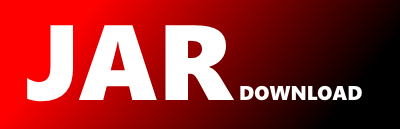
com.adobe.xfa.layout.BoxModelCaptionable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.layout;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.Element;
import com.adobe.xfa.EnumAttr;
import com.adobe.xfa.Measurement;
import com.adobe.xfa.STRS;
import com.adobe.xfa.XFA;
import com.adobe.xfa.content.Content;
import com.adobe.xfa.content.DateTimeValue;
import com.adobe.xfa.content.DateValue;
import com.adobe.xfa.content.DecimalValue;
import com.adobe.xfa.content.ExDataValue;
import com.adobe.xfa.content.FloatValue;
import com.adobe.xfa.content.IntegerValue;
import com.adobe.xfa.content.TextValue;
import com.adobe.xfa.content.TimeValue;
//import com.adobe.xfa.font.FontService;
import com.adobe.xfa.font.GfxTextAttrs;
import com.adobe.xfa.gfx.GFXColour;
import com.adobe.xfa.template.TemplateResolver;
import com.adobe.xfa.template.containers.Draw;
import com.adobe.xfa.template.containers.ExclGroup;
import com.adobe.xfa.template.containers.Field;
import com.adobe.xfa.text.TextAttr;
import com.adobe.xfa.text.TextBaselineShift;
import com.adobe.xfa.text.TextDisplay;
import com.adobe.xfa.text.TextMeasurement;
import com.adobe.xfa.text.TextPosn;
import com.adobe.xfa.text.TextPosnBase;
import com.adobe.xfa.text.TextRange;
import com.adobe.xfa.text.TextRegion;
import com.adobe.xfa.text.markup.MarkupRtfOut;
import com.adobe.xfa.text.markup.MarkupXHTMLAttr;
import com.adobe.xfa.text.markup.MarkupXHTMLIn;
import com.adobe.xfa.text.markup.MarkupXHTMLOut;
import com.adobe.xfa.ut.CoordPair;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.Rect;
import com.adobe.xfa.ut.ResId;
import com.adobe.xfa.ut.StringUtils;
import com.adobe.xfa.ut.UnitSpan;
import java.util.ArrayList;
import java.util.List;
/**
*
* @exclude from published api.
*/
abstract class BoxModelCaptionable extends BoxModelRenderProxy {
//is there caption region at all, not necessarily text
protected boolean m_bReserveCaptionSpace;
protected UnitSpan m_oReserveCaptionSpace = UnitSpan.ZERO;
protected int m_eCaptionPlacement;
protected int m_eCaptionPresence;
protected UnitSpan m_oLineHeight = UnitSpan.ZERO;
//box model subregions - caption and non-caption, aka content
// Javaport: TODO
// protected AXTEDisplay m_oCaptionDisplay;
protected Rect m_oCaptionDisplayExtent = Rect.ZERO;
protected boolean m_bSplitCaption;
protected TextPosn m_oStartCaptionPosn = new TextPosn();
protected TextPosn m_oEndCaptionPosn = new TextPosn();
// Flag to indicate whether we need to keep the text stream from being destroyed in re-initialization
protected boolean m_bKeepCaptionTextStream;
protected boolean m_bAllowRenderProxy;
public BoxModelCaptionable(LayoutEnv oEnv, boolean bAllowRenderProxy) {
super(oEnv);
m_bReserveCaptionSpace = false;
m_eCaptionPlacement = EnumAttr.PLACEMENT_LEFT;
m_eCaptionPresence = EnumAttr.PRESENCE_VISIBLE;
m_bSplitCaption = false;
m_bKeepCaptionTextStream = false;
m_bAllowRenderProxy = bAllowRenderProxy;
}
public void clear() {
//Empty the box model
// Javaport: TODO
// m_oCaptionDisplay.setNull();
m_bKeepCaptionTextStream = false;
m_bReserveCaptionSpace = false;
m_eCaptionPlacement = EnumAttr.PLACEMENT_LEFT;
m_eCaptionPresence = EnumAttr.PRESENCE_VISIBLE;
m_bSplitCaption = false;
m_bKeepCaptionTextStream = false;
m_oReserveCaptionSpace = UnitSpan.ZERO;
super.clear();
// Javaport: TODO
// assert(m_oCaptionDisplay == null);
}
public void reinitialize(Element oNode) {
if (! m_bKeepCaptionTextStream) {
super.reinitialize(oNode);
return;
}
else {
//Don't do clean up here. We want to keep caption and content
// text stream alive here.
assert(oNode != null);
initialize(oNode);
}
}
public boolean allowRenderProxy() {
return m_bAllowRenderProxy;
}
public Rect getContentExtent() {
//If no caption, return default content (ne - margins)
//If is caption, return portion of content not covered by caption
if (hasCaption())
return m_oContentExtent;
else
return super.getContentExtent();
}
public Rect getContentBorderExtent() {
// default is empty
//Border = CE - border_margins
Rect oCE = getContentExtent();
return new Rect(oCE.left().add(m_oContentBorderMargins.marginLeft()).subtract(m_oContMargins.marginLeft()),
oCE.top().add(m_oContentBorderMargins.marginTop()).subtract(m_oContMargins.marginTop()),
oCE.right().subtract(m_oContentBorderMargins.marginRight()).add(m_oContMargins.marginRight()),
oCE.bottom().subtract(m_oContentBorderMargins.marginBottom()).add(m_oContMargins.marginBottom()));
}
public Rect getCaptionExtent() {
//If no caption, return default (empty)
//If caption, return portion of the content covered by it
if (hasCaption())
return m_oCaptionExtent;
else
return super.getCaptionExtent();
}
public abstract boolean hasContent();
public abstract String getContentByType(String sType,
List colorTable,
List fontTable);
public abstract boolean enumerateContent(LayoutHandler pHandler,
CoordPair oOffset,
boolean bWrapText,
boolean bTruncate /* = false */,
Rect oInvalidatedRect /* = Rect.ZERO */);
public boolean enumerateCaption(LayoutHandler pHandler,
CoordPair oLocalOrigin,
boolean bTruncate /* = false */,
Rect oInvalidatedRect /* = Rect.ZERO */) {
boolean bTextFits = true;
if (hasCaption() && hasVisibleCaption()) {
CoordPair oNewOrigin = oLocalOrigin;
Rect oInvalid = oInvalidatedRect;
if (oInvalid.width().equals(UnitSpan.ZERO) && oInvalid.height().equals(UnitSpan.ZERO))
oInvalid = Rect.ZERO;
if (m_bSplitCaption) {
oInvalid = m_oCaptionDisplayExtent;
oNewOrigin = new CoordPair(oNewOrigin.x(), oNewOrigin.y().subtract(m_oCaptionDisplayExtent.top()));
}
boolean bSplitCaptionFits = true;
if (m_oCaptionDisplayExtent.width().gt(getCaptionExtent().width())) {
oInvalid = oInvalid.width(getCaptionExtent().width(), false);
bSplitCaptionFits = false;
}
if (m_oCaptionDisplayExtent.height().gt(getCaptionExtent().height())) {
oInvalid = oInvalid.height(getCaptionExtent().height(), false);
bSplitCaptionFits = false;
}
bTextFits = m_oGfxLayoutEnv.renderText(pHandler,
getCaptionDisplay(),
oNewOrigin,
false,
bTruncate,
m_oRotationAngle,
oInvalid);
if (m_bSplitCaption)
return bSplitCaptionFits;
}
return bTextFits;
}
public String getCaptionByType(String sType,
List colorTable,
List fontTable) {
if (hasCaption() && null != getCaption())
return getStringByType(getCaption(), null, sType, m_oStartCaptionPosn, m_oEndCaptionPosn, colorTable, fontTable);
else
return "";
}
public abstract void resizeToNominal(UnitSpan oW, UnitSpan oH, Element oNode);
public abstract void resizeToNominalWidth(UnitSpan oW, Element oNode);
public abstract void resizeToContent(UnitSpan oW, UnitSpan oH, Element oNode);
public abstract boolean splitImpl(UnitSpan oNewHeight, BoxModelLayout oNewBM, Element oNode);
public boolean isFontSubstituted() {
boolean bIsFontSubstituted = false;
TextDisplay pDisp = getCaptionDisplay();
if (pDisp != null)
bIsFontSubstituted = pDisp.hasFontSubstitution();
return bIsFontSubstituted;
}
public boolean getKeepCaptionTextStreamFlag() {
return m_bKeepCaptionTextStream;
}
public void setKeepCaptionTextStreamFlag(boolean bKeepTextStream) {
m_bKeepCaptionTextStream = bKeepTextStream;
}
/*
* Interface for box model text editing
*/
public abstract void update(Element oNode);
public TextDisplay getCaptionDisplay() {
// Javaport: TODO
// if (m_oCaptionDisplay != null)
// return m_oCaptionDisplay.getDisplay();
return null;
}
public TextRegion getCaption() {
// Javaport: TODO
// if (m_oCaptionDisplay != null)
// return m_oCaptionDisplay.getRegion();
return null;
}
public TextRange getCaptionRangeAttrs() {
// Javaport: TODO
// assert (m_oCaptionDisplay != null);
// return m_oCaptionDisplay.getRangeAttr();
return null;
}
/*
* Misc utils for derived classes
*/
protected void setVertAlign(int eVAlign, TextAttr attr) {
if (eVAlign == EnumAttr.VALIGN_TOP) {
attr.justifyV(TextAttr.JUST_V_TOP);
}
else if (eVAlign == EnumAttr.VALIGN_MIDDLE) {
attr.justifyV(TextAttr.JUST_V_MIDDLE);
}
else if (eVAlign == EnumAttr.VALIGN_BOTTOM) {
attr.justifyV(TextAttr.JUST_V_BOTTOM);
}
else {
attr.justifyV(TextAttr.JUST_V_UNKNOWN);
}
}
protected void setHorizAlign(int eHAlign, TextAttr attr) {
if (eHAlign == EnumAttr.HALIGN_LEFT) {
attr.justifyH(TextAttr.JUST_H_LEFT);
}
else if (eHAlign == EnumAttr.HALIGN_CENTER) {
attr.justifyH(TextAttr.JUST_H_CENTRE);
}
else if (eHAlign == EnumAttr.HALIGN_RIGHT) {
attr.justifyH(TextAttr.JUST_H_RIGHT);
}
else if (eHAlign == EnumAttr.HALIGN_JUSTIFY) {
attr.justifyH(TextAttr.JUST_H_SPREAD);
}
else if (eHAlign == EnumAttr.HALIGN_JUSTIFY_ALL) {
attr.justifyH(TextAttr.JUST_H_SPREAD_ALL);
}
else if (eHAlign == EnumAttr.HALIGN_RADIX) {
attr.justifyH(TextAttr.JUST_H_RADIX);
}
else {
attr.justifyH(TextAttr.JUST_H_UNKNOWN);
}
}
protected void setFont(Element oFontNode, Element oParaNode, boolean bContentFont, TextAttr attr, String sLang) {
//Strategy
//Set up text engine ambient font attributes given an xfa font node.
//If bContentFont=true then apply font settings to the content attribute range,
//otherwise apply font settings to caption attribute range.
//
// Note: If this is a rich text object, any other fonts specified in the
// XHTML markup will be resolved later (see the Reconcile method in the
// XFALayoutDriver class) when the Text Engine actually needs to layout
// the object.
assert(oFontNode != null);
// Resolve the font.
// This may include getting metrics, or even a font mapping.
//FontService pFontService = m_oGfxLayoutEnv.fontService();
GfxTextAttrs oGfxExtras = new GfxTextAttrs();
// Javaport: TODO
// FontInstance oResolvedFont = pFontService.resolveXFAFont(oFontNode, oGfxExtras, sLang);
// //
// // Used to divide our content and caption regions.
// //
// if (! bContentFont)
// m_oLineHeight = oResolvedFont.getSpacing();
//Note: must associate text attr with the global mapper/pool objects
//in order to maintain proper reference count.
//For performance reasons, collect all
// Javaport: TODO
// attr.attrPool(m_oGfxLayoutEnv.getGfxAttrPool());
// attr.fontInstance(oResolvedFont, oGfxExtras.sOriginalTypeface);
attr.fontService(m_oGfxLayoutEnv.fontService());
// Set color, baselineshift and line props
attr.colour(oGfxExtras.m_oColour);
attr.underline(oGfxExtras.m_eUndercode);
attr.overline(oGfxExtras.m_eOvercode);
attr.strikeout(oGfxExtras.m_eStrikeOut);
attr.baselineShift(new TextBaselineShift(oGfxExtras.m_oBaseLineShift));
//Set para attributes. We don't need to know whether this font lives under / or
boolean bLineSpace = false;
// set default, so it is enabled.
attr.spaceBefore(TextMeasurement.ZERO);
attr.spaceAfter(TextMeasurement.ZERO);
attr.special(TextMeasurement.ZERO);
attr.marginL(TextMeasurement.ZERO);
attr.marginR(TextMeasurement.ZERO);
if (oParaNode != null) {
if (oParaNode.isPropertySpecified(XFA.LINEHEIGHTTAG, true, 0)) {
Measurement oLineHeight = new Measurement(oParaNode.getAttribute(XFA.LINEHEIGHTTAG));
if (! bContentFont)
m_oLineHeight = oLineHeight.getUnitSpan();
attr.spacing(new TextMeasurement(oLineHeight.getUnitSpan()));
bLineSpace = true;
}
if (oParaNode.isPropertySpecified(XFA.SPACEABOVETAG, true, 0)) {
Measurement oSpaceBefore = new Measurement(oParaNode.getAttribute(XFA.SPACEABOVETAG));
attr.spaceBefore(new TextMeasurement(oSpaceBefore.getUnitSpan()));
}
if (oParaNode.isPropertySpecified(XFA.SPACEBELOWTAG, true, 0)) {
Measurement oSpaceAfter = new Measurement(oParaNode.getAttribute(XFA.SPACEBELOWTAG));
attr.spaceAfter(new TextMeasurement(oSpaceAfter.getUnitSpan()));
}
if (oParaNode.isPropertySpecified(XFA.TEXTINDENTTAG, true, 0)) {
Measurement oSpecial = new Measurement(oParaNode.getAttribute(XFA.TEXTINDENTTAG));
attr.special(new TextMeasurement(oSpecial.getUnitSpan()));
}
if (oParaNode.isPropertySpecified(XFA.MARGINLEFTTAG, true, 0)) {
Measurement oLeftIndent = new Measurement(oParaNode.getAttribute(XFA.MARGINLEFTTAG));
attr.marginL(new TextMeasurement(oLeftIndent.getUnitSpan()));
}
if (oParaNode.isPropertySpecified(XFA.MARGINRIGHTTAG, true, 0)) {
Measurement oRightIndent = new Measurement(oParaNode.getAttribute(XFA.MARGINRIGHTTAG));
attr.marginR(new TextMeasurement(oRightIndent.getUnitSpan()));
}
}
// If neither is set, then we are the FF99 standard
// control, and default spacing is 0pt.
if (! bLineSpace) {
UnitSpan oSpan = new UnitSpan(0.0, UnitSpan.MM_25K);
attr.spacing(new TextMeasurement(oSpan));
}
}
protected void setTabs(Attribute oTabsDefault, Attribute oTabStops, TextAttr attr) {
String sTabsDefault = oTabsDefault.toString();
boolean bTabsDefault = !StringUtils.isEmpty(sTabsDefault);
String sTabStops = oTabStops.toString();
boolean bTabStops = !StringUtils.isEmpty(sTabStops);
if (bTabsDefault || bTabStops) {
if (bTabsDefault)
MarkupXHTMLIn.tabDefault(sTabsDefault, attr, MarkupXHTMLAttr.getDefault());
if (bTabStops)
MarkupXHTMLIn.tabSet(sTabStops, attr, MarkupXHTMLAttr.getDefault());
}
}
protected void initCaption(Element oNode) {
//Strategy
//1)If caption is present, create text stream
// and set text value + font attributes
//2)Flag inline caption for later processing
//Do not create caption display at this time
String sCaption = null;
hasCaption(false);
m_bReserveCaptionSpace = false;
if (oNode instanceof Draw || oNode instanceof Field || oNode instanceof ExclGroup) {
if (oNode.isPropertySpecified(XFA.CAPTIONTAG, true, 0)) {
//Get the caption specifics
boolean bRichCaption = false;
Element oCaption = oNode.peekElement(XFA.CAPTIONTAG, true, 0);
m_eCaptionPresence = oCaption.getEnum(XFA.PRESENCETAG);
Element oCapValue = oCaption.peekElement(XFA.VALUETAG, true, 0);
Content oCapContent = (Content) oCapValue.getOneOfChild(true, true);
m_eCaptionPlacement = oCaption.getEnum(XFA.PLACEMENTTAG);
if (EnumAttr.PRESENCE_HIDDEN == m_eCaptionPresence || EnumAttr.PRESENCE_INACTIVE == m_eCaptionPresence)
return;
//
//Yes Virginia, there is a caption.
//
hasCaption(true);
boolean bExData = oCapContent instanceof ExDataValue;
if (bExData) {
String sContentType = oCapContent.getAttribute(XFA.CONTENTTYPETAG).toString();
bRichCaption = (STRS.TEXTHTML.equals(sContentType));
}
if (oCapContent instanceof TextValue) {
sCaption = ((TextValue) oCapContent).getValue();
}
else if (oCapContent instanceof DecimalValue) {
double dTemp = ((DecimalValue) oCapContent).getValue();
sCaption = Double.toString(dTemp);
}
else if (oCapContent instanceof IntegerValue) {
int nTemp = ((IntegerValue) oCapContent).getValue();
sCaption = Integer.toString(nTemp);
}
else if (oCapContent instanceof FloatValue) {
double dTemp = ((FloatValue) oCapContent).getValue();
sCaption = Double.toString(dTemp);
}
else if (oCapContent instanceof DateValue) {
sCaption = ((DateValue) oCapContent).getValue();
}
else if (oCapContent instanceof DateTimeValue) {
sCaption = ((DateTimeValue) oCapContent).getValue();
}
else if (oCapContent instanceof TimeValue) {
sCaption = ((TimeValue) oCapContent).getValue();
}
else if (oCapContent instanceof ExDataValue) {
AppModel appModel = oCapContent.getAppModel();
// watson bug 1795969 old forms need to use the legacy XHTML processing
boolean bLegacy = appModel.getLegacySetting(AppModel.XFA_LEGACY_V27_XHTMLVERSIONPROCESSING);
// If we are here then the content type was not
// text/html, but text/plain, so we will get the
// value here. If it's RichText, it will be handled
// later in this method.
if (bExData && ! bRichCaption)
sCaption = ((ExDataValue) oCapContent).getValue(false, false, bLegacy);
}
if (! m_bKeepCaptionTextStream) { // Do full initialization
//Init text engine members
// Javaport: TODO
// m_oCaptionDisplay = new AXTEDisplay(new TextRegion(), m_oGfxLayoutEnv);
// getCaption().setText(sCaption);
// getCaption().allowOverflow(false);
// getCaptionRangeAttrs().associate(getCaption());
//For performance reasons, collect all text attributes
//so they can be set only once.
TextAttr oCaptionAttr = new TextAttr();
oCaptionAttr.transparent(true);
oCaptionAttr.fontService(m_oGfxLayoutEnv.fontService());
// Get the locale setting for the field/draw if specified
// and set it on the text attr.
String sLang = oNode.getInstalledLocale();
if (!StringUtils.isEmpty(sLang)) {
oCaptionAttr.locale(sLang);
}
//Record caption attributes such as placement, reserve, alignment
//but do not try to place it.
//Note: Cannot set the width/height of the caption text block yet -
//this can't be known until internal formatting takes place.
if (! hasInlineCaption()) {
Element oPara = oCaption.peekElement(XFA.PARATAG, true, 0);
int eCaptionVAlign = oPara.getEnum(XFA.VALIGNTAG);
int eCaptionHAlign = oPara.getEnum(XFA.HALIGNTAG);
//Be aware that justifyAll is not compatible with horiz-growableness.
//One indicates 'grow to the content extent' and the other says 'extend the content along the
//entire extent'.
if (EnumAttr.HALIGN_JUSTIFY_ALL == eCaptionHAlign && hasGrowableW())
eCaptionHAlign = EnumAttr.HALIGN_JUSTIFY;
setVertAlign(eCaptionVAlign, oCaptionAttr);
setHorizAlign(eCaptionHAlign, oCaptionAttr);
// Check for Radix Offset
if (EnumAttr.HALIGN_RADIX == eCaptionHAlign) {
UnitSpan oRO = new Measurement(oPara.getAttribute(XFA.RADIXOFFSETTAG)).getUnitSpan();
oCaptionAttr.radixOffset(new TextMeasurement(oRO));
}
setFont(oCaption.peekElement(XFA.FONTTAG, true, 0), oPara, false, oCaptionAttr, oCaption.getInstalledLocale());
m_bReserveCaptionSpace = oCaption.isPropertySpecified(XFA.RESERVETAG, true, 0);
}
else
m_bReserveCaptionSpace = false;
//Apply text attributes (do so before resolving any xhtml fragment)
// Javaport: TODO
// getCaptionRangeAttrs().attribute(oCaptionAttr);
if (bRichCaption) {
AppModel appModel = oCapContent.getAppModel();
// watson bug 1795969 old forms need to use the legacy XHTML processing
boolean bLegacy = appModel.getLegacySetting(AppModel.XFA_LEGACY_V27_XHTMLVERSIONPROCESSING);
sCaption = ((ExDataValue) oCapContent).getValue(true, false, bLegacy);
// Supply an ambient attribute so that we can process the
// xhtml fragment better. This fixes an immediate problem
// where we need to tell text services what the ambient font is.
if (!StringUtils.isEmpty(sCaption)) {
// Javaport: TODO
// TextAttr pAmbientAttr = getCaptionRangeAttrs().position(TextRange.POSN_START).attribute();
// MarkupXHTMLIn oMarkup = new MarkupXHTMLIn(sCaption, null, pAmbientAttr, null);
// getCaptionRangeAttrs().markup(oMarkup);
}
}
//Do not consider there to be a caption for inline
if (hasInlineCaption())
hasCaption(false);
}
else {
//Was reset at top of this function. Redetermine it's appropriate value
if (! hasInlineCaption())
m_bReserveCaptionSpace = oCaption.isPropertySpecified(XFA.RESERVETAG, true, 0);
else
m_bReserveCaptionSpace = false;
}
if (m_bReserveCaptionSpace) {
assert(oCaption.isPropertySpecified(XFA.RESERVETAG, true, 0));
Measurement oMeas = new Measurement(oCaption.getAttribute(XFA.RESERVETAG));
m_oReserveCaptionSpace = oMeas.getUnitSpan();
//Explicitly specifying a reserve value <=0 is treated as auto-expand-to-fit.
if (UnitSpan.ZERO.gte(m_oReserveCaptionSpace))
m_bReserveCaptionSpace = false;
}
// Javaport: TODO. For now, just throw if sized-to-fit and caption is non-null.
if (! m_bReserveCaptionSpace && hasCaption() && sCaption.length() != 0) {
throw new ExFull(ResId.UNSUPPORTED_OPERATION, "BoxModelCaptionable#initCaption");
}
}
else {
//Empty the box model
// Javaport: TODO
// m_oCaptionDisplay.setNull();
m_bKeepCaptionTextStream = false;
m_bHasCaption = false;
m_bReserveCaptionSpace = false;
m_eCaptionPlacement = EnumAttr.PLACEMENT_LEFT;
m_eCaptionPresence = EnumAttr.PRESENCE_VISIBLE;
m_bSplitCaption = false;
m_bKeepCaptionTextStream = false;
m_oCaptionExtent = Rect.ZERO;
m_oContentExtent = Rect.ZERO;
m_oReserveCaptionSpace = UnitSpan.ZERO;
}
}
if (hasCaption()) {
TextPosnBase oPosn = new TextPosnBase(getCaption());
m_oStartCaptionPosn = new TextPosn(oPosn.stream());
m_oEndCaptionPosn = new TextPosn(oPosn.stream());
m_oStartCaptionPosn.first();
m_oEndCaptionPosn.last();
}
}
protected String getStringByType(TextRegion pTB,
TextAttr poAmbientAttr,
String sType,
TextPosn oStart,
TextPosn oEnd,
List colorTable,
List fontTable) {
assert(null != pTB);
assert(!StringUtils.isEmpty(sType));
String sText = null;
if (null != pTB) {
if (sType.equals("rtf")) {
// Our XHTML parser wants the colours as GfxColours
// So we need to convert them here.
List oColours = new ArrayList();
if (null != colorTable) {
for (int i = 0; i < colorTable.size(); i++) {
TemplateResolver.RGB rgb = (TemplateResolver.RGB) colorTable.get(i);
oColours.add(new GFXColour(rgb.mnRed, rgb.mnGreen, rgb.mnBlue, 255));
}
}
TextRange oRange = new TextRange(pTB);
oRange.start(oStart);
oRange.end(oEnd);
MarkupRtfOut oMarkupOut = new MarkupRtfOut (null, oColours, fontTable);
oRange.markup(oMarkupOut, null, true, false);
sText = oMarkupOut.translation();
}
else if (sType.equals("html")) {
TextRange oRange = new TextRange(pTB);
oRange.start(oStart);
oRange.end(oEnd);
MarkupXHTMLOut oMarkupOut = new MarkupXHTMLOut(null, UnitSpan.INCHES_72K, poAmbientAttr, true);
oRange.markup(oMarkupOut, null, true, false);
sText = oMarkupOut.translation();
}
else if (sType.equals("plain")) {
TextRange oRange = new TextRange(pTB);
oRange.start(oStart);
oRange.end(oEnd);
// Javaport: TODO
oRange.text(/*sText,*/ true);
}
}
return sText;
}
protected boolean hasInlineCaption() {
return (EnumAttr.PLACEMENT_INLINE == m_eCaptionPlacement);
}
protected boolean hasVisibleCaption() {
return hasCaption() && (EnumAttr.PRESENCE_VISIBLE == m_eCaptionPresence);
}
/**
* Return true if this box model contains text that overflows it's allotted space
* and false otherwise.
* @return false if this object contains no text.
* @see #BoxModelLayout.hasOverflowingCaptionText()
* @exclude from published api.
*/
public boolean hasOverflowingCaptionText() {
// Javaport: not yet available. TODO
/*
if ((m_oCaptionDisplay != null) && null != m_oCaptionDisplay.getDisplay()) {
Rect rcCaptionRuntime = m_oCaptionDisplay.getDisplay().runtimeExtent();
Rect rcCaptionAllotted = getCaptionExtent();
if (m_bWasRotated && (m_oRotationAngle.degrees() == 90 || m_oRotationAngle.degrees() == 270)) {
//Flip it - compare allotted-content width vs runtime h etc
return rcCaptionAllotted.height() < rcCaptionRuntime.width() || rcCaptionAllotted.width() < rcCaptionRuntime.height();
}
return rcCaptionAllotted.width() < rcCaptionRuntime.width() || rcCaptionAllotted.height() < rcCaptionRuntime.height();
}
*/
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy