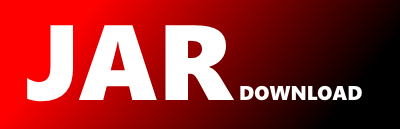
com.adobe.xfa.layout.FormLayout Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.layout;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.font.FontService;
import com.adobe.xfa.service.Service;
import com.adobe.xfa.service.href.HrefService;
/**
* This object represents a high level transformation that acts on a given FormModel
* and completely describes the final layout of a form.
*
*
* The FormLayout object encapsulates the following information:
* i) what type of object to render (form node)
* ii) what page the object resides
* iii) object's (x,y) position on a given page
* It does so by storing an array of layout node trees, with each tree representing
* the layout of a new page.
*
* The layout hierarchy is comprised of LayoutNode objects.
* Instances of the LayoutNode class are assembled in parent/child relationships, each
* having an association with a single Form Model node.
* A LayoutNode represent a frame of reference that applies a coordinate space
* transformation to it's form node as well as any child layout nodes.
*
* The FormLayout isructed by calling FormLayout::layout() member function
* Upon return it completely describes where all page breaks have occurred and where every object
* has been positioned.
*
* Consider the form outlined below:
*
* <subform name="SFr">
* <pageSet>
* <pageArea name="P1">
* <draw name="D0" />
* <contentArea name="CA1" />
* <contentArea name="CA2" />
* </pageArea>
* </pageSet>
*
* <subform name="SF1">
* <field name="F1" />
* <field name="F2" />
* <field name="F3" />
* </subform>
* </subform>
*
*
* Assume for this example that both fields F1,F2 fit on page 1, content area 1, but they fill content area 1
* completely. F3 then starts on page 1, content area 2, and assume it overflows to page 2, content
* area 1.
* The corresponding FormLayout structure would look
* as follows:
*
*
* FormLayout
*
* Pages[0]: LN (P1)
* |
* |-LN (D0)
* |-LN (CA1)
* | |-LN (SFr)
* | |-LN (SF1)
* | |-LN (F1)
* | |-LN (F2)
* |-LN (CA2)
* |-LN (SFr)
* |-LN (SF1)
* |-LN (F3)
*
* Pages[1]: LN (P1)
* |
* |-LN (D0)
* |-LN (CA1)
* |-LN (SFr)
* |-LN (SF1)
* |-LN (F3)
*
*
*
* Legend
* LN(x) layout node associated with form node 'x'
*
*
* @exclude from published api.
*/
public class FormLayout extends Layout {
public FormLayout(AppModel oAppModel) {
super(oAppModel);
assert(oAppModel != null);
// moRuntimeTextResolver = oAppModel;
mbNeedSecondPass = false;
mbSecondPass = false;
// moDebuggerData = null;
// moBoxModelPool.extendSize(64);
// if (XFADebugger::isActive()) {
// //Basically register this layout with the debugger.
// moDebuggerData = new XFADebuggerLayoutData(this);
// Debugger.getDebugger().setLayoutData(moDebuggerData);
// }
}
public FormLayout(FontService oFontService, /*Image*/Service oImageService, HrefService oHrefService, AppModel oAppModel) {
super(oAppModel);
assert(oAppModel != null);
assert(oFontService != null);
assert(oImageService != null);
assert(oHrefService != null);
// moRuntimeTextResolver = oAppModel;
mbNeedSecondPass = false;
mbSecondPass = false;
// moDebuggerData = null;
moLayoutDriver = new LayoutDriver();
// moBoxModelPool.extendSize(64);
LayoutEnv oEnv = new LayoutEnv(oFontService, oImageService, oHrefService, moLayoutDriver);
// moRuntimeTextResolver.registerTextResolver(poEnv);
super.setLayoutEnv(oEnv);
// if (XFADebugger::isActive()) {
// //Basically register this layout with the debugger.
// moDebuggerData = new XFADebuggerLayoutData(this);
// Debugger.getDebugger().setLayoutData(moDebuggerData);
// }
}
// public FormLayout(FontService oFontService, ImageService oImageService, HrefService oHrefService, TextContext oTextContext, AppModel oAppModel);
/**
* Perform a full or incremental layout as indicated.
* @param bFullLayout true indicates a full layout is to be
* performed. false indicates that an incremental layout is
* to be attempted.
*/
public void layout(boolean bFullLayout /* = true */) {
// JavaPort: TODO
}
// /**
// * Create a new LayoutNodeNode around a given Node.
// * @param oNode xfanode for which this layout node applies
// * @return an LayoutNode.
// * Node returned is orphaned, i.e. not a member of
// * any page hierarchy.
// */
// public LayoutNode createNode(Node oNode);
// public LayoutNode createNode(Node oNode, BoxModelLayout oBoxModel);
// /**
// * Create and return the entire layout subhierarchy for a given node.
// * This returned layout tree represents the layout oNode and all it's children.
// * This is to provide an opportunity for xfalayouts to shortcut the regular layout building for this xfanode.
// * FormLayout always returns null layout nodel causing oNode layout to traversed layout
// * created in the regular manner
// */
// public LayoutNode createLayoutTree(Node oNode);
// /**
// * Query for the leader or trailer Subform with given id.
// * It is layout's responsibility to record occur information
// * and determine whether this is in fact the next leader/trailer
// * available.
// * @param sReference reference to a subform to use as a leader/trailer, either by ID or SOM
// * @param oParentNode parent context under which to create a leader/trailer
// * false indicates the bookend leader/trailer is required.
// * @param bPeek true if layout node is requested without modifying model
// * @return an LayoutNode.
// */
// public Node getLeaderNode(String sReference, Node oParentNode, boolean bPeek);
// public Node getTrailerNode(String sReference,Node oParentNode, boolean bPeek);
// /**
// * Notify the layout of a node that is relevant to layout
// * but is not directly present within the layout itself.
// * Such nodes are referred to as 'anonymous'
// * i.e. xfa nodes that don't have corresponding layout nodes
// * such as subformSets, pageSets, exclgroups.
// */
// public void notifyAnonymousNode(Node oNode);
// /**
// * Notify the layout that a new pageSet scope is to be begin.
// * Currently only relevant for subforms.
// */
// public void setPageSetScope(Node oNode);
// /**
// * Notify the layout that a pageSet scope may be coming to an end.
// * Currently only relevant for subforms
// **/
// public void resetPageSetScope(Node oNode);
// /**
// * Reset the layout
// * The layout is empty upon return (no pages)
// * This function allows the optional exception of any pooled resources that may be
// * useful for a subsequent layout pass, ie between a failed incremental attempt
// * in preparation for a full layout.
// * @param bClearPooledResources boolean to indicate whether to also reset any pooled resources
// * In general external classes should use reset() or reset(true)
// */
// public void reset(boolean bClearPooledResources /* = true */);
// /**
// * Add a page
// * @param oPageLayoutNode root node of page
// * @param bFront true indicates the given page represents a front, false for back
// */
// public void addPage(LayoutNode oPageLayoutNode, boolean bFront);
// /**
// * Remove the last page
// */
// public void removeLastPage();
// /*
// * Return the FormModel used for this layout;
// * @return FormModel.
// */
// public FormModel getFormModel();
// /**
// * Register the $layout pseudo model that
// * is bound to this form layout.
// * Throws an exception if a $layout already exists.
// * param bMarkAsReady - true if layout pseudo model is to be marked as ready
// */
// public void registerPseudoModel(boolean bMarkAsReady /* = false */);
// /**
// * Un-register the $layout pseudo model that
// * is bound to this form layout by removing
// * from the app model.
// * May be called at any time as well it is called
// * in FormLayout destructor.
// */
// public void unRegisterPseudoModel();
//$layout helpers
// /**
// * Relayout the pageArea content for a given page
// * @param nPageNum the page number for the page background to relayout
// */
// public void relayoutPageArea(int nPageNum);
// /**
// * Get the absolute number of pages
// * @param bBatchContext if true get the page count for all layouts, else
// * get the page count for this layout
// * @param bScripting if true the call is made from scripting, and will
// * require a second pass.
// * @param bTotal only checked on the second pass : if true return the
// * current batch count, otherwise the complete batch total. (Added because
// * both values need to be returned on the second pass.)
// * @return the number of pages
// */
// public int getAbsPageCount(boolean bBatchContext /* = false */, boolean bScripting /* = false */, boolean bTotal /* = true */);
// /**
// * Get the number of logical pages
// * @param bBatchContext if true get the page count for all layouts, else
// * get the page count for this layout
// * @param bScripting if true the call is made from scripting, and will
// * require a second pass.
// * @return the number of pages
// */
// public int getLogicalPageCount(boolean bBatchContext /* = false */, boolean bScripting /* = false */);
// /**
// * Get the number of sheets
// * @param bBatchContext if true get the sheet count for all layouts, else
// * get the sheet count for this layout
// * @param bScripting if true the call is made from scripting, and will
// * require a second pass.
// * @param bTotal only checked on the second pass : if true return the
// * current batch count, otherwise the complete batch total. (Added because
// * both values need to be returned on the second pass.)
// * @return the number of pages
// */
// public int getSheetCount(boolean bBatchContext /*= false */, boolean bScripting /*= false */, boolean bTotal /*= true */);
// /**
// * Get the logical page number for an abs page num
// * @param nPage abs local page num
// * @param bBatchContext if true get the page number in context of all layouts, else
// * get the page num for this layout
// * @param bScripting if true the call is made from scripting, and will
// * require a second pass.
// * @return logical page number (1 based)
// */
// public int getLogicalPageNum(int nPage, boolean /* bBatchContext = false */); // one based, 0 if not counted
/**
* Check if we need to perform a second pass layout of the remaining records
* @return true if a second pass is need, else false
* Inclusive of the current record
*/
public boolean needsSecondPass() {
return mbNeedSecondPass;
}
/**
* Notifies layout that a second pass will occur
* This is called by the $host document counting methods.
*/
public void setNeedsSecondPass() {
mbNeedSecondPass = ! mbSecondPass;
}
// /**
// * Notifies layout that we are on the second pass
// * @param nAbsPageCount starting page count
// * @param nLogicalPageCount starting page count
// */
// public void startSecondPass(int nAbsPageCount, int nLogicalPageCount, int nSheetCount);
// /**
// * Notifies layout that we the second pass is complete
// */
// public void endSecondPass();
// /**
// * Repair any damged layout nodes that occurred from $layout:ready scripts
// * and on fields/draws
// * Only call if some script fires that changes the Form DOM post $layout:ready.
// */
// public void repairLayoutReadyDamage();
// /**
// * Rest the page counts
// * @param bFullRest true if all counts are to be reset to 0, false
// * for the batch count to be reset to the state prior to the last layout
// * Only call if starting a new job.
// */
// public void resetPageCounts(boolean bFullReset /* = true */);
// /**
// * Utility function that indicates whether the given xfanode contains render cache
// * information (i.e. renderCache processing instructions)
// * Such a node would have been deemed render-proxyable at design-save time
// * for this to have occurred.
// */
// public boolean hasRenderProxyInfo(Node oNode);
// /**
// * Utility function to disable/enable rendercache.
// * @param bEnable boolean parameter, true enables
// * the use of renderCache, false disables it.
// */
// public void enableRenderCachedNodes(boolean bEnable) {
// mbAllowRenderCachedNodes = bEnable;
// }
// /**
// * Protected ctor that allows derived classes to provide a custom LayoutEnv.
// */
// protected FormLayout(AppModel oAppModel);
/**
* Returns the GfxDriver used for form based layout.
*/
protected LayoutDriver getLayoutDriver() {
return moLayoutDriver;
}
// protected LayoutTextResolver getTextResolver() {
// return &moRuntimeTextResolver;
// }
// protected void layout(Subform oSubform);
// protected Subform getRootSubform();
// protected PageSet getRootPageSet();
// /**
// * Allow a layout object to perform any operations that need to occur after
// * a layout has completed.
// */
// protected void postLayout();
// /**
// * Called when a layout has completed
// */
// protected void ready();
// /**
// * Return true if the layout supports incremental relayout
// * and false otherwise.
// * FormLayout supports incremental relayout to a small degree.
// * That is, it will repair damage done by $layout:ready scripts but nothing more.
// * It is the responsibility of the script writer to request a full layout for scripts
// * that modify the form model to any real degree or to form nodes other than fields/draws.
// */
// protected boolean supportsIncrementalLayout();
// /**
// * Remove all pages
// */
// protected void removeAllPages();
// /**
// * Kick off an incremental layout
// * @return true if incremental layout was successfully completed
// * and false otherwise. FormLayout must always return true.
// * FormLayout supports a very limited incremental layout in that
// * it will only repair damage to fields/draws a result of $layout:ready events.
// * It is the responsibility of the script writer to request a full layout for scripts
// * that modify the form model to any real degree or to form nodes other than fields/draws.
// */
// protected boolean incrementalLayout();
// /**
// * Called after a successful full layout
// * has finished
// */
// protected void postFullLayout();
// /**
// * Called before a full layout is about to proceed.
// */
// protected void preFullLayout();
// /**
// * Add the given page context to the debugger-viewable data
// */
// protected void setDebuggerPageContext(LayoutPageContext oPageContext);
// protected void clearDebuggerPageContext();
//private data
// private boolean mbRegisteredPseudoModel = false;
// private boolean mbDamaged = false;
//Text engine callback object to resolve embedded text references at runtime
// private LayoutTextResolver moRuntimeTextResolver;
//gfx driver for form based layout
private LayoutDriver moLayoutDriver;
// private Storage moPagesNum; // used to store the logical page number
private boolean mbNeedSecondPass;
private boolean mbSecondPass;
// private boolean mbResetPageNum = false;
// private boolean mbHaveNumberedPage = false; // tells us if there is a numberd page
// private int mnAbsPageCount = 0; // count for this layout
// private int mnBatchAbsPageCount = 0; // count of all previous layouts
// private int /nBatchAbsPageCountTotal = 0; // final calculated page count
// private int mnLocicalPageCount = 0; // count for this layout
// private int mnBatchLogicalPageCountt = 0; // count of all previous layouts
// private int mnBatchLogicalPageCountTotalt = 0; // final calculated page count
// private int mnSheetCount = 0; // count for this layout
// private int mnBatchSheetCount = 0; // count of all previous layouts
// private int mnBatchSheetCountTotal = 0; // final calculated page count
// private void updateBoxModels(LayoutNode oNode);
// private void updateBoxModels(LayoutNode oLayoutNode, BoxModelLayout oNewBM);
// private SortedArray moBoxModelPool;
//Control whether render cached box models will be allowed
// private boolean mbAllowRenderCachedNodes = true;
//Pointer to debugger data, if debugging is present
// private DebuggerLayoutData moDebuggerData;
// private boolean isPoolableBoxModel(Node oNode);
// look to see if a boxModel is already pooled... if it is not and it is poolable then
// the boxModel will be created and added to the pool only if bAddToPool is true... otherwise
// (and if the node is not poolable) this method returns an null boxModel
// private BoxModelLayout lookupPooledBoxModel(Node oNode, boolean bAddToPool);
// private boolean isPageAreaBackground(Node oNode);
//Get the pageSet defined on the template side of things for the given subform.
// private PageSet getTemplatePageSet(FormSubform oFormSF);
//hide these
// private FormLayout();
// private FormLayout(FormLayout oObj);
// private FormLayout operator=(FormLayout right);
}