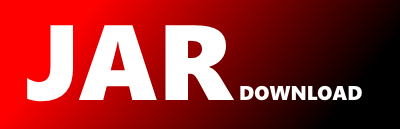
com.adobe.xfa.pmp.adobepdf417pmp.PDF417ByteCompactor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* File: PDF417ByteCompactor.java
*
**************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.xfa.pmp.adobepdf417pmp;
import java.util.List;
/**
* Ported from PDF417ByteCompactor.cpp
*/
class PDF417ByteCompactor {
// ////////////////////////////////////////////////////////////////////
/**
* Do the byte compaction.
*
* @param message
* - The input binary message.
* @returns A vector of the of the binary encoded message in PDF417 code
* words.
*/
// ////////////////////////////////////////////////////////////////////
static void compact(List message, List compactedMessage) {
// Leave remove for compaction mode indicator.
compactedMessage.add(0);
// Create two utility vectors for coverting from base 2 to base 900.
char[] base256 = new char[6];
int[] base900 = new int[5];
// Do the compaction
int srcIdx = 0;
while ((srcIdx + 6) <= message.size()) {
base256[0] = message.get(srcIdx++);
base256[1] = message.get(srcIdx++);
base256[2] = message.get(srcIdx++);
base256[3] = message.get(srcIdx++);
base256[4] = message.get(srcIdx++);
base256[5] = message.get(srcIdx++);
base256ToBase900(base256, base900);
compactedMessage.add(base900[0]);
compactedMessage.add(base900[1]);
compactedMessage.add(base900[2]);
compactedMessage.add(base900[3]);
compactedMessage.add(base900[4]);
}
// Handle any reminding bytes
if (srcIdx != message.size()) {
compactedMessage.set(0, PDF417SpecialCode.ByteCompactionLatch0
.getValue());
while (srcIdx < message.size())
compactedMessage.add((int) message.get(srcIdx++));
} else {
compactedMessage.set(0, PDF417SpecialCode.ByteCompactionLatch1
.getValue());
}
}
// ////////////////////////////////////////////////////////////////////
/**
* Converts a base 256 number to base 900.
*/
// ////////////////////////////////////////////////////////////////////
static void base256ToBase900(char[] in, int[] out) {
// [dsiu] _int64 is NOT a portable type. use PMPInt64 instead
long v;
//long b = in[0];
long v1 = in[0];
v1 <<= 40;
long v2 = in[1];
v2 <<= 32;
long v3 = in[2];
v3 <<= 24;
long v4 = in[3];
v4 <<= 16;
long v5 = in[4];
v5 <<= 8;
long v6 = in[5];
v = v1 | v2 | v3 | v4 | v5 | v6;
int c;
int idx;
for (idx = 4; idx >= 0; idx--) {
c = (int) (v % 900);
v = v / 900;
out[idx] = c;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy