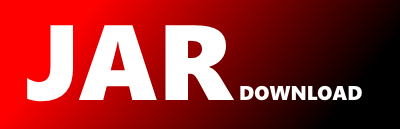
com.adobe.xfa.pmp.datamatrixpmp.DataMatrixReedSolomon Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* File: DataMatrixReedSolomon.java
*
**************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.xfa.pmp.datamatrixpmp;
import java.util.List;
/**
* Ported from DataMatrixReedSolomon.cpp
*/
class DataMatrixReedSolomon {
private static final int GEN = 0x2d;
private static int m_gfpwr[] = new int[1000];
private static int m_gflog[] = new int[1000];
// ////////////////////////////////////////////////////////////////////
/**
* Generator Polynomials.
*/
// ////////////////////////////////////////////////////////////////////
final static int m_poly5[] = { 62, 111, 15, 48, 228 };
final static int m_poly7[] = { 254, 92, 240, 134, 144, 68, 23 };
final static int m_poly10[] = { 61, 110, 255, 116, 248, 223, 166, 185, 24,
28 };
final static int m_poly11[] = { 120, 97, 60, 245, 39, 168, 194, 12, 205,
138, 175 };
final static int m_poly12[] = { 242, 100, 178, 97, 213, 142, 42, 61, 91,
158, 153, 41 };
final static int m_poly14[] = { 185, 83, 186, 18, 45, 138, 119, 157, 9, 95,
252, 192, 97, 156 };
final static int m_poly18[] = { 188, 90, 48, 225, 254, 94, 129, 109, 213,
241, 61, 66, 75, 188, 39, 100, 195, 83 };
final static int m_poly20[] = { 172, 186, 174, 27, 82, 108, 79, 253, 145,
153, 160, 188, 2, 168, 71, 233, 9, 244, 195, 15 };
final static int m_poly24[] = { 193, 50, 96, 184, 181, 12, 124, 254, 172,
5, 21, 155, 223, 251, 197, 155, 21, 176, 39, 109, 205, 88, 190, 52 };
final static int m_poly28[] = { 255, 93, 168, 233, 151, 120, 136, 141, 213,
110, 138, 17, 121, 249, 34, 75, 53, 170, 151, 37, 174, 103, 96, 71,
97, 43, 231, 211 };
final static int m_poly36[] = { 112, 81, 98, 225, 25, 59, 184, 175, 44,
115, 119, 95, 137, 101, 33, 68, 4, 2, 18, 229, 182, 80, 251, 220,
179, 84, 120, 102, 181, 162, 250, 130, 218, 242, 127, 245 };
final static int m_poly42[] = { 5, 9, 5, 226, 177, 150, 50, 69, 202, 248,
101, 54, 57, 253, 1, 21, 121, 57, 111, 214, 105, 167, 9, 100, 95,
175, 8, 242, 133, 245, 2, 122, 105, 247, 153, 22, 38, 19, 31, 137,
193, 77 };
final static int m_poly48[] = { 19, 225, 253, 92, 213, 69, 175, 160, 147,
187, 87, 176, 44, 82, 240, 186, 138, 66, 100, 120, 88, 131, 205,
170, 90, 37, 23, 118, 147, 16, 106, 191, 87, 237, 188, 205, 231,
238, 133, 238, 22, 117, 32, 96, 223, 172, 132, 245 };
final static int m_poly56[] = { 46, 143, 53, 233, 107, 203, 43, 155, 28,
247, 67, 127, 245, 137, 13, 164, 207, 62, 117, 201, 150, 22, 238,
144, 232, 29, 203, 117, 234, 218, 146, 228, 54, 132, 200, 38, 223,
36, 159, 150, 235, 215, 192, 230, 170, 175, 29, 100, 208, 220, 17,
12, 238, 223, 9, 175 };
final static int m_poly62[] = { 204, 11, 47, 86, 124, 224, 166, 94, 7, 232,
107, 4, 170, 176, 31, 163, 17, 188, 130, 40, 10, 87, 63, 51, 218,
27, 6, 147, 44, 161, 71, 114, 64, 175, 221, 185, 106, 250, 190,
197, 63, 245, 230, 134, 112, 185, 37, 196, 108, 143, 189, 201, 188,
202, 118, 39, 210, 144, 50, 169, 93, 242 };
final static int m_poly68[] = { 186, 82, 103, 96, 63, 132, 153, 108, 54,
64, 189, 211, 232, 49, 25, 172, 52, 59, 241, 181, 239, 223, 136,
231, 210, 96, 232, 220, 25, 179, 167, 202, 185, 153, 139, 66, 236,
227, 160, 15, 213, 93, 122, 68, 177, 158, 197, 234, 180, 248, 136,
213, 127, 73, 36, 154, 244, 147, 33, 89, 56, 159, 149, 251, 89,
173, 228, 220 };
static {
initLogTables();
}
static void initLogTables() {
int i, j;
for (j = 0; j < 256; j++) {
m_gflog[j] = 0;
}
i = 1;
for (j = 0; j < 255; j++) {
m_gfpwr[j] = i;
m_gflog[i] = j;
i <<= 1;
if (i > 255) {
i ^= GEN + 256;
}
}
}
// ////////////////////////////////////////////////////////////////////
/**
* Add the ECC code words to the message.
*
* param pMessage
* - The message that needs ECC added.
* param level
* - The PDF417 ECC level.
*
* Output - The ECC code words are appended to the end of
* pMessage.
* @throws DataMatrixEncoderException
*/
// ////////////////////////////////////////////////////////////////////
static void addECC(List data, // In/Out: The message to be placed
// in the bacode.
int symbolSize) throws DataMatrixEncoderException // In: The desired
// symbol size.
{
// Calculate Code 1 version, interleave for F & G need to be added
int dsize = DataMatrixInfo.DATA_SYMBOLS[symbolSize];
int csize = DataMatrixInfo.CHECK_SYMBOLS[symbolSize];
int isize = DataMatrixInfo.NUM_INTERLEAVES[symbolSize];
// Add the initialized ECC code words
int i;
for (i = 0; i < csize; i++)
data.add(0);
// Get the generator polynomial
int[] p = null;
switch (csize / isize) {
case 5:
p = m_poly5;
break;
case 7:
p = m_poly7;
break;
case 10:
p = m_poly10;
break;
case 11:
p = m_poly11;
break;
case 12:
p = m_poly12;
break;
case 14:
p = m_poly14;
break;
case 18:
p = m_poly18;
break;
case 20:
p = m_poly20;
break;
case 24:
p = m_poly24;
break;
case 28:
p = m_poly28;
break;
case 36:
p = m_poly36;
break;
case 42:
p = m_poly42;
break;
case 48:
p = m_poly48;
break;
case 56:
p = m_poly56;
break;
case 62:
p = m_poly62;
break;
case 68:
p = m_poly68;
break;
default:
throw new DataMatrixEncoderException(
DataMatrixEncoderErrorCode.SYMBOL_SIZE);
}
// Calculate the ECC codewords for each block.
int v;
for (v = 0; v < isize; v++) {
int n;
// For 144 symbols, there are 10 ECC blocks, but the last two blocks
// only have 155 codewords.
int dataBlockSize = dsize
/ isize
+ ((symbolSize == DataMatrixInfo.Sizes.SIZE_144_144.value)
& (v < 8) ? 1 : 0);
for (n = 0; n < dataBlockSize; n++) {
int t = data.get(v + dsize) ^ data.get(v + (n * isize));
int k;
for (i = v + dsize, k = 0; i < dsize + csize - isize; i += isize, k++) {
data.set(i, data.get(i + isize) ^ (GFmul(t, p[k])));
}
data.set(v + dsize + csize - isize, (GFmul(t,
p[(csize / isize) - 1])));
}
}
}
// ////////////////////////////////////////////////////////////////////
/**
* Galios Field Multiplication.
*/
// ////////////////////////////////////////////////////////////////////
static int GFmul(int p1, int p2) {
int i;
if ((p1 == 0) || (p2 == 0))
return (0);
i = m_gflog[p1] + m_gflog[p2];
if (i > 254)
i -= 255;
i = m_gfpwr[i];
return (i);
}
// ////////////////////////////////////////////////////////////////////
/**
* Galios Field Division.
*/
// ////////////////////////////////////////////////////////////////////
static int GFdiv(int p1, int p2) {
int i;
if (p2 == 0)
return (0);
if (p1 == 0)
return (0);
i = m_gflog[p1] - m_gflog[p2];
if (i < 0)
i += 255;
if (i > 254)
i -= 255;
i = m_gfpwr[i];
return (i);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy