exclude from published api.
*/
public void fit (double dScale, CoordPair oOrigin, int nFit) {
if (dScale != mdScale) {
mdScale = (dScale > 0) ? dScale : 1.0;
mdXScale = dScale;
mdYScale = dScale;
// We'd like to use a int integer to express scale. See if we lose
// precision when we convert to/from int integer.
// mlScale = (int) mdScale;
// if (fabs (mdScale - (double) mlScale) > 0.000001) {
// mlScale = -1;
// }
scaleChanged();
}
moOrigin = new CoordPair (new UnitSpan (UnitSpan.INCHES_72K, oOrigin.x().units(), oOrigin.x().value()),
new UnitSpan (UnitSpan.INCHES_72K, oOrigin.y().units(), oOrigin.y().value()));
int lWidth = width();
if ((nFit & GFXEnv.FIT_CENTRE) == GFXEnv.FIT_CENTRE) {
mlDUOriginX = (lWidth + 2 * mlDUOffsetX) / 2;
} else if ((nFit & GFXEnv.FIT_RIGHT) == GFXEnv.FIT_RIGHT) {
mlDUOriginX = lWidth + mlDUOffsetX;
} else {
mlDUOriginX = -mlDUOffsetX;
}
int lHeight = height();
if ((nFit & GFXEnv.FIT_MIDDLE) == GFXEnv.FIT_MIDDLE) {
mlDUOriginY = (lHeight + 2 * mlDUOffsetY) / 2;
} else if ((nFit & GFXEnv.FIT_BOTTOM) == GFXEnv.FIT_BOTTOM) {
mlDUOriginY = lHeight + mlDUOffsetY;
} else {
mlDUOriginY = -mlDUOffsetY;
}
}
/**
* @exclude from published api.
*/
public CoordPair rotatePoint (CoordPair oPoint) {
CoordPair oRP = rotationPoint (false);
int oX = UnitSpan.convertUnit (UnitSpan.INCHES_72K, oPoint.x().units(), oPoint.x().value());
int oY = -UnitSpan.convertUnit (UnitSpan.INCHES_72K, oPoint.y().units(), oPoint.y().value());
int oRPX = UnitSpan.convertUnit (UnitSpan.INCHES_72K, oRP.x().units(), oRP.x().value());
int oRPY = -UnitSpan.convertUnit (UnitSpan.INCHES_72K, oRP.y().units(), oRP.y().value());
int lX = oX;
int lY = -oY;
int lXR = oRPX;
int lYR = -oRPY;
Angle oAngle = angle (false);
switch (oAngle.degrees ())
{
case 0:
break;
case 90:
oX = lXR - (lY - lYR);
oY = -(lYR + (lX - lXR));
break;
case 180:
oX = lXR - (lX - lXR);
oY = -(lYR - (lY - lYR));
break;
case 270:
oX = lXR + (lY - lYR);
oY = -(lYR - (lX - lXR));
break;
default:
double dRadians = oAngle.degrees() * (Math.PI / 180.0);
double dCosAngle = Math.cos (dRadians);
double dSinAngle = Math.sin (dRadians);
oX = (int) Math.round (lXR + (lX - lXR) * dCosAngle - (lY - lYR) * dSinAngle);
oY = (int) Math.round (lYR + (lY - lYR) * dCosAngle + (lX - lXR) * dSinAngle);
break;
}
return new CoordPair (new UnitSpan (UnitSpan.INCHES_72K, oX),
new UnitSpan (UnitSpan.INCHES_72K, oY));
}
/**
* @exclude from published api.
*/
public CoordPair offsetPoint (CoordPair oPoint) {
return oPoint.add (offset (false));
}
/**
* @exclude from published api.
*/
public double scale () {
return mdScale;
}
/**
* @exclude from published api.
*/
public UnitSpan unitW (int lWidth) {
checkInitialized();
int lWMI;
// if (mlScale == -1) {
lWMI = (int) Math.round (lWidth / mdXScale);
// } else {
// lWMI = JfApplyFactor (lWidth, FU_PER_INCH, mlDUInchX * mlScale);
// }
return new UnitSpan (UnitSpan.defaultUnits(), UnitSpan.INCHES_72K, lWMI);
}
/**
* @exclude from published api.
*/
public UnitSpan unitH (int lHeight) {
checkInitialized();
int lHMI;
// if (mlScale == -1) {
lHMI = (int) Math.round (lHeight / mdYScale);
// } else {
// lHMI = JfApplyFactor (lHeight, FU_PER_INCH, mlDUInchY * mlScale);
// }
return new UnitSpan (UnitSpan.defaultUnits(), UnitSpan.INCHES_72K, lHMI);
}
/**
* @exclude from published api.
*/
public CoordPair unitPoint (int lX, int lY) {
return new CoordPair (unitX (lX), unitY (lY));
}
/**
* @exclude from published api.
*/
public Rect unitRect (int lLeft, int lTop, int lRight, int lBottom) {
return new Rect (unitX (lLeft), unitY (lTop), unitX (lRight), unitY (lBottom));
}
/**
* @exclude from published api.
*/
public UnitSpan unitX (int lX) {
checkInitialized();
int lXMI;
// if (mlScale == -1) {
lXMI = ((int) Math.round ((lX - mlDUOriginX) / mdXScale)) + moOrigin.x().value();
// } else {
// lXMI = JfApplyFactor ((lX - mlDUOriginX), FU_PER_INCH, mlDUInchX * mlScale) + moOrigin.X().value();
// }
return new UnitSpan (UnitSpan.defaultUnits(), UnitSpan.INCHES_72K, lXMI);
}
/**
* @exclude from published api.
*/
public UnitSpan unitY (int lY) {
checkInitialized();
int lYMI;
// if (mlScale == -1) {
lYMI = ((int) Math.round ((lY - mlDUOriginY) / mdYScale)) + moOrigin.y().value();
// } else {
// lYMI = JfApplyFactor (lY - mlDUOriginY, FU_PER_INCH, mlDUInchY * mlScale) + moOrigin.Y().value();
// }
return new UnitSpan (UnitSpan.defaultUnits(), UnitSpan.INCHES_72K, lYMI);
}
/**
* @exclude from published api.
*/
public int mode () {
return meMode;
}
/**
* @exclude from published api.
*/
public void mode (int eNewMode) {
meMode = eNewMode;
}
/**
* @exclude from published api.
*/
public GFXLineAttr lineAttr () {
return mpoLineAttr;
}
/**
* @exclude from published api.
*/
public void lineAttr (GFXLineAttr poNewAttr) {
if (poNewAttr == null) {
mpoLineAttr = moDefaultLineAttr;
} else {
mpoLineAttr = new GFXLineAttr (poNewAttr); // TODO: C++ implementation just stores a ref
}
}
/**
* @exclude from published api.
*/
public GFXFillAttr fillAttr () {
return mpoFillAttr;
}
/**
* @exclude from published api.
*/
public void fillAttr (GFXFillAttr poNewAttr) {
if (poNewAttr == null) {
mpoFillAttr = moDefaultFillAttr;
} else {
mpoFillAttr = new GFXFillAttr (poNewAttr); // TODO: C++ implementation just stores a ref
}
}
/**
* @exclude from published api.
*/
public GFXTextAttr textAttr () {
return mpoTextAttr;
}
/**
* @exclude from published api.
*/
public void textAttr (GFXTextAttr poNewAttr) {
if (poNewAttr == null) {
mpoTextAttr = moDefaultTextAttr;
} else {
mpoTextAttr = new GFXTextAttr (poNewAttr); // TODO: C++ implementation just stores a ref
}
}
/**
* @exclude from published api.
*/
public FontInstance fontInstance () {
return moFontInstance;
}
/**
* @exclude from published api.
*/
public void fontInstance (FontInstance poNewFont) {
if (poNewFont == null) {
GFXEnv pEnv = env();
if (pEnv != null) {
FontService pFontService = pEnv.fontService();
if (pFontService != null) {
moFontInstance = pFontService.getDefaultFontInstance();
}
}
assert (moFontInstance != null);
} else {
moFontInstance = poNewFont;
}
}
/**
* @exclude from published api.
*/
public int glyphOrientation () {
return meGlyphOrientation;
}
/**
* @exclude from published api.
*/
public void glyphOrientation (int eNewGlyphOrientation) {
meGlyphOrientation = eNewGlyphOrientation;
}
/**
* Returns the character spacing currently in effect for this driver.
*
* Note that not all driver implementations support character spacing.
* @return Character spacing value currently in effect for this driver.
* The default implementation returns zero.
*
* @exclude from published api.
*/
public UnitSpan charSpacing () {
return UnitSpan.ZERO;
}
/**
* Set a new character spacing for subsequent text output.
*
* Note that not all driver implementations support character spacing.
* @param oNewSpacing - New character spacing value. Negative values
* are allowed.
*
* @exclude from published api.
*/
public void charSpacing (UnitSpan oNewSpacing) {
}
/**
* Query whether this driver implementation supports character spacing.
*
* If it does not, there is no point in calling the CharSpacing()
* overloads. AXTE uses the result of this method to determine whether
* a text run with a spacing override must be rendered as individual
* glyphs or can be put out as a run.
* @return True if the driver supports character spacing; false if not.
* The default implementation returns false.
*
* @exclude from published api.
*/
public boolean charSpacingSupported () {
return false;
}
/**
* Returns the word spacing currently in effect for this driver.
*
* Note that not all driver implementations support word spacing.
* @return Word spacing value currently in effect for this driver. The
* default implementation returns zero.
*
* @exclude from published api.
*/
public UnitSpan wordSpacing () {
return UnitSpan.ZERO;
}
/**
* Set a new word spacing for subsequent text output.
*
* Note that not all driver implementations support word spacing. Note
* also that word and character spacing support are independent of each
* other. A driver may support one, the other, both or neither.
* @param oNewSpacing - New word spacing value. Negative values are
* allowed.
*
* @exclude from published api.
*/
public void wordSpacing (UnitSpan oNewSpacing) {
}
/**
* Query whether this driver implementation supports word spacing.
*
* If it does not, there is no point in calling the WordSpacing()
* overloads.
* @return True if the driver supports word spacing; false if not. The
* default implementation returns false.
*
* @exclude from published api.
*/
public boolean wordSpacingSupported () {
return false;
}
/**
* @exclude from published api.
*/
public void pushOffset (boolean bRelative, CoordPair oOffset) {
if (moOffsetStack == null) {
moOffsetStack = new ArrayList();
}
if (bRelative) {
oOffset = oOffset.add (offset());
}
moOffsetStack.add (oOffset);
}
/**
* @exclude from published api.
*/
public void popOffset () {
// if (moOffsetStack.size() == 0) {
// JfExThrow (GFXDRIVER_ERR_OFFSET_STACK_EMPTY);
// }
moOffsetStack.remove(moOffsetStack.size() - 1);
}
/**
* @exclude from published api.
*/
public CoordPair offset (boolean bRelative) {
if ((moOffsetStack == null) || (moOffsetStack.size() == 0)) {
return CoordPair.zeroZero();
}
CoordPair last = moOffsetStack.get(moOffsetStack.size() - 1);
if ((! bRelative) || (moOffsetStack.size() == 1)) {
return last;
}
return last.subtract (moOffsetStack.get (moOffsetStack.size() - 2));
}
/**
* @exclude from published api.
*/
public CoordPair offset () {
return offset (false);
}
/**
* @exclude from published api.
*/
public void pushAngle (boolean bRelative, Angle oAngle, boolean bOffsetPoint, CoordPair oRotationPoint) {
if (moAngleStack == null) {
moAngleStack = new ArrayList();
}
if (bOffsetPoint) {
oRotationPoint = oRotationPoint.add (offset (false));
}
AnglePair pair = new AnglePair(
bRelative && moAngleStack.size() > 0 ? oAngle.add(oAngle) : oAngle,
oRotationPoint);
moAngleStack.add (pair);
}
/**
* @exclude from published api.
*/
public void popAngle () {
// if (moAngleStack.size() == 0) {
// JfExThrow (GFXDRIVER_ERR_ANGLE_STACK_EMPTY);
// }
moAngleStack.remove(moAngleStack.size() - 1);
}
/**
* @exclude from published api.
*/
public Angle angle (boolean bRelative) {
if ((moAngleStack == null) || (moAngleStack.size() == 0)) {
return Angle.ZERO;
}
AnglePair last = getAngle (moAngleStack.size() - 1);
if ((! bRelative) || (moAngleStack.size() == 1)) {
return last.angle;
}
AnglePair secondLast = getAngle (moAngleStack.size() - 2);
Angle result = last.angle;
return result.subtract (secondLast.angle);
}
/**
* @exclude from published api.
*/
public Angle angle () {
return angle (false);
}
/**
* @exclude from published api.
*/
public CoordPair rotationPoint (boolean bRelative) {
if ((moAngleStack == null) || (moAngleStack.size() == 0)) {
return CoordPair.zeroZero();
}
AnglePair last = getAngle (moAngleStack.size() - 1);
if ((! bRelative) || (moAngleStack.size() == 1)) {
return last.point;
}
return last.point.subtract (offset());
}
/**
* @exclude from published api.
*/
public void pushClipRect (boolean bRelative, Rect oClipRect) {
if (moClipStack == null) {
moClipStack = new ArrayList();
}
if (bRelative) {
oClipRect = oClipRect.add (offset (false));
}
if (moClipStack.size() > 0) {
oClipRect = oClipRect.intersection (clipRect());
}
moClipStack.add (oClipRect);
setClipRect (clipRect (false));
}
/**
* @exclude from published api.
*/
public void popClipRect () {
// if (moClipStack.size() == 0) {
// JfExThrow (GFXDRIVER_ERR_CLIP_STACK_EMPTY);
// }
moClipStack.remove(moClipStack.size() - 1);
setClipRect (clipRect (false));
}
/**
* @exclude from published api.
*/
public Rect clipRect (boolean bRelative) {
if ((moClipStack == null) || (moClipStack.size() == 0)) {
return visibleArea();
}
Rect last = moClipStack.get(moClipStack.size() - 1);
if ((! bRelative) || (moOffsetStack.size() == 0)) {
return last;
}
return last.subtract (offset());
}
/**
* @exclude from published api.
*/
public Rect clipRect () {
return clipRect (false);
}
/**
* @exclude from published api.
*/
public void clearStacks () {
moOffsetStack = null;
moAngleStack = null;
moClipStack = null;
setClipRect (visibleArea());
}
// public void CacheRemoveAll () {
// CacheRemoveAllButType (0);
// }
/**
* @exclude from published api.
*/
public CoordPair relPosition () {
return absPosition().subtract (offset (false));
}
/**
* @exclude from published api.
*/
public void relPosition (CoordPair oStart) {
absPosition (oStart.add (offset (false)));
}
/**
* @exclude from published api.
*/
public void relLine (CoordPair oEnd, int eDrawMode) {
absLine (oEnd.add (offset (false)), eDrawMode);
}
/**
* @exclude from published api.
*/
public void relLine (CoordPair oStart, CoordPair oEnd, int eDrawMode) {
CoordPair oOffset = offset (false);
absLine (oStart.add (oOffset), oEnd.add (oOffset), eDrawMode);
}
/**
* @exclude from published api.
*/
public void relLine (CoordPair oStart, CoordPair oEnd) {
CoordPair oOffset = offset (false);
absLine (oStart.add (oOffset), oEnd.add (oOffset), DRAW_2D);
}
/**
* @exclude from published api.
*/
public void relFillRect (Rect oRect, int eDrawMode) {
absFillRect (oRect.add (offset (false)), eDrawMode);
}
/**
* @exclude from published api.
*/
public void relFillRect (Rect oRect) {
absFillRect (oRect.add (offset (false)), DRAW_2D);
}
/**
* @exclude from published api.
*/
public void relText (String oText, int eDrawMode) {
absText (oText, eDrawMode);
}
/**
* @exclude from published api.
*/
public void relText (String oText) {
absText (oText, DRAW_2D);
}
/**
* @exclude from published api.
*/
public void relGlyphs (int[] pnGlyphs, int length) {
absGlyphs (pnGlyphs, length);
}
/**
* @exclude from published api.
*/
public CoordPair absPosition () {
return getAbsPosition();
}
/**
* @exclude from published api.
*/
public void absPosition (CoordPair oStart) {
setAbsPosition (oStart);
}
/**
* @exclude from published api.
*/
abstract public void absLine (CoordPair oEnd, int eDrawMode);
/**
* @exclude from published api.
*/
public void absLine (CoordPair oStart, CoordPair oEnd, int eDrawMode) {
CoordPair oTemp = absPosition();
absPosition (oStart);
absLine (oEnd, eDrawMode);
absPosition (oTemp);
}
/**
* @exclude from published api.
*/
abstract public void absFillRect (Rect oRect, int eDrawMode);
/**
* @exclude from published api.
*/
abstract public void absText (String oText, int eDrawMode);
/**
* @exclude from published api.
*/
public void absGlyphs (int[] pnGlyphs, int length) {
}
// public boolean AbsScroll (UnitSpan oDX, UnitSpan oDY, Rect oScrollArea) {
// return false;
// }
/**
* Set the Unicode characters that are about to be rendered by
* subsequent calls to the driver.
*
* If the driver requires any sort of mapping, the caller must provide
* the raw Unicode content through this call before calling any of the
* text rendering methods RelText(), AbsText(), RelGlyphs() or
* AbsGlyphs() to render that content.
*
*
* The caller typically calls this method once for each "line" of text
* and then makes one or more calls to the text rendering methods to
* render the text of the line. If the driver requires mapping, each
* text rendering call must be preceded by an appropriate call, in order
* to determine how the rendering run maps to the Unicode content.
*
* @param text - Unicode text content, UTF32 format.
*
* @exclude from published api.
*/
public void setUnicodeChars (int[] text) {
}
/**
* Indicates the caller needs to perform some specific rendering without
* losing the overall rendering context.
*
* The SetUnicodeChars() method establishes a context that the driver
* may need for subsequent rendering and mapping calls. There are
* situations where that context needs to be put on hold, while the
* caller goes off and performs some other sort of rendering, before
* coming back to the context originally set by SetUnicodeChars().
*
*
* In particular, consider the case where a tab leader is filled with a
* sequence of repeating glyphs. The caller will call SetUnicodeChars()
* with the Unicode content of the complete line, which contains text
* and tab characters. In filling the leader for a single tab, it will
* push a new context, with the text used to fill the tab leader. After
* rendering the tab leader, it will pop the context back to that of the
* original line.
*
* @param pcText - New Unicode text context to establish.
* @param pnCharIndex - (optional) Index of the character in the parent
* context that led to this new context. For example, in the case of
* tab leaders, this would be the index of a tab character. A future
* overload may allow for a more elaborate mapping specification.
*
* @exclude from published api.
*/
public void pushRenderContext (int[] pcText, int pnCharIndex) {
}
/**
* Restore the rendering context.
* Please see the description of PushRenderContext() for a description
* of rendering contexts. The client must call this once for each call
* it makes to PushRenderContext().
*
* @exclude from published api.
*/
public void popRenderContext () {
}
/**
* Legacy character/glyph mapping.
*
* If the driver needs legacy mapping, the caller must preceed each call
* to a text rendering method with a call to this method. Legacy
* mapping can describe only simple character/glyph relationships:
*
*
* -
* A sequence of N consecutive characters mapping 1:1,
* left-to-right to N consecutive glyphs.
*
* -
* A sequence of N consecutive characters mapping N:1 to a
* single glyph.
*
*
*
* If the driver uses legacy mapping, the caller must break each
* ligature and each RTL glyph out into its own run.
*
*
* @exclude from published api.
*/
public void mapChars (int nIndex, int nLength) {
}
/**
* Full character/glyph mapping.
*
* If the driver needs legacy mapping, the caller must preceed each call
* to a text rendering method with a call to this method. Full mapping
* supports 1:1, N:1, 1:M and N:M mappings,
* as well as mappings that involve non-contiguous runs of Unicode
* content and glyph output.
* @param oMappings - Description of the mappings for the subsequent
* text rendering call. The Unicode character indexes in the mappings
* are relative to the line's text passed in SetUnicodeChars(). The
* glyph indexes are zero-based, relative to the glyphs in the next text
* rendering call.
* @param bIsRTL - True if the subsequent run represents RTL text; FALSE
* for LTR text.
*
* @exclude from published api.
*/
public void mapGlyphs (GFXMappingList oMappings, boolean bIsRTL) {
}
/**
* Return the mapping required by the driver.
* @return Mapping level that the client must implement in order to
* satisfy the driver's needs.
*
* @exclude from published api.
*/
public int getMappingLevel () {
return MAPPING_LEGACY;
}
// abstract public GFXDevInfo DevInfo ();
/**
* @exclude from published api.
*/
public int dotsPerInch () {
checkInitialized();
return mlDUInchX;
}
/**
* @exclude from published api.
*/
abstract public int height ();
/**
* @exclude from published api.
*/
abstract public int width ();
/**
* @exclude from published api.
*/
abstract public boolean interactive ();
/**
* @exclude from published api.
*/
public Rect visibleArea () {
return unitRect (0, 0, width(), height());
}
// public GFXColour Reconcile (GFXColour poSource) {
// return new GFXColour (poSource);
// }
// public GFXLineAttr Reconcile (GFXLineAttr poSource) {
// return new GFXLineAttr (poSource);
// }
// public GFXFillAttr Reconcile (GFXFillAttr poSource) {
// return new GFXFillAttr (poSource);
// }
// public GFXTextAttr Reconcile (GFXTextAttr poSource) {
// return new GFXTextAttr (poSource);
// }
/**
* @exclude from published api.
*/
public CoordPair nonPrintOffset () {
return unitPoint (mlDUOffsetX, mlDUOffsetY);
}
// public void UseChar (GFXFont poFont, Char pcChar) {
// }
/**
* @exclude from published api.
*/
public void paraHint () {
}
// public void Attach (boolean bInfoOnly) {
// if (bInfoOnly == false) {
// LineAttr (null);
// FillAttr (null);
// TextAttr (null);
// Font (null);
// FontInstance (null);
// }
// }
// public void Detach (boolean bInfoOnly) {
// mpoLineAttr = null;
// mpoFillAttr = null;
// mpoTextAttr = null;
// mpoFont = null;
//
// if (bInfoOnly) {
// moOffsetStack.clear();
// moAngleStack.clear();
// moClipStack.clear();
// } else {
// ClearStacks();
// }
// }
// public void CaretShow () {
// }
// public void CaretHide () {
// }
// public boolean CaretIsVisible () {
// return false;
// }
// public void CaretMove (CoordPair oPosition) {
// }
// public void CaretSize (UnitSpan oWidth, UnitSpan oHeight) {
// }
// public CoordPair CaretPosition () {
// return getDefaultCoordPair();
// }
// public UnitSpan CaretWidth () {
// return getDefaultUnitSpan();
// }
// public UnitSpan CaretHeight () {
// return getDefaultUnitSpan();
// }
// public void Focus (boolean bFocus) {
// }
/**
* @exclude from published api.
*/
public void resolutionChanged () {
}
/**
* @exclude from published api.
*/
public UnitSpan heightInUnits () {
checkInitialized();
int lHMI = (int) (height() * (FU_PER_INCH / mlDUInchY)); // TODO: major truncation errors
return new UnitSpan (UnitSpan.defaultUnits(), UnitSpan.INCHES_72K, lHMI);
}
/**
* @exclude from published api.
*/
public UnitSpan widthInUnits () {
checkInitialized();
int lWMI = (int) (width() * (FU_PER_INCH / mlDUInchX)); // TODO: major truncation errors
return new UnitSpan (UnitSpan.defaultUnits(), UnitSpan.INCHES_72K, lWMI);
}
/**
* @exclude from published api.
*/
public boolean draw3dEffects () {
return mb3dEffects;
}
/**
* @exclude from published api.
*/
public void draw3dEffects (boolean b3dEffects) {
mb3dEffects = b3dEffects;
}
// public void ReleaseObject (GFXDevObj poDevObj, boolean bUpdateDevObj) {
// if (mpoLineAttr == poDevObj) {
// LineAttr (null);
// } else if (mpoFillAttr == poDevObj) {
// FillAttr (null);
// } else if (mpoTextAttr == poDevObj) {
// TextAttr (null);
// } else if (mpoFont == poDevObj) {
// Font (null);
// }
//
// for (int i = moCache.size(); i > 0; ) {
//// Now remove all instances of it from the cache.
//// Search cache from end, so removals don't cause us to skip items
// i--;
// if (moCache[i].key == poDevObj) {
// CacheRemove (i, bUpdateDevObj);
// }
// }
// }
/**
* @exclude from published api.
*/
protected CoordPair getAbsPosition () {
return moAbsPosition;
}
/**
* @exclude from published api.
*/
protected void setAbsPosition (CoordPair oAbsPosition) {
moAbsPosition = oAbsPosition;
}
/**
* @exclude from published api.
*/
protected void initDeviceUnitsPerInch (double dUnits) {
mdXScale = dUnits;
mdYScale = dUnits;
mlDUInchX = (int) Math.round (dUnits); // as close as we can get
mlDUInchY = mlDUInchX;
mdScale = dUnits / mlDUInchX; // to account for rounding error
// mlScale = -1;
}
/**
* @exclude from published api.
*/
protected void initDeviceUnitsPerInch (int lX, int lY) {
// TODO: how to set up new scales? is this used?
mlDUInchX = lX;
mlDUInchY = lY;
}
/**
* @exclude from published api.
*/
protected void initDeviceOffsets (int lX, int lY) {
mlDUOffsetX = lX;
mlDUOffsetY = lY;
}
/**
* @exclude from published api.
*/
protected void scaleChanged () {
}
/**
* @exclude from published api.
*/
abstract protected void setClipRect (Rect oRect);
/**
* @exclude from published api.
*/
protected boolean angleIsRight () {
if ((moAngleStack != null) && (moAngleStack.size() > 0)) {
int lDegrees = getAngle(moAngleStack.size() - 1).angle.degrees();
return (lDegrees == 0) || (lDegrees == 90) || (lDegrees == 180) || (lDegrees == 270);
} else {
return true;
}
}
// Device Object Cache Functions
// protected int CacheSize () {
// return mnCacheSize;
// }
// protected void CacheSize (int nNewSize) {
// if (moCache.size() == 0) {
// mnCacheSize = nNewSize;
// if (nNewSize > 0) {
// moCache.granularity (nNewSize); // limit reallocations
// }
// }
// }
// protected void CacheAdd (GFXCacheItem poCacheItem, GFXCacheObj poCacheObj) {
// poCacheItem.driverConnect (this);
// GFXCacheAssoc oAssoc = {poCacheItem, poCacheObj}; // required for MSVC 2.0
//
// if ((moCache.size() >= mnCacheSize) && (mnCacheSize > 0)) {
//// If necessary, call CacheRemove() to free up room in the cache.
// GFXDevObj poRemove = moCache[0].key.devObj();
//
//// If this object is in the cache more than once, don't release it
// boolean bRelease = true;
// int nSize = moCache.size();
// for (int i = 1; i < nSize; i++) {
// if (moCache[i].key.devObj() == poRemove) {
// bRelease = false;
// break;
// }
// }
// CacheRemove (0, bRelease);
// }
// moCache.append (oAssoc);
// }
// protected GFXCacheObj CacheFind (GFXCacheItem oCacheItem) {
// for (int i = moCache.size(); i > 0; ) { // search cache from live end
// i--;
//
// if (moCache[i].key == oCacheItem) {
// GFXCacheObj poCacheObj = moCache[i].value;
//
// if (i < moCache.size() - 1) {
//// Move to the head (high end) of the cache, to be found next time
// GFXCacheItem poCacheItem = moCache[i].key;
// GFXCacheAssoc oAssoc = {poCacheItem, poCacheObj};
// moCache.remove (i);
// moCache.append (oAssoc);
// }
// return poCacheObj;
// }
// }
//
// return null;
// }
// protected void CacheRemoveAllButType (ObjType oType) {
// for (int i = moCache.size(); i > 0; ) {
//// search cache from end, so removals don't cause a lot of copying
// i--;
// if (moCache[i].key.devObj().type() != nType) {
// CacheRemove (i, true);
// }
// }
//
// if (mpoLineAttr != null) { // ie Driver is Attached
// LineAttr (null); // If Driver is attached, then reset the current attributes.
// FillAttr (null);
// TextAttr (null);
// Font (null);
// FontInstance (null);
// }
// }
private void checkInitialized () {
if (mlDUInchX <= 0 || mlDUInchY <= 0) {
// JfExThrow (GFXDRIVER_ERR_NOTINIT);
}
}
// private void CacheRemove (int nRemove, boolean bUpdateDevObj) {
// GFXCacheAssoc oAssoc = moCache[nRemove];
// moCache.remove (nRemove);
//
// if (bUpdateDevObj) {
// oAssoc.key.driverDisconnect();
// }
// delete Key;
// delete Value;
//
// private AnglePair getAngle (int index) {
// assert moAngleStack != null;
// return moAngleStack.get (index);
// }
// }
private int devW (int pts1000) {
return (int) Math.round (pts1000 * mdXScale);
}
private int devH (int pts1000) {
return (int) Math.round (pts1000 * mdYScale);
}
private AnglePair getAngle (int index) {
if ((moAngleStack == null) || (moAngleStack.size() == 0)) {
return null;
}
return moAngleStack.get (index);
}
}