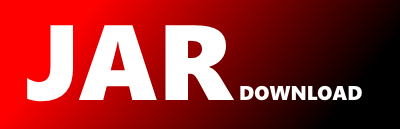
com.adobe.xfa.scripthandler.rhino.LiveObject Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.scripthandler.rhino;
import java.io.IOException;
import java.lang.reflect.Method;
import org.mozilla.javascript.Context;
import org.mozilla.javascript.Function;
import org.mozilla.javascript.FunctionObject;
import org.mozilla.javascript.Scriptable;
import org.mozilla.javascript.ScriptableObject;
import org.mozilla.javascript.Undefined;
import com.adobe.xfa.Arg;
import com.adobe.xfa.DependencyTracker;
import com.adobe.xfa.Obj;
import com.adobe.xfa.ScriptDebugger;
import com.adobe.xfa.ScriptFuncObj;
import com.adobe.xfa.ut.ExFull;
/**
* A class to expose XFA objects to JavaScript scripting.
* Each scriptable XFA objects is peered to instances of this class.
*
* @author Mike Tardif
*/
public class LiveObject extends ScriptableObject {
static final long serialVersionUID = 4351208801270519263L;
/**
* @exclude from published api.
*/
public static final String CLASSNAME = "XFAObject";
private static final Method mInvokeMethod;
static {
Method m = null;
try {
m = LiveObject.class.getMethod(
"invoke",
new Class[] { Context.class, Scriptable.class, Object[].class, Function.class });
}
catch (NoSuchMethodException ex) {
assert false; // not possible
}
mInvokeMethod = m;
}
protected transient RhinoScriptHandler moHandler;
protected transient ScriptObject moScriptObj;
protected transient Obj moXFAObject; // The XFA object this LiveObject represents
/**
* Instantiates a LiveObject object.
* The lone constructor used by this package to create
* instances of this class.
* @param handler a Rhino script handler.
* @param xfaObject the XFA object that is peered to this object.
*/
public LiveObject(RhinoScriptHandler handler, Obj xfaObject) {
jsConstructor(handler, xfaObject);
}
/**
* Defines the JavaScript constructor to Rhino.
*
* This method is required by the Scriptable interface
* and must mirror the signature of the class constructor.
*
* @param handler a Rhino script handler.
* @param xfaObject the XFA object that is peered to this object.
*
* @exclude from published api.
*/
public void jsConstructor(Object handler, Object xfaObject) {
assert (xfaObject instanceof Obj);
moXFAObject = (Obj) xfaObject;
assert (handler instanceof RhinoScriptHandler);
moHandler = (RhinoScriptHandler) handler;
}
/**
* Gets the name of the class.
*
* @exclude from published api.
*/
public String getClassName() {
return CLASSNAME;
}
/**
* Gets the value of the named property of this object.
* @param name the name of the property.
* @param start the object in which the lookup began.
* @return the value of the property, or NOT_FOUND
* if the property is not a property of this object's
* peered XFA object.
* @throws org.mozilla.javascript.EvaluatorException
* whenever an ExFull was thrown while getting the XFA
* property.
*
* @exclude from published api.
*/
public Object get(String name, Scriptable start) {
if (name.equals("Function"))
return super.get(name, start);
else if (moScriptObj != null)
return moScriptObj.get(name, start);
else {
try {
ScriptFuncObj scriptFuncObj = moXFAObject.getScriptMethodInfo(name);
if (scriptFuncObj != null) {
//
// JavaPort: This test of the version approximates what is in XFALiveObject::getIDForName.
// If the method is not valid for this XFA version, then pretend that the method wasn't found.
//
if (! moXFAObject.validateUsageFailedIsFatal(scriptFuncObj.getXFAVersion(), scriptFuncObj.getAvailability())) {
return new FunctionObject(name, mInvokeMethod, this);
}
}
DependencyTracker oDependencyTracker = moHandler.getAppModel().dependencyTracker();
Arg oArg = new Arg();
moXFAObject.getScriptProperty(oArg, name, oDependencyTracker, false, false);
resolveArg(name, start, oArg);
ScriptDebugger oDebugger = moHandler.getDebugger();
if (oDebugger != null)
oDebugger.resolvedValue(name, oArg);
return moHandler.argToVariant(oArg);
} catch (ExFull oEx) {
moHandler.throwError(oEx);
}
}
return Scriptable.NOT_FOUND;
}
/**
* Resolved the argument.
* @param name the name of the property.
* @param start the object in which the lookup began.
* @param oArg The argument.
*
* @exclude from published api.
*/
protected void resolveArg(String name, Scriptable start, Arg oArg) {
//Do nothing. This is meant to be extended by Gibson
}
/**
* Puts the value of the named property of the start object.
*
* This method may (or not) set the property in the start object.
* @param name the name of the property.
* @param start the object whose property is being set.
* @param value the value to set the property to.
*
* @exclude from published api.
*/
public void put(String name, Scriptable start, Object value) {
if (moScriptObj != null)
moScriptObj.put(name, start, value);
else {
try {
moXFAObject.setScriptProperty(name, moHandler.variantToArg(value), false);
} catch (ExFull oEx) {
moHandler.throwError(oEx);
}
}
}
/**
* @exclude from published api.
*/
public static Object invoke(Context cx, Scriptable obj, Object[] args, Function func) {
LiveObject oThis = (LiveObject) obj;
try {
DependencyTracker oDependencyTracker = oThis.moHandler.getAppModel().dependencyTracker();
Arg oRetVal = new Arg();
String sFunctionName = ((FunctionObject) func).getFunctionName();
Arg[] oArgs = new Arg[args.length];
for (int i = 0; i < args.length; i++) {
oArgs[i] = oThis.moHandler.variantToArg(args[i]);
}
oThis.moXFAObject.invokeFunction(oRetVal, sFunctionName, oArgs, oDependencyTracker, false);
ScriptDebugger oDebugger = oThis.moHandler.getDebugger();
if (oDebugger != null)
oDebugger.resolvedValue(sFunctionName, oRetVal);
return oThis.moHandler.argToVariant(oRetVal);
} catch (ExFull oEx) {
oThis.moHandler.throwError(oEx);
}
return Undefined.instance;
}
void setScriptObject(ScriptObject oScriptObj) {
moScriptObj = oScriptObj;
}
Obj getXFAObject() {
return moXFAObject;
}
/**
* This class is not really serializable, so prevent attempts to serialize from succeeding.
*/
private void writeObject(java.io.ObjectOutputStream out) throws IOException {
throw new java.io.NotSerializableException(getClass().getSimpleName());
}
}