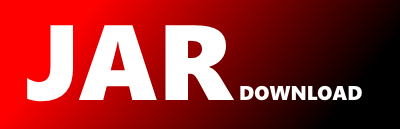
com.adobe.xfa.scripthandler.rhino.RhinoEngine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.scripthandler.rhino;
import org.mozilla.javascript.Context;
import org.mozilla.javascript.ClassShutter;
import org.mozilla.javascript.ContextFactory;
import org.mozilla.javascript.EvaluatorException;
import org.mozilla.javascript.Scriptable;
import org.mozilla.javascript.ScriptableObject;
/**
* A class to access instances of the Rhino JavaScript engine.
*
* @author Mike Tardif
*/
public abstract class RhinoEngine {
/**
* Creates a Javascript Context which is thread local. It also:
*
* - disables Rhino's LiveConnect feature,
*
- sets the desired JavaScript language.
*
*/
public static Context getThreadLocalRuntimeContext(){
Context ctx = Context.getCurrentContext();
if (null == ctx){
ctx = new ContextFactory().enterContext();
// Disable all LiveConnect features.
ctx.setClassShutter(new ClassShutter() {
public boolean visibleToScripts(String name) {
if (name.equals("org.mozilla.javascript.EvaluatorException"))
return true;
return false;
}
});
//
// Trying to sync with version of EScript and ExtentScript
// is near impossible. Use best guest for now. This
// Rhino engine tracks the Acrobat EScript version.
//
ctx.setLanguageVersion(Context.VERSION_1_7);
if (null != moThreadLocalScriptableObject.get()){
ScriptableObject initializedScriptableObject = (ScriptableObject)ctx.initStandardObjects(moThreadLocalScriptableObject.get());
moThreadLocalScriptableObject.set(initializedScriptableObject);
}
}else{
if (moThreadLocalTopLevelScopeDirtied.get()){
assert(moThreadLocalScriptableObject.get() != null);
moThreadLocalTopLevelScopeDirtied.set(false);
ScriptableObject initializedScriptableObject = (ScriptableObject)ctx.initStandardObjects(moThreadLocalScriptableObject.get());
moThreadLocalScriptableObject.set(initializedScriptableObject);
}
}
return ctx;
}
/**
* Destroys the Rhino engine.
*/
public static void destroy() {
if (null != Context.getCurrentContext()) Context.exit();
}
/**
* @exclude from published api.
*/
public RhinoEngine(){
}
/**
* Gets this engine's top level scope.
* @return the Rhino engine's top level scope object.
*/
public static Scriptable getTopLevelScope() {
getThreadLocalRuntimeContext();
assert(moThreadLocalScriptableObject != null && moThreadLocalScriptableObject.get() != null);
return moThreadLocalScriptableObject.get();
}
/**
* Sets the top level scope from the given global object.
* @param scriptableObject a scriptable object. This object
* is registered as a global object, with all other
* JavaScript global objects.
*/
public static void setTopLevelScope(ScriptableObject scriptableObject) {
moThreadLocalScriptableObject.set(scriptableObject);
moThreadLocalTopLevelScopeDirtied.set(true);
}
/**
* Throws a runtime RhinoException of the given error.
* @param sError an error message.
* @throws org.mozilla.javascript.EvaluatorException
* with the given error message.
*/
protected static void throwException(String sError) {
throw Context.throwAsScriptRuntimeEx(new EvaluatorException(sError));
}
/**
* @exclude from published api.
*/
protected static ThreadLocal moThreadLocalScriptableObject = new ThreadLocal(){
@Override
protected ScriptableObject initialValue() {
return null;
}
};
/**
* @exclude from published api.
*/
protected final static ThreadLocal moThreadLocalTopLevelScopeDirtied = new ThreadLocal(){
@Override
protected Boolean initialValue() {
return false;
}
};
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy