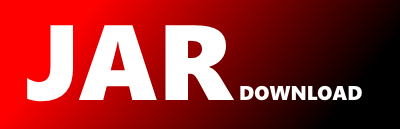
com.adobe.xfa.layout.LayoutDriver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.layout;
import com.adobe.xfa.Element;
import com.adobe.xfa.EnumAttr;
import com.adobe.xfa.Model;
import com.adobe.xfa.Measurement;
import com.adobe.xfa.XFA;
import com.adobe.xfa.content.RectangleValue;
import com.adobe.xfa.font.FontInstance;
import com.adobe.xfa.font.GfxTextAttrs;
import com.adobe.xfa.gfx.GFXAttr;
import com.adobe.xfa.gfx.GFXDriver;
import com.adobe.xfa.gfx.GFXGlyphOrientation;
import com.adobe.xfa.gfx.GFXLineAttr;
import com.adobe.xfa.template.formatting.Color;
import com.adobe.xfa.ut.CoordPair;
import com.adobe.xfa.ut.Rect;
import com.adobe.xfa.ut.UnitSpan;
import com.adobe.xfa.gfx.GFXMappingList;
/**
* A superclass of GFXDriver used by the layout package.
*
* @exclude from published api.
*/
public class LayoutDriver extends GFXDriver {
/**
* Default c'tor
*
* @exclude from published api.
*/
public LayoutDriver() {
super(null);
moEnv = null;
mnMappingLevel = GFXDriver.MAPPING_SUPPRESS;
initDeviceUnitsPerInch(1200, 1200);
}
/**
* Constructor, creates an LayoutDriver object.
*
* @exclude from published api.
*/
public LayoutDriver(LayoutEnv oEnv) {
super(oEnv);
moEnv = oEnv;
mnMappingLevel = GFXDriver.MAPPING_SUPPRESS;
initDeviceUnitsPerInch(1200, 1200);
}
// /**
// * Hidden constructor.
// */
// private LayoutDriver(LayoutDriver obj) {
// }
/**
* Set the gfx environment
*
* @exclude from published api.
*/
public void setLayoutEnv(LayoutEnv oEnv) {
moEnv = oEnv;
}
// Absolute Drawing Methods
/**
* @exclude from published api.
*/
public void absLine(CoordPair oEnd, int eDrawMode /* = DRAW_2D */) {
LayoutHandler oLayoutHandler = layoutHandler();
if (oLayoutHandler == null)
return;
//
// Create an Rectangle
//
Model model = oLayoutHandler.getModel();
Element oLine = model.createElement(XFA.LINETAG, XFA.LINE);
//
// Set the handedness
//
switch (lineAttr().hand()) {
case GFXLineAttr.HAND_LEFT:
oLine.setAttribute(EnumAttr.HAND_LEFT, XFA.HANDTAG);
break;
case GFXLineAttr.HAND_RIGHT:
oLine.setAttribute(EnumAttr.HAND_RIGHT, XFA.HANDTAG);
break;
default:
oLine.setAttribute(EnumAttr.HAND_EVEN, XFA.HANDTAG);
}
//
// Get the edge. This will create a default property which we'll modify
//
Element oEdge = oLine.getElement(XFA.EDGETAG, 0);
switch (lineAttr().cap()) {
case GFXLineAttr.CAP_BUTT:
oEdge.setAttribute(EnumAttr.BUTT, XFA.CAPTAG);
break;
case GFXLineAttr.CAP_ROUND:
oEdge.setAttribute(EnumAttr.ROUND, XFA.CAPTAG);
break;
default:
oEdge.setAttribute(EnumAttr.SQUARE, XFA.CAPTAG);
}
Color oColor = (Color) oEdge.getElement(XFA.COLORTAG, 0);
oColor.setRed(lineAttr().colour().r());
oColor.setGreen(lineAttr().colour().g());
oColor.setBlue(lineAttr().colour().b());
//
// Set the thickness
//
oEdge.setAttribute(new Measurement(lineAttr().width()), XFA.THICKNESSTAG);
//
// Setup the style for the edge.
//
switch (lineAttr().style()) {
case GFXAttr.STYLE_UNKNOWN:
break;
case GFXAttr.STYLE_NONE:
break;
case GFXAttr.STYLE_SOLID:
oEdge.setAttribute(EnumAttr.STROKE_SOLID, XFA.STROKETAG);
break;
case GFXAttr.STYLE_DOT:
oEdge.setAttribute(EnumAttr.STROKE_DOTTED, XFA.STROKETAG);
break;
case GFXAttr.STYLE_DASH:
oEdge.setAttribute(EnumAttr.STROKE_DASHED, XFA.STROKETAG);
break;
case GFXAttr.STYLE_DOT_DASH:
oEdge.setAttribute(EnumAttr.STROKE_DASHDOT, XFA.STROKETAG);
break;
case GFXAttr.STYLE_DOT_DOT_DASH:
oEdge.setAttribute(EnumAttr.STROKE_DASHDOTDOT, XFA.STROKETAG);
break;
}
//
// Get the layout handler to render the line
//
CoordPair oP1 = rotatePoint(getAbsPosition());
CoordPair oP2 = rotatePoint(oEnd);
oLayoutHandler.drawLine(new Measurement(oP1.x()),
new Measurement(oP1.y()),
new Measurement(oP2.x().subtract(oP1.x())),
new Measurement(oP2.y().subtract(oP1.y())),
oLine);
setAbsPosition(oEnd);
}
/**
* @exclude from published api.
*/
public void absFillRect(Rect oRect, int eDrawMode /* = DRAW_2D */) {
LayoutHandler oLayoutHandler = layoutHandler();
if (oLayoutHandler == null)
return;
//UnitSpan oW = oRect.width();
//
// Create an Rectangle
//
Model model = oLayoutHandler.getModel();
RectangleValue oRectangle = (RectangleValue) model.createElement(XFA.RECTANGLETAG, XFA.RECTANGLE);
oRectangle.setAttribute(EnumAttr.HAND_RIGHT, XFA.HANDTAG);
//
// OK, Let's deal with the fill first.
//
Element oFill = oRectangle.getElement(XFA.FILLTAG, 0);
//
// Set the Fill Colour if specified
//
Color oColor = (Color) model.createElement(XFA.COLORTAG, XFA.COLOR);
oColor.setRed(fillAttr().colour().r());
oColor.setGreen(fillAttr().colour().g());
oColor.setBlue(fillAttr().colour().b());
oFill.setElement(oColor, XFA.COLORTAG, 0);
//
// Set the Style/Pattern
//
Element oPattern = null;
switch (fillAttr().style()) {
case GFXAttr.STYLE_UNKNOWN:
case GFXAttr.STYLE_NONE:
break;
case GFXAttr.STYLE_SOLID: {
Element oSolid = model.createElement(XFA.SOLIDTAG, XFA.SOLID);
oFill.setOneOfChild(oSolid);
break;
}
case GFXAttr.STYLE_HORZ:
oPattern = oFill.getElement(XFA.PATTERNTAG, 0);
oPattern.setAttribute(EnumAttr.HORIZONTAL_HATCHING, XFA.TYPETAG);
break;
case GFXAttr.STYLE_VERT:
oPattern = oFill.getElement(XFA.PATTERNTAG, 0);
oPattern.setAttribute(EnumAttr.VERTICAL_HATCHING, XFA.TYPETAG);
break;
case GFXAttr.STYLE_CROSS:
oPattern = oFill.getElement(XFA.PATTERNTAG, 0);
oPattern.setAttribute(EnumAttr.CROSS_HATCHING, XFA.TYPETAG);
break;
case GFXAttr.STYLE_DIAG_LEFT:
oPattern = oFill.getElement(XFA.PATTERNTAG, 0);
oPattern.setAttribute(EnumAttr.DIAGONAL_LEFT_HATCHING, XFA.TYPETAG);
break;
case GFXAttr.STYLE_DIAG_RIGHT:
oPattern = oFill.getElement(XFA.PATTERNTAG, 0);
oPattern.setAttribute(EnumAttr.DIAGONAL_RIGHT_HATCHING, XFA.TYPETAG);
break;
case GFXAttr.STYLE_DIAG_CROSS:
oPattern = oFill.getElement(XFA.PATTERNTAG, 0);
oPattern.setAttribute(EnumAttr.CROSS_DIAGONAL_HATCHING, XFA.TYPETAG);
break;
}
//
// OK, Fill's done, now let's setup the non-existent edge.
//
Element oEdge = oRectangle.getElement(XFA.EDGETAG, 0);
oEdge.setAttribute(new Measurement(UnitSpan.ZERO), XFA.THICKNESSTAG);
//
// Get the layout handler to render the rectangle
//
oLayoutHandler.drawRect(new Measurement(oRect.left()),
new Measurement(oRect.top()),
new Measurement(oRect.width()),
new Measurement(oRect.height()),
oRectangle);
}
/**
* @exclude from published api.
*/
public void absText(String oText, int eDrawMode /* = DRAW_2D */) {
LayoutHandler oLayoutHandler = layoutHandler();
if (oLayoutHandler == null)
return;
//
//Set the clip rect in the layout handler. The handler can choose to enforce it or not.
//
oLayoutHandler.setClipRect(moRect);
//
// Get the model so we can create objects.
//
//Model model = oLayoutHandler.getModel();
//LayoutEnv oEnv = (LayoutEnv) env();
FontInstance oFontInstance = fontInstance();
assert(oFontInstance != null);
GfxTextAttrs oAttrs = new GfxTextAttrs(
textAttr().colour(),
textAttr().underline(),
textAttr().overline(),
textAttr().strikeout(),
UnitSpan.ZERO,
GFXGlyphOrientation.HORIZONTAL);
//
// Get the layout handler to render the text
//
String sNonConst = oText; // TBD: does handler really need non-string?
oLayoutHandler.handleTextRun(new Measurement(getAbsPosition().x()),
new Measurement(getAbsPosition().y()),
new Measurement(new UnitSpan(0, UnitSpan.POINTS_1K)),
new Measurement(new UnitSpan(0, UnitSpan.POINTS_1K)),
sNonConst,
oFontInstance,
oAttrs);
//Reset clip rect
moRect = Rect.ZERO;
oLayoutHandler.setClipRect(moRect);
}
/**
* @exclude from published api.
*/
public void absGlyphs(int[] nGlyphs, int nSize) {
LayoutHandler oLayoutHandler = layoutHandler();
if (oLayoutHandler == null)
return;
//Set the clip rect in the layout handler. The handler can choose to enforce it or not.
oLayoutHandler.setClipRect(moRect);
//
// Get the model so we can create objects.
//
//Model model = oLayoutHandler.getModel();
FontInstance oFontInstance = fontInstance();
assert(oFontInstance != null);
GfxTextAttrs oAttrs = new GfxTextAttrs(
textAttr().colour(),
textAttr().underline(),
textAttr().overline(),
textAttr().strikeout(),
UnitSpan.ZERO,
glyphOrientation());
//
// Get the layout handler to render the glyphs
//
oLayoutHandler.handleGlyphRun(new Measurement(getAbsPosition().x()),
new Measurement(getAbsPosition().y()),
new Measurement(new UnitSpan(0, UnitSpan.POINTS_1K)),
new Measurement(new UnitSpan(0, UnitSpan.POINTS_1K)),
nGlyphs,
nSize,
oFontInstance,
oAttrs);
//
// Reset clip rect
//
moRect = Rect.ZERO;
oLayoutHandler.setClipRect(moRect);
}
// Character/glyph mapping methods
// SetUnicodeChars and MapGlyphs are needed to pass unicode/glyph mappings along to
// the cache driver.
public void setUnicodeChars (String text, int nSize) {
LayoutHandler poLayoutHandler = layoutHandler();
if (poLayoutHandler == null)
return;
poLayoutHandler.handleUnicodeChars(text, nSize);
}
public void mapGlyphs(GFXMappingList mappingList, boolean bIsRTL) {
LayoutHandler poLayoutHandler = layoutHandler();
if (poLayoutHandler == null)
return;
poLayoutHandler.handleGlyphMapping(mappingList, bIsRTL);
}
/**
* @exclude from published api.
*/
public int getMappingLevel() {
return mnMappingLevel;
}
/**
* @exclude from published api.
*/
public void setMappingLevel(int nSLevel) {
mnMappingLevel = nSLevel;
}
// Device Property Querying Methods
// Javaport: TODO
// public GFXDevInfo devInfo() {
// return GFXDevInfo(type(), DotsPerInch(),
// GFXDevInfo.DOT_FRACTIONAL_MONO); // 4.x compatibility kludge
// }
/**
* @exclude from published api.
*/
public int height() {
return moRect.height().valueAsUnit(UnitSpan.INCHES_72K);
}
/**
* @exclude from published api.
*/
public int width() {
return moRect.width().valueAsUnit(UnitSpan.INCHES_72K);
}
/**
* @exclude from published api.
*/
public boolean interactive() {
return false;
}
// Device Property Querying Methods
// Javaport: TODO
// public GFXFont reconcile(GFXFont oSource, char pcRequired) {
// // the gfxfont interface into the text engine is depricated
// assert(false);
// return null;
// }
// Javaport: TODO
// public void useChar(GFXFont oSource, char pcChar) {
// // the gfxfont interface into the text engine is depricated
// assert(false);
// return;
// }
/**
* @exclude from published api.
*/
public void setClipRect(Rect oRect) {
moRect = oRect;
}
/**
* @exclude from published api.
*/
public void paraHint() {
LayoutHandler oLayoutHandler = layoutHandler();
if (oLayoutHandler != null)
oLayoutHandler.newPara();
}
// Other methods
/**
* @exclude from published api.
*/
protected LayoutHandler layoutHandler() {
if (moEnv != null)
return moEnv.layoutHandler();
else
return null;
}
/**
* @exclude from published api.
*/
protected LayoutEnv layoutEnv() {
return moEnv;
}
/**
* @exclude from published api.
*/
protected Rect moRect = Rect.ZERO;
private LayoutEnv moEnv;
// By suppressing mapping, AXTE won't go to the effort of generating full
// mapping definitions and it won't create unnecessary run breaks for
// legacy mapping.
private int mnMappingLevel;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy